In C++, the `link` refers to the process of combining multiple object files to create a final executable, and it can be done using the linker command in a terminal or build system.
Here's a simple example of how to compile a C++ program with `g++`, linking multiple source files `main.cpp` and `helper.cpp`:
g++ main.cpp helper.cpp -o my_program
Overview of C++ Link
What is Linking in C++?
Linking in C++ is a crucial step in the compilation process that combines various pieces of code and resolves symbol references. When you write a C++ program, it usually consists of several source code files. The compiler processes these files individually, creating object files for each, but the linking stage is where these object files come together to form a fully functional executable.
The entire compilation process can be broken down into four main stages: preprocessing, compilation, assembling, and linking. While preprocessing involves handling directives like `#include`, compilation translates code into machine language, assembling creates object files, and linking ultimately resolves all references to produce the final output.
Types of Linking
Linking can primarily be categorized into two types: static linking and dynamic linking.
-
Static Linking: During static linking, all the necessary code from libraries and object files is combined into a single executable at compile time. This results in larger binary files but ensures all dependencies are embedded within the executable, leading to self-contained applications. One downside is that any updates to libraries require recompilation of the program.
-
Dynamic Linking: In contrast, dynamic linking links libraries at runtime rather than compile time. The executable only contains references to the libraries, which can be shared among multiple programs. This leads to smaller binaries and makes updates easier, as the executable can use the latest version of the library without recompilation.
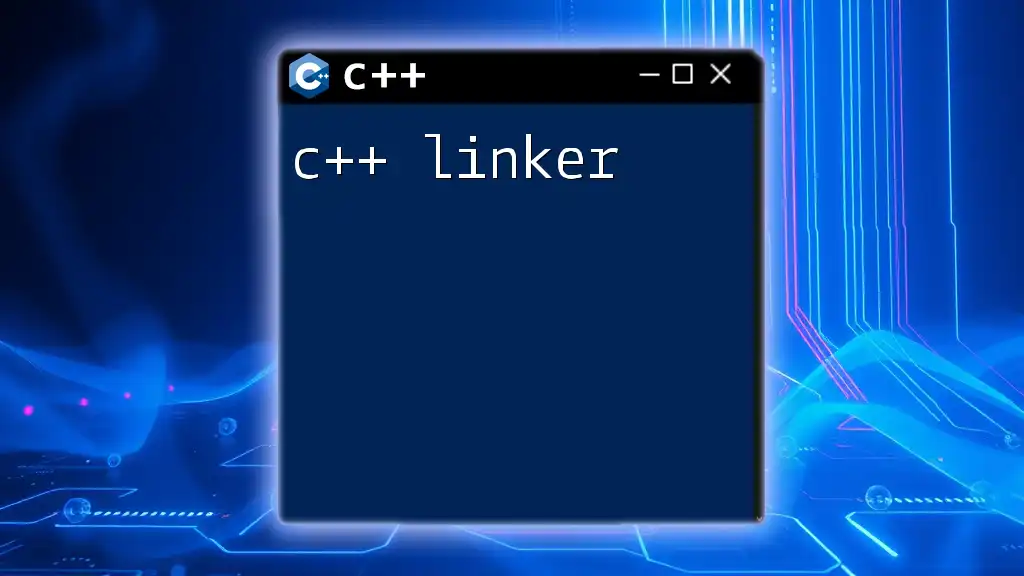
Linkage and Linking
What is Linkage?
Linkage is the property of a variable or function that determines its accessibility across different translation units (source files). Understanding linkage is essential for organizing and managing symbol visibility in complex programs.
-
External Linkage: When a variable or function can be accessed across multiple source files, it exhibits external linkage. By default, functions and global variables have external linkage unless defined otherwise.
-
Internal Linkage: Variables or functions that are only accessible within the file they are declared in possess internal linkage. This can be achieved using the `static` keyword.
Linkage Specifications
-
Extern Keyword: The `extern` keyword allows for declarations of variables that are defined in other files. It tells the compiler that the variable exists and will be linked later.
extern int globalVar; // Declaration for external linkage
-
Static Keyword: The `static` keyword, when applied to variables at global scope, restricts their linkage to the file they are declared in. This helps prevent name clashes in larger projects.
static int localVar; // Definition for internal linkage
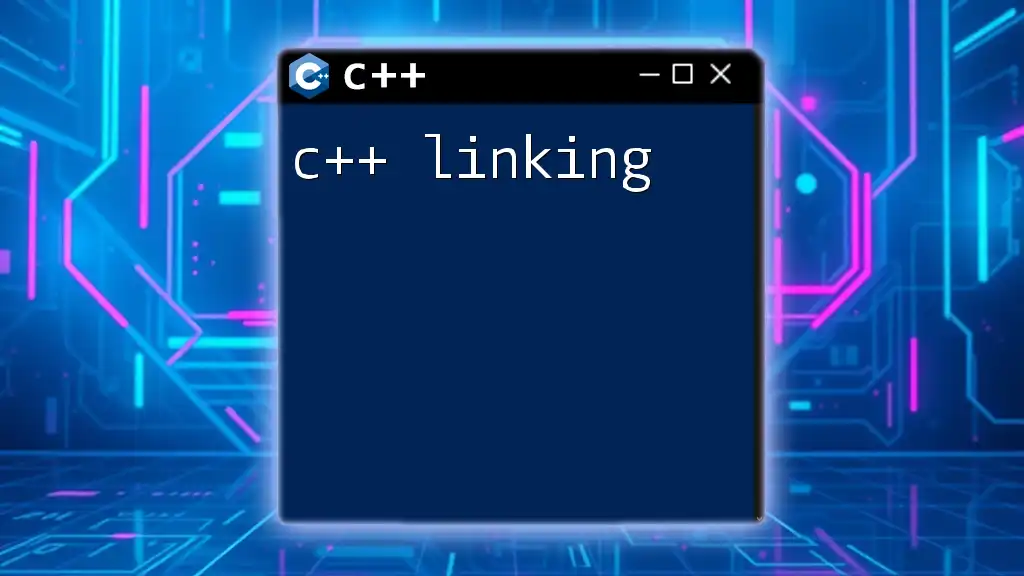
The Linking Process
How Linking Works
During the linking stage, the linker takes multiple object files generated by the compiler and combines them into a single executable. This process involves examining symbols from all object files, resolving any cross-references, and generating the necessary addressing information.
Role of Object Files
Object files are the intermediary products of the compilation process. They contain compiled code and metadata, such as symbol tables and relocation information. Object files typically have the `.o` extension, and they allow code to be modular.
You can use the following command to compile a single source file into an object file:
g++ -c myfile.cpp
This generates `myfile.o`, which can later be linked with other object files to create an executable.
The Linker’s Tasks
The linker performs various essential tasks, such as resolving symbol references—this means it takes care of what functions or variables are being called from different object files. It also combines the various sections (like text, data, etc.) from the object files into the final executable format.
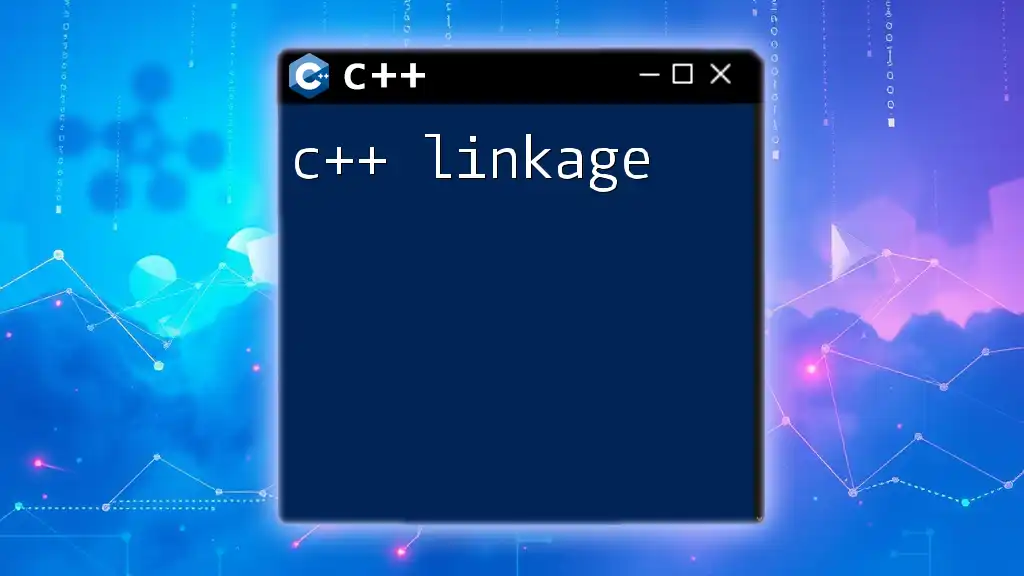
Common Linker Errors
Types of Errors
-
Undefined Reference: This error occurs when the linker encounters a symbol that it cannot find a definition for. For instance, if you declare a function but forget to define it elsewhere, such as:
void myFunction(); // Declaration int main() { myFunction(); // Undefined reference error if not defined return 0; }
-
Multiple Definitions: If a function or variable is defined in multiple source files without proper management, the linker will throw a multiple definitions error. For example:
int myVar = 5; // Definition in one file int myVar = 10; // Definition in another file (causes error)
Debugging Linker Errors
Debugging linker errors requires careful examination of your code and linking structure. Here are some tips to troubleshoot:
- Check for Correct Includes: Ensure you aren’t mistakenly including files or headers that cause ambiguous references.
- Use Tools: Tools like `gdb`, `nm`, and `objdump` can help diagnose issues by listing defined symbols and helping you spot discrepancies.
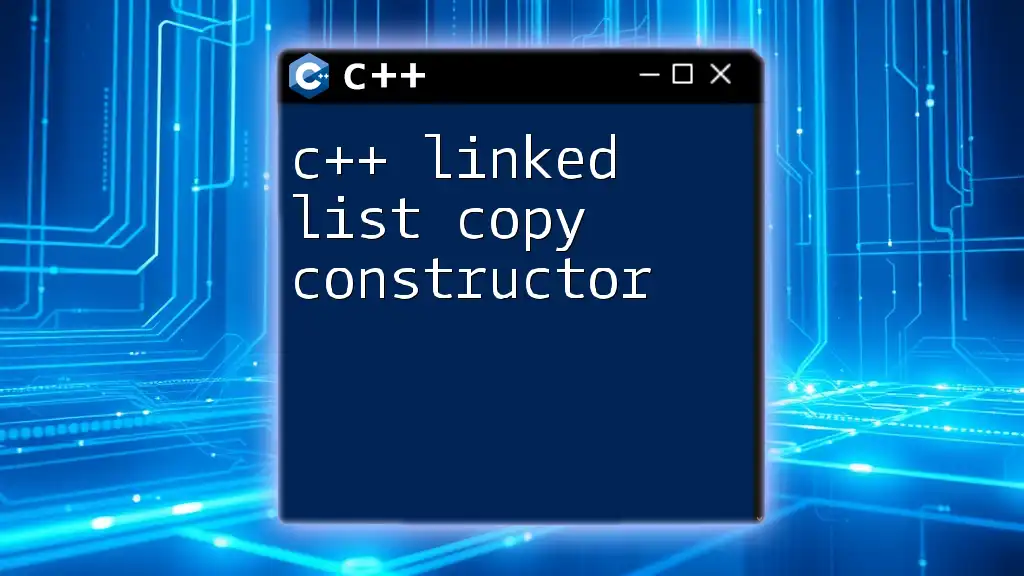
Best Practices for Linking in C++
Organizing Code for Easy Linking
To facilitate easy linking, it’s essential to adopt a clean code organization strategy. This includes structuring your header files correctly, utilizing header guards, and ensuring that function prototypes are easily accessible to other files without unnecessary duplications.
Header guards prevent problems caused by double declaration. Here’s an example of header guard usage:
#ifndef MY_HEADER_H
#define MY_HEADER_H
void myFunction();
#endif // MY_HEADER_H
Use of Makefiles
Makefiles help in managing workflows and dependencies efficiently. A basic Makefile could look like this:
all: myprogram
myprogram: main.o myfunction.o
g++ -o myprogram main.o myfunction.o
main.o: main.cpp
g++ -c main.cpp
myfunction.o: myfunction.cpp
g++ -c myfunction.cpp
clean:
rm -f *.o myprogram
This structure lets you easily compile your project incrementally, re-compiling only what has changed.
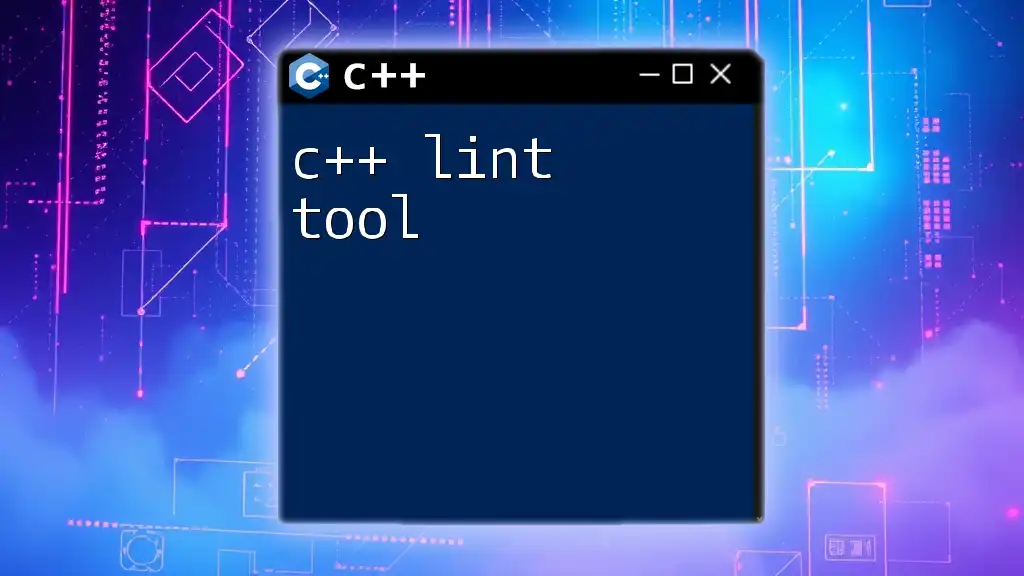
Conclusion
Importance of Proper Linking in C++
Understanding the linking process is pivotal for preventing errors and ensuring that your C++ programs run smoothly. A solid grasp of how linking works can help you avoid many common pitfalls, especially in larger codebases where multiple files interact.
Final Thoughts
Practicing linking through various projects can significantly enhance your coding skills and make you more efficient as a developer. Don’t hesitate to experiment with different linking strategies and share your experiences and solutions to linking-related challenges in your journey.
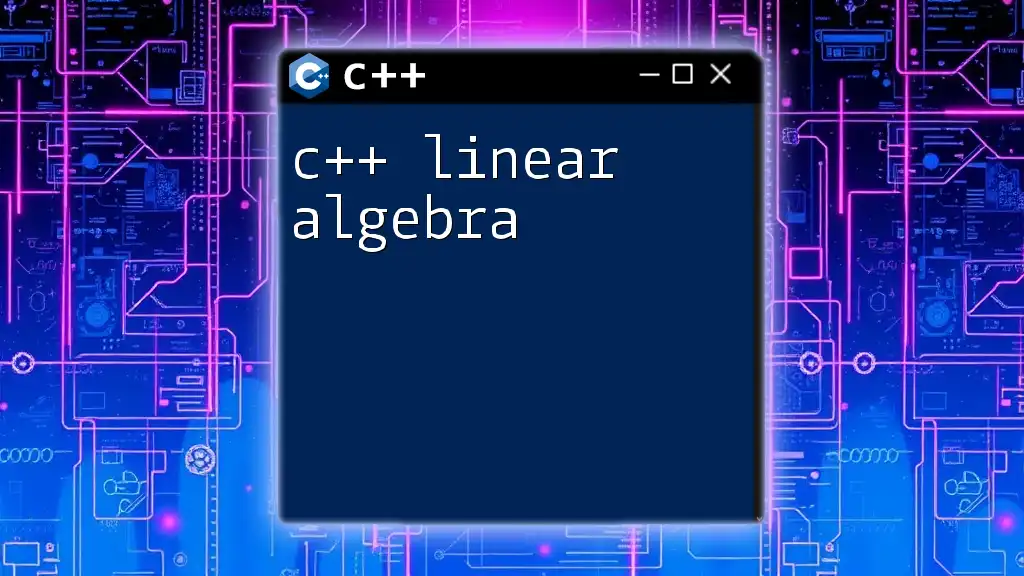
Additional Resources
Books and Online Courses
Several resources can further expand your knowledge, including classic texts on C++ programming and various online courses that focus on the intricacies of the language.
Sample Projects and Exercises
Engaging in sample projects or exercises is a great way to practice linking. Consider building small applications that require you to manage multiple files and libraries, which will deepen your understanding of the linking process.