The `map::at` function in C++ is used to access the value associated with a specific key in a map container, throwing an exception if the key does not exist.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
std::cout << "The value associated with key 2 is: " << myMap.at(2) << std::endl; // Output: Two
return 0;
}
What is a Map in C++?
A C++ map is an associative container that stores elements in key-value pairs. The keys have to be unique, and the data is automatically sorted according to the keys. This structure allows for quick retrieval based on the key, making maps an exceptional choice for many programming tasks.
Why Use Maps?
Maps are particularly useful in scenarios where you need to:
- Access data efficiently: Since keys are indexed, you can quickly retrieve values without having to search linearly.
- Manage collections of related data: Relating keys and values provides a straightforward approach to manage complex data types.
- Maintain sorted order: Maps automatically sort their elements based on the keys, which can simplify many algorithms.
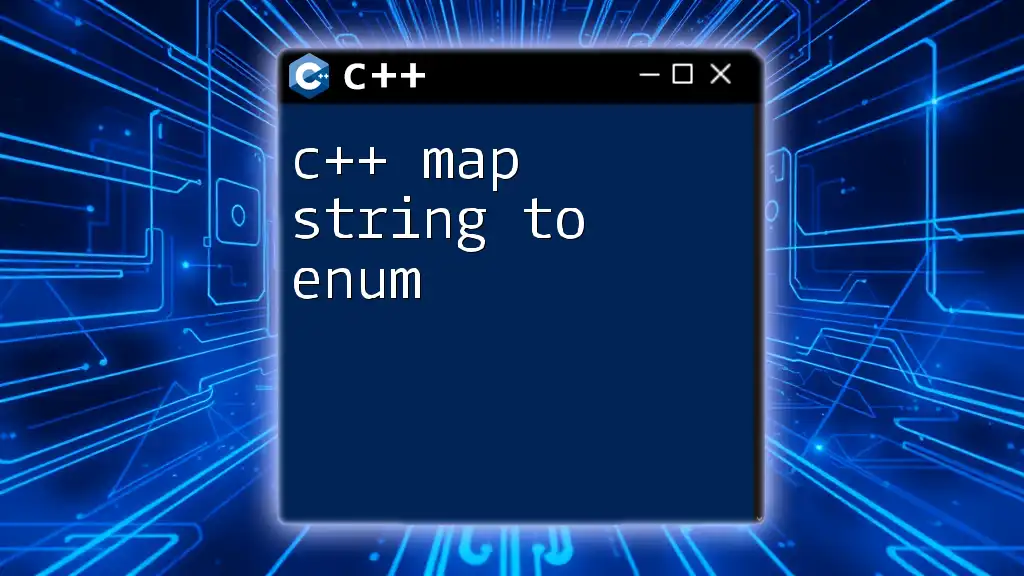
Understanding the `at` Method in C++ Maps
Introduction to the `at` Method
The `at` method is a member function of the C++ map class that allows you to retrieve the value associated with a specific key. This method is beneficial as it provides bounds-checked access to the elements in the map.
Syntax of the `at` Method
The syntax for the `at` method is quite straightforward:
value_type value = myMap.at(key);
Where `myMap` is your map, `key` is the key for which you want to fetch the value, and `value_type` denotes the type of the values stored, which will depend on the specific map definition.
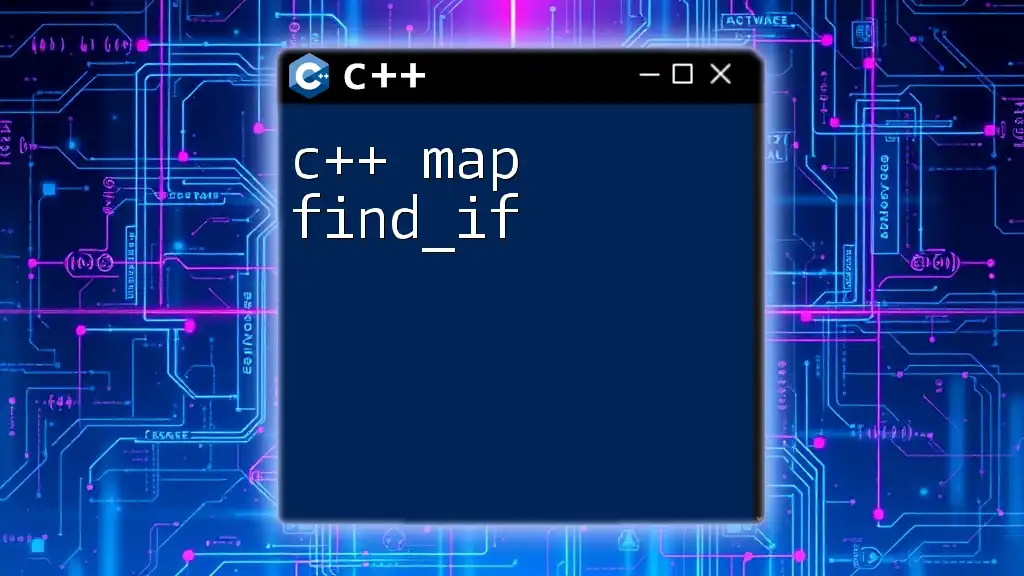
Using the `at` Method: A Step-by-Step Guide
Step 1: Creating a Map
To utilize the `at` method, you first need to create a map. Here’s a simple example of how to declare and initialize a C++ map:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> productInventory = {
{"Apples", 100},
{"Bananas", 50},
{"Cherries", 75}
};
//... further code will go here
}
This code snippet creates a map called `productInventory`, mapping product names (strings) to their quantities (integers).
Step 2: Accessing Elements with `at`
To retrieve values from a map using the `at` method, you simply pass the desired key as follows:
int applesCount = productInventory.at("Apples");
std::cout << "Count of Apples: " << applesCount << std::endl;
In this example, using `at` retrieves the number of apples, enabling efficient and straightforward access to the value associated with the key "Apples".
Step 3: Handling Key Errors
What Happens with Invalid Keys?
When you use `at` with a key that does not exist in the map, it throws an exception. Unlike `operator[]`, which would create a new entry with a default value, `at` helps you avoid silent failures or unintended insertions into your map.
Exception Handling in C++
To handle potential key errors, you can catch the `std::out_of_range` exception like this:
try {
int grapesCount = productInventory.at("Grapes");
} catch (const std::out_of_range &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This code will provide an appropriate error message if you attempt to access a non-existent key, keeping your application robust.
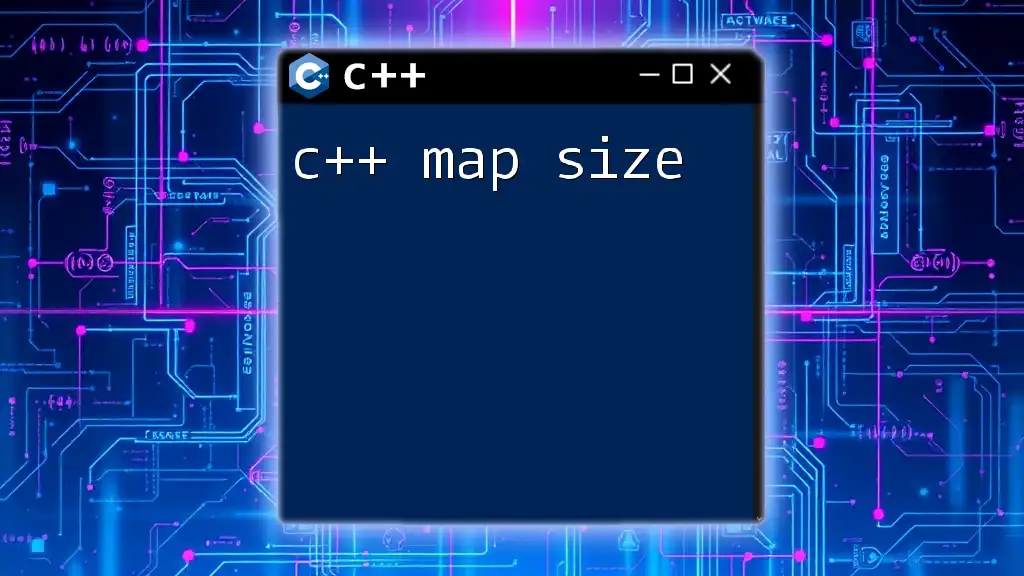
Practical Examples
Example 1: Using `at` to Retrieve Values
Let's say you want to implement a small product inventory system. We can show how to utilize the `at` method effectively:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> productInventory = {
{"Apples", 100},
{"Bananas", 50},
{"Cherries", 75}
};
std::string product = "Bananas";
try {
std::cout << product << " count: " << productInventory.at(product) << std::endl;
} catch(const std::out_of_range &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
This example retrieves the count of bananas from the inventory using the `at` method, demonstrating its effectiveness in access and error handling.
Example 2: Combining Maps and Loops
You can also loop over the entries in a map while using the `at` method, as shown below:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> productInventory = {
{"Apples", 100},
{"Bananas", 50},
{"Cherries", 75}
};
for (const auto &item : productInventory) {
std::cout << item.first << ": " << productInventory.at(item.first) << std::endl;
}
return 0;
}
This code snippet loops through the map, displaying each product and its quantity, effectively demonstrating how to access values dynamically using keys during iteration.
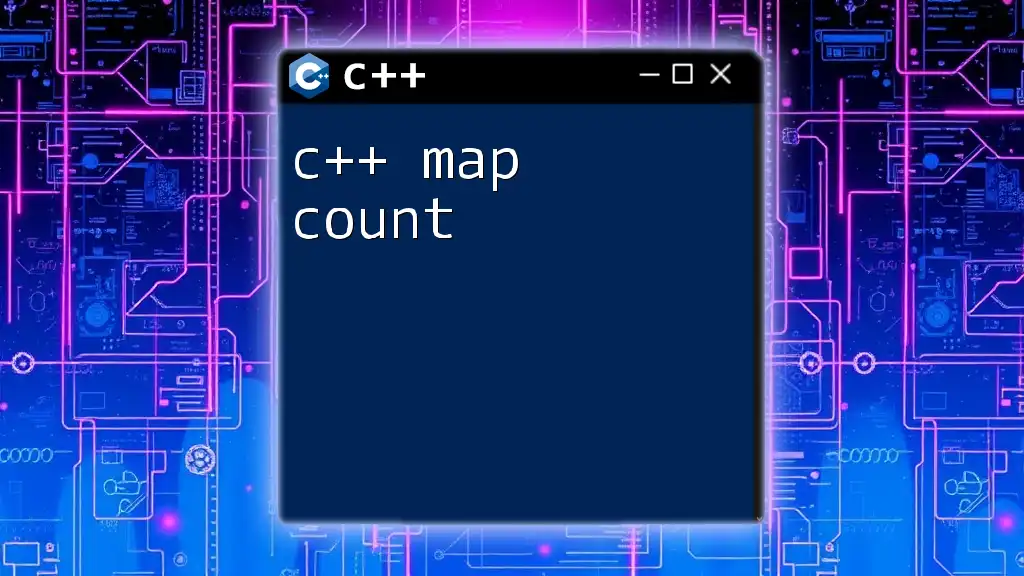
Performance Considerations
Efficiency of the `at` Method
The `at` method has a time complexity of O(log n), which is efficient due to the underlying red-black tree structure used for storing the keys in a map.
Best Practices
When deciding whether to use `at` or `operator[]`, consider:
- Use `at` when you want to enforce key existence before accessing values.
- Use `operator[]` when you're okay with automatically initializing a new entry when the key is not found.
Understanding these methods helps create efficient and bug-free code.
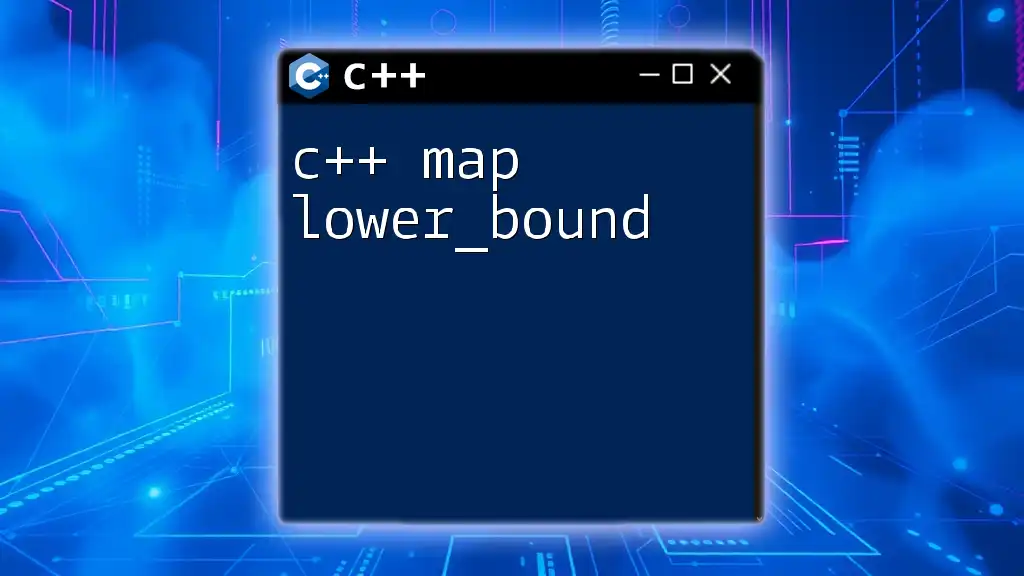
Conclusion
The C++ map `at` method serves as a powerful tool for associative data management. By offering a safe, bounds-checked means of accessing values, `at` enhances both the reliability and performance of your C++ applications.
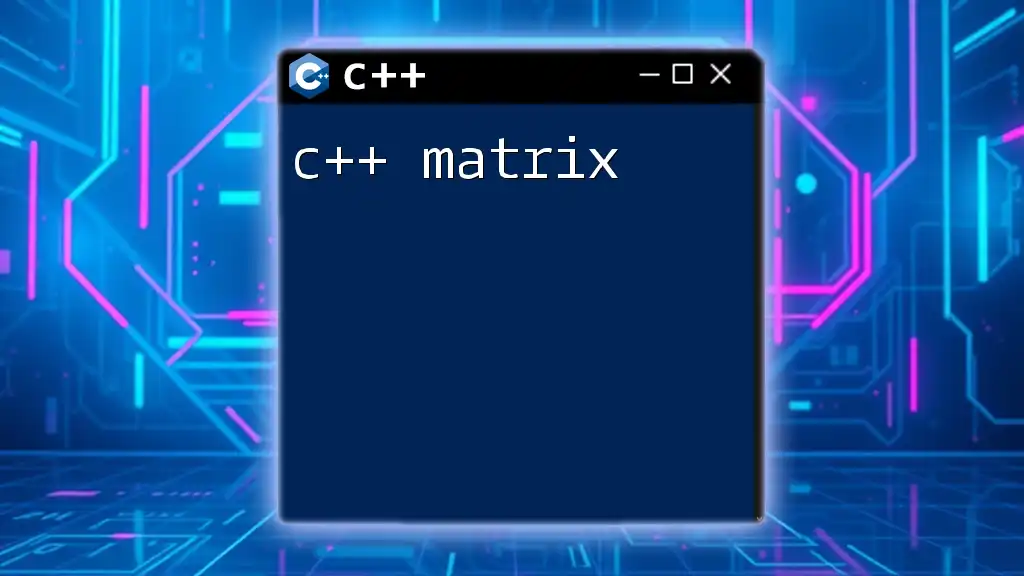
FAQs on C++ Map `at`
What is the difference between `at` and `operator[]`?
`at` throws an exception if the key does not exist in the map, while `operator[]` initializes a key with a default value if it does not exist. This fundamental difference necessitates careful usage, depending on your needs.
Can I use `at` with custom data types?
Yes, you can use `at` with custom data types as long as you are using a valid key type that matches the map's key type.
How does `at` affect memory management in C++ maps?
The `at` method itself does not directly affect memory management; however, it ensures that access to non-existent keys does not lead to memory allocation or undefined behavior. It helps to create safer code with clearer intentions.