In C++, the `std::map::size()` function returns the number of key-value pairs in the map, allowing you to determine its current capacity.
Here’s a simple example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "one";
myMap[2] = "two";
std::cout << "Size of myMap: " << myMap.size() << std::endl; // Output: Size of myMap: 2
return 0;
}
Understanding C++ Maps
What is a Map in C++?
A map in C++ is a key-value data structure that allows for efficient storage and retrieval of data. It is part of the C++ Standard Template Library (STL), which offers several powerful container classes for managing collections of objects. Each element in a map consists of a key and a value, where keys are unique. This means that if you try to insert a new element with an existing key, the old value will be replaced.
Maps maintain the order of elements based on the keys in sorted order. This distinguishes maps from other container types like vectors, which maintain the order of insertion without any specific sorting.
Characteristics of C++ Maps
Maps have some defining features:
- Key-Value Pairs: Each element consists of one key and its associated value.
- Ordered and Unique Keys: Keys in a map are stored in sorted order, and each key must be unique.
- Memory Management and Performance Considerations: Maps in C++ use tree-like structures (typically red-black trees) which allow for logarithmic time complexity for search, insertion, and deletion, making them efficient for a variety of applications.

Getting Started with C++ Maps
How to Include Maps in Your Program
To use maps in your program, you need to include the appropriate header from the C++ Standard Library. This can be done using the following line:
#include <map>
The Standard Library is crucial for C++ developers as it provides a wealth of useful features, including maps, which can significantly reduce development time.
Initializing a Map
You can initialize a map in multiple ways, providing flexibility based on your needs. Here are a few common methods:
- Using Constructors: You can declare a map without initializing it first.
std::map<int, std::string> myMap;
- Using Initializer Lists: This is a more concise method to create and initialize a map at the same time.
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
In this example, the map `myMap` is initialized with three key-value pairs.

Working with Maps
Common Operations on Maps
Inserting Elements
Inserting elements into a map can be accomplished in several ways:
- Using `insert()`: This method can be called to add new key-value pairs to the map.
myMap.insert(std::make_pair(4, "Four"));
- Using `operator[]`: This operator allows you to insert a new key-value pair or update an existing key.
myMap[5] = "Five"; // Inserts a new element with key 5, value "Five"
Accessing Elements
To retrieve values, you use the keys associated with them. Here’s an example of how to access an element:
std::cout << "Value associated with key 2: " << myMap[2] << std::endl;
It’s important to check whether a key exists before accessing its value to avoid unintended behavior.
Computing the Size of a Map
Using the size() Function
The `size()` function is a key utility in C++ maps. It returns the number of elements currently stored in the map. The syntax is simple and effective:
std::cout << "Size of myMap: " << myMap.size() << std::endl;
This statement will output the current size of `myMap`.
Why Size Matters in Maps
Performance Implications
Understanding the size of your map can directly impact performance. As the number of elements increases, you may need to consider the efficiency of the operations you’re performing. Large maps can lead to increased search and retrieval times, especially if they surpass certain thresholds that might trigger rebalancing.
Real-World Use Cases
In a real-world application, monitoring the size of a map can be essential. For instance, in an inventory management system, a larger map size could indicate increased stock, leading to the need for more sophisticated algorithms for searching or sorting.
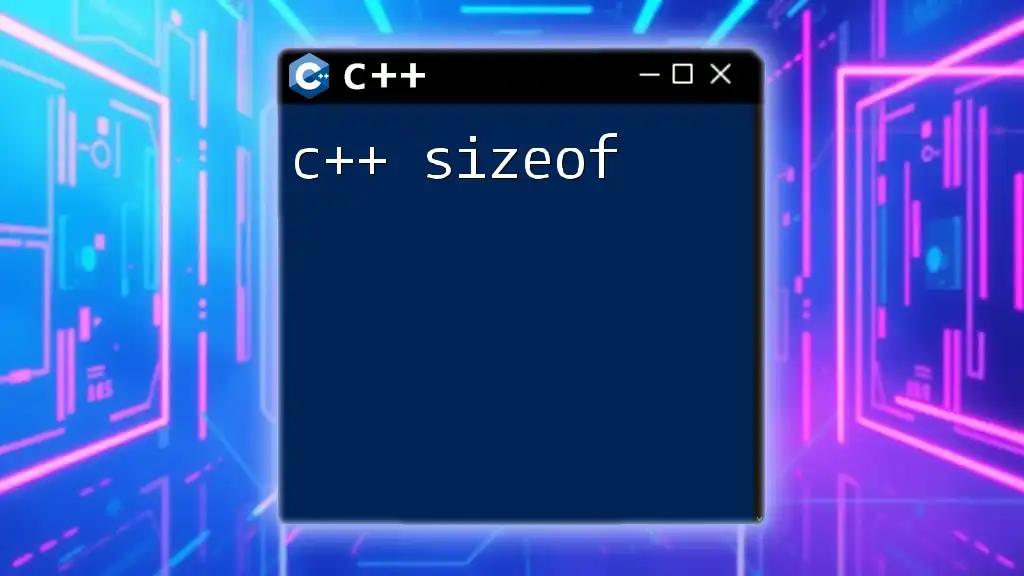
Advanced Concepts with Map Size
Capacity and Size Management
Understanding capacity() vs. size()
While `size()` returns the actual number of elements in the map, the `capacity()` function doesn’t apply directly to maps as it does in vectors. Instead, the focus should be on how the underlying structure handles resizing and performance.
Shrinking a Map
There are scenarios when you may want to clear a map entirely or reduce its size. You can achieve this with the `clear()` function:
myMap.clear();
std::cout << "Size after clearing: " << myMap.size() << std::endl;
Using this function removes all elements, and the size instantly reflects zero.
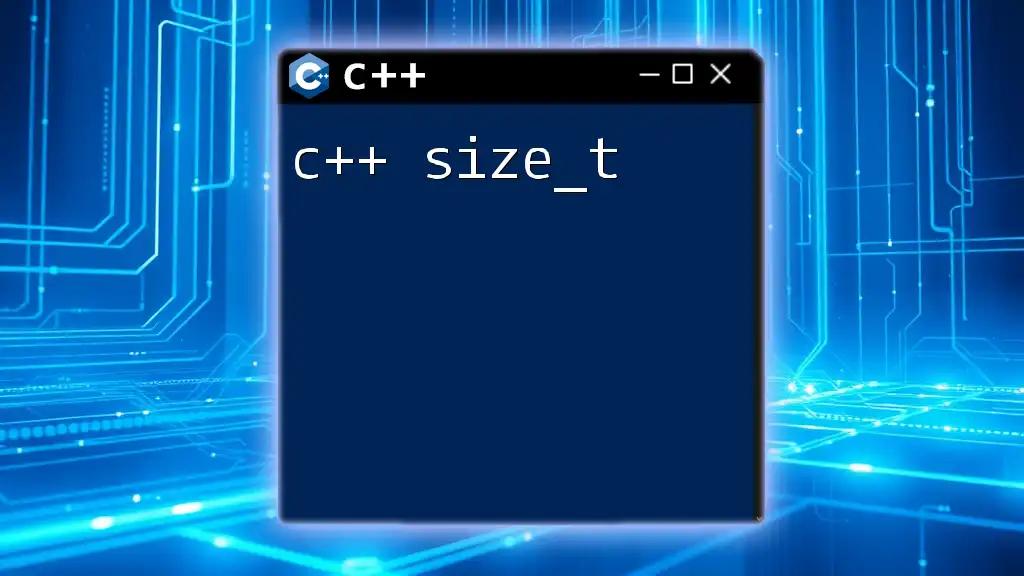
Best Practices for Using Map Size in C++
Efficient Memory Management
When dealing with maps, especially large ones, consider using smart pointers or reserved initial sizes if applicable. This helps manage memory efficiently and can reduce potential reallocations.
Common Pitfalls
One common misconception is confusing the maximum size a map can handle with its current size. Also, in multi-threaded applications, pay attention to concurrent access and modifications to ensure thread safety.
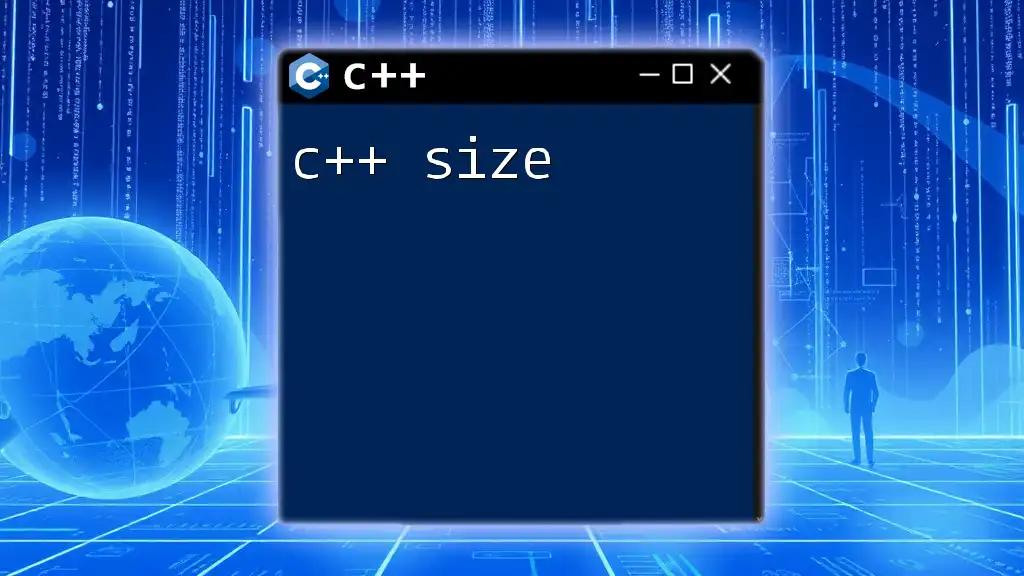
Conclusion
Key Takeaways
Mastering how to work with `c++ map size` is essential for efficient programming. Understanding the operations related to maps, especially how to measure size and implications on performance, equips developers to build efficient and effective applications.
Further Reading and Resources
For those wanting to deepen their understanding, a plethora of resources are available. Books, online tutorials, and the official C++ documentation are excellent places to start your journey into mastering C++ maps and their size functionalities.

Appendix
Example Project
Creating a small application that uses C++ maps effectively can illustrate the concepts discussed in this guide. For a hands-on approach, consider building a simple contact list application where each contact's name is a key and their phone number is the value, showcasing the use of `size()` to display the number of contacts dynamically. Full code listings can be crafted to demonstrate these principles in action.