A C++ XML parser allows developers to easily read and manipulate XML data structures using libraries such as TinyXML or Xerces.
Here's a simple example using TinyXML to parse an XML file:
#include "tinyxml2.h"
#include <iostream>
int main() {
tinyxml2::XMLDocument doc;
doc.LoadFile("example.xml"); // Load XML file
tinyxml2::XMLElement* root = doc.FirstChildElement("RootElement"); // Get root element
if (root) {
const char* data = root->Attribute("attributeName"); // Access attribute
std::cout << "Attribute value: " << data << std::endl; // Print attribute value
}
return 0;
}
Understanding XML Structure
XML (eXtensible Markup Language) is a flexible text format derived from SGML (Standard Generalized Markup Language). It is primarily designed to transport and store data, making it a common choice for data interchange among web services and applications.
XML documents consist of a hierarchical structure, which organizes data in a tree-like format composed of nodes. Each node can represent elements, attributes, and text. Understanding this structure is crucial for effective parsing.
Basics of XML Syntax
An XML document is built using a combination of user-defined tags. Here is a simple XML snippet that illustrates the structure:
<person age="30">
<name>John Doe</name>
<email>johndoe@example.com</email>
</person>
In this example, `<person>` is the root element, which has an attribute `age`. The `<name>` and `<email>` tags are child elements containing character data.
Hierarchical Structure of XML
XML represents data using a tree structure, where each element can have child elements, forming parent-child relationships. This hierarchy is vital for navigating through the XML data. Success in parsing relies on understanding how to traverse this structure effectively.
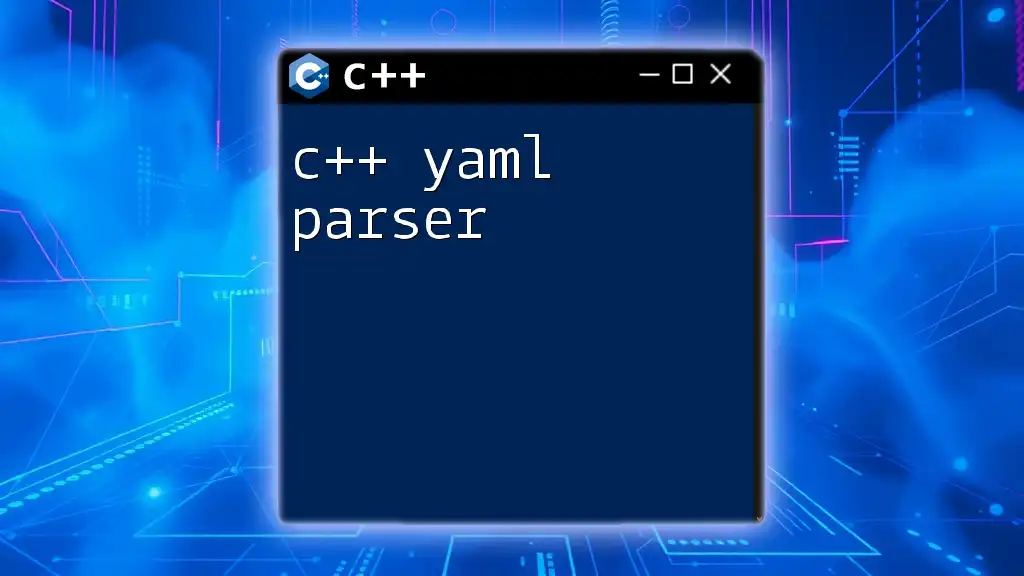
C++ Libraries for XML Parsing
When implementing a C++ XML parser, several libraries offer varying functionalities. Among the most popular are:
- TinyXML: This is lightweight and easy to use, making it ideal for smaller applications.
- libxml2: A powerful library known for its performance, it works well with large XML files but has a steeper learning curve.
- pugixml: Designed for rapid development and ease of use, pugixml combines speed and functionality.
Choosing the right library depends on the project requirements, such as performance needs and user experience.
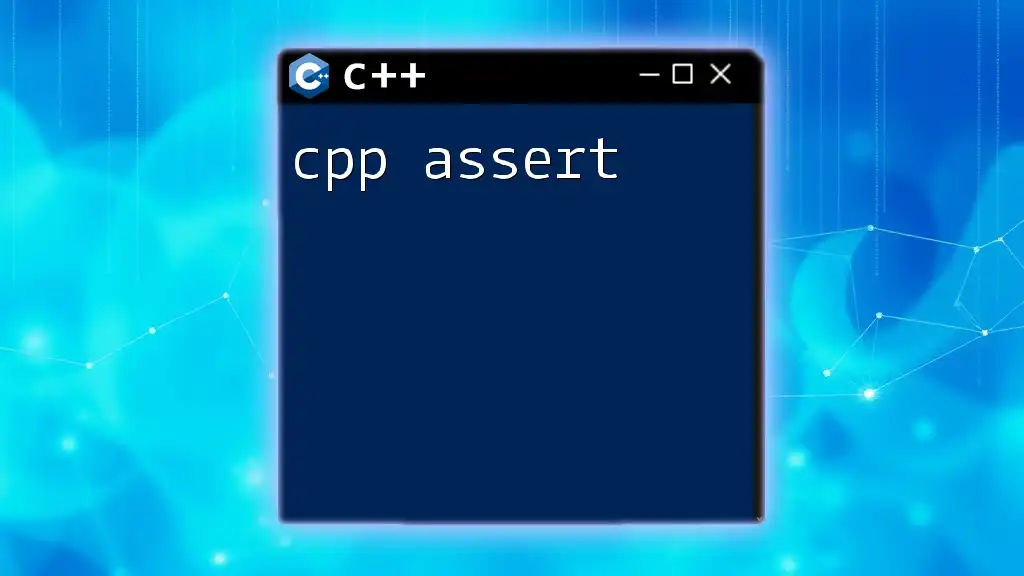
Setting Up the Environment
Getting started with XML parsing in C++ involves setting up the appropriate library. For this guide, we will use pugixml as our main library due to its balance of performance and ease of use.
Installing the XML Parser Library
Download the pugixml library from its official site or GitHub repository. You can either download the precompiled binaries or the source code.
Configuring Your Project
Once installed, include the pugixml header files in your project. If you're using an IDE like Visual Studio or Code::Blocks, ensure to link against the pugixml library. Your project should be able to recognize pugixml's classes and methods seamlessly.
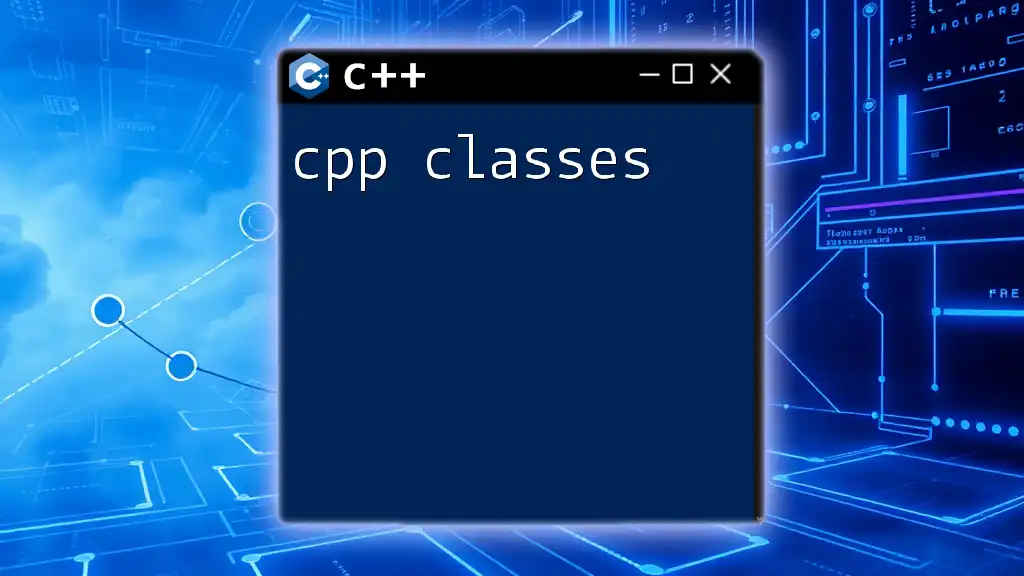
Basic XML Parsing with C++
Now that the environment is set up, let's look at how to parse an XML file. The fundamental operations include loading an XML document, navigating it, and extracting values.
Reading XML Files
To read an XML file using pugixml, you first create a `xml_document` object and load your file. Here’s how:
pugi::xml_document doc;
pugi::xml_parse_result result = doc.load_file("example.xml");
if (!result) {
std::cerr << "XML parsed with errors, attr value: "
<< doc.child("person").attribute("age").value() << "\n";
}
In this code snippet, you load an XML file called `example.xml`. If parsing fails, an error is logged, alerting you to potential issues.
Navigating XML Nodes
After successfully loading an XML document, you can navigate through its elements. For instance, to access the name of the person:
pugi::xml_node person = doc.child("person");
const char* name = person.child("name").text().as_string();
std::cout << "Name: " << name << std::endl;
This snippet shows how to access the `<name>` child element of the `<person>` node and output its text content.
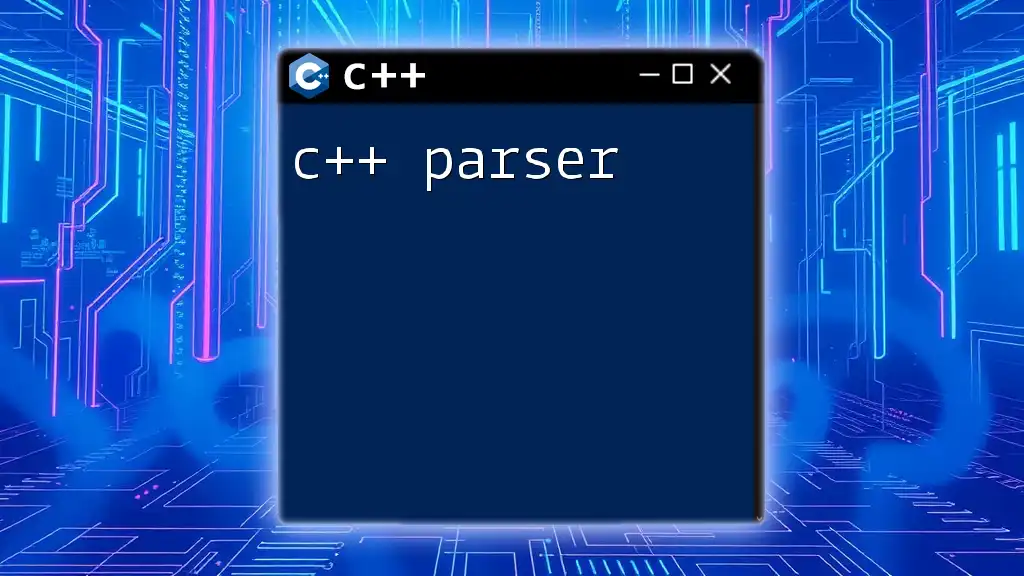
Advanced XML Parsing Techniques
Once you're comfortable with the basics, you can explore more advanced parsing techniques.
Handling Attributes
In many XML files, elements may include attributes that convey additional information. For example, to retrieve the age attribute from the `<person>` element:
int age = person.attribute("age").as_int();
std::cout << "Age: " << age << std::endl;
This code extracts the `age` attribute, providing a straightforward mechanism to access attributes on nodes.
Modifying XML Content
Using pugixml, you can also modify the loaded XML data. For instance, adding a new element:
person.append_child("newElement").text().set("value");
This script adds a child named `<newElement>` with a value of "value" to the `<person>` node.
Writing XML Files
After modifications, saving the updated XML back to a file is just as easy:
doc.save_file("modified.xml");
This command rewrites the document to `modified.xml`, preserving any changes you made during the session.
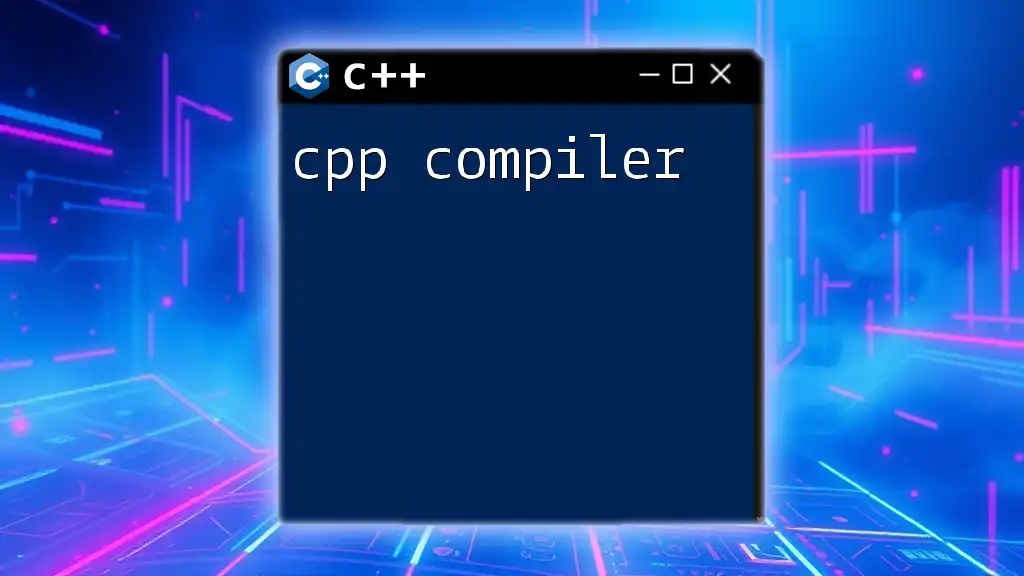
Error Handling in XML Parsing
When working with XML data, error handling is crucial to ensure robustness:
Common Parsing Errors
Common issues include malformed XML or unexpected structure. To manage these errors, pugixml provides error codes when loading files, which allows you to identify and rectify issues easily.
Using Error Codes
By checking the error codes returned during parsing, you can implement interactions such as logging errors or prompting the user for correct input. For example:
if (result.status != pugi::status_ok) {
std::cerr << "Error loading XML file: " << result.description() << "\n";
}
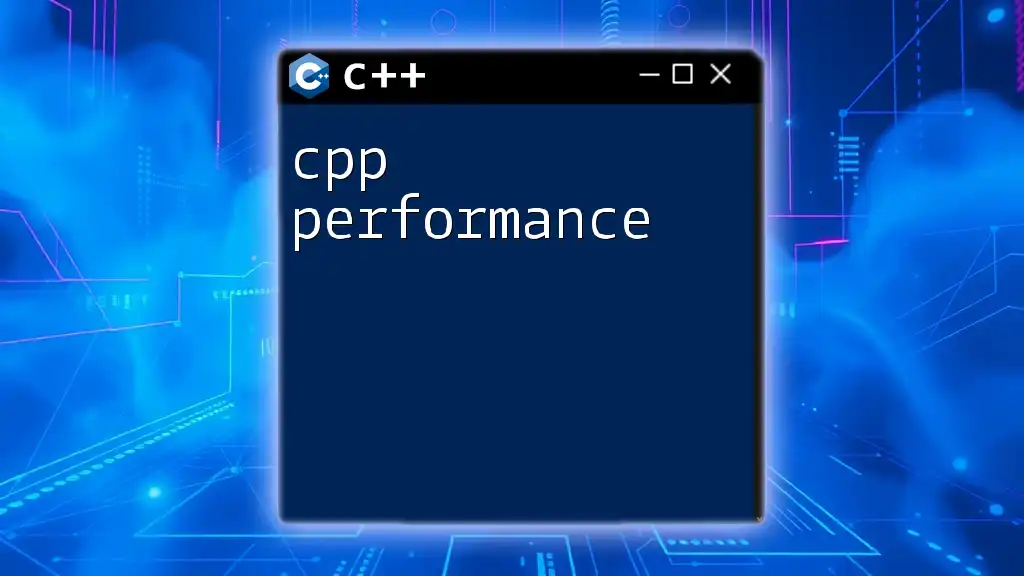
Practical Applications of XML Parsing in C++
The applications of XML parsing in real-world scenarios are vast:
Use Cases for XML Parsing
Common use cases include reading configuration files, parsing server responses in web services, and handling serialized data in applications.
Integrating XML Parsing in Larger C++ Projects
When integrating XML parsing components into larger C++ projects, organize the parser into functions or classes to encapsulate functionality. This organization promotes reusability and eases maintenance.
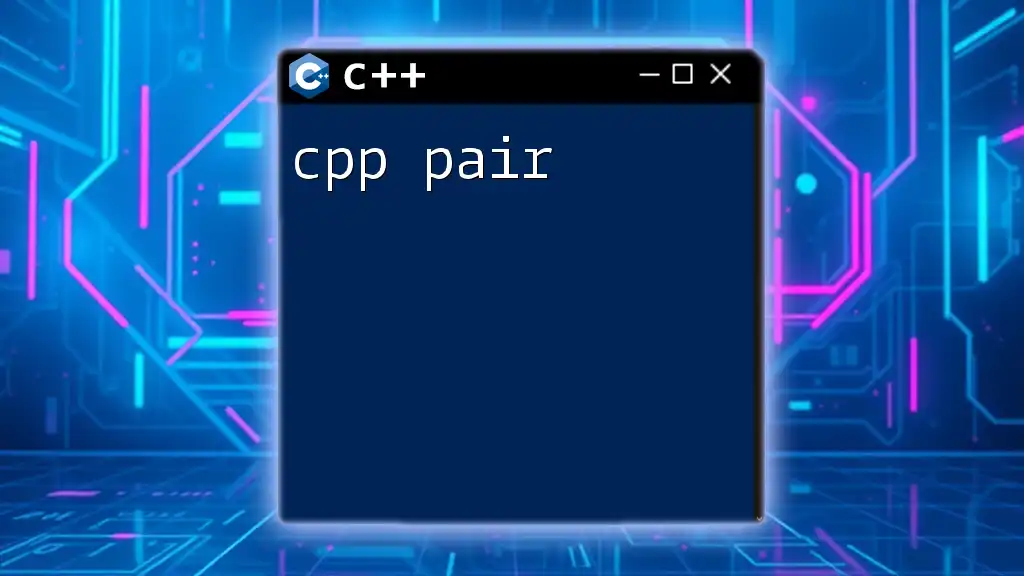
Conclusion
In summary, utilizing a C++ XML parser empowers developers to efficiently handle, modify, and understand XML data structures. Mastering XML parsing opens up numerous possibilities in various applications, from web development to data management.
Encouragement to Experiment
I encourage you to explore the libraries discussed, experiment with code snippets provided, and practice effective XML parsing techniques. Your hands-on experience will deepen your understanding and proficiency in this essential skill.
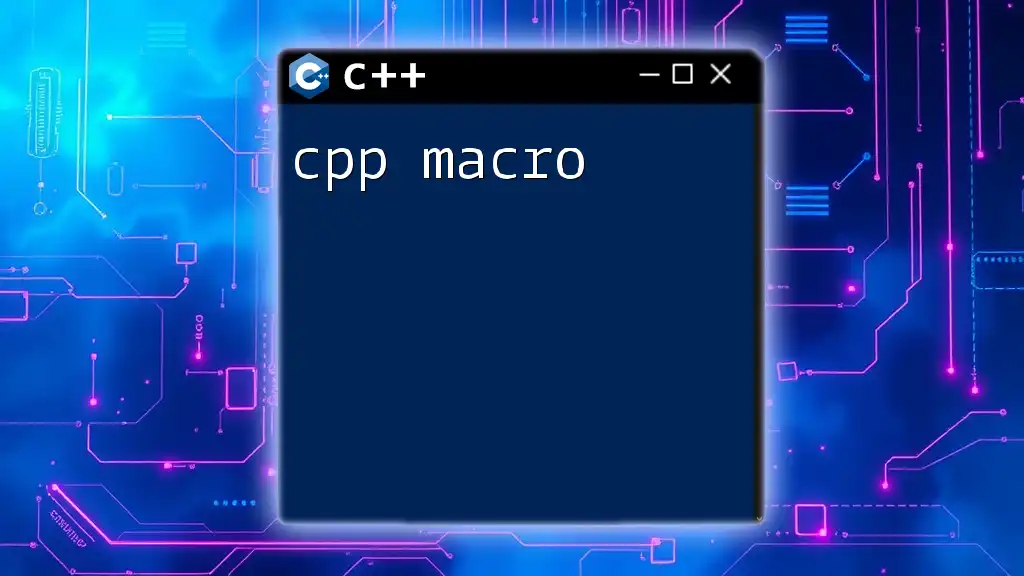
Additional Resources
For those looking to delve deeper into the subject, explore further reading materials, tutorial content, and community forums dedicated to C++ programming and XML parsing. Engaging with others can provide valuable insights and support as you continue your learning journey.