In C++, an lvalue represents an object that occupies some identifiable location in memory (i.e., it has an address), whereas an rvalue represents a temporary value that does not have a persistent address.
Here's a code snippet that demonstrates the difference between lvalues and rvalues:
#include <iostream>
void processValue(int &&rvalue) { // rvalue reference
std::cout << "Rvalue: " << rvalue << std::endl;
}
int main() {
int x = 10; // x is an lvalue
processValue(x); // Error: cannot bind rvalue reference to lvalue
processValue(20); // 20 is an rvalue
return 0;
}
What is an lvalue?
Definition of lvalue
An lvalue is defined as an expression that refers to a specific memory location. This means that an lvalue can persist beyond a single expression and is typically used to represent variables or objects that have a defined address in memory.
Characteristics of lvalues
- Persistent memory address: An lvalue has a stable memory address, enabling it to remain accessible throughout its scope.
- Examples of lvalues:
- Variables like `int x = 10;` where `x` refers to an address.
- Array elements such as `arr[0]` which can be modified.
- Dereferenced pointers like `*ptr` which also refer to a memory location.
Code Snippet: Example of lvalue
int x = 10; // Here, 'x' is an lvalue.
x = x + 5; // 'x' can appear on the left side of the assignment.
In the above example, `x` maintains its identity between operations, showcasing its lvalue nature.
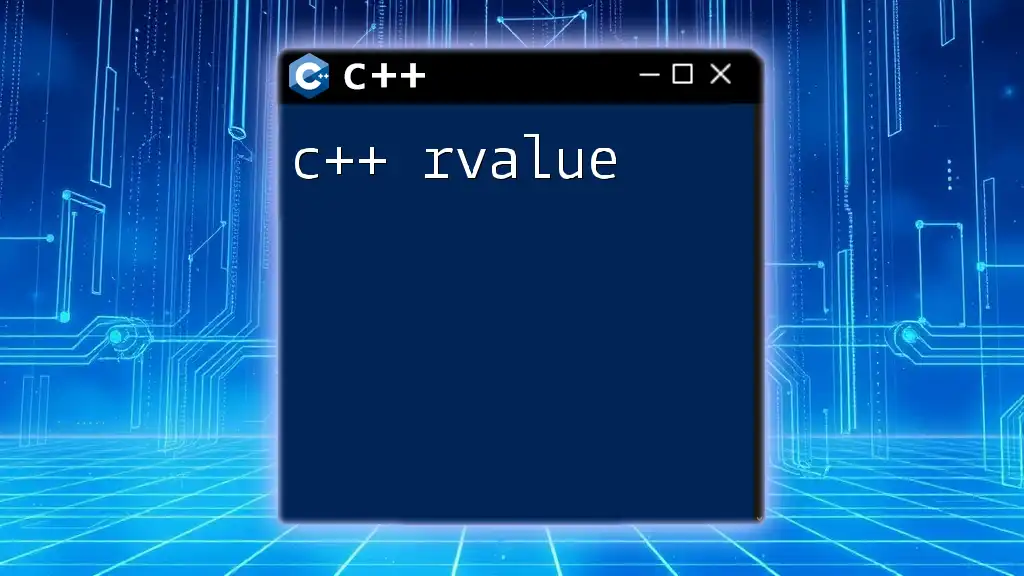
What is an rvalue?
Definition of rvalue
An rvalue refers to a temporary object or value that is not bound to a persistent memory location. These values typically arise during expressions and calculations, representing temporary data created by operations.
Characteristics of rvalues
- Temporary nature: rvalues exist only during the execution of the expression that uses them.
- Examples of rvalues:
- Literals like `5`, `3.14`, or `"Hello"` which are constant values.
- Temporary objects returned from functions, as they do not have a designated memory address accessible after the expression evaluation.
Code Snippet: Example of rvalue
int getValue() {
return 42; // The return value is an rvalue.
}
In this function, the `42` is an rvalue. It represents a temporary value generated for the duration of the function call.
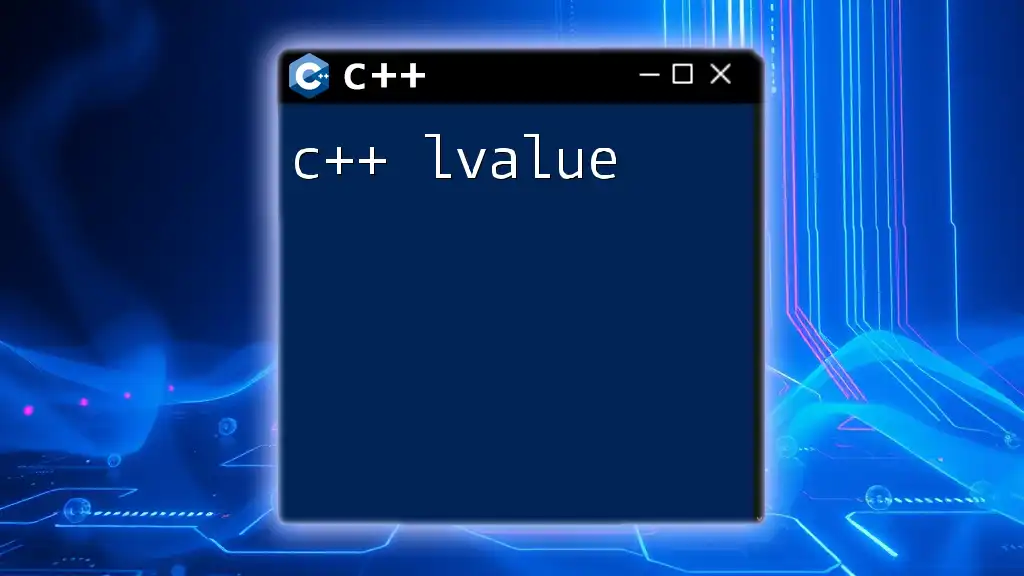
The Relationship Between lvalues and rvalues
lvalue and rvalue c++ concepts
In C++, lvalues and rvalues are fundamentally interrelated. Understanding this relationship helps in grasping how expressions evaluate and how memory is managed during assignments and function calls.
Implicit conversions
An important aspect of these types is that lvalues can often be converted into rvalues, particularly during the assignment process. For instance, in an expression, an lvalue can be used as an rvalue when the expression is evaluated.
Code Snippet: Implicit conversion example
int x = 5; // 'x' is an lvalue.
int y = x; // In this context, 'x' is treated as an rvalue.
Here, `x` behaves like an rvalue when it is assigned to `y`. The compiler creates a temporary snapshot of `x`'s value, which is then assigned to `y`.
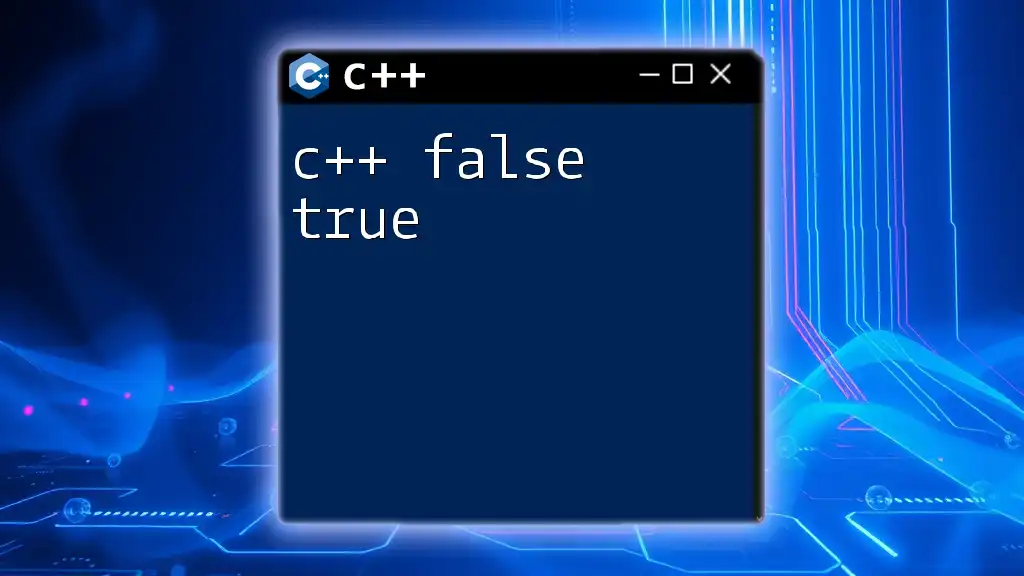
Move Semantics and rvalues in C++
Introduction to move semantics
Introduced in C++11, move semantics allows developers to perform more efficient operations by transferring resources from one object to another without duplicating them. This is especially beneficial for managing temporary objects, which are often rvalues.
What is std::move?
The function `std::move` is a powerful tool for converting an lvalue into an rvalue. This marks the object as eligible for moving, effectively allowing the transfer of its internal resources instead of copying them. This results in better performance, especially in resource-intensive applications.
Code Snippet: Using std::move
#include <utility>
#include <iostream>
void processValue(int &&val) {
std::cout << val << std::endl; // 'val' is an rvalue reference, can be modified inside.
}
int main() {
int x = 10;
processValue(std::move(x)); // 'x' is cast to an rvalue, allowing resource transfer.
}
In this example, `std::move` transforms `x` into an rvalue. The `processValue` function then accepts this rvalue, allowing optimization since it does not require a copy of the `x` variable.
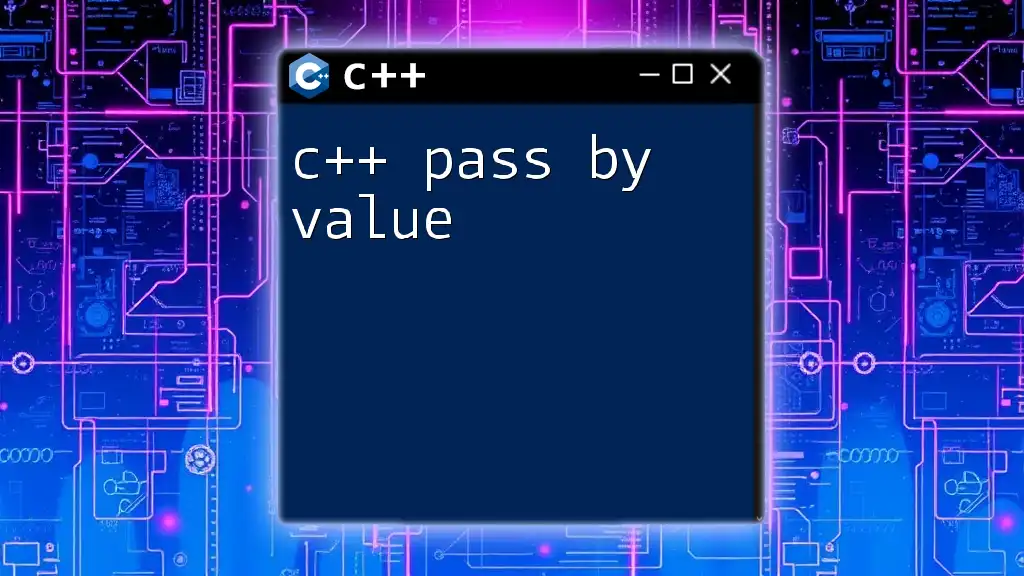
Common Misunderstandings
Confusion between lvalues and rvalues
One frequent error among developers is misidentifying lvalues and rvalues, especially when understanding how they operate in expressions. This confusion can lead to inefficient code or runtime errors.
Practical advice
To differentiate them reliably, remember: lvalues have a fixed memory address and can appear on the left-hand side of an assignment, whereas rvalues are temporary and typically appear on the right-hand side.
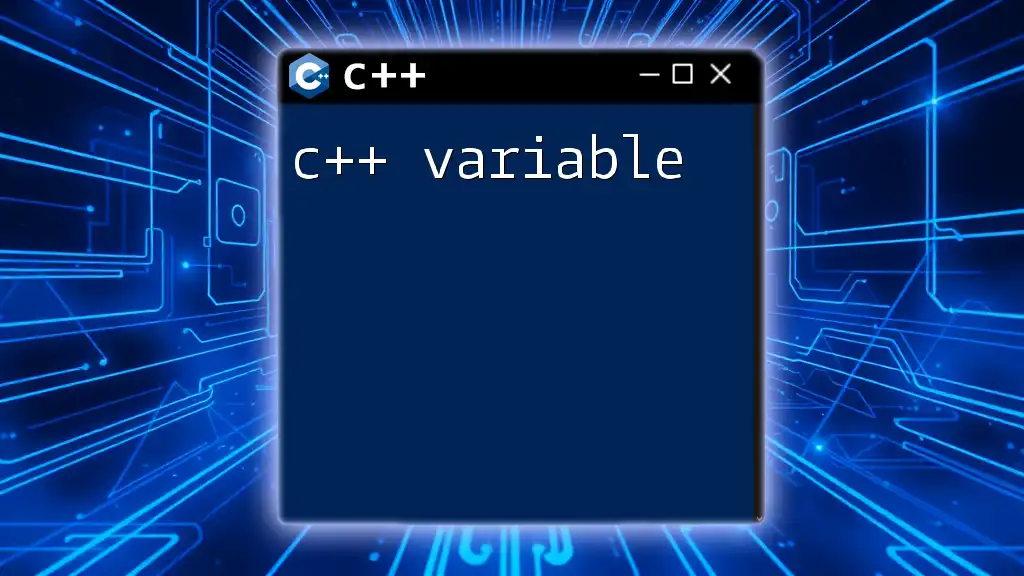
Conclusion
Understanding the distinction between C++ lvalue vs rvalue is not just an academic exercise; it is crucial for effective programming. Proper grasp of these concepts can lead to more efficient code and better resource management, particularly in environments that leverage move semantics.
Exploring the interplay between lvalues and rvalues opens doors to cleaner, faster, and more effective C++ programming practices. Engaging in hands-on coding with these concepts will enhance your skill set and deepen your understanding of C++.