The C++ lint tool is a static code analysis tool that helps programmers identify potential errors and improve the quality of their C++ code by analyzing it for stylistic and programming errors before compilation.
Here’s an example of using a linting command in your terminal:
cppcheck --enable=all my_code.cpp
This command will run `cppcheck`, enabling all checks on the specified `my_code.cpp` file.
What is a C++ Linter?
A linter is a static code analysis tool that evaluates source code to flag programming errors, bugs, stylistic errors, and suspicious usage. In the context of C++, a C++ linter is specifically designed to help developers catch syntax mistakes, enforce coding standards, and improve overall code quality.
By utilizing C++ lint tools, developers can benefit from:
- Improved Code Quality: Lint tools help ensure that code adheres to predefined coding standards, leading to more maintainable and reliable code.
- Enhanced Readability: By enforcing consistent formatting and style, code becomes easier for teams to read and understand.
- Early Detection of Bugs: Many lint tools can highlight potential issues before the code is executed, reducing debugging time later on.
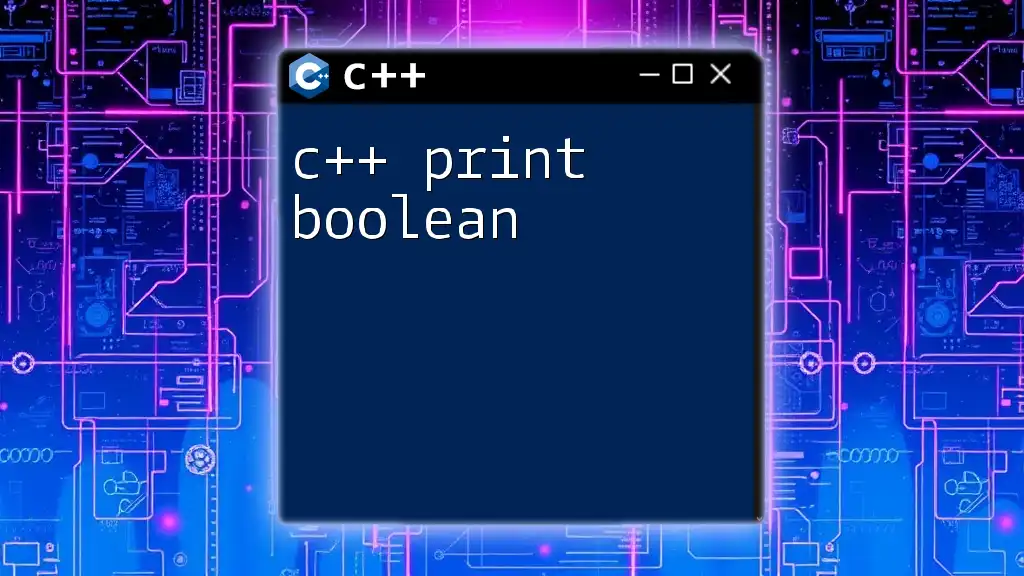
Popular C++ Lint Tools
Clang-Tidy
Clang-Tidy is a powerful linter that's part of the LLVM project. It offers a wide range of checks and fixes, making it an excellent choice for maintaining code quality in C++ projects.
- Key Features:
- Supports a variety of checks for readability, performance, and security.
- Can automatically suggest fixes for many issues.
- Integrates well with build systems and IDEs.
To install Clang-Tidy, follow these steps depending on your platform:
- Linux: Install through your package manager (e.g., `sudo apt install clang-tidy`).
- macOS: Use Homebrew (`brew install llvm`).
- Windows: Download it as part of the LLVM package from the official website.
Basic Usage Example: To use Clang-Tidy, you simply run the following command:
clang-tidy my_code.cpp -- -std=c++11
This command lints the `my_code.cpp` file under C++11 standard.
cpplint
cpplint is a lightweight linter that focuses on Google's C++ style guide. It checks the formatting and style of your C++ code to help you maintain consistency.
- Features:
- Simple output, highlighting deviations from style guidelines.
- Configurable to match personal or team coding styles.
To install cpplint, you can use pip:
pip install cpplint
Configuring cpplint: You can create a configuration file named `.pylintrc` to define custom linting rules that fit your project’s needs.
Code Example: To lint a C++ file, run:
cpplint my_code.cpp
This will check `my_code.cpp` against the Google C++ style guide.
CPPcheck
CPPcheck is another excellent lint tool that emphasizes error detection rather than style compliance. It specifically targets bugs and potential vulnerabilities.
- Distinct Features:
- Static analysis capability that can discover complex bugs that other compilers might miss.
- Supports configuration files to customize checks that are performed.
To install CPPcheck, you can follow these steps:
- Linux: Install via package managers (`sudo apt install cppcheck`).
- macOS: Install using Homebrew (`brew install cppcheck`).
- Windows: Download the installer from the official CPPcheck website.
Example Usage: To analyze a C++ file, simply use:
cppcheck my_code.cpp
CPPcheck will provide insights into potential issues within the code, helping you to enhance the overall quality.
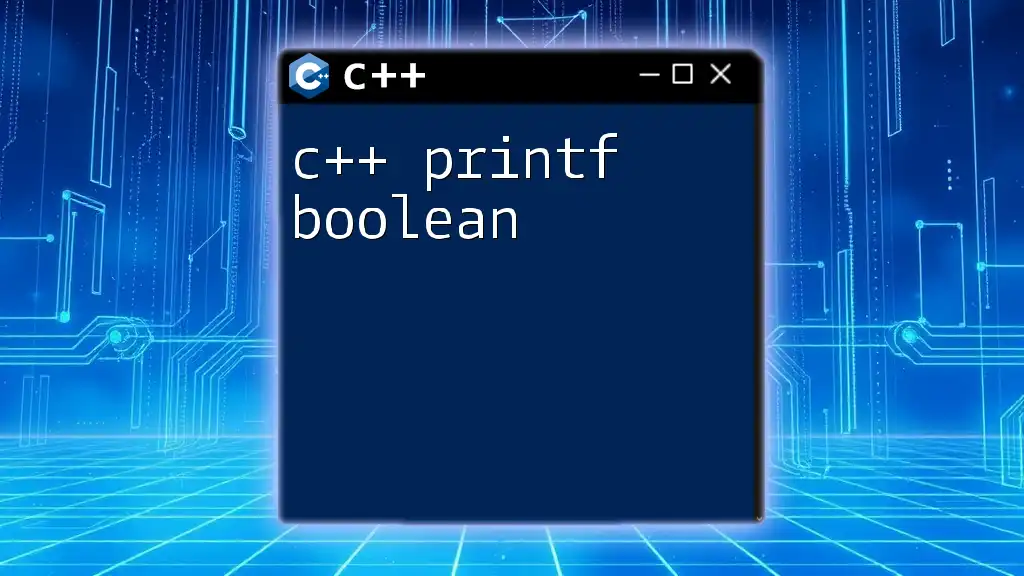
How to Choose the Right C++ Linter
Selecting the appropriate C++ lint tool involves various factors, including project size, team expertise, and adherence to specific coding standards.
- Project Size: A smaller project might only require basic linting, while larger projects may benefit from more comprehensive tools like Clang-Tidy.
- Team Expertise: Consider tools that your team is comfortable with or willing to learn. A familiar tool will lead to greater efficiency.
- Specific Coding Standards: If your team follows a particular coding standard, select a linter that can be easily configured to enforce those rules.
A quick comparison of popular C++ linters can help guide your choice:
- Clang-Tidy: Versatile with extensive checks, great for ensuring code quality.
- cpplint: Straightforward and focused on style, suited for smaller projects adhering to Google C++ standards.
- CPPcheck: Robust error detection capabilities make it ideal for catching bugs.
Additionally, integrating a linter into your Continuous Integration/Continuous Deployment (CI/CD) pipeline ensures that code is checked with every commit, promoting a culture of code quality.
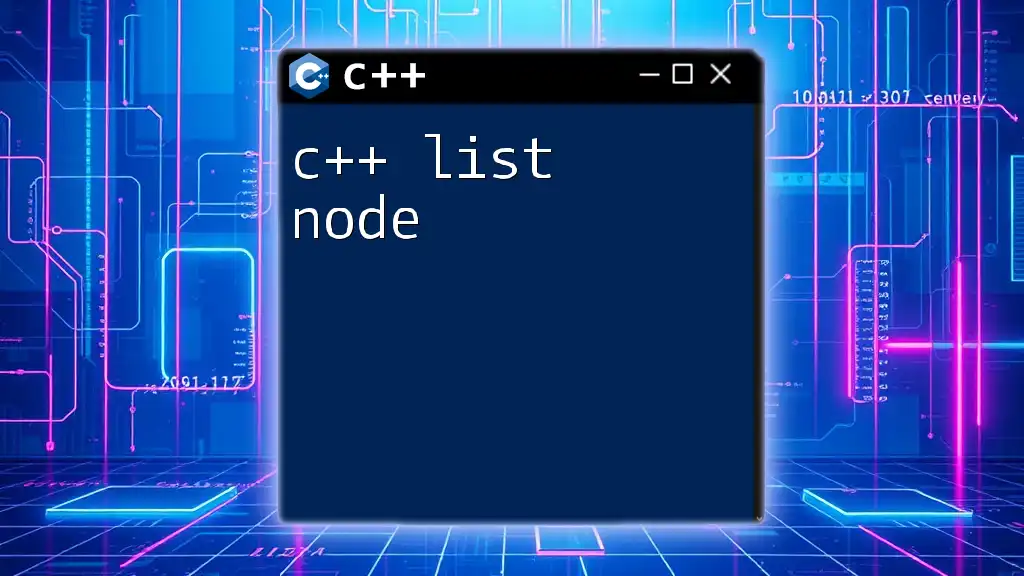
Best Practices for Using C++ Lint Tools
Regular Integration in Development Workflow
To maximize the benefits of C++ lint tools, integrating them into your daily development routine is essential. This can be accomplished by setting up:
- Automated Linting: Configure your build system to run lint checks whenever code is compiled. This practice ensures real-time feedback on code quality.
- Using Pre-commit Hooks: Implement hooks in version control systems like Git that automatically run linting checks before code is committed.
Interpreting Linter Output
When running a linter, developers must understand and respond to the warnings and errors provided in the output. Common types of feedback might include:
- Style Violations: Deviations from defined coding styles that can be easily fixed.
- Performance Suggestions: Tips for improving code efficiency.
Example: If a linter suggests refactoring a piece of code, consider the following:
// Original Code
for (int i = 0; i < my_vector.size(); i++) {
doSomething(my_vector[i]);
}
// Suggested Refactor
for (const auto& item : my_vector) {
doSomething(item);
}
This refactoring improves readability and efficiency by using a range-based for loop.
Customizing Linting Rules
Most C++ lint tools allow for customization to suit your team’s standards. You can often edit configuration files to specify which checks to enable or disable.
Example for Clang-Tidy:
Checks: '-*,clang-analyzer-*'
The above configuration enables only Clang Analyzer checks, ignoring all other warnings.
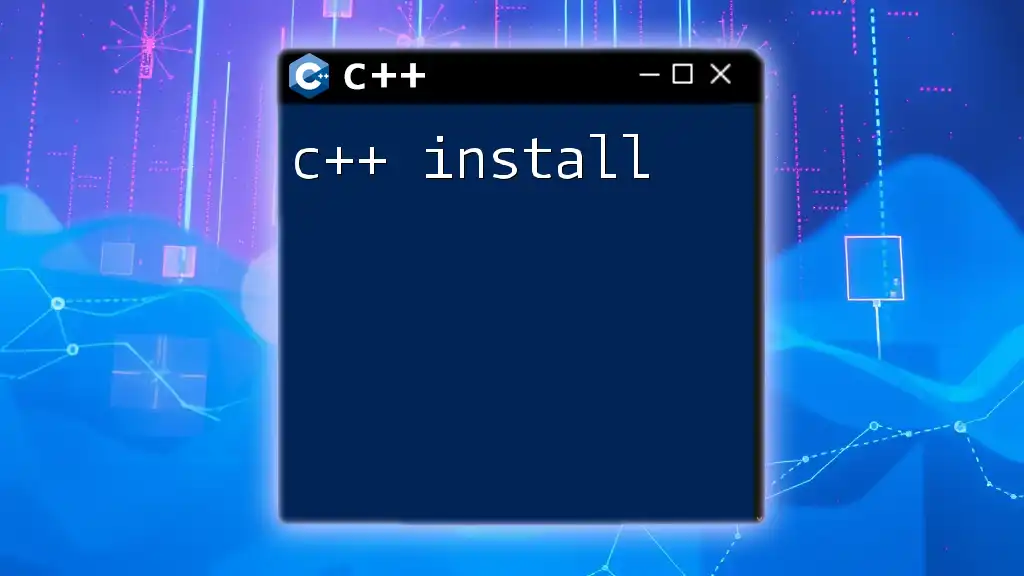
Conclusion
Using a C++ lint tool is crucial for maintaining high code quality and reducing the likelihood of bugs. By understanding the various lint tools available, their functionalities, and how to integrate them into your workflow, you can significantly enhance the quality and maintainability of your codebase.
Start utilizing lint tools today and experience the benefits of cleaner, more efficient, and reliable C++ programming.
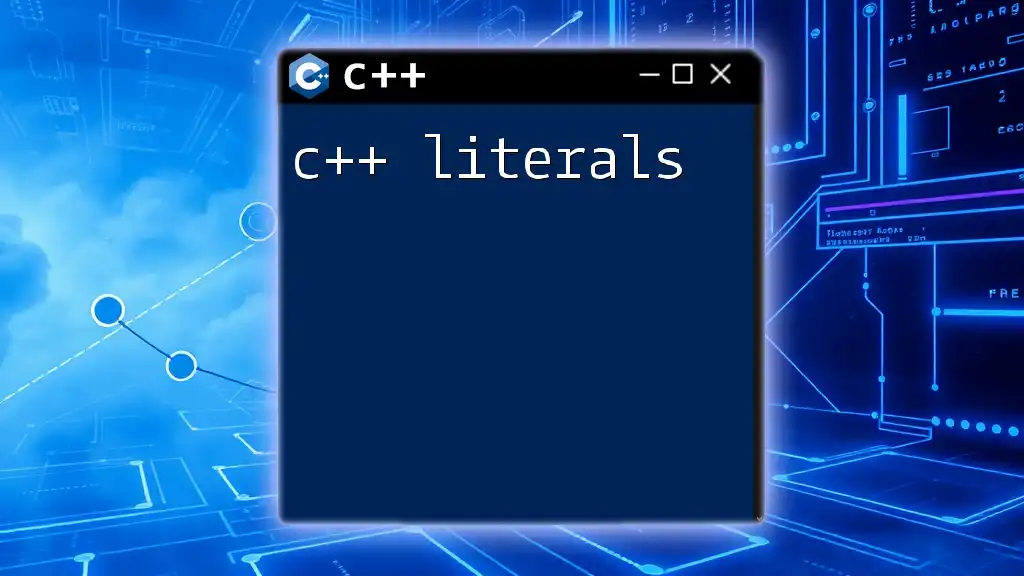
Additional Resources
For further learning and engagement with the C++ community, explore online forums, documentation, and additional resources that can expand your knowledge about C++ linting and coding best practices.
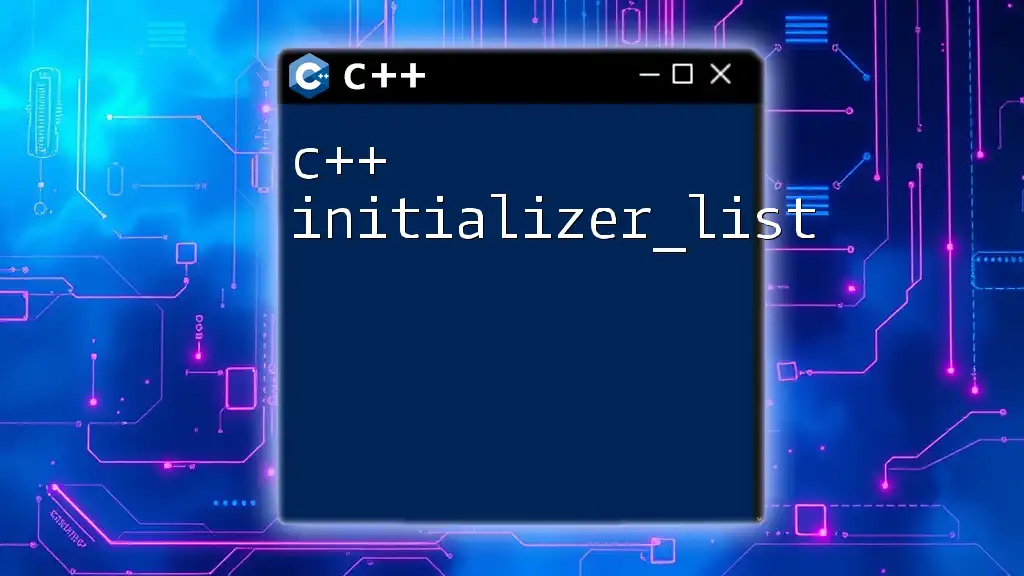
FAQ Section
What is the difference between a linter and a compiler? A linter checks code for stylistic and programming errors, whereas a compiler translates code into executable form without necessarily checking for the quality of the code.
Can lint tools catch semantic errors? While some lint tools can highlight potential semantic issues, they are primarily focused on stylistic and traditional bugs rather than logical flaws.
How can I contribute to improving lint tools? You can contribute by reporting bugs, suggesting new features, or even contributing code to open-source linting projects. Many tools welcome community involvement to enhance their functionality.