A C++ reverse iterator allows you to traverse a container in reverse order, providing a convenient way to access elements from the end to the beginning.
Here’s a simple code snippet demonstrating the use of a reverse iterator with a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto rit = vec.rbegin(); rit != vec.rend(); ++rit) {
std::cout << *rit << " ";
}
return 0;
}
What is an Iterator?
An iterator is a fundamental concept in C++ that provides a way to traverse elements in a collection, such as arrays or containers. Iterators abstract away the details of the underlying data structure and enable developers to work with various collections uniformly. This allows algorithms to be applied across different data types without needing to understand their specifics.
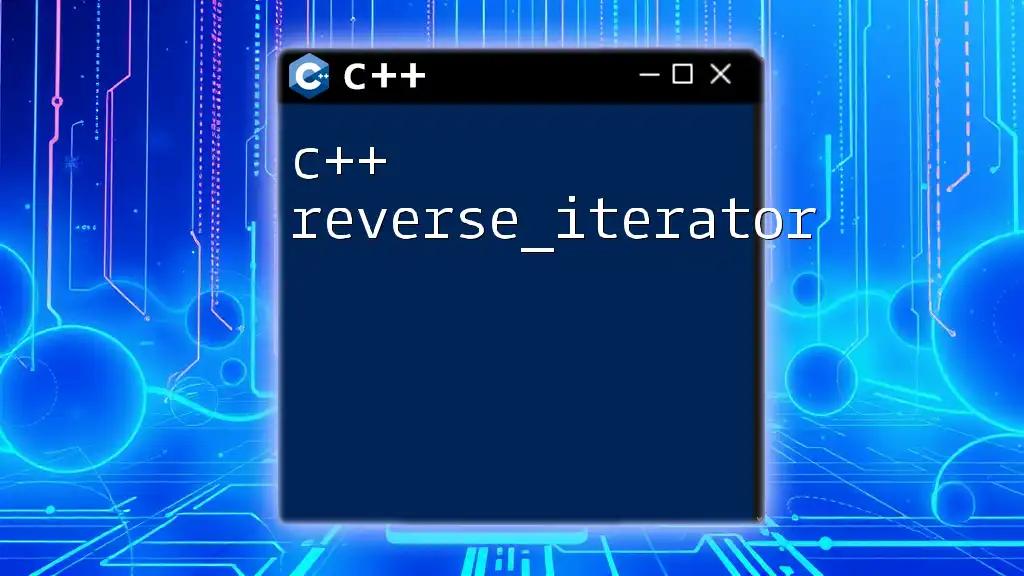
Understanding Reverse Iterators
Definition of Reverse Iterator
A reverse iterator is a type of iterator that traverses a collection in the opposite direction. When using a reverse iterator, the last element of the collection is accessed first, and the first element is accessed last. This mechanism is highly beneficial for scenarios where you might want to process or evaluate elements starting from the end of a container.
Difference between Normal and Reverse Iterators
The primary distinction between normal iterators and reverse iterators is their directionality. While normal iterators move from the beginning of a collection to the end, reverse iterators move from the end to the beginning. This is codified in their API with functions like `rbegin()` and `rend()`.
Use Cases for Reverse Iterators in C++
Reverse iterators can be particularly useful when:
- You want to analyze data from the last element back to the first.
- You aim to avoid modifying the underlying collection during iteration yet want to perform operations backward.
- You're implementing algorithms that are inherently defined to work in reverse order, such as sorted data analyses.
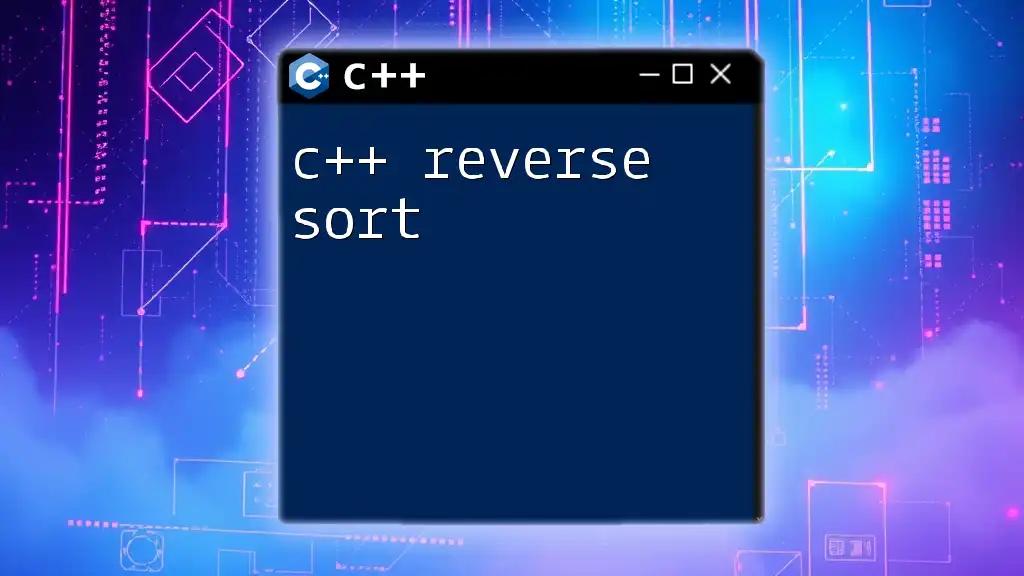
Basic Concepts of Reverse Iterators
The rbegin() and rend() Functions
To utilize reverse iterators in C++, you primarily use the `rbegin()` and `rend()` member functions.
- `rbegin()` returns a reverse iterator pointing to the last element of the collection.
- `rend()` returns a reverse iterator pointing to one position before the first element.
Example Code Snippet: Simple usage of `rbegin()` and `rend()`
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Reverse vector traversal: ";
for (auto it = vec.rbegin(); it != vec.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, the program sweeps through a vector in reverse order, printing elements from last to first.
Common Containers with Reverse Iterators
Vector
Reverse iterators work seamlessly with the `std::vector`, giving developers the ability to traverse its contents backward.
Example Code Snippet: Iterating through a vector in reverse
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::cout << "Vector elements in reverse: ";
for (auto it = vec.rbegin(); it != vec.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
List
`std::list` is another container that allows for reverse iteration. Using reverse iterators with lists can be advantageous due to their bidirectional nature, which supports efficient insertions and deletions.
Example Code Snippet: Reverse iteration in a list
#include <iostream>
#include <list>
int main() {
std::list<std::string> strList = {"one", "two", "three", "four"};
std::cout << "List elements in reverse: ";
for (auto it = strList.rbegin(); it != strList.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Deque
The `std::deque` container can also utilize reverse iterators, permitting similar functionality as with vectors and lists.
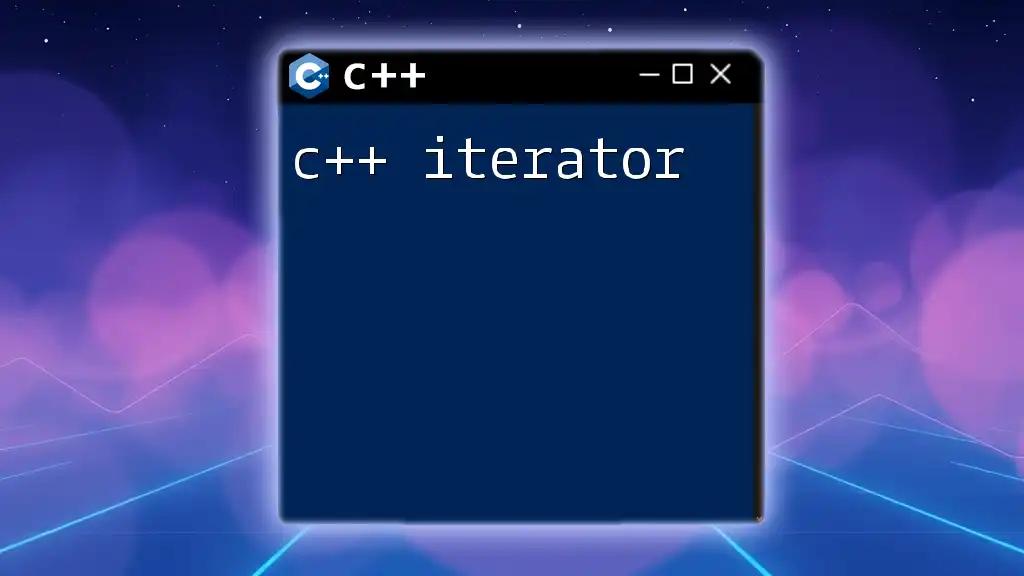
Practical Applications of Reverse Iterators
Traversing Collections in Reverse
One of the most straightforward applications of reverse iterators is to traverse collections backward. This can be particularly useful in various programming scenarios, such as displaying items in reverse order or processing a stack of results.
Code Example: Traversing a vector and printing elements in reverse
#include <iostream>
#include <vector>
int main() {
std::vector<char> charVec = {'A', 'B', 'C', 'D', 'E'};
std::cout << "Characters in reverse order: ";
for (auto it = charVec.rbegin(); it != charVec.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Modifying Elements in Reverse
When using reverse iterators, modifications can be performed safely; however, care should be taken not to invalidate the iterator. You can alter elements during the backward traversal, ensuring the integrity of the data structure remains intact.
Code Example: Changing values of elements in a vector using reverse iterators
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (auto it = nums.rbegin(); it != nums.rend(); ++it) {
*it += 2; // Increment each element by 2
}
std::cout << "Modified elements: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
return 0;
}
Filtering Collections in Reverse
Reverse iterators can also be instrumental in filtering elements. For instance, when iterating backward, you may choose to remove certain elements and necessitate a check against the entire collection.
Example Code Snippet: Removing elements from a vector using reverse iterators
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> data = {10, 15, 20, 25, 30};
for (auto it = data.rbegin(); it != data.rend(); ) {
if (*it > 20) {
it = decltype(it)(data.erase((++it).base())); // Remove elements greater than 20
} else {
++it;
}
}
std::cout << "Remaining elements: ";
for (const auto& num : data) {
std::cout << num << " ";
}
return 0;
}

Advanced Techniques with Reverse Iterators
Creating Custom Reverse Iterators
For specialized use cases, you might find the need to create your custom reverse iterator. This is typically achieved by inheriting from standard iterators and overriding necessary functions.
Code Example: Creating a custom reverse iterator class
#include <iostream>
#include <vector>
#include <iterator>
template<typename T>
class CustomReverseIterator : public std::reverse_iterator<T> {
public:
CustomReverseIterator(T iter) : std::reverse_iterator<T>(iter) {}
// Example of a custom function
void customLogic() {
// Custom behavior can be defined here.
}
};
// Usage
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
CustomReverseIterator<std::vector<int>::iterator> it(nums.end());
for (; it != nums.rbegin(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Combining with Algorithms in STL
You can use `std::reverse_iterator` with Standard Template Library (STL) algorithms to achieve functionality that might not be immediately obvious. For instance, finding the maximum value in a reversed order is straightforward using `std::max_element`.
Code Example: Finding a maximum value using `std::max_element` in reverse
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {5, 3, 8, 9, 1};
auto maxElement = std::max_element(nums.rbegin(), nums.rend());
std::cout << "Maximum element in reverse: " << *maxElement << std::endl;
return 0;
}
Performance Considerations
When using iterators, performance is a crucial factor. In terms of memory usage, reverse iterators are lightweight, as they do not hold additional data. However, keep in mind that the choice of iterator can lead to performance variations depending on the underlying container—some operations in linked lists and vectors, for example, differ significantly.
Performance comparison: Regular vs. reverse iterators
In most cases, the performance difference between regular and reverse iterators is negligible. However, understanding your data structure ensures optimal choices, such as employing reverse iterators for bidirectional traversals.
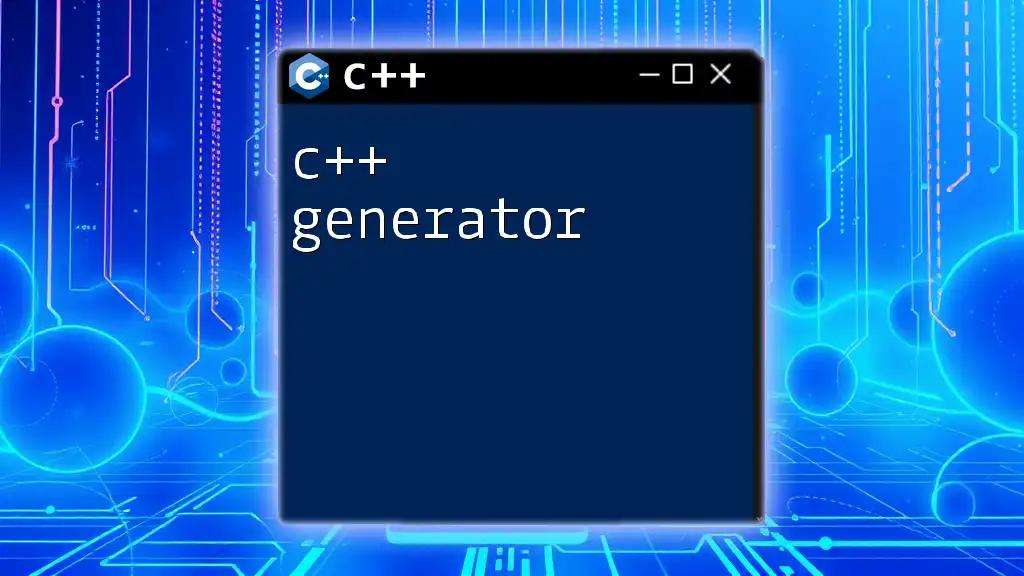
Best Practices
When to Use Reverse Iterators
Reverse iterators are beneficial when:
- You are dealing with algorithms or applications that require reverse processing.
- There is a need to avoid modifying the central part of the container during iteration.
- You want to cleanly and efficiently express backward operations in code.
Common Pitfalls to Avoid When Using Reverse Iterators
When utilizing reverse iterators, be cautious of:
- Modifying the underlying container while traversing it, which may invalidate the iterator.
- Assuming reverse iterators work on all STL containers—the behavior may vary based on the properties of the container.
Code Readability and Maintainability
Whenever you implement reverse iterators in your code, prioritize clarity. Using meaningful variable names, adding comments, and maintaining straightforward logic helps future maintainers (including you) understand your code better.

Conclusion
C++ reverse iterators offer a powerful way to traverse collections in reverse order, enhancing the flexibility of your programming tools. By mastering the use of reverse iterators, you open up new possibilities for data manipulation and analysis. Practice implementing reverse iterators in your projects to fully appreciate their utility and efficiency. Share your experiences or queries regarding reverse iterators as you dive deeper into the world of C++ programming!

Additional Resources
For further learning, consider diving into these resources:
- C++ Reference: [cppreference.com](https://en.cppreference.com/w/)
- Online courses focusing on STL and advanced C++ features
- Books on modern C++ practices to broaden your understanding and skills