In C++, an lvalue refers to a location in memory that has a persistent address (an object that occupies identifiable space), allowing it to be assigned a value, as illustrated in the following code snippet:
int a = 5; // 'a' is an lvalue
a = a + 2; // 'a' can be assigned a new value
Defining Lvalue
Understanding Lvalues
An lvalue (locator value) is a fundamental concept in C++ programming. It refers to an object that occupies an identifiable location in memory, meaning it has a specific address. This characteristic allows lvalues to hold value assignments and be easily modified. Essentially, lvalues serve as the variables and objects in your code that you can operate on and change.
Characteristics of Lvalues
- Modifiable: Lvalues can be altered or assigned new values after their declaration.
- Addressable: You can obtain the address of an lvalue using the address-of operator (`&`).
Examples of Lvalues
Consider the following code snippet:
int a = 10; // 'a' is an lvalue
int* p = &a; // You can obtain the address of 'a'
In this example, `a` is an lvalue since it can be assigned a value, and you can retrieve its memory address using `&`.
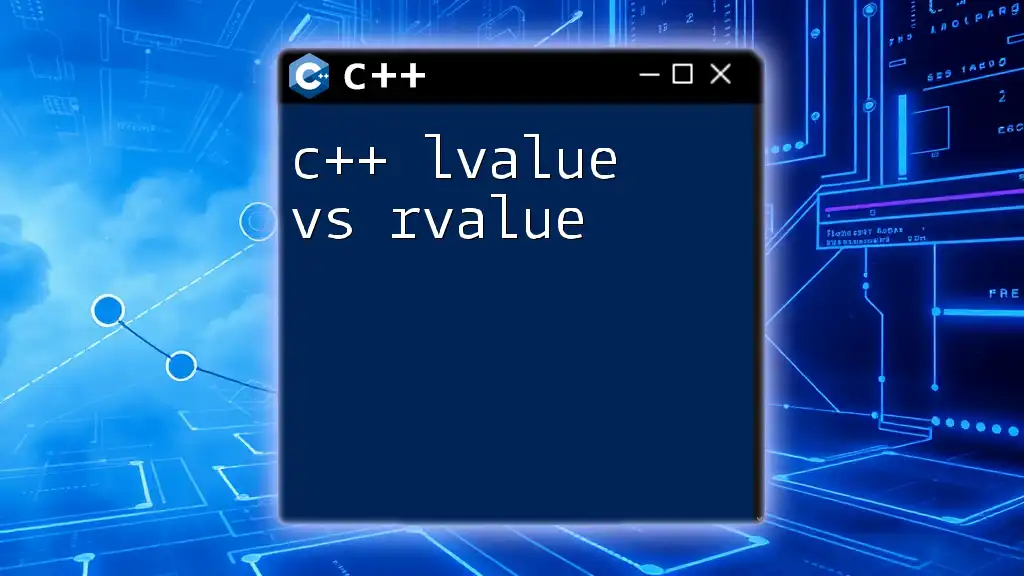
Differentiating Lvalue and Rvalue
The Concept of Rvalue
An rvalue (read value), on the other hand, is a temporary object that does not occupy a persistent memory location, meaning you can't reliably reference it. Rvalues typically appear in expressions and include literals and temporary variables.
Key Differences
To better understand the distinction between lvalues and rvalues, it's essential to note the following:
- Persistence: Lvalues are persistent variables; rvalues are typically temporary results from expressions.
- Assignability: You can assign values to lvalues, but rvalues cannot be assigned because they lack a memory address.
Code Snippet for Illustration
Here’s a quick demonstration to illustrate the difference:
int a = 10; // 'a' is an lvalue
int b = a + 5; // 'a + 5' is an rvalue
In this code, `a` is a variable that can be referenced and modified (an lvalue), while the expression `a + 5` evaluates to a temporary value, thus classified as an rvalue.
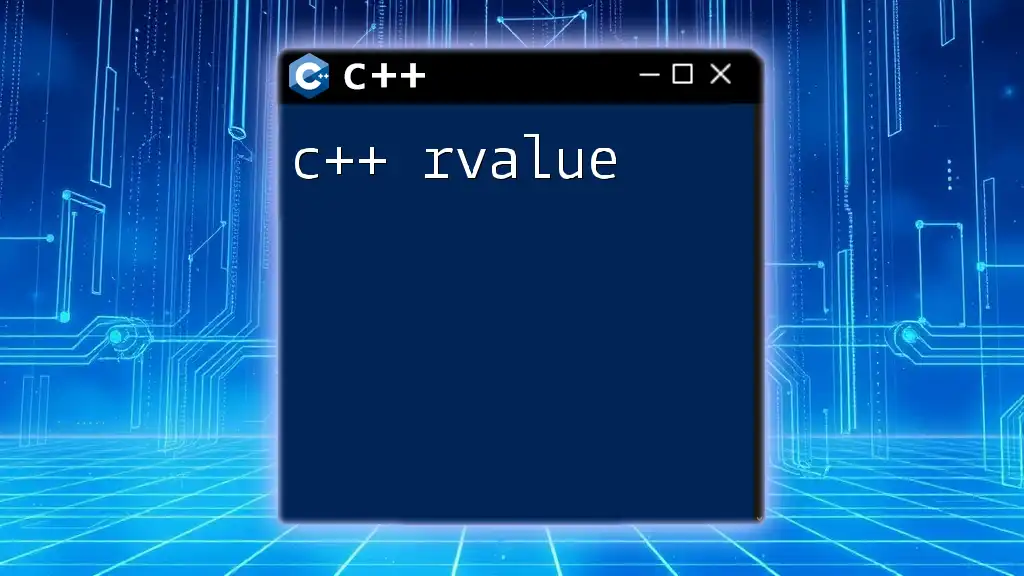
Types of Lvalues in C++
Non-const Lvalues
Non-const lvalues are values that can be modified during their scope. For example:
int num = 5; // Non-const lvalue
num += 10; // Modifiable; legal operation
In this case, `num` can be incremented freely.
Const Lvalues
Contrarily, const lvalues cannot be modified after their initialization. They are useful for protecting certain variables from being changed inadvertently. Here’s an example:
const int constantNum = 10; // Const lvalue
// constantNum += 5; // Error: cannot modify const value
Attempting to modify a const lvalue will lead to a compilation error, emphasizing the importance of using `const` when you want to safeguard a variable's value.
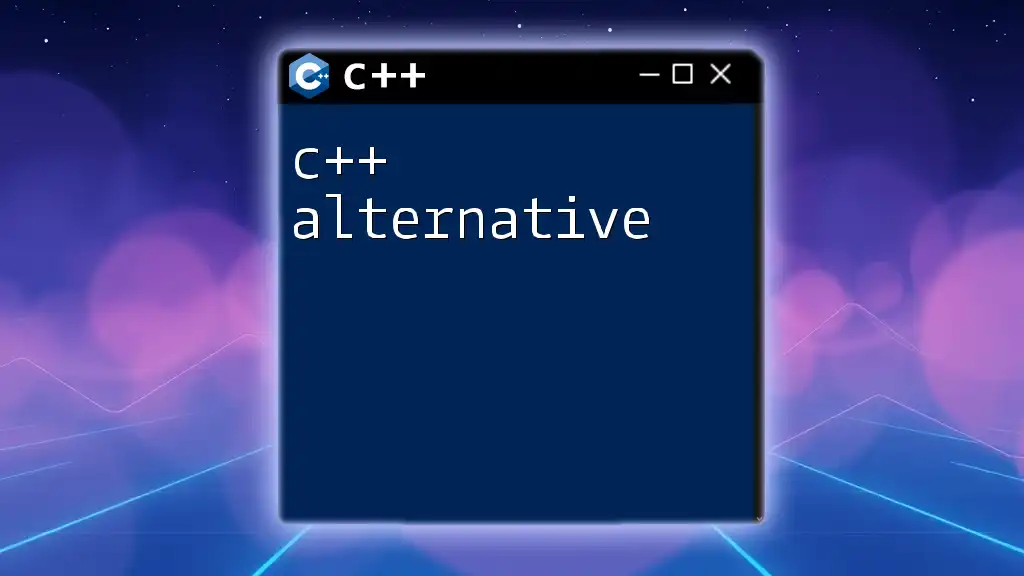
The Role of Lvalues and Rvalues in Expressions
Mixed Usage in Expressions
Lvalues and rvalues can interact seamlessly within expressions. Recognizing how both operate will enhance your coding efficiency. For instance:
int a = 10;
int b = a + 5; // Here, 'a' is an lvalue and '5' is an rvalue
In this code, `a` is an lvalue (since it can be addressed), while the number `5`, being a literal, acts as an rvalue in the computation.
Lvalue and Rvalue References
A deeper understanding of lvalues involves references, namely lvalue references and rvalue references.
Understanding Lvalue References
- An lvalue reference is an alias for an lvalue.
- It is declared with a syntax such as `int&`, allowing the programmer to create references to existing variables.
Example:
int& ref = a; // Here, 'ref' is an lvalue reference to 'a'
Understanding Rvalue References
Conversely, an rvalue reference is used to reference temporary values—this is a feature that C++ introduced to facilitate move semantics, which enhances performance by eliminating unnecessary copies. An rvalue reference is declared with syntax `int&&`.
Example:
int&& rvalueRef = 10; // rvalue reference to a temporary number
In this case, `rvalueRef` can bind to an rvalue, which allows it to represent temporary objects.
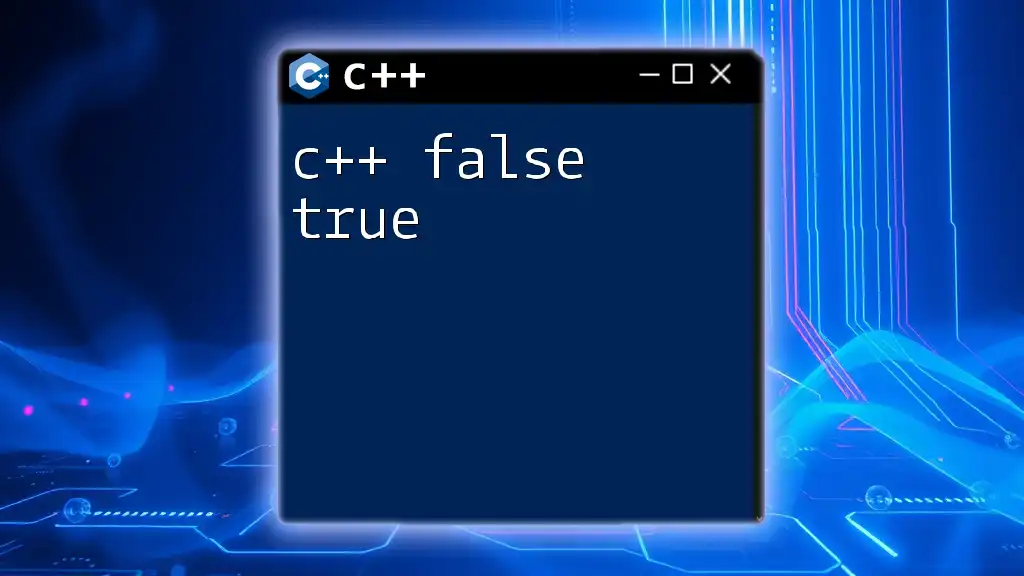
Practical Applications of Lvalues
Using Lvalues in Functions
One significant application of lvalues in programming comes in the form of functions. By passing variables as references, you increase performance because it avoids creating copies of objects. Consider the following function that increments a value:
void increment(int& value) {
value++; // Increments the lvalue passed
}
When you call `increment(a)`, it modifies the original variable `a` directly.
Returning Lvalues from Functions
You can also return lvalues from functions, which adds flexibility to your code. Here’s an example:
int& getReference(int &num) {
return num; // Return lvalue reference
}
When you use this function, you can receive a reference to an integer variable, allowing you to modify the original variable outside of the function's scope.
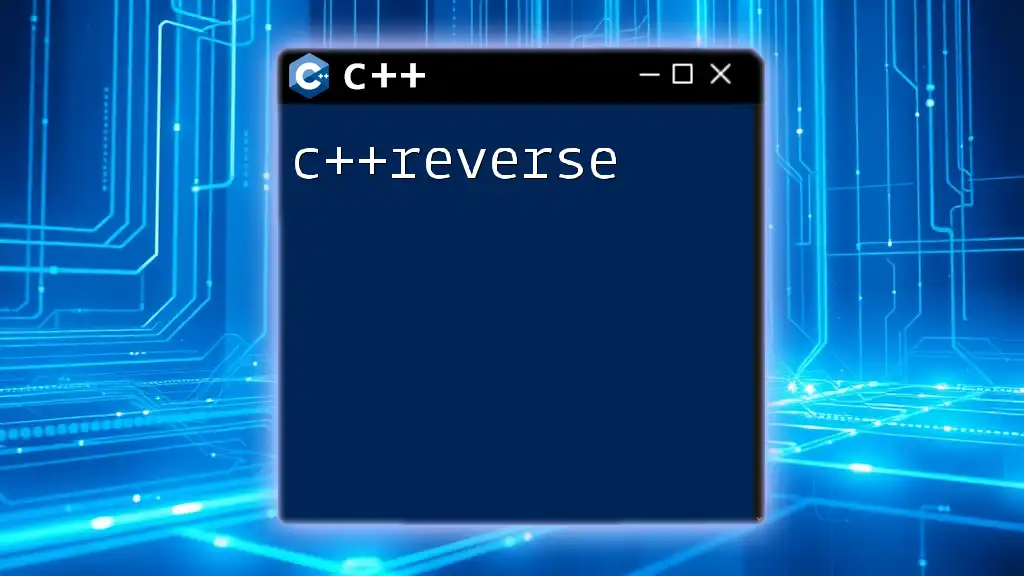
Common Mistakes with Lvalues
Misunderstanding Lvalue and Rvalue Context
A common pitfall is attempting to bind an rvalue to an lvalue reference. Consider the erroneous example:
int& ref = 10; // Error: cannot bind rvalue to lvalue reference
This line will fail to compile because you cannot assign a temporary value (rvalue) to an lvalue reference.
Unintentional Modifications
It’s important to ensure that const lvalues are not accidentally modified. For example:
const int num = 5;
// num++; // Error: cannot modify const lvalue
This code will result in an error if we attempt to change `num`, reinforcing why the `const` keyword is crucial for protecting certain variables.
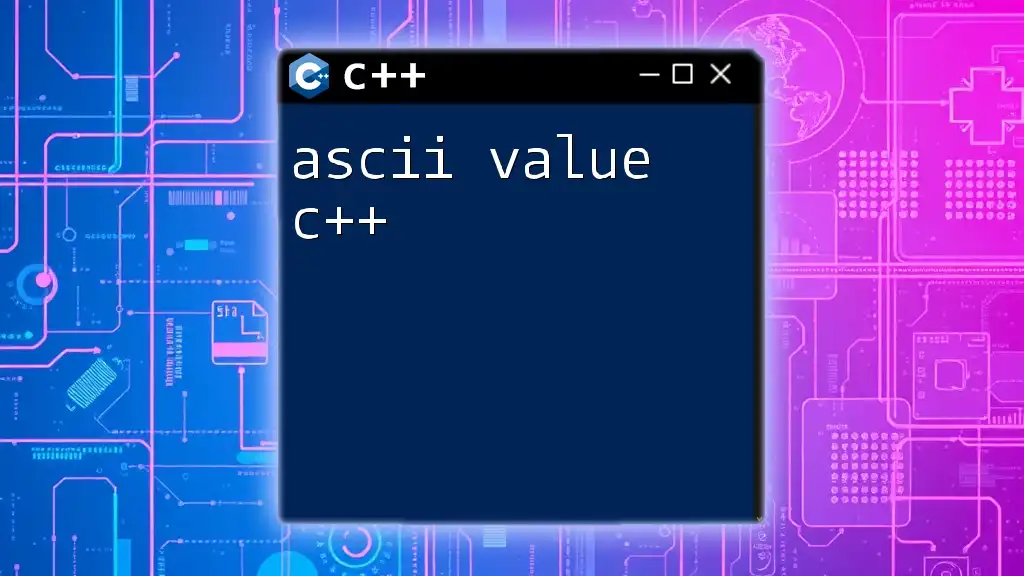
Conclusion
Understanding c++ lvalue is essential for mastering C++ and improving your programming skills. Lvalues provide the foundation for managing data in your code, enhancing performance and efficiency. With a solid grasp of this concept, including how it interacts with rvalues, you'll find yourself writing more robust and optimized C++ applications. Familiarity with lvalues also prepares you for more advanced topics like references and move semantics, bridging the gap to proficient C++ programming.