A C++ listen socket is a server-side socket that waits for incoming connection requests from clients, enabling communication in a networked application.
#include <iostream>
#include <cstring>
#include <arpa/inet.h>
#include <unistd.h>
int main() {
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR | SO_REUSEPORT, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
std::cout << "Listening on port 8080..." << std::endl;
return 0;
}
What is a Listen Socket?
A listen socket is a specialized type of socket that is fundamental in establishing a connection between a server and multiple clients in a client-server architecture. Its primary role is to "listen" for incoming connection requests from client sockets. Upon accepting one of these requests, the listen socket may create a separate connection socket specifically for communication with the client, thereby enabling multiple clients to connect concurrently.
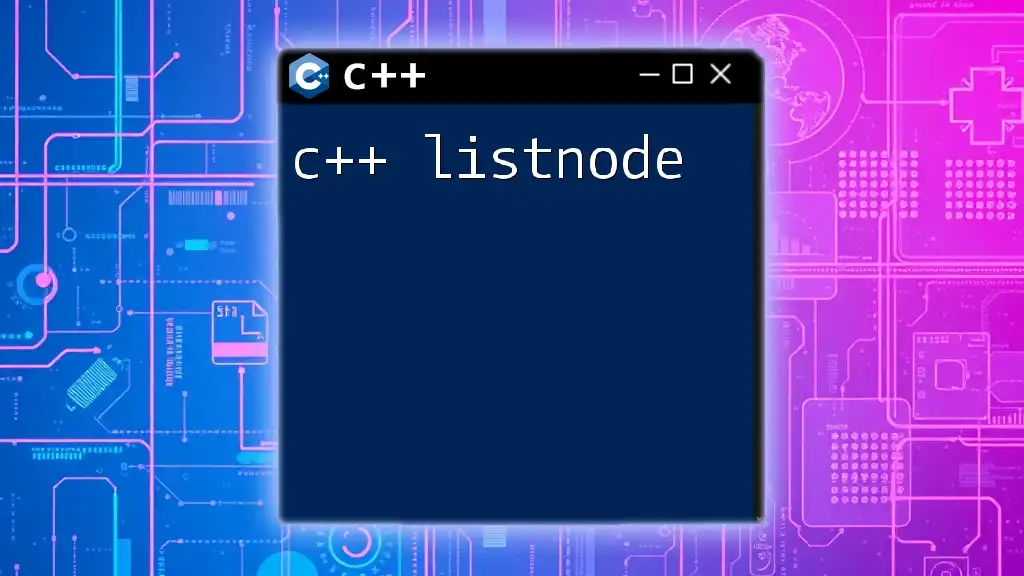
Understanding Socket Programming Basics
What is Socket Programming?
Socket programming is a form of network programming that enables communication between computers over a network. It involves creating a socket, which serves as an endpoint for sending and receiving data across a network. There are two main types of communication in socket programming:
- Connection-oriented (TCP): Utilizes streams of bytes and ensures reliable communication.
- Connectionless (UDP): An efficient method that sends independent packets without ensuring delivery.
Key Concepts in Socket Programming
- Client Socket vs Listen Socket: The client socket initiates a connection to a server, while the listen socket waits for connection requests from clients.
- IP Address and Port Number: The combination of an IP address and a port number uniquely identifies a socket on a network.
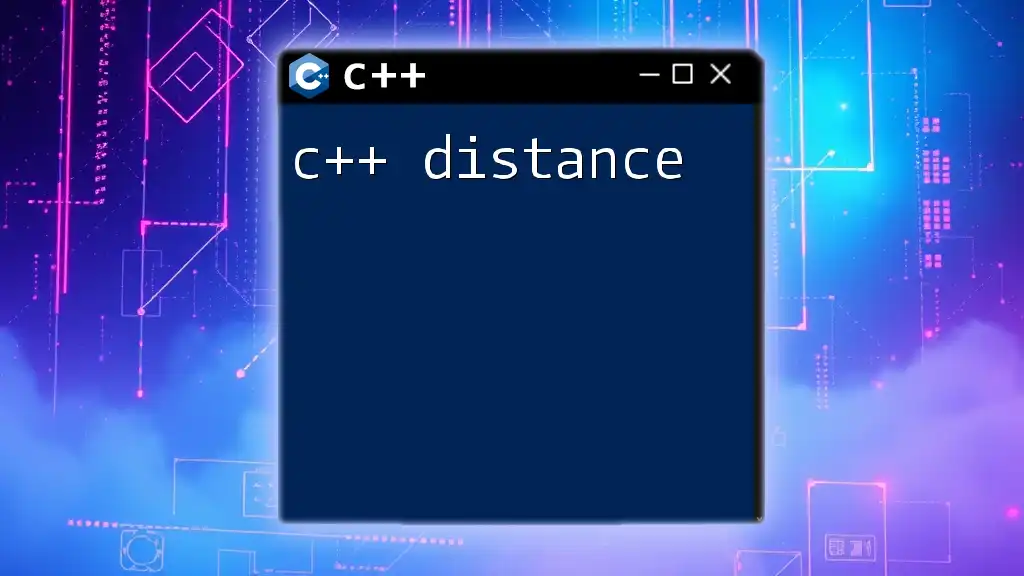
Setting Up a Listen Socket in C++
To effectively create a listen socket in C++, you must include specific libraries comprised of utility functions and definitions that facilitate socket operations. These commonly include:
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
Creating a Socket
The first step in setting up a listen socket involves creating the socket itself. The `socket` function is used to generate a new socket descriptor.
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Error creating socket");
exit(EXIT_FAILURE);
}
In this code, `AF_INET` specifies the address family (IPv4), while `SOCK_STREAM` denotes a TCP socket. The function returns a socket descriptor that is used in subsequent calls. If socket creation fails, the program exits gracefully after printing an error message.
Binding the Socket
Once the socket is created, the next crucial step is to bind it to an IP address and a port number through the `bind` function. This establishes an association between the socket and a specific address.
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = INADDR_ANY; // Accept connections from any IP
server_addr.sin_port = htons(PORT); // Convert port number to network byte order
if (bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr)) < 0) {
perror("Bind failed");
exit(EXIT_FAILURE);
}
In this snippet, `INADDR_ANY` allows the server to accept connections on any available interface. The port number must be converted to network byte order using the `htons` function to ensure it is correctly interpreted by different systems.
Listening for Connections
After binding the socket to an address, it is time to listen for incoming connection requests. The `listen` function is called with the maximum number of pending connections specified.
if (listen(sockfd, SOMAXCONN) < 0) {
perror("Listen failed");
exit(EXIT_FAILURE);
}
The `SOMAXCONN` macro specifies the maximum length of the queue for pending connections. It is a best practice to check the return value of the `listen` function to handle any potential errors.
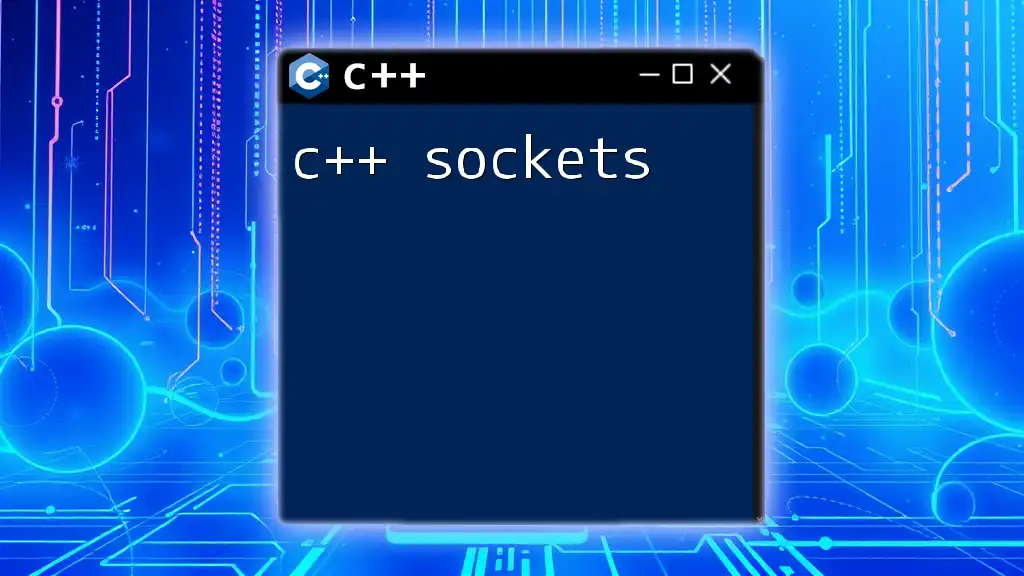
Accepting Connections
Accepting a Client Connection
Once the socket is set to listen, the server can accept client connections using the `accept` function. This function creates a new socket for communication with the connected client.
struct sockaddr_in client_addr;
socklen_t addrlen = sizeof(client_addr);
int client_sock = accept(sockfd, (struct sockaddr*)&client_addr, &addrlen);
if (client_sock < 0) {
perror("Accept failed");
exit(EXIT_FAILURE);
}
Here, `client_sock` becomes the new socket for communication with the client. The `accept` function also populates the `client_addr` structure with the client's details, which can be useful for logging or debugging.
Handling Multiple Clients
For applications that demand support for multiple simultaneous connections, handling each client connection in isolation is vital. Two common strategies are:
-
Multi-threading: Each client connection is handled in a separate thread. This allows simultaneous processing but requires careful management of resources to prevent data corruption.
-
Using `select()`: This technique enables monitoring the status of multiple sockets, allowing the server to interact with multiple clients without the overhead of threading.
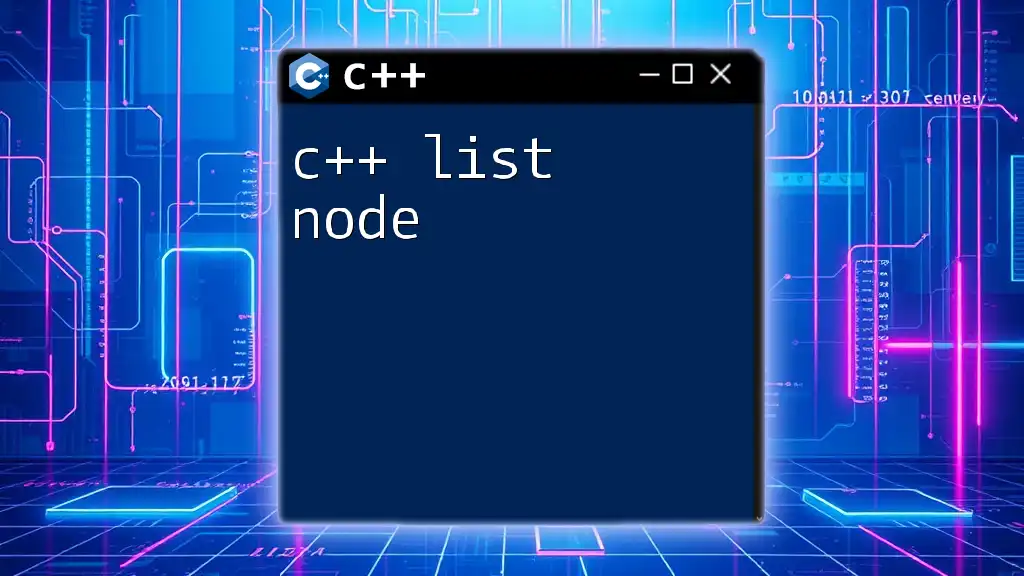
Implementing a Simple Server Example
Here's how you can construct a basic TCP server using the concepts discussed:
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define PORT 8080
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Error creating socket");
exit(EXIT_FAILURE);
}
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = INADDR_ANY;
server_addr.sin_port = htons(PORT);
if (bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr)) < 0) {
perror("Bind failed");
exit(EXIT_FAILURE);
}
if (listen(sockfd, SOMAXCONN) < 0) {
perror("Listen failed");
exit(EXIT_FAILURE);
}
printf("Server is listening on port %d\n", PORT);
while (1) {
struct sockaddr_in client_addr;
socklen_t addrlen = sizeof(client_addr);
int client_sock = accept(sockfd, (struct sockaddr*)&client_addr, &addrlen);
if (client_sock < 0) {
perror("Accept failed");
continue; // Skip to the next iteration
}
char buffer[1024];
memset(buffer, 0, sizeof(buffer));
read(client_sock, buffer, sizeof(buffer));
printf("Received: %s\n", buffer);
write(client_sock, "Message received!", 17);
close(client_sock);
}
close(sockfd);
return 0;
}
In this example, the server listens on port 8080, accepts messages from a connected client, prints the received message, and sends a response. The loop allows handling multiple connections sequentially.
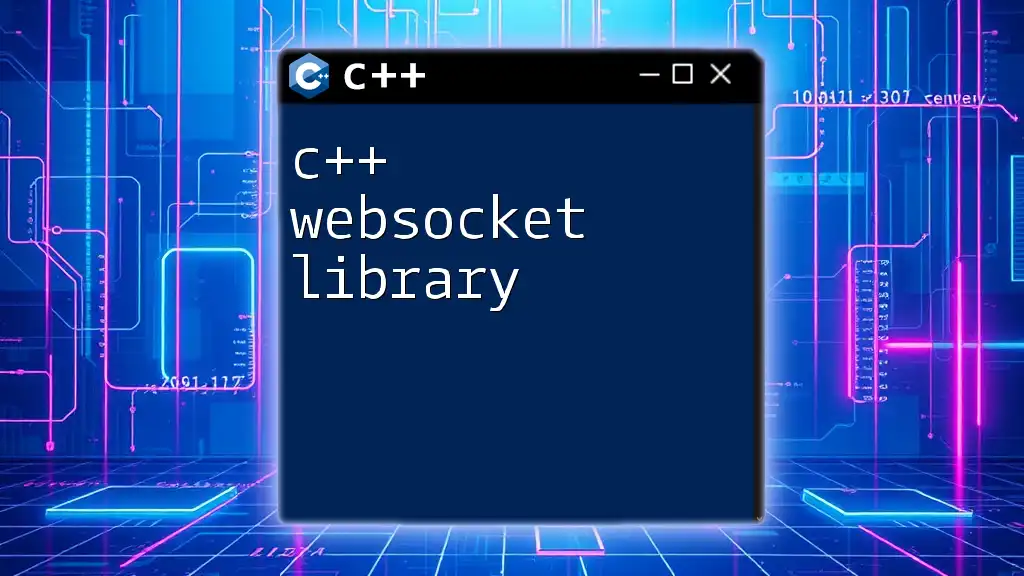
Common Errors and Troubleshooting Tips
When working with C++ listen sockets, you may encounter typical errors. Here’s how to address them:
- Error in Binding: Ensure the port is not already in use and that your program has appropriate permissions to bind to the desired port.
- Connection Issues: Verify that the client can indeed reach the server's IP address and port. Check firewall settings that may block communication.
Implementing error checking in your functions will go a long way in diagnosing problems early.
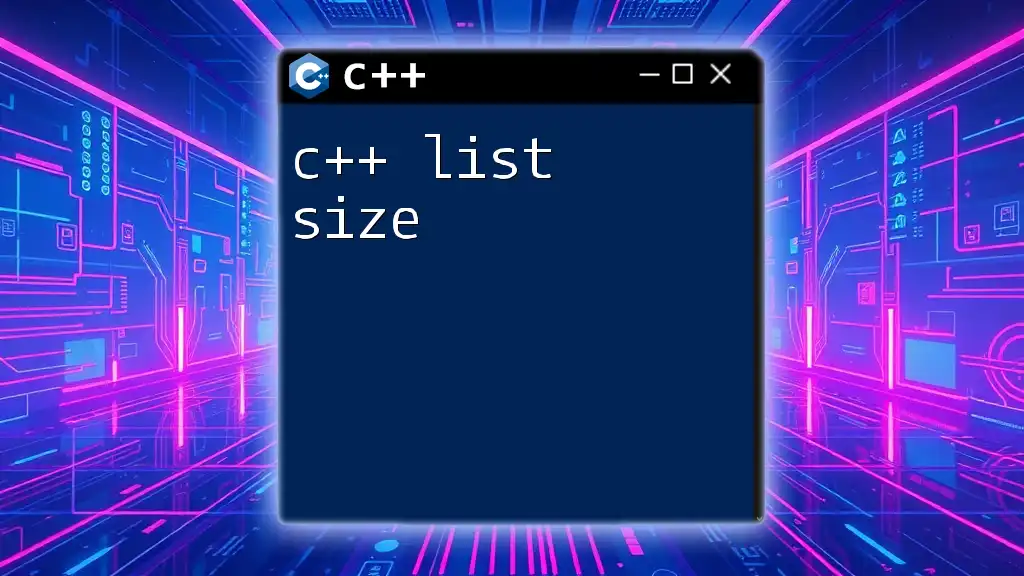
Advanced Topics
Non-blocking Sockets
Using non-blocking sockets allows your application to continue running without waiting for a connection to be established. This can be achieved by setting socket options:
#include <fcntl.h>
fcntl(sockfd, F_SETFL, O_NONBLOCK);
This is especially useful for applications that require high responsiveness, as it eliminates the waiting time that can occur during client connections.
Using Select for Multiple Connections
The `select()` function can monitor multiple sockets, enabling your server to handle multiple client connections efficiently. Here’s a basic implementation:
fd_set master_set, read_fds;
FD_ZERO(&master_set);
FD_SET(sockfd, &master_set);
int max_sd = sockfd;
while (1) {
read_fds = master_set; // Copy the master set
if (select(max_sd + 1, &read_fds, NULL, NULL, NULL) < 0) {
perror("Select error");
exit(EXIT_FAILURE);
}
for (int i = 0; i <= max_sd; i++) {
if (FD_ISSET(i, &read_fds)) { // Activity on this socket
if (i == sockfd) {
// Accept a new client
int new_sock = accept(sockfd, (struct sockaddr*)&client_addr, &addrlen);
FD_SET(new_sock, &master_set); // Add new socket to master set
if (new_sock > max_sd) max_sd = new_sock;
} else {
// Handle existing connection
// ...
}
}
}
}
In this implementation, the server can handle multiple incoming connections without blocking on any single socket.
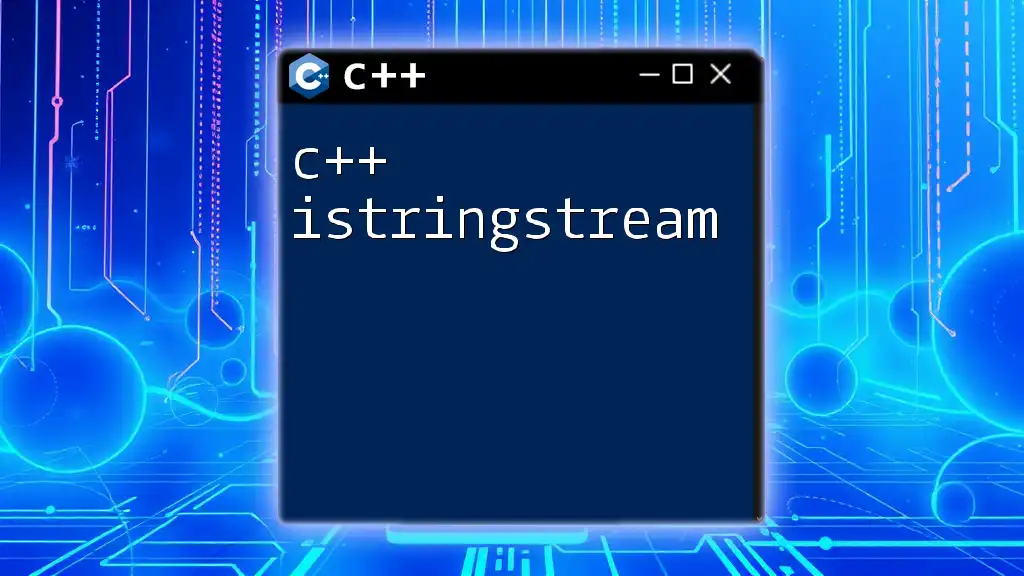
Conclusion
The C++ listen socket is a crucial foundation for building effective client-server applications. Understanding its mechanics, from socket creation to accepting connections, provides the necessary knowledge to build robust network applications. As you advance in your C++ socket programming journey, integrating more sophisticated techniques such as multi-threading, non-blocking sockets, and the `select()` function will enhance your application's scalability and performance.
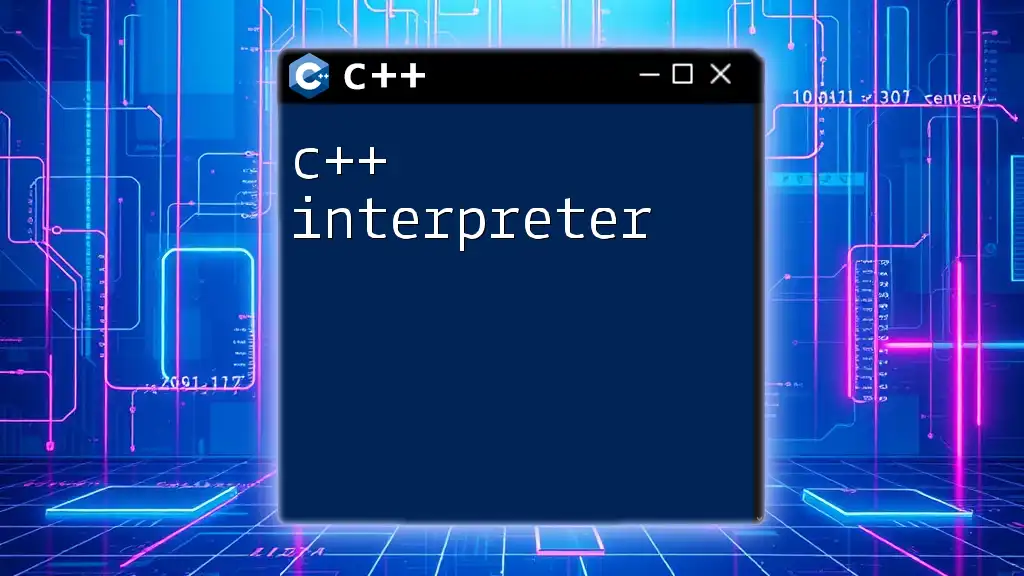
FAQs about C++ Listen Socket
-
What is the difference between UDP and TCP?: UDP is connectionless, does not guarantee delivery, and is faster, while TCP is connection-oriented, ensuring reliable, ordered delivery of data.
-
How do I handle errors effectively in C++ socket programming?: Implement thorough error checks after every socket function call. Use standard error reporting functions like `perror` to understand what went wrong.
-
Can I create a secure server using Listen Sockets?: Yes, implementing SSL/TLS on top of your TCP connections can provide a secure layer for data transmission between client and server. Use libraries like OpenSSL for this purpose.