`argparse` in C++ is a library that simplifies command-line argument parsing, allowing developers to easily define and manage command-line options for their applications.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <argparse/argparse.hpp>
int main(int argc, const char* argv[]) {
argparse::ArgumentParser program("example");
program.add_argument("input")
.help("input file");
program.add_argument("--verbose", "-v")
.help("increase output verbosity")
.default_value(false)
.implicit_value(true);
try {
program.parse_args(argc, argv);
} catch (const std::runtime_error& err) {
std::cerr << err.what() << std::endl;
return 1;
}
std::string input = program.get<std::string>("input");
bool verbose = program.get<bool>("--verbose");
std::cout << "Input file: " << input << std::endl;
if (verbose) {
std::cout << "Verbose mode is on." << std::endl;
}
return 0;
}
Understanding Command-Line Arguments
Command-line arguments are the values and parameters passed to a program when it is executed from the command line interface (CLI). Unlike function parameters within the program, these arguments provide essential inputs that define how the program should behave. For instance, a command-line application that processes files may require the user to specify filenames and options directly via the command line, allowing for greater flexibility.
Using C++ argparse simplifies this process by allowing programmers to efficiently manage and parse these command-line inputs. Unlike manual parsing methods, which can be cumbersome and error-prone, argparse provides a high-level interface to define expected arguments clearly, handle their types, and manage help documentation automatically.
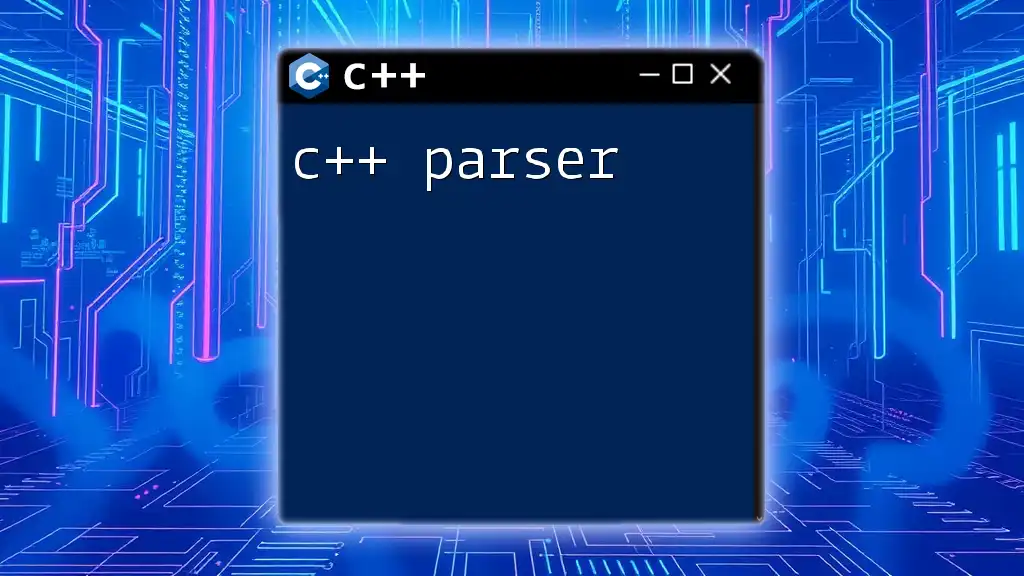
Getting Started with C++ Argparse
To start using C++ argparse, you first need to install the library. It’s commonly available through various package managers. For example, if you are using vcpkg, you can install it using the following command:
vcpkg install argparse
Incorporate the library into your project by including the relevant header file. Here's an overview of how your basic argparse program structure will look:
#include <iostream>
#include "argparse.hpp"
int main(int argc, char *argv[]) {
argparse::ArgumentParser program("example");
}
This code initializes a new instance of the `ArgumentParser`, which you will build upon.
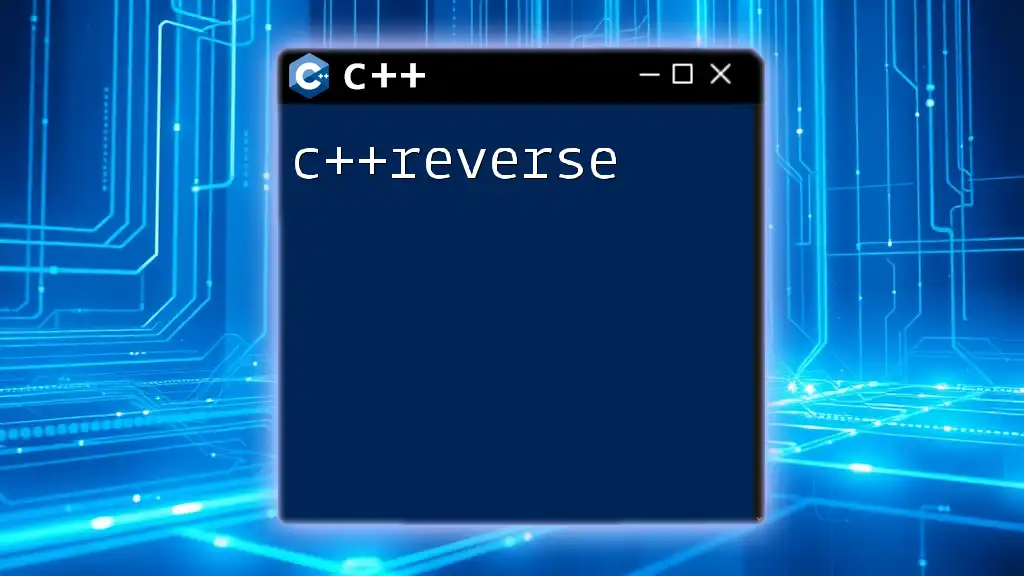
Creating Your First Argument Parser
Setting Up the Argument Parser
Once you have set up your argument parser, you can begin adding the necessary arguments for your application. The following segment demonstrates how to define arguments:
program.add_argument("input")
.help("Input file to process");
This command establishes a positional argument named "input", which is mandatory when the program is executed. Users must provide this argument or else they will encounter an error.
Adding Arguments
Optional arguments can enhance user flexibility. For instance:
program.add_argument("-o", "--output")
.help("Output file name")
.default_value("default_output.txt");
In this example, we’ve created an optional argument that allows the user to specify an output file. If the user doesn't provide it, the program defaults to "default_output.txt".
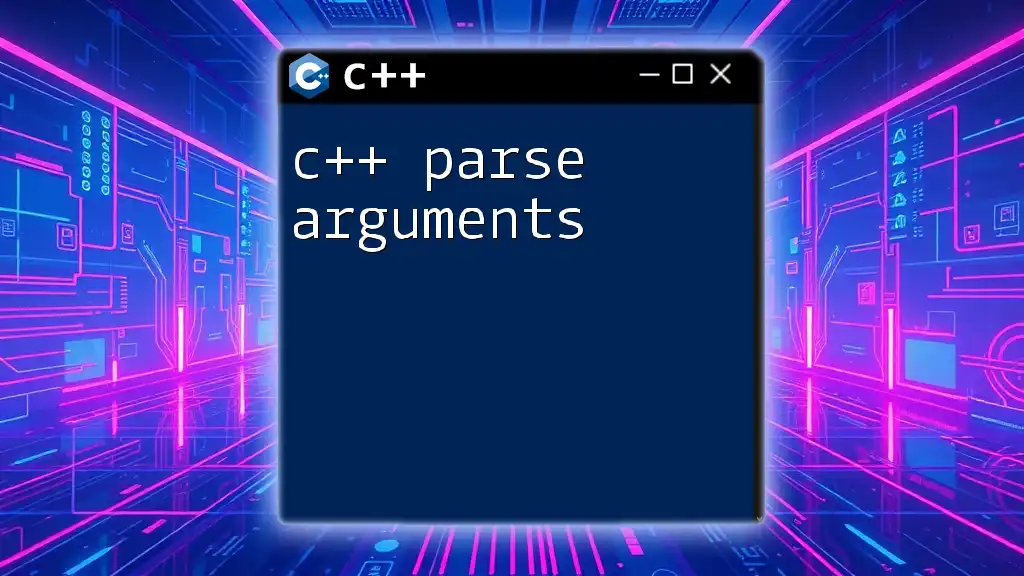
Advanced Argument Configuration
Setting Argument Types
C++ argparse allows you to enforce data types on arguments, enhancing input validation. For an integer argument, you would write:
program.add_argument("-n", "--number")
.help("An integer value")
.scan<'i', int>();
This command tells the parser to expect an integer when the `-n` or `--number` option is used.
For a floating-point number, use:
program.add_argument("-d", "--decimal")
.help("A decimal value")
.scan<'g', double>();
Implementing type checks helps prevent erroneous inputs, ensuring that the program behaves as expected.
Mutually Exclusive Arguments
Sometimes, you may want to restrict users from providing conflicting options. The `add_mutually_exclusive_group` function allows you to achieve this:
program.add_mutually_exclusive_group()
.add_argument("-v", "--verbose")
.help("Enable verbose output")
.default_value(false)
.implicit_value(true);
program.add_argument("-q", "--quiet")
.help("Suppress all output");
In this setup, the user can choose either verbose logging or a quiet mode, but not both.
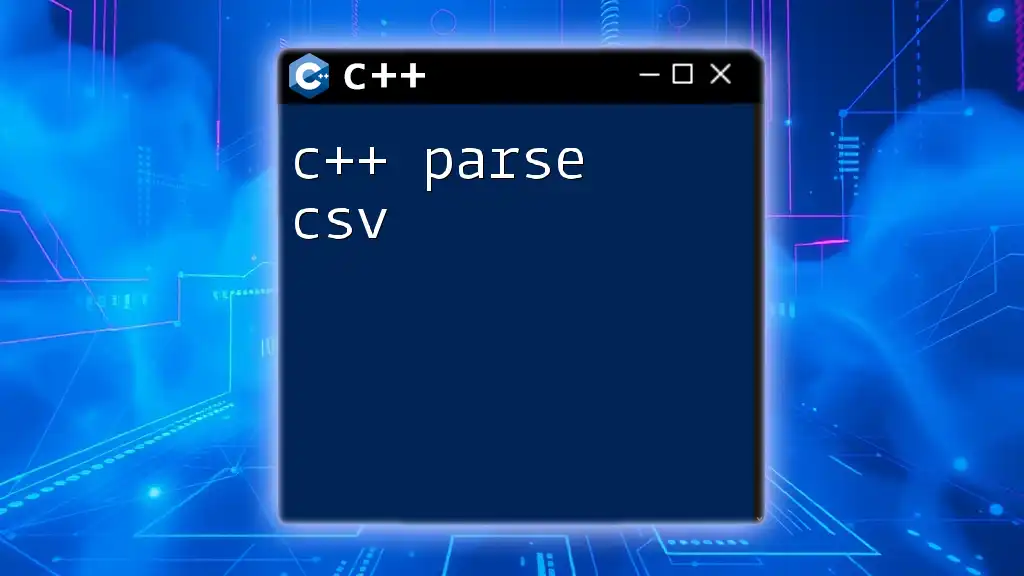
Customizing Help Messages
Another powerful feature of C++ argparse is the ability to customize help messages. This can significantly improve user experience. You can print the help output using a simple command:
program.print_help();
Additionally, you can add a program description and version information:
program.description("This program processes input files.");
program.version("0.1");
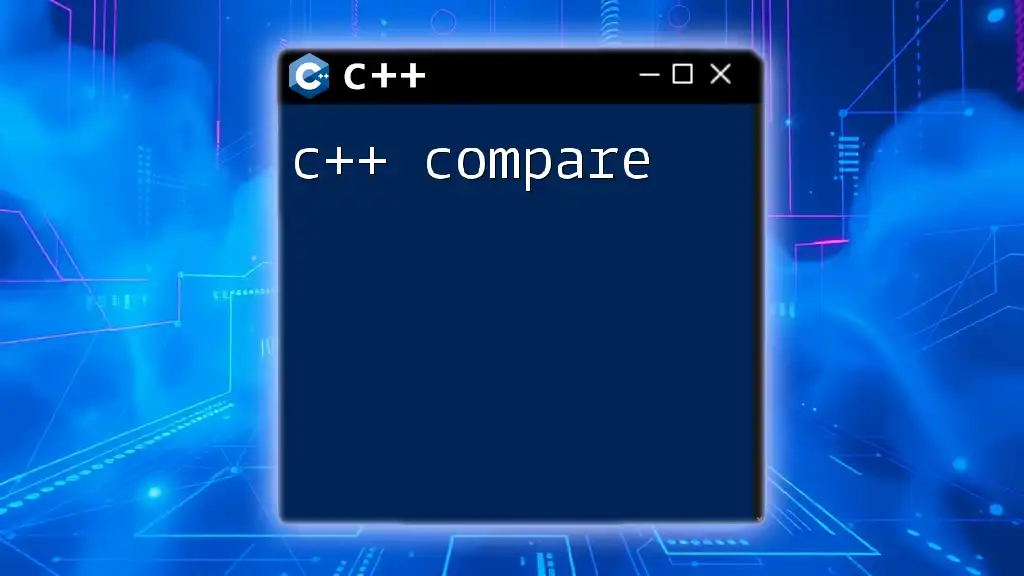
Parsing and Handling Arguments
After defining your arguments, you will need to parse them. The following snippet illustrates how to handle this smoothly:
try {
program.parse_args(argc, argv);
} catch (const std::runtime_error &err) {
std::cerr << err.what() << std::endl;
return 1;
}
This code not only attempts to parse the command line but also catches any parsing errors, providing user-friendly feedback.
Accessing Parsed Values
To effectively use your parsed arguments, retrieve values with ease:
std::string input_file = program.get<std::string>("input");
bool verbose = program.get<bool>("--verbose");
This streamlined access allows the program to use the provided arguments directly in your logic.
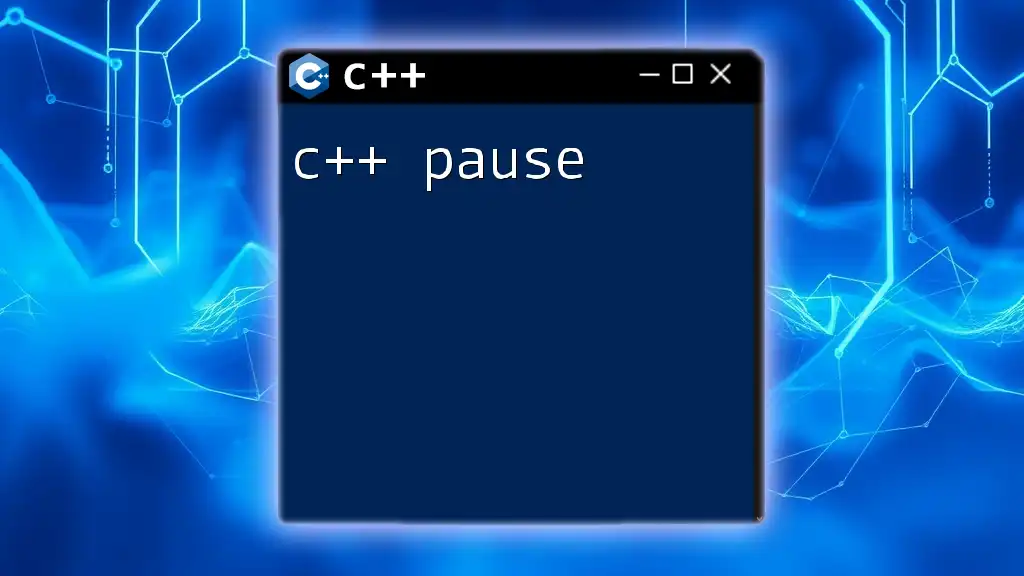
Error Handling and Validation
Handling parsing errors gracefully is crucial for a good user experience. Common issues might include missing required arguments or providing unexpected input types. Implement checks to ensure that the program exits cleanly or prompts the user for correct input.
Moreover, validating input data, such as checking the existence of specified files or directories, enhances the robustness of your application, ensuring users have a smooth experience.
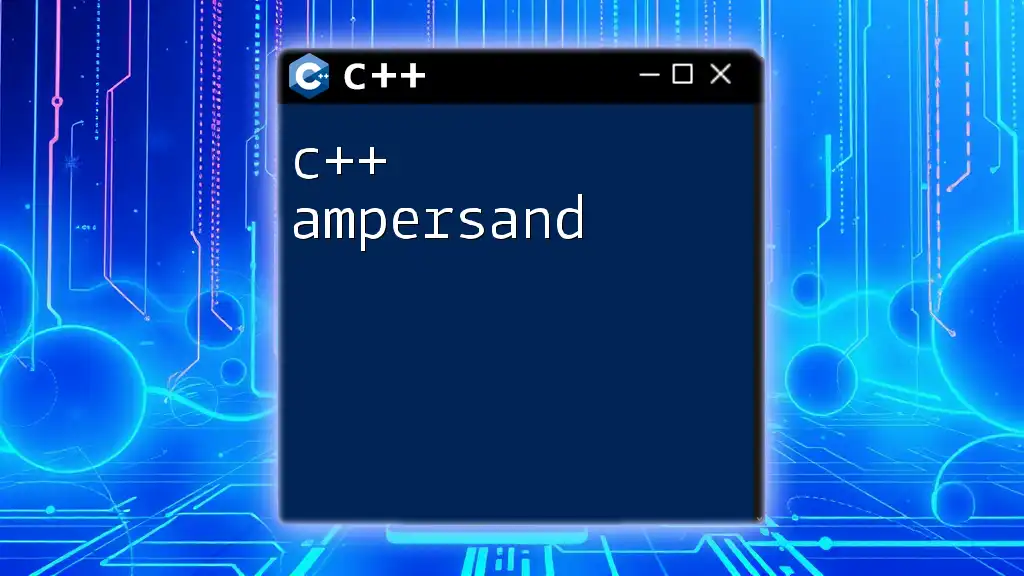
Use Cases for C++ Argparse
The practical applications of C++ argparse are vast. Many command-line tools, including file processors, data analysis utilities, and configuration management systems, can benefit from implementing argparse due to the ease of managing complex command-line interfaces.
In particular, tools needing various input formats or options can leverage C++ argparse’s features to maintain a user-friendly experience while managing complex backend functionality.
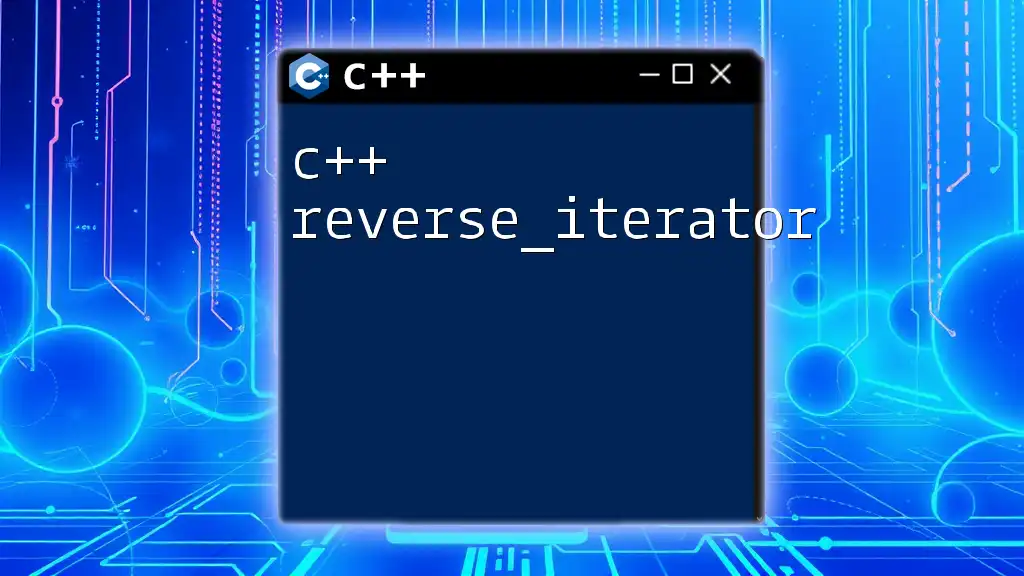
Conclusion
In summary, C++ argparse streamlines the process of managing command-line arguments, making it a compelling choice for developers looking to enhance their CLI tools. Its ease of use, advanced features, and built-in help management empower programmers to create robust applications efficiently.
Further Learning Resources
For deeper dives into C++ argparse, explore the official documentation and additional reading materials, which provide comprehensive guides as well as best practices to utilize this library to its fullest potential.
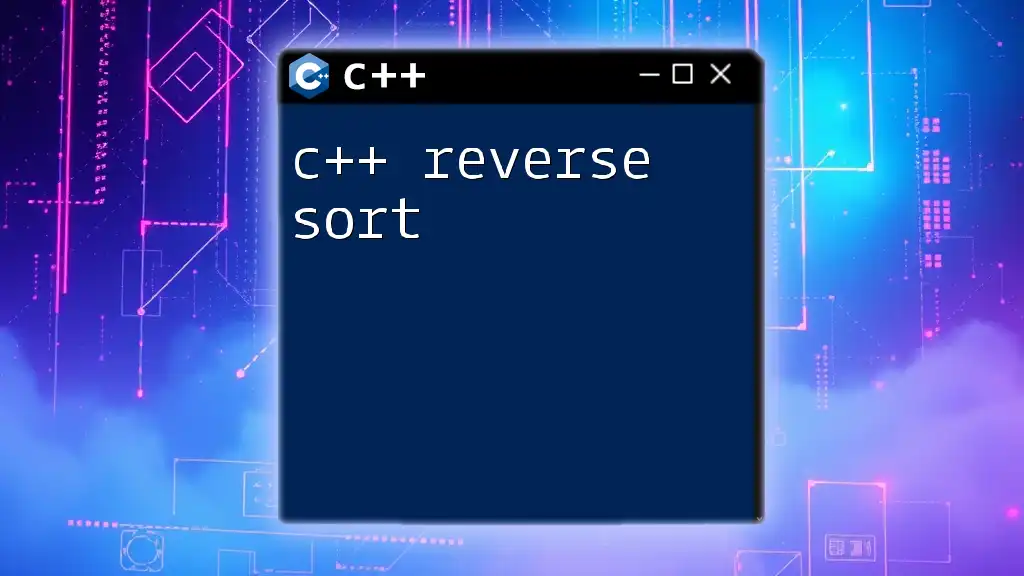
Call to Action
Now that you understand the capabilities of C++ argparse, why not take the plunge? Create your command-line application using these techniques, and share your experience and insights. Feedback and shared knowledge help foster a vibrant developer community!