In C++, you can determine the size of a list by using the `size()` member function, which returns the number of elements currently stored in the list.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
std::cout << "Size of the list: " << myList.size() << std::endl;
return 0;
}
What is a List in C++?
A list in C++ is a sequence container that allows for the storage of a collection of elements. It is defined in the C++ Standard Library under the header `<list>`. Lists are implemented as doubly linked lists, which means that each element points to both the next and the previous element in the sequence. This structure provides efficient insertion and deletion operations at any point in the list without the need to reallocate or copy the entire container.
Unlike arrays or vectors, lists do not store elements in contiguous memory locations. This allows them to easily grow or shrink when elements are added or removed. However, it also means that accessing elements by index is significantly slower compared to arrays and vectors.
Key features of C++ standard library lists include:
- Dynamic sizing
- Bidirectional iteration
- Storage of duplicate elements
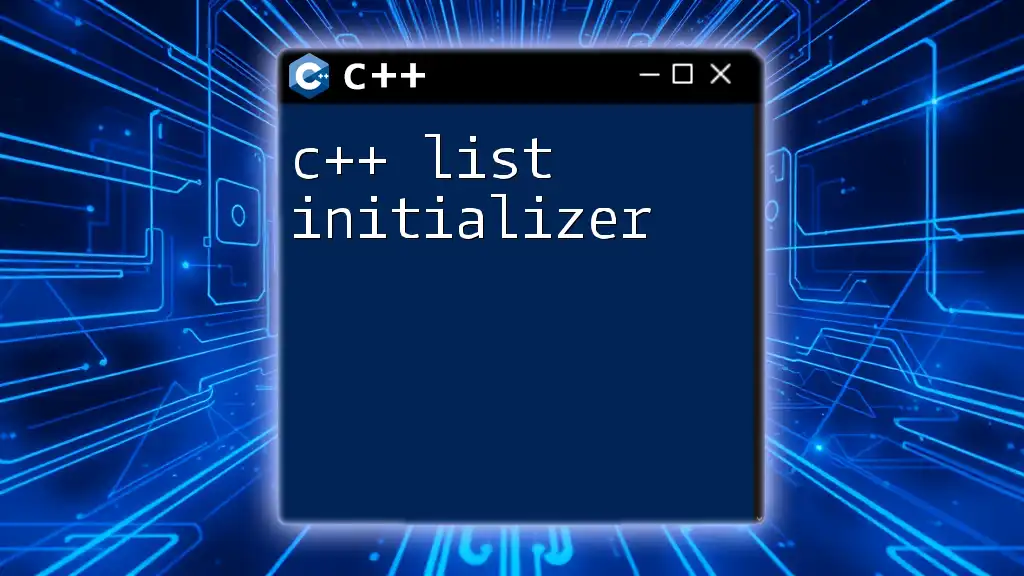
Why Knowing the List Size is Important
Understanding the C++ list size is critical for various programming tasks. Here are a few reasons why it matters:
- Memory Management: Knowing how many elements are in a list can assist in optimizing memory usage.
- Loop Control: When iterating through a list, having the size can help in avoiding unnecessary iterations.
- Data Integrity: Maintaining awareness of the list size can help validate data integrity when adding or removing elements.
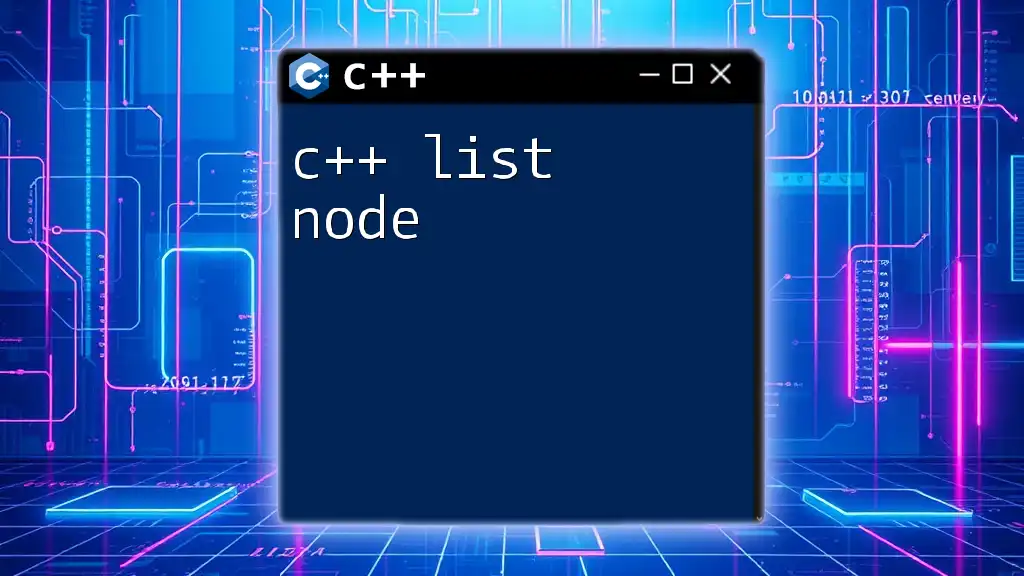
Understanding the `size()` Function
What is the `size()` Function?
The `size()` member function is utilized to retrieve the number of elements in a list. This is essential when you need to make decisions based on how many items are present.
Syntax of the `size()` Function
The basic syntax of the `size()` function can be visualized as follows:
listName.size();
Here’s an example that demonstrates its use:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
std::cout << "Size of the list: " << myList.size() << std::endl;
return 0;
}
In this code, we initialize a list with five integers and then use the `size()` function to print the number of elements in `myList`.
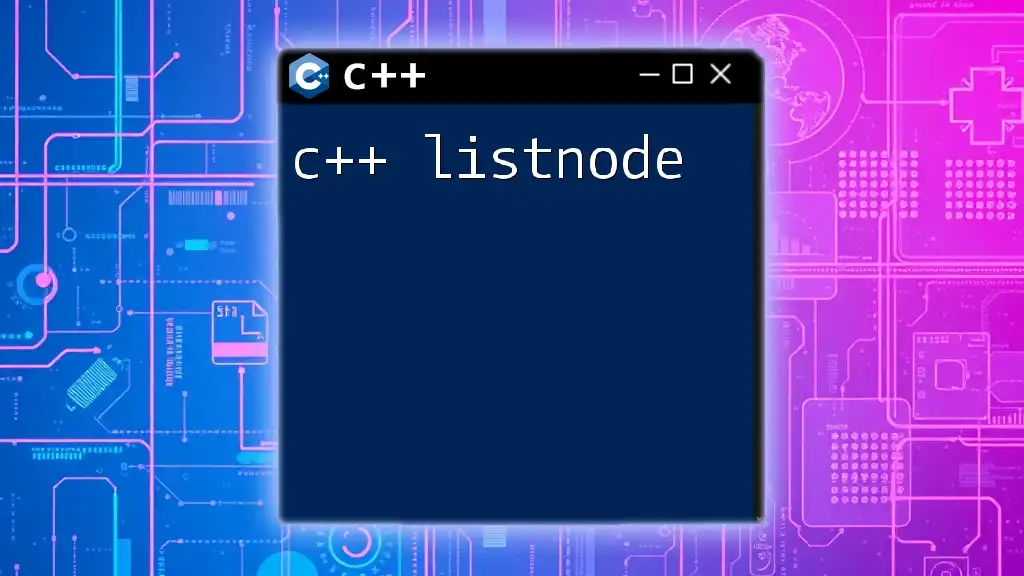
How to Get the Size of a List
Example: Creating and Sizing a List
Creating a C++ list and obtaining its size is straightforward. Here's a step-by-step guide:
- Include the `<list>` header.
- Use the `std::list` constructor to create a list.
- Call the `size()` function.
#include <iostream>
#include <list>
int main() {
std::list<std::string> fruits = {"Apple", "Banana", "Cherry"};
std::cout << "Number of fruits: " << fruits.size() << std::endl; // Output: Number of fruits: 3
return 0;
}
This snippet demonstrates the creation of a list called `fruits` containing three strings. The `size()` function retrieves and outputs the number of items.
Handling an Empty List
When working with lists, it's essential to know how `size()` behaves when you have an empty list. For example:
#include <iostream>
#include <list>
int main() {
std::list<int> emptyList;
std::cout << "Size of empty list: " << emptyList.size() << std::endl; // Output: Size of empty list: 0
return 0;
}
In this code, the `emptyList` has zero elements, and calling `size()` accurately returns `0`.
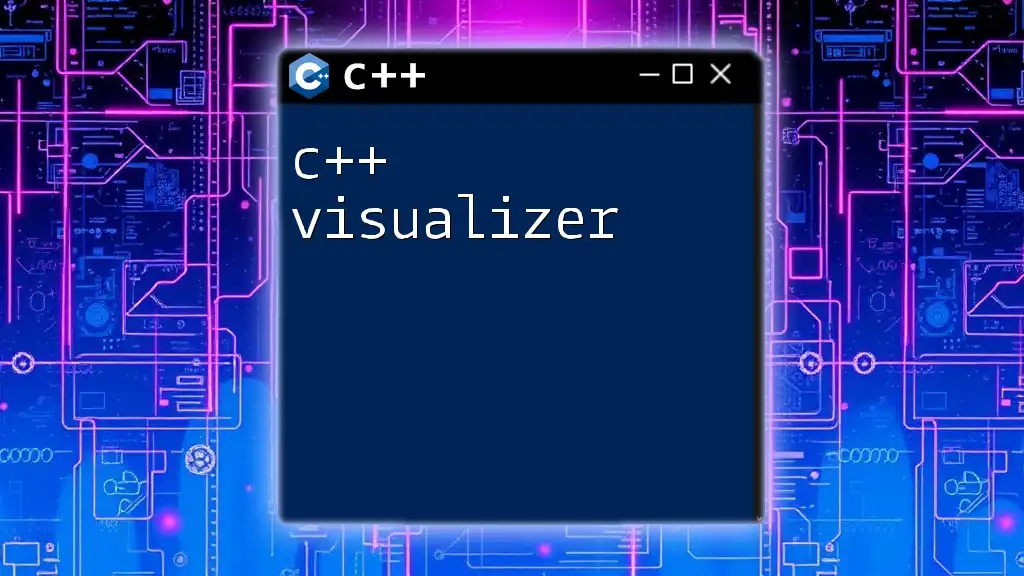
Modifying the List Size
Adding Elements to the List
To work with lists effectively, you often need to add elements. You can use methods such as `push_back()` and `push_front()` to add elements to the end or front of the list, respectively. After each addition, it’s good practice to check the size to verify it reflects the change.
#include <iostream>
#include <list>
int main() {
std::list<int> myList;
myList.push_back(1);
myList.push_back(2);
std::cout << "Size after adding elements: " << myList.size() << std::endl; // Output: Size after adding elements: 2
return 0;
}
Removing Elements from the List
Removing elements can be equally important. You can use functions like `pop_back()` and `pop_front()` for this purpose. Each removal affects the list size as well.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
myList.pop_back();
std::cout << "Size after removing an element: " << myList.size() << std::endl; // Output updated size
return 0;
}
In this snippet, we populate `myList` with five integers and then remove the last one, updating the size accordingly.
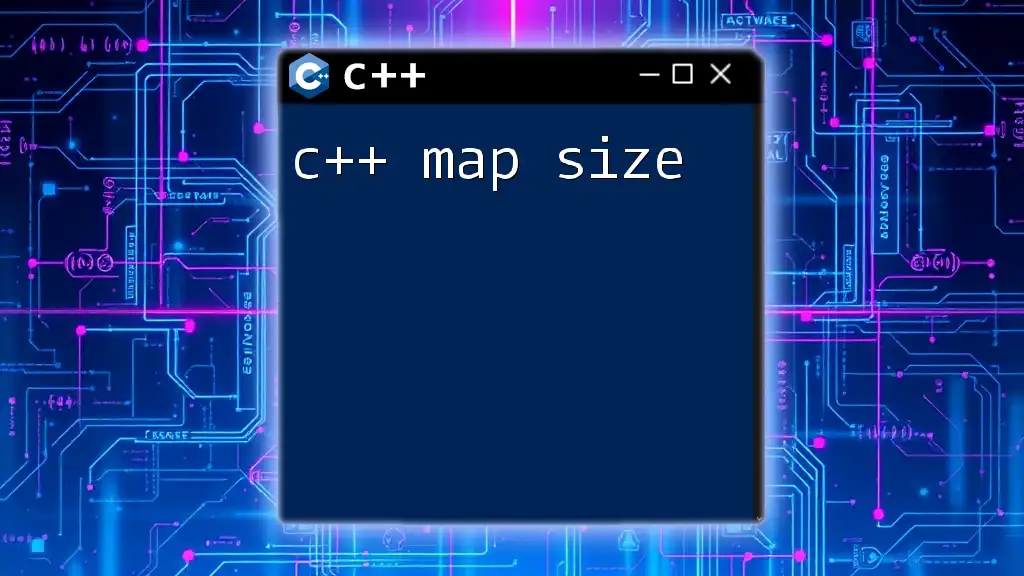
Performance Considerations
Time Complexity of `size()`
One of the advantages of C++ lists is that calling the `size()` function operates in constant time, O(1). This means it retrieves the count of elements without traversing the list, making it efficient.
Best Practices for Managing List Size
While it’s easy to get the size of a list, you should use this feature judiciously. Frequent calls to `size()` can lead to less readable code, especially in loops. Consider storing the size in a variable if you plan to use it multiple times.
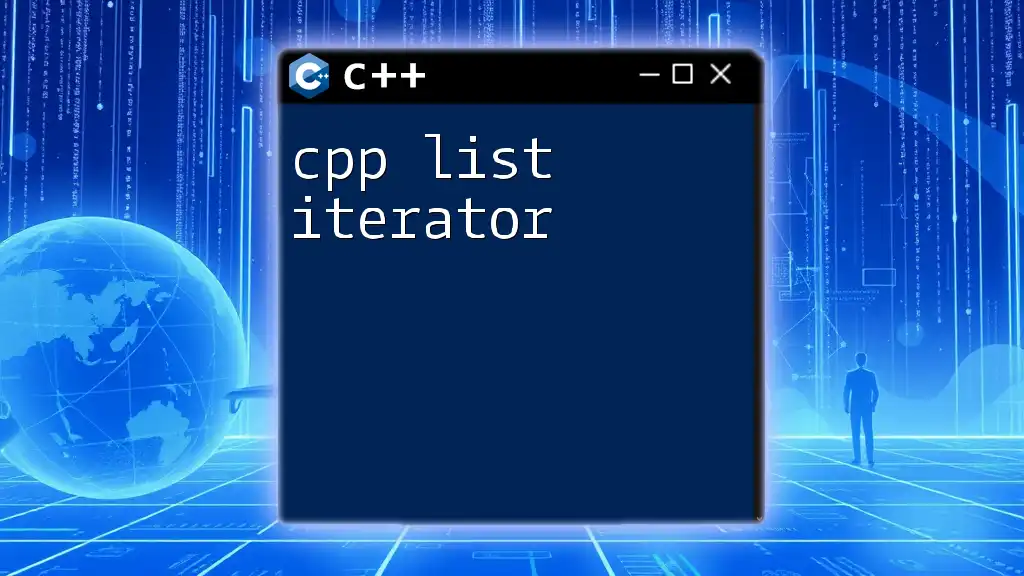
Common Mistakes to Avoid
Misunderstanding List Size
A typical misconception is to assume that the size of a list will automatically change when accessing elements or that it behaves similarly to arrays or vectors. Unlike vectors, lists don’t always guarantee contiguous memory allocation.
Comparison of List Size with Other Containers
It's also essential to understand how list size compares with other containers. For example, while both vectors and lists have a `size()` method, vectors offer direct element access via indices, which makes them faster for certain operations.
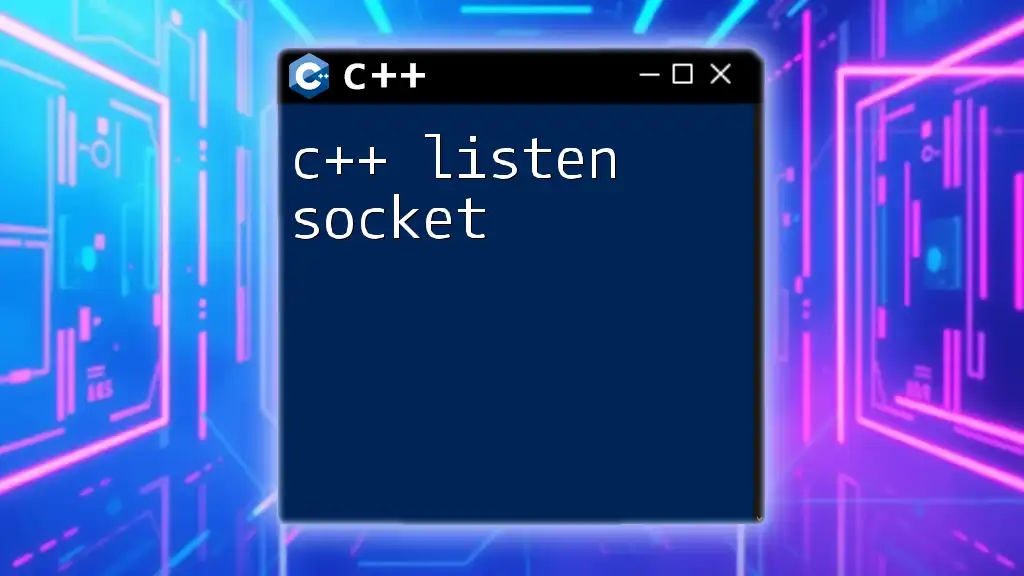
Conclusion
In summary, understanding C++ list size is integral to efficiently working with lists in any C++ application. By mastering the `size()` function, and knowing how to add and remove elements, you can effectively manage your data structures for optimal performance. Happy coding!
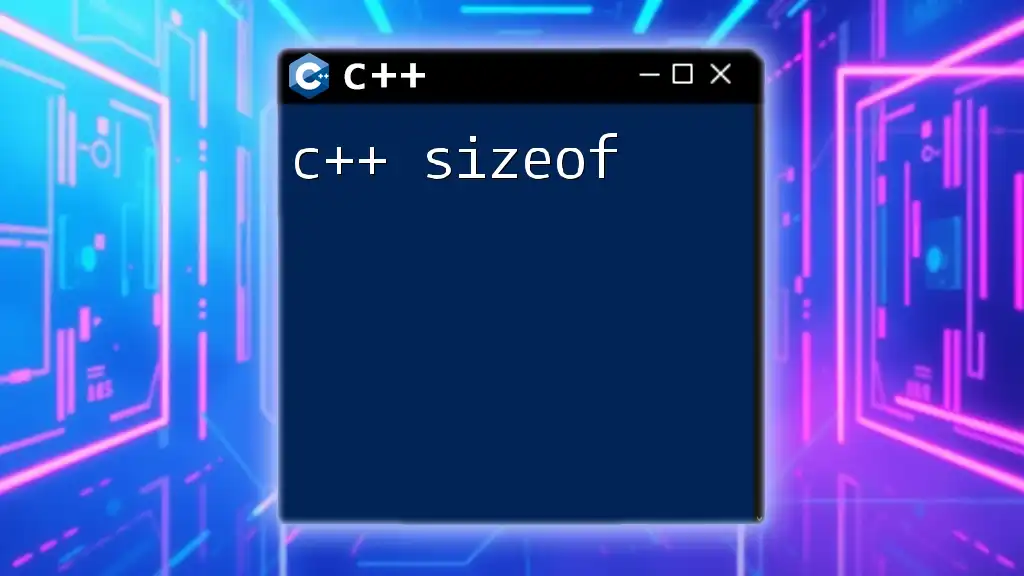
Further Reading and Resources
For those looking to deepen their understanding of C++ lists, consider exploring additional resources such as C++ programming textbooks, online tutorials, and documentation on the C++ Standard Library. These can provide you with more in-depth knowledge and practical applications.