In C++, you can call a base class constructor from a derived class constructor using an initializer list to ensure that the base part of the object is properly initialized before executing the derived class's constructor body.
Here's an example:
#include <iostream>
class Base {
public:
Base(int x) {
std::cout << "Base constructor called with value: " << x << std::endl;
}
};
class Derived : public Base {
public:
Derived(int x) : Base(x) { // Calling Base class constructor
std::cout << "Derived constructor called." << std::endl;
}
};
int main() {
Derived obj(10); // Outputs the base constructor followed by the derived constructor
return 0;
}
Understanding Constructors in C++
What are Constructors?
Constructors are special member functions in C++ that are automatically called when an object of a class is instantiated. Their primary role is to initialize the object. There are several types of constructors, including:
- Default Constructors: These constructors do not take any parameters and initialize objects to default values.
- Parameterized Constructors: These allow the initialization of an object with specific values provided by the user.
- Copy Constructors: These create a new object as a copy of an existing object.
The Role of Base Class Constructors
In a typical object-oriented programming structure, classes are often designed to inherit attributes and behaviors from other classes. The base class (or parent class) is the class being inherited from, whereas the derived class (or child class) inherits characteristics from the base class. Calling the base class constructor is essential in ensuring that the base portion of an object is initialized correctly before the derived part is constructed.
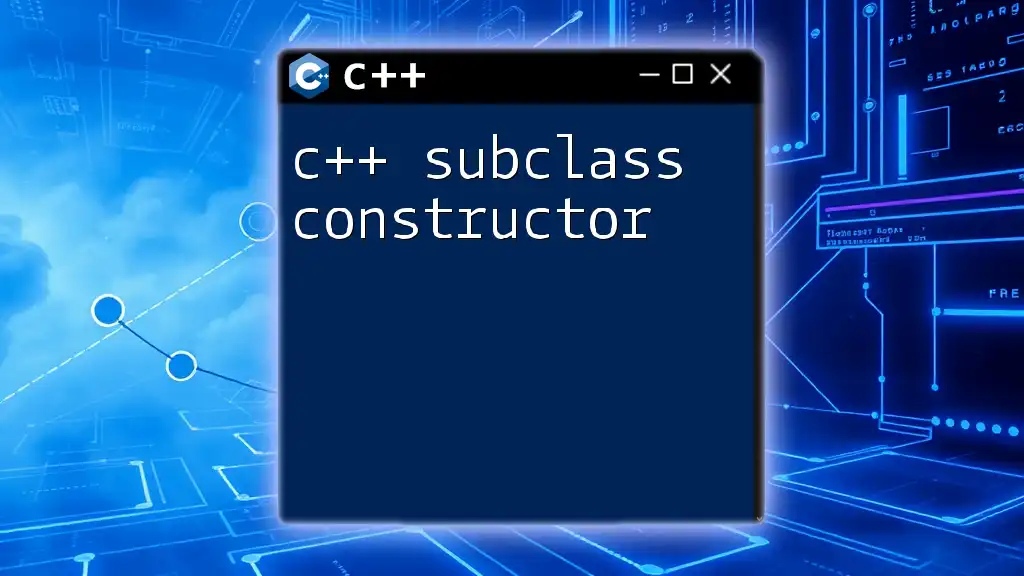
Basic Syntax of Calling Base Class Constructor
The Syntax Overview
The syntax for calling a base class constructor in C++ generally involves using the member initializer list, allowing the programmer to specify which base class constructor to call when a derived class is instantiated. Here's a concise look at how it works:
class Base {
public:
Base() {
// Default Constructor
}
Base(int x) {
// Parameterized Constructor
}
};
class Derived : public Base {
public:
Derived() : Base() { // Calling default constructor of base class
// Derived class constructor
}
Derived(int y) : Base(y) { // Calling parameterized constructor of base class
// Derived class constructor
}
};
Breakdown of the Syntax
Using Member Initializer List
The member initializer list allows you to call the base class constructor by specifying it directly in the constructor’s declaration of the derived class. This provides a clean and efficient way to ensure that the base class is initialized properly before any member-specific setups within the derived class.
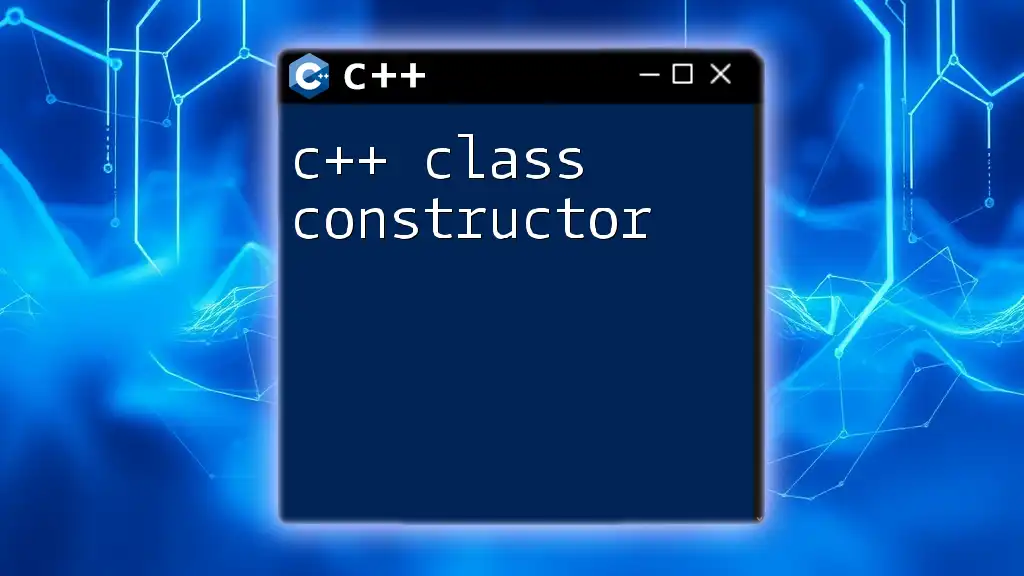
When to Call Base Class Constructors
Default vs. Parameterized Constructors
Choosing between default and parameterized constructors often depends on the needs of your derived class. If your derived class requires specific data to function correctly (for example, a number representing an object’s size or identifier), a parameterized constructor would be the right choice.
For instance, if you have a base class constructor that initializes a specific characteristic of an object like its number of wheels, using a parameterized constructor can ensure that this value is explicitly set at the time of constructing derived class objects:
class Vehicle {
public:
Vehicle(int wheels) {
std::cout << "Vehicle with " << wheels << " wheels created." << std::endl;
}
};
class Car : public Vehicle {
public:
Car() : Vehicle(4) { // Calls Vehicle with 4 wheels
std::cout << "Car created." << std::endl;
}
};
Constructor Initialization Order
Understanding the order of constructor calls is fundamental. When a derived class object is created, the base class constructor is invoked first, followed by the derived class constructor. This sequence ensures that the base class portion of the derived object is correctly initialized before any derived class-specific initialization occurs.
For example:
class Base {
public:
Base() {
std::cout << "Base class constructor called." << std::endl;
}
};
class Derived : public Base {
public:
Derived() {
std::cout << "Derived class constructor called." << std::endl;
}
};
// Output will show that Base constructor gets called first
In this snippet, the output clearly demonstrates that the base class constructor is executed before the derived class constructor.
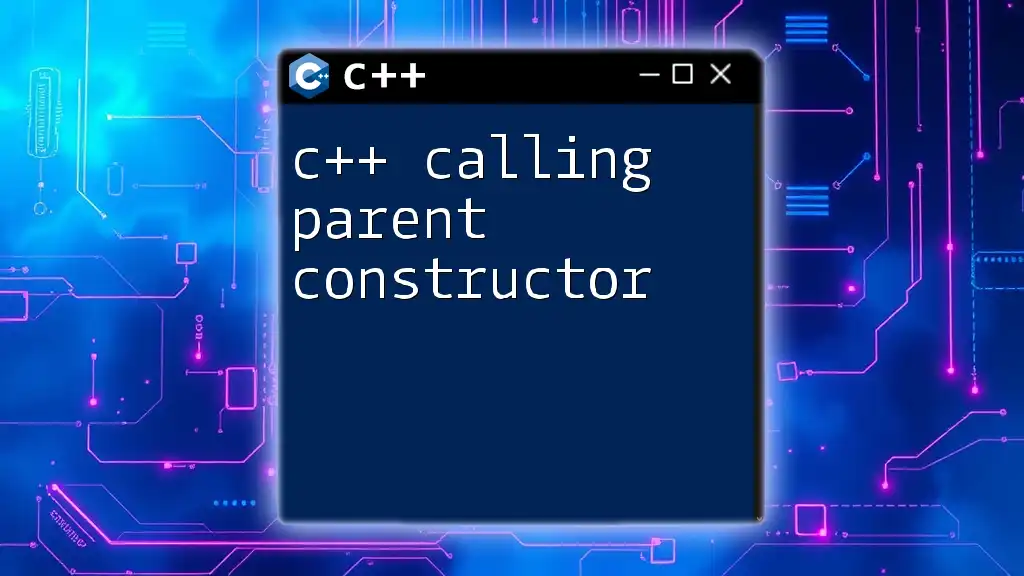
Practical Examples
Example 1: Simple Class Inheritance
Consider a situation where you have a simple inheritance structure with an `Animal` base class and a `Dog` derived class. Here’s how you can call the base class constructor:
class Animal {
public:
Animal() {
std::cout << "Animal created." << std::endl;
}
};
class Dog : public Animal {
public:
Dog() : Animal() { // Calls the Animal constructor
std::cout << "Dog created." << std::endl;
}
};
// Main function
int main() {
Dog myDog; // Output: Animal created. Dog created.
return 0;
}
In this example, creating an instance of the `Dog` class initiates the `Animal` constructor first, demonstrating the concept effectively.
Example 2: Parameterized Constructors
This example enhances the understanding by implementing parameterized constructors. Suppose you want to create a `Vehicle` base class that initializes with a specific number of wheels:
class Vehicle {
public:
Vehicle(int wheels) {
std::cout << "Vehicle with " << wheels << " wheels created." << std::endl;
}
};
class Car : public Vehicle {
public:
Car() : Vehicle(4) { // Calls Vehicle with 4 wheels
std::cout << "Car created." << std::endl;
}
};
// Main function
int main() {
Car myCar; // Output: Vehicle with 4 wheels created. Car created.
return 0;
}
Here, the derived `Car` class utilizes the parameterized constructor of the base `Vehicle` class to initialize it with a specific value.
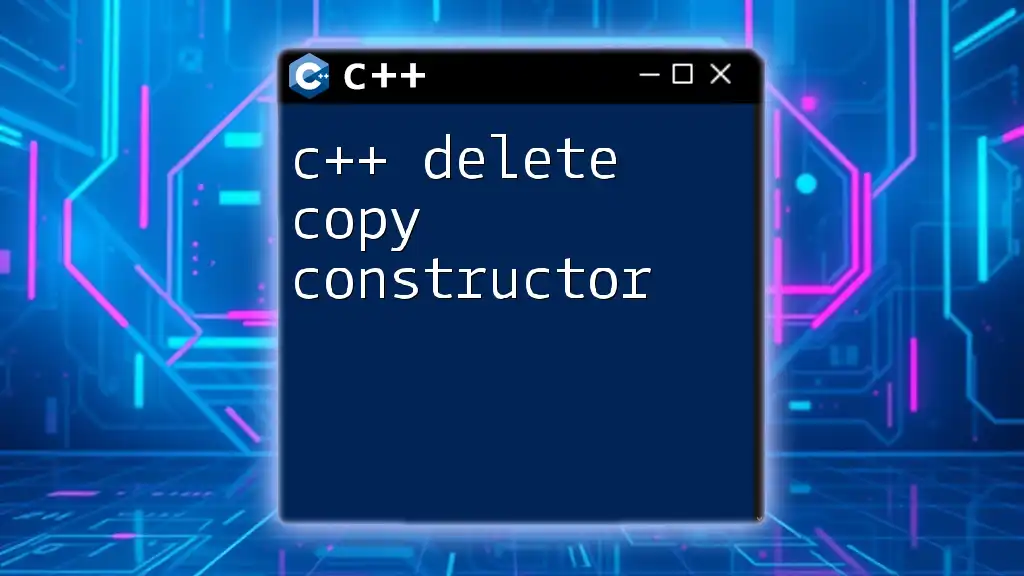
Common Pitfalls and Best Practices
Avoiding Common Mistakes
There are several common mistakes programmers can make when calling base class constructors:
- Forgetting to Initialize the Base Class: Not explicitly calling the base class constructor can lead to uninitialized memory, potentially causing undefined behavior.
- Incorrect Use of Constructor Parameters: Incorrect handling of parameters can lead to logic errors or crashes.
- Misunderstanding Constructor Call Order: Failing to grasp the order of constructor calls could lead to bugs in program execution, with derived class objects not correctly initialized.
Best Practices for Derived Class Constructors
- Always Use Initializer Lists: This practice helps in maintaining clarity and ensuring proper initialization of base class constructors.
- Parameter Management: Be mindful of the data that needs to be passed from the derived constructor to the base. Providing clear, default values where applicable can enhance safety while avoiding unnecessary complexity.
- Documentation and Comments: When working with complex inheritance structures, well-placed comments can assist in making your code understandable.
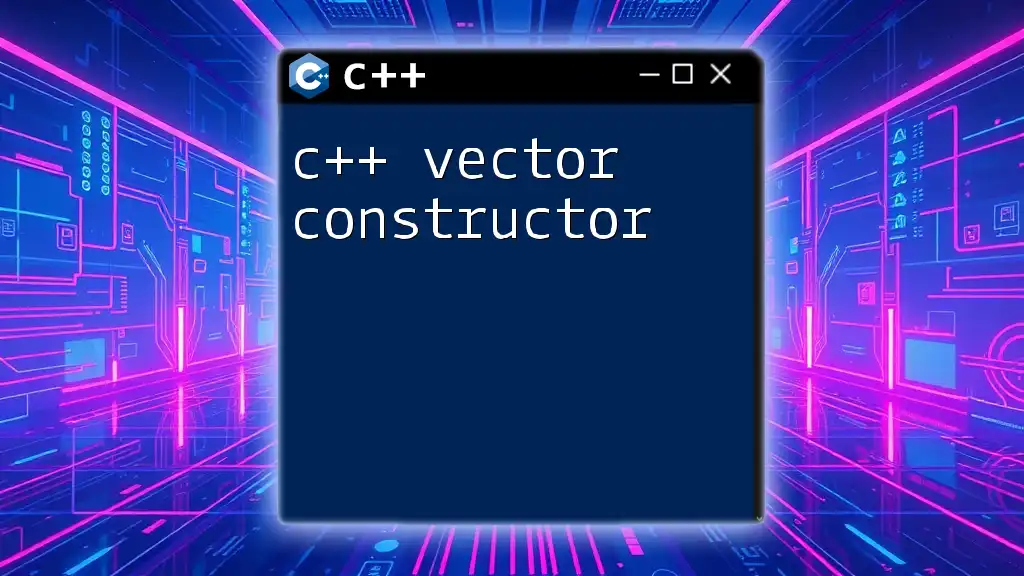
Conclusion
In summary, the process to c++ call base class constructor is an essential aspect of object-oriented programming. Understanding how and when to call base class constructors ensures efficient initialization and management of object properties, fostering more reliable code. Emphasizing best practices, such as using initializer lists and being cautious with parameter management, can safeguard against most common pitfalls.
To further enhance your mastery of C++, consider delving into advanced topics on inheritance, virtual functions, and polymorphism. Engaging in community forums and exploring various learning resources will also prove beneficial in elevating your programming skills.