In C++, a list node is a fundamental component of linked lists, typically consisting of a data element and a pointer to the next node in the sequence. Here's a basic example of a list node structure in C++:
struct ListNode {
int data; // Data held by the node
ListNode* next; // Pointer to the next node
ListNode(int val) : data(val), next(nullptr) {} // Constructor to initialize the node
};
Understanding C++ List Nodes
What is a List Node?
A list node is a fundamental building block of linked data structures in C++. It serves as a container that holds data and a pointer to the next node in the sequence. This is essential for implementing dynamic data structures, allowing for efficient insertion, deletion, and traversal of elements.
Structure of a List Node
The basic structure of a list node consists of two main components: the data part and the pointer part. The data part holds the information you want to store, while the pointer part connects this node to the next node in the list.
Here’s a simple representation of a list node in C++:
struct ListNode {
int data; // Data part
ListNode* next; // Pointer to the next node
};
In this structure, `data` is an integer, but it can be modified to hold different data types, such as strings or custom objects. The `next` pointer is crucial as it determines the connection within the list, enabling traversal from one node to another.
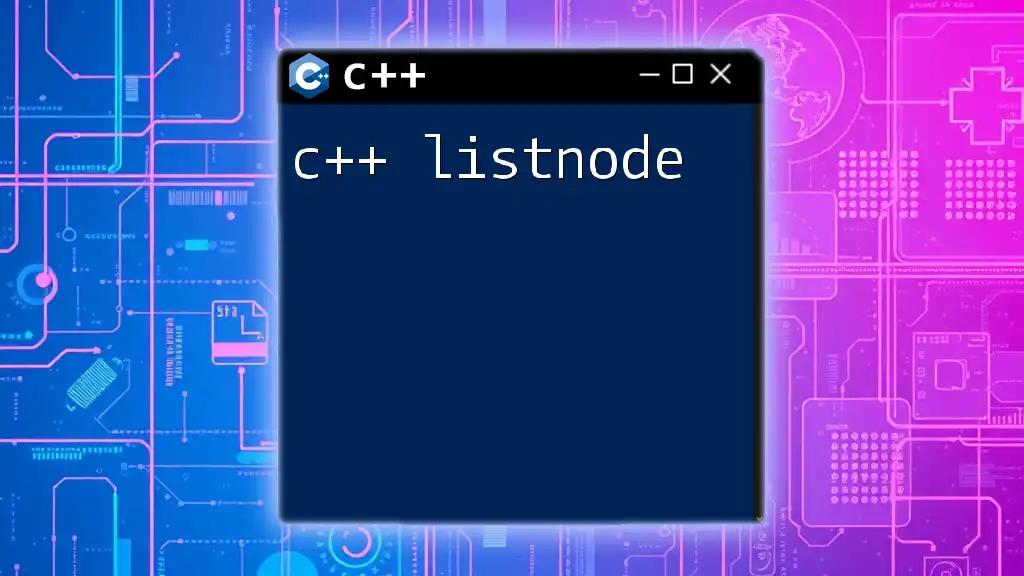
Types of Linked Lists
Singly Linked List
A singly linked list consists of nodes where each node points only to the next node. This structure allows for efficient memory use but only supports forward traversal.
Here’s a basic example of implementing a singly linked list:
class SinglyLinkedList {
ListNode* head; // Pointer to the first node
public:
SinglyLinkedList() : head(nullptr) {}
void insertAtHead(int value);
void insertAtTail(int value);
void displayList();
void deleteNode(int value);
// Additional methods can be defined here
};
The pros of singly linked lists include:
- Simplicity in implementation.
- Less memory overhead per node compared to doubly linked lists.
However, the cons comprise:
- Inefficient traversal if the last node is reached, as backward traversal is not possible.
- Complicated deletion if the previous node needs to be accessed.
Doubly Linked List
A doubly linked list enhances the singly linked list by allowing traversal in both directions. Each node contains two pointers: one pointing to the next node and another to the previous node.
Here is a simple representation of a doubly linked list node:
struct DoublyListNode {
int data;
DoublyListNode* next; // Pointer to the next node
DoublyListNode* prev; // Pointer to the previous node
};
The key advantages of using a doubly linked list include:
- Ability to traverse in both directions, allowing easier deletions when altering the list.
- Efficient insertion and deletion given access to both previous and next nodes.
Despite these strengths, the added complexity and greater memory overhead per node can be seen as the disadvantages of this structure.
Circular Linked List
A circular linked list is a variation where the last node points back to the first node, forming a circle. This can be implemented with either singly or doubly linked nodes.
The primary benefit of circular linked lists lies in avoiding null pointers at the end of the list, enabling continuous traversal of the list without explicit checks for the end.
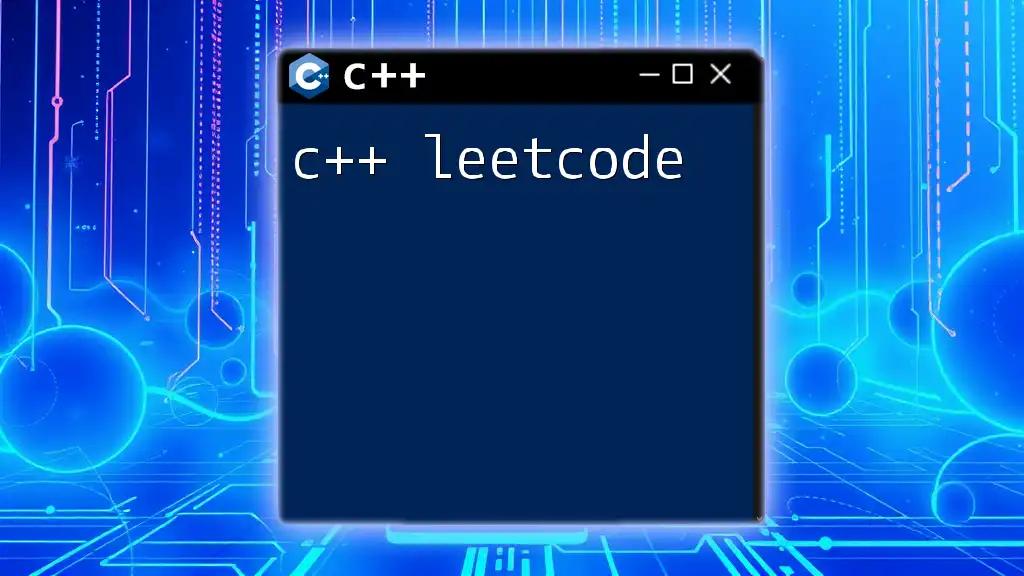
Operations on List Nodes
Insertion in a Linked List
Insertion is a crucial operation for managing a linked list. Here, we’ll focus on two common methods: inserting at the head and at the tail.
Inserting at the Head is often a straightforward operation. By creating a new node and updating its pointer to the current head, the `insertAtHead` function can be defined as follows:
void insertAtHead(int value) {
ListNode* newNode = new ListNode{value, head};
head = newNode;
}
Inserting at the Tail requires traversing the list to find the last node and linking the new node as follows:
void insertAtTail(int value) {
ListNode* newNode = new ListNode{value, nullptr};
if (!head) {
head = newNode;
return;
}
ListNode* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = newNode;
}
Deletion in a Linked List
Deleting a node involves searching the list for the target node and adjusting pointers accordingly.
For example, to delete a node by value, you can use the following code snippet:
void deleteNode(int value) {
if (!head) return; // List is empty
if (head->data == value) {
ListNode* temp = head;
head = head->next;
delete temp;
return;
}
ListNode* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
ListNode* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
Traversing a Linked List
Traversal is vital for accessing the elements in the linked list. Here’s a simple implementation of a traversal function:
void displayList() {
ListNode* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << std::endl;
}
Searching in a Linked List
Searching through a linked list is done by iterating through each node until the target value is found. Here’s a method to search for a node:
bool searchNode(int value) {
ListNode* current = head;
while (current) {
if (current->data == value) return true;
current = current->next;
}
return false;
}
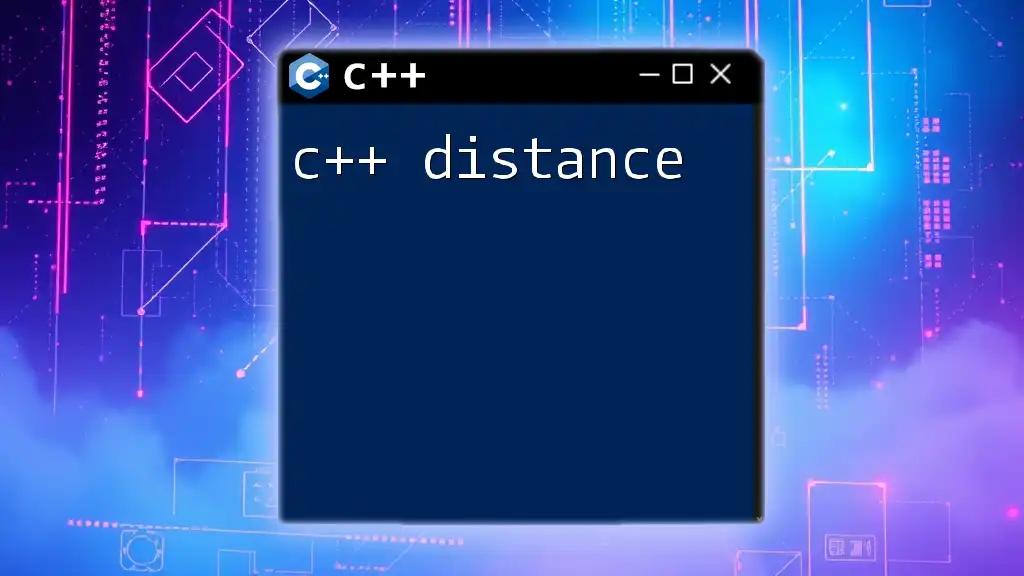
Memory Management in Linked Lists
Dynamic Memory Allocation
In C++, memory allocation for linked list nodes occurs dynamically. Using the `new` keyword allows for creating nodes on the heap, which ensures their lifetime extends beyond the stack scope. However, when nodes are no longer needed, careful deletion is necessary to avoid memory leaks. Always remember to use the `delete` keyword when removing nodes.
Avoiding Memory Leaks
To prevent memory leaks, adhere to best practices such as:
- Always delete nodes once they are no longer required.
- Utilize smart pointers where applicable. For example, using `std::unique_ptr` can automate memory management, thus helping to avoid leaks.
When implementing linked lists with smart pointers, the structure may look like this:
#include <memory>
struct SmartListNode {
int data;
std::unique_ptr<SmartListNode> next; // Smart pointer to avoid leaks
};

Conclusion
In summary, understanding C++ list nodes is crucial for effectively leveraging linked data structures within C++. They facilitate dynamic memory usage and allow for powerful data manipulation through simple yet flexible structures. To refine your skills, experiment with implementing and modifying different types of linked lists.
By equipping yourself with this knowledge, you set a strong foundation for advancing into more complex data structures and algorithms in your programming journey.