In C++, the function `std::string::length()` is used to determine the number of characters in a string, which can be referred to as "len" for brevity.
#include <iostream>
#include <string>
int main() {
std::string example = "Hello, World!";
std::cout << "Length of the string: " << example.length() << std::endl; // Output: Length of the string: 13
return 0;
}
Understanding C++ Strings
What are C++ Strings?
C++ strings are a collection of characters and are part of the Standard Library through the `std::string` class. They provide a robust way to handle text in your applications, making string manipulation easier and more versatile when compared to C-style strings (character arrays).
C++ strings manage memory automatically, meaning you won't have to worry about allocating or deallocating memory manually, which is a common pitfall in C-style strings. This automatic memory management helps avoid some common errors, such as memory leaks and buffer overflows.
How C++ Strings Work
When you create a C++ string, it abstracts away low-level operations and provides essential functions you can use to manipulate text. For instance, a simple string can be initialized as follows:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
The `.length()` Function Overview
The `.length()` function is a member of the `std::string` class and returns the number of characters present in a string. Its fundamental syntax is straightforward:
string_variable.length();
For instance, in the following code snippet, we demonstrate how to use the `.length()` function:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length of the string: " << myString.length() << std::endl;
return 0;
}
In this example, "Length of the string: 13" would be printed, indicating that the string contains 13 characters, including spaces and punctuation.
The `size()` Function
In addition to `.length()`, C++ provides the `size()` function which effectively performs the same task. The choice between using `.length()` or `size()` is often a matter of personal preference, as both yield the same result.
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length using length(): " << myString.length() << std::endl;
std::cout << "Length using size(): " << myString.size() << std::endl;
return 0;
}
Here, both function calls return 13. While it might seem redundant to have both, it offers programmers flexibility, as some might find `size()` more intuitive in contexts dealing with containers.
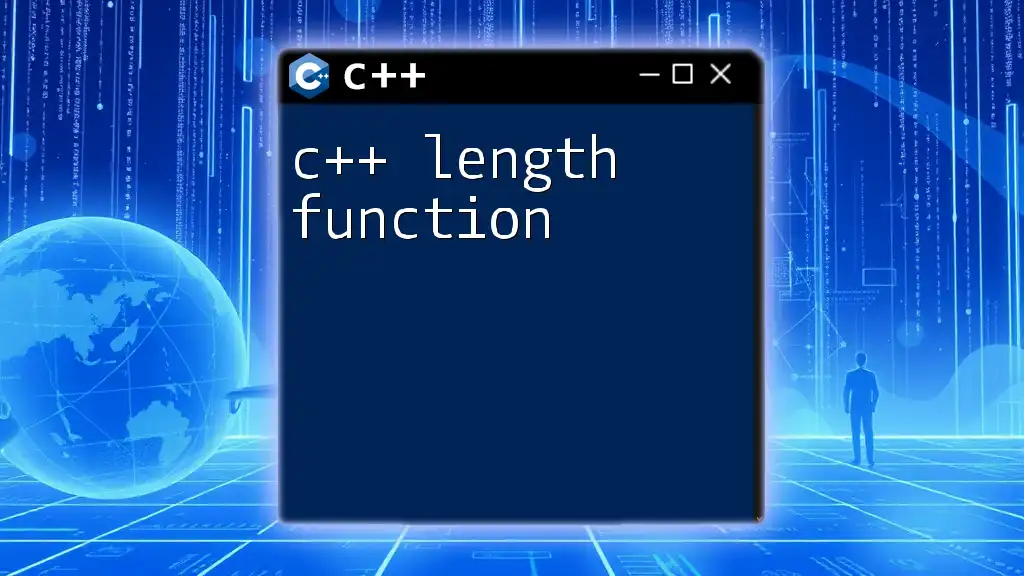
Practical Applications of C++ String Length
Basic String Length Checks
Understanding string length is critical for many programming tasks, including validating user input. A common scenario involves checking if a string is empty:
#include <iostream>
#include <string>
int main() {
std::string myString;
if (myString.length() == 0) {
std::cout << "The string is empty." << std::endl;
}
return 0;
}
In this case, before processing or manipulating the string, you can ensure that it contains some data, which helps avoid errors down the line.
Looping Through Characters
The length of a string can also be employed when you need to loop through each character for processing. For example, you can use string length to print each character separately:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello";
for (size_t i = 0; i < myString.length(); ++i) {
std::cout << myString[i] << std::endl;
}
return 0;
}
This code snippet prints each character on a new line. The `length()` function tells the loop how many times to iterate, thereby preventing out-of-bounds errors.
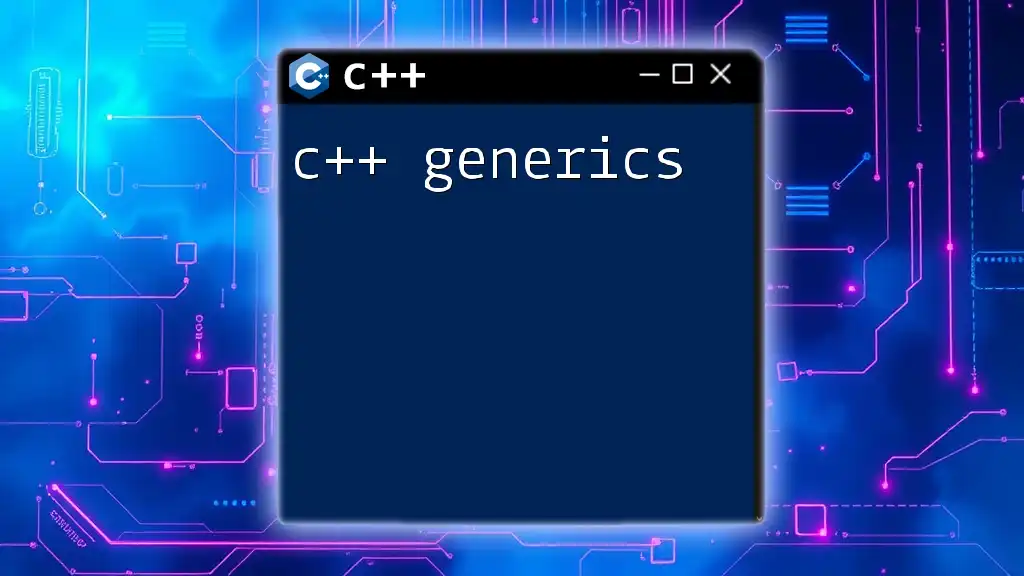
Common Pitfalls When Using C++ String Length
Misunderstanding Indexing
It's crucial to remember that C++ uses zero-based indexing. This means that when accessing characters in a string, the first character is at index 0, and the last character is at `length() - 1`. Here's an important illustrative example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello";
// Accessing the last character
char lastChar = myString[myString.length() - 1];
std::cout << "Last character: " << lastChar << std::endl;
return 0;
}
In this case, the output will be "Last character: o", demonstrating that knowing the length is crucial for correctly indexing into the string.
Performance Considerations
While the length function is efficient in C++, there are some performance considerations to be mindful of, especially in cases of repeated function calls in high-performance applications. If you frequently need the length, it may be beneficial to store it in a variable instead of calling the length function multiple times, as this avoids unnecessary recalculations.
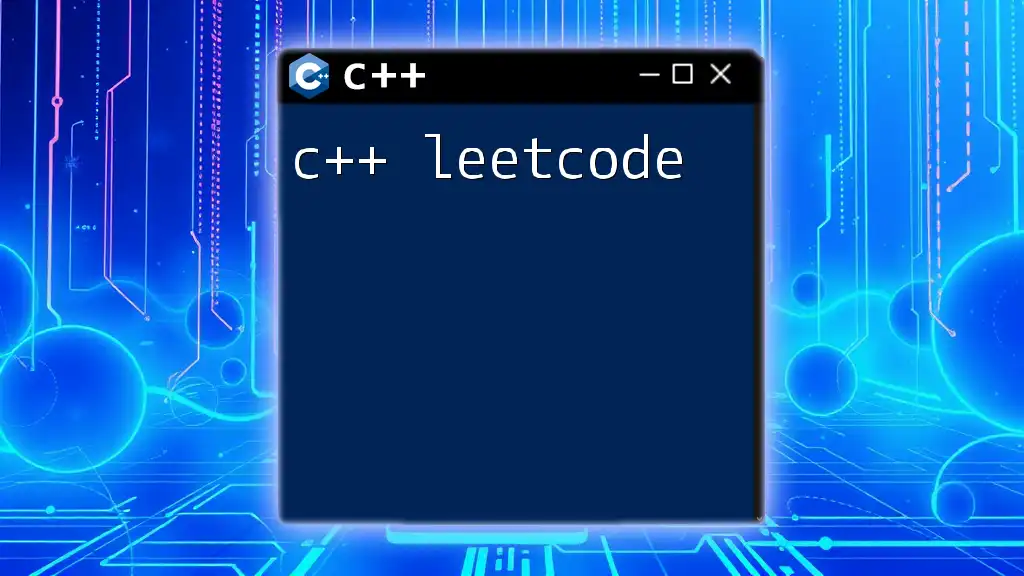
Conclusion
Understanding how to effectively use the `c++ len`, or the `.length()` function, is essential for any C++ programmer. This knowledge equips you to manipulate strings confidently and check properties like length, ultimately leading to more robust code.
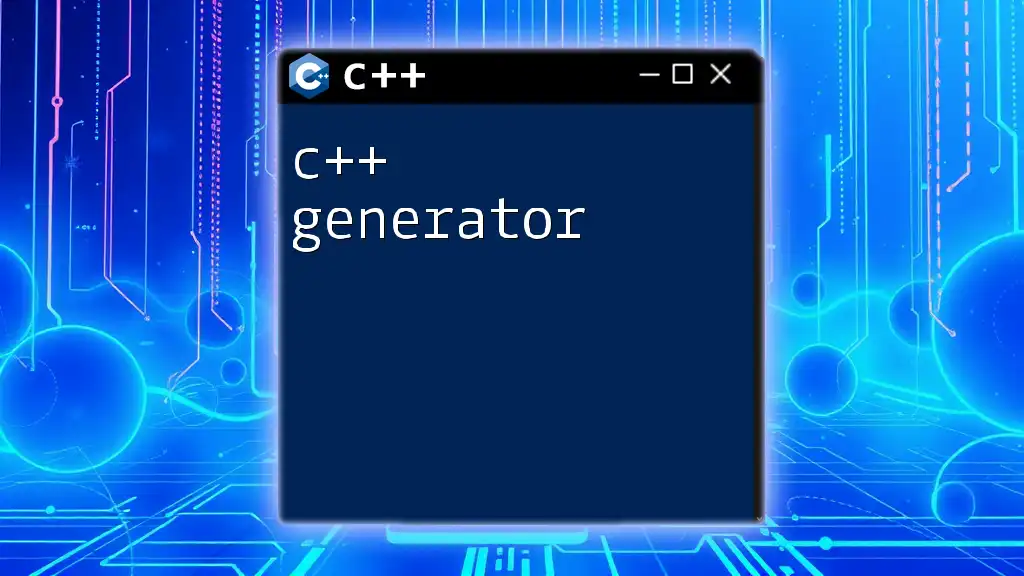
Additional Resources
Recommended Reading
For those looking to deepen their understanding of C++ strings and related functions, consider visiting the official C++ documentation or exploring recommended books and online courses that focus on C++ programming fundamentals.
FAQs about C++ String Length
In the world of programming, questions often arise surrounding string length. Some common queries include how to handle null characters, best practices for string manipulation, and tips for debugging common errors related to string length. Staying curious and informed can significantly enhance your programming skills.