A C++ YAML parser allows developers to easily read and write data in YAML format, facilitating configuration management and data interchange in applications.
Here's a simple code snippet demonstrating how to use a YAML parser in C++ with the popular library `yaml-cpp`:
#include <iostream>
#include <yaml-cpp/yaml.h>
int main() {
YAML::Node config = YAML::LoadFile("config.yaml");
std::string setting = config["setting"].as<std::string>();
std::cout << "Setting: " << setting << std::endl;
return 0;
}
Make sure to link against `yaml-cpp` when compiling your code!
What is a C++ YAML Parser?
A C++ YAML parser is a tool that allows developers to read and write YAML files using the C++ programming language. YAML (YAML Ain't Markup Language) is a human-readable data serialization format commonly used for configuration files and data exchange between languages. By utilizing a C++ YAML parser, developers can efficiently manage configuration data for their applications.
Popular Libraries for C++ YAML Parsing
Two of the most noteworthy libraries for parsing YAML in C++ are yaml-cpp and libyaml:
-
yaml-cpp: This is perhaps the most widely used C++ library for YAML parsing. It’s designed to be simple and efficient, providing a high-level interface to read and write YAML documents. The yaml-cpp library offers extensive functionality for working with both basic and complex YAML data structures.
-
libyaml: This library is a lower-level C library for YAML parsing that can be interfaced with C++. While it is faster and more lightweight than yaml-cpp, it requires more detailed management from the user, making it less beginner-friendly.
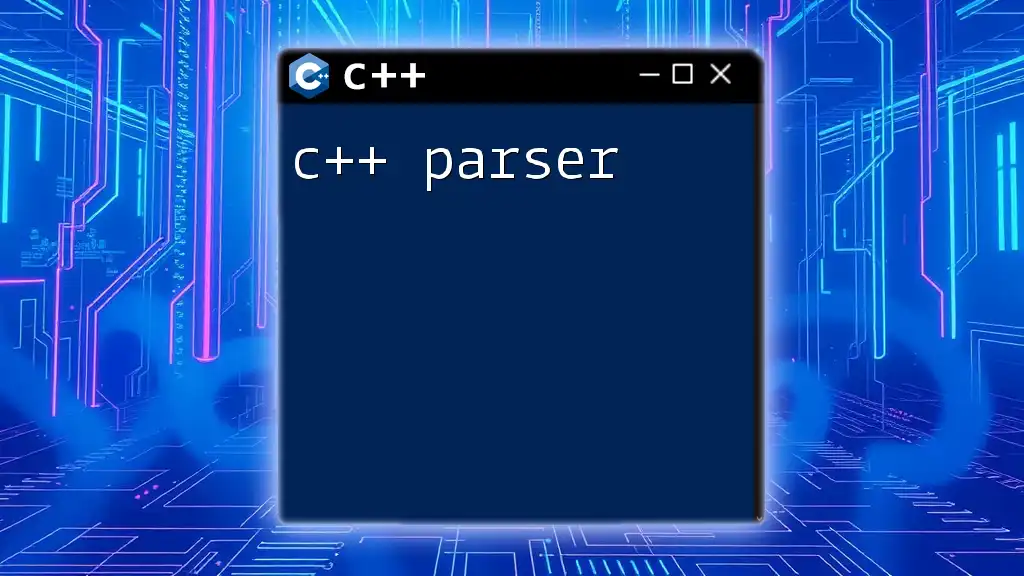
Setting Up Your C++ Environment for YAML Parsing
Installing the yaml-cpp Library
To start using a C++ YAML parser, you first need to install the yaml-cpp library. Here's how to do it across different platforms:
-
On Windows: Use a package manager like vcpkg:
vcpkg install yaml-cpp
-
On macOS: Use Homebrew:
brew install yaml-cpp
-
On Linux: Depending on your distribution, you can typically use apt:
sudo apt install libyaml-cpp-dev
Configuring Your Project
Once the library is installed, you need to configure your project to link yaml-cpp. If you are using CMake, you can include the following lines in your `CMakeLists.txt`:
find_package(yaml-cpp REQUIRED)
target_link_libraries(your_target_name yaml-cpp)
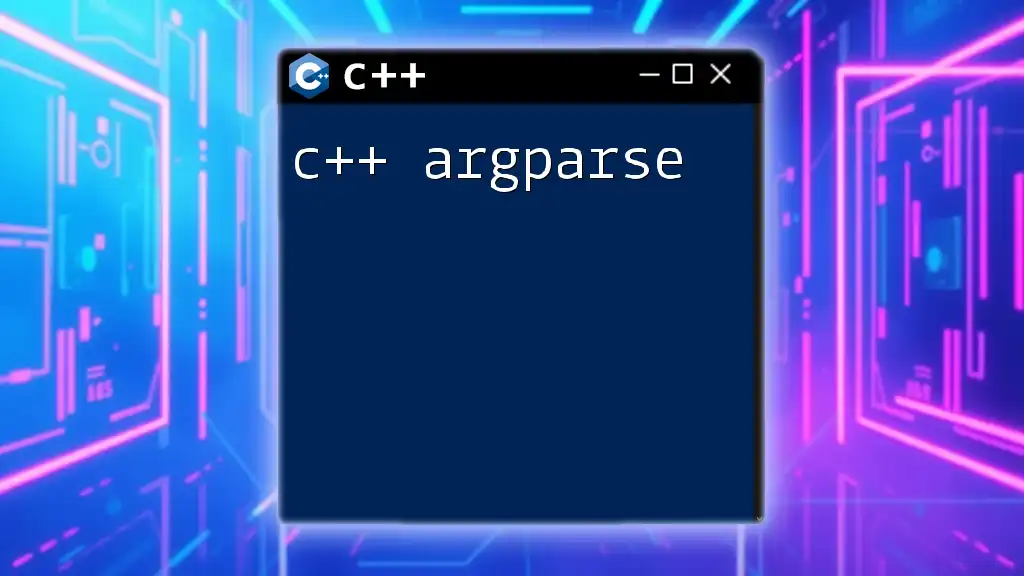
Basic Syntax and Structure of YAML
Understanding YAML syntax is crucial for effective parsing. YAML uses a structure that is easy to read, featuring:
- Scalars: Individual values like strings, integers, and floats.
- Sequences: Ordered lists, similar to arrays in C++.
- Mappings: Key-value pairs, akin to dictionaries or objects in C++.
Example of a Simple YAML Document
Here’s an example of a YAML document for a hypothetical database configuration:
database:
host: localhost
port: 3306
username: user
password: pass
Data Types in YAML
YAML supports various data types, including:
- Scalars: Strings (both quoted and unquoted), integers, and floats.
- Sequences: Represented by a list of items prefixed by hyphens.
- Mappings: Represented with key-value pairs using a colon.
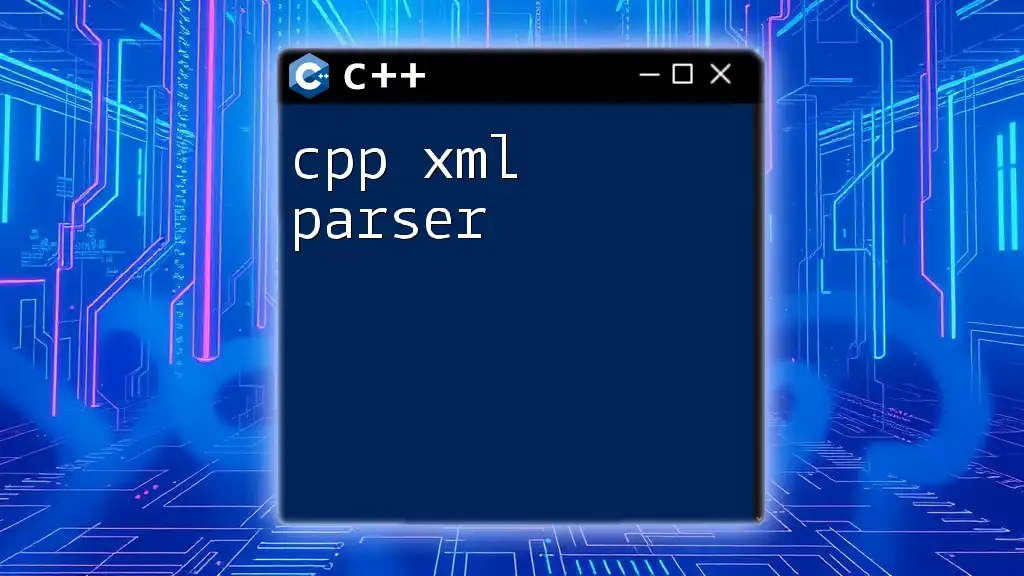
Parsing YAML in C++ Using yaml-cpp
To parse YAML in C++, you can leverage the yaml-cpp library. Here’s a simple example of reading a YAML file:
#include <yaml-cpp/yaml.h>
#include <iostream>
int main() {
YAML::Node config = YAML::LoadFile("config.yaml");
std::cout << "Database Host: " << config["database"]["host"].as<std::string>() << std::endl;
return 0;
}
Explanation of the Code
In this example:
- The `YAML::LoadFile` function is used to load a YAML file into a `YAML::Node` object.
- The values can be accessed using the key names. The `as<T>()` method is used to convert the data into the desired C++ type.
Error Handling While Parsing
When parsing YAML files, it is essential to handle potential errors. Here are common scenarios:
- File Not Found: Check if the file exists before attempting to load it.
- Invalid Format: Use try-catch blocks to catch exceptions thrown during parsing.
Example of handling errors:
try {
YAML::Node config = YAML::LoadFile("config.yaml");
} catch(const YAML::ParserException& e) {
std::cerr << "Error parsing YAML: " << e.what() << std::endl;
}
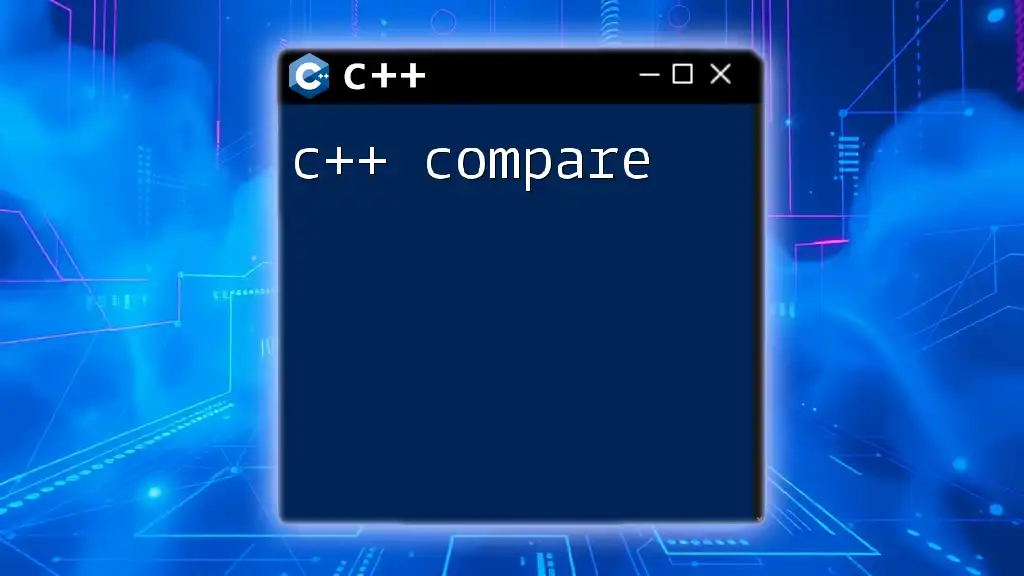
Writing YAML in C++ with yaml-cpp
You can also create and write YAML files using the yaml-cpp library. Here’s how to do it:
Creating a YAML Document
#include <yaml-cpp/yaml.h>
#include <fstream>
int main() {
YAML::Node config;
config["database"]["host"] = "localhost";
config["database"]["port"] = 3306;
std::ofstream fout("config.yaml");
fout << config;
return 0;
}
Explanation of Writing Data
In this code snippet:
- A `YAML::Node` object named `config` is created.
- Values are added to the YAML structure using simple assignment.
- The YAML document is then written to a file using an `ofstream`.
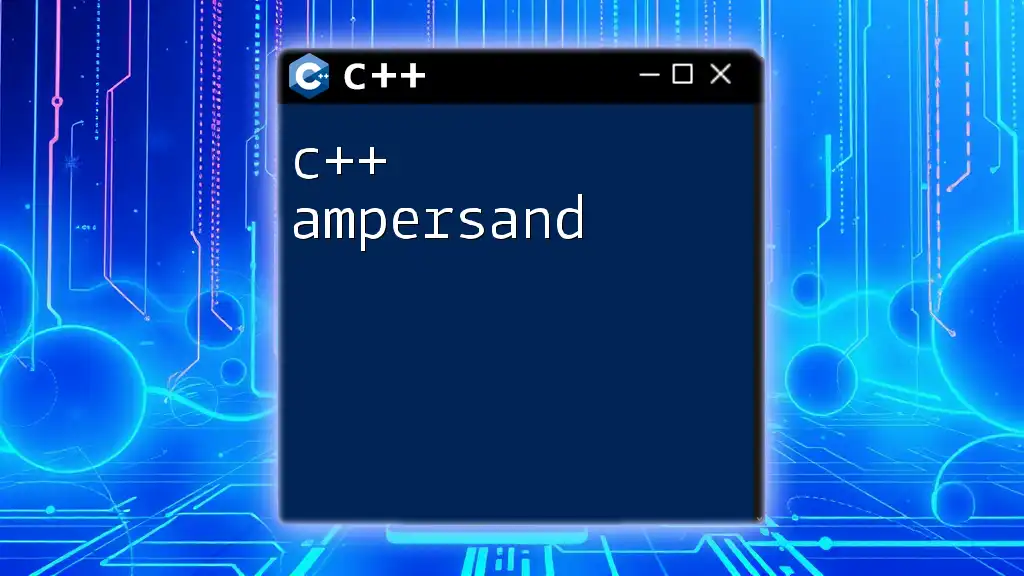
Advanced Features of C++ YAML Parsers
Custom Data Types
Handling complex data types and custom classes can be done using yaml-cpp. You can register custom serializers.
Example of serialization for a custom class:
struct Person {
std::string name;
int age;
};
namespace YAML {
template<>
struct convert<Person> {
static Node encode(const Person& person) {
Node node;
node["name"] = person.name;
node["age"] = person.age;
return node;
}
static bool decode(const Node& node, Person& person) {
person.name = node["name"].as<std::string>();
person.age = node["age"].as<int>();
return true;
}
};
}
Using Anchors and Aliases
YAML supports anchors and aliases, which can help reduce redundancy in YAML files. An example structure could be:
defaults: &defaults
host: localhost
port: 3306
development:
<<: *defaults
username: dev_user
password: dev_pass
In this example, the defaults anchor is reused in the development mapping, maintaining consistency.
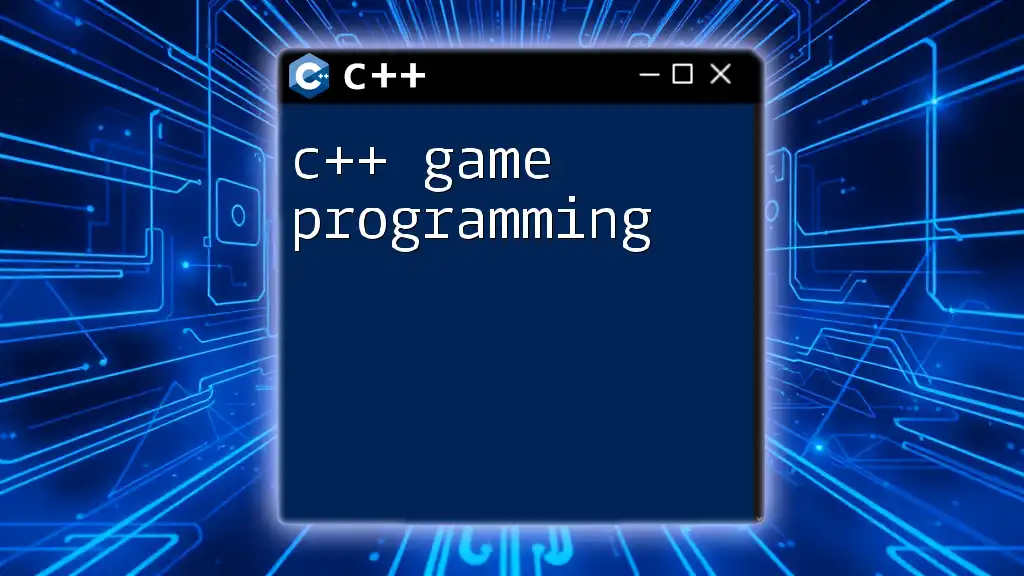
Common Use Cases for C++ YAML Parsers
Configuration Files
YAML offers a versatile format for storing configuration settings, allowing for easy updates and readability. It’s ideal for application settings, such as database configurations or feature toggles.
Data Serialization and Deserialization
In game development or web applications, YAML can be useful for saving game states or transferring data over APIs due to its lightweight nature and readability.
Interfacing with External Systems
YAML serves as a compatible format for interfacing with other systems, allowing for straightforward data exchange in a human-readable format.
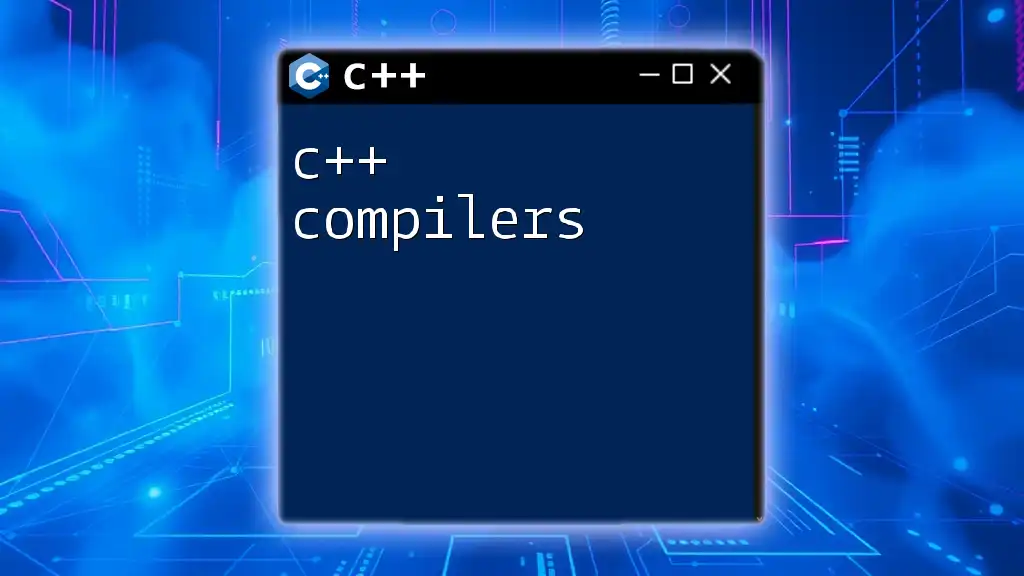
Best Practices for C++ YAML Parsing
Maintainability
Writing clear and concise YAML files is essential. Organize your YAML files using comments and consistent formatting. This will enhance readability for others and your future self.
Error Checking
Implement effective error checking both for YAML syntax and during parsing. Validate the YAML structure before runtime to catch common issues early. Ensure that your application gracefully handles any parsing errors.
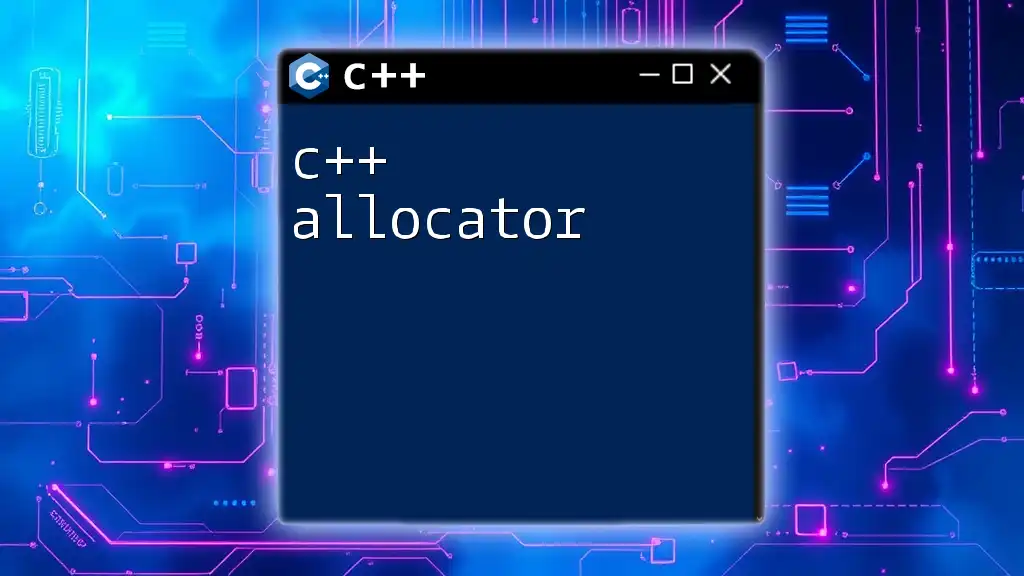
Resources for Learning More
To deepen your understanding of C++ YAML parsing, consider the following resources:
- Official Documentation: Check out the [yaml-cpp GitHub repository](https://github.com/jbeder/yaml-cpp) for tutorials and API references.
- Books and Online Courses: Look for learning materials focusing on C++ and data serialization which include YAML.
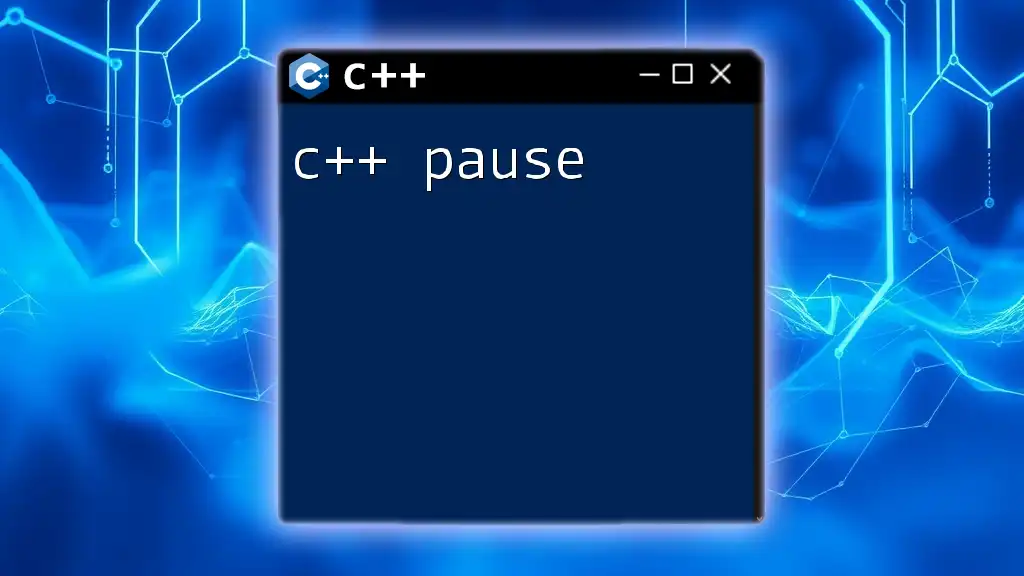
Conclusion
In summary, a C++ YAML parser like yaml-cpp significantly enhances your ability to manage YAML files within your C++ applications. Through effective parsing and writing of YAML documents, developers can leverage the strengths of this data format for configuration management and more. Experimenting with YAML in your projects will not only improve maintainability but also streamline data handling processes.
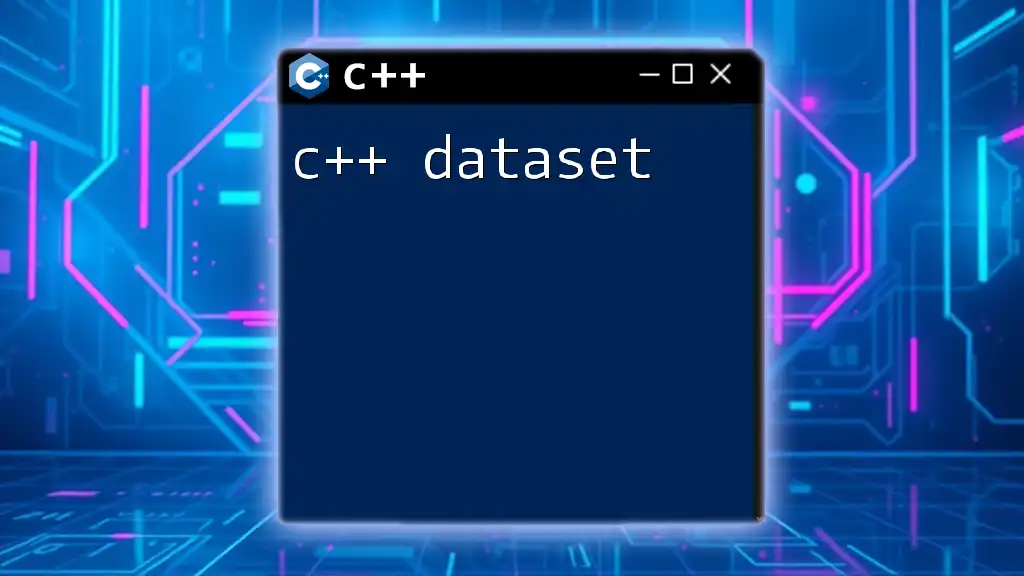
FAQs
What are some common errors when using C++ YAML parser?
Common errors include file not found exceptions, invalid format errors, and type mismatches during data extraction.
Can I use YAML for real-time applications?
Yes, YAML's simplicity and readability make it suitable for real-time applications, especially for configuration purposes, although care should be taken regarding parsing overhead.
Is yaml-cpp thread-safe?
The yaml-cpp library is not inherently thread-safe. If you plan to use it across multiple threads, ensure proper synchronization mechanisms are in place.