The `lower_bound` function in a C++ map returns an iterator pointing to the first element that is not less than a specified key, effectively finding the position where the key could be inserted while maintaining order.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {3, "three"}, {5, "five"}};
auto it = myMap.lower_bound(3);
if (it != myMap.end()) {
std::cout << "The lower_bound for key 3 is: " << it->first << " => " << it->second << std::endl;
}
return 0;
}
Understanding C++ Maps
What is a C++ Map?
A C++ map is a part of the Standard Template Library (STL) that allows the storage of pairs consisting of keys and values. Each key in a map is unique and, as such, allows for efficient retrieval of values. Maps are implemented as self-balancing binary search trees, which means they maintain the order of elements based on the keys.
Characteristics of Maps
- Ordered Storage of Elements: C++ maps store their elements in an ordered fashion based on the key.
- Unique Keys: Each key in a map is unique, which means you cannot have duplicate keys. If you insert a key that already exists, the current value will be replaced.
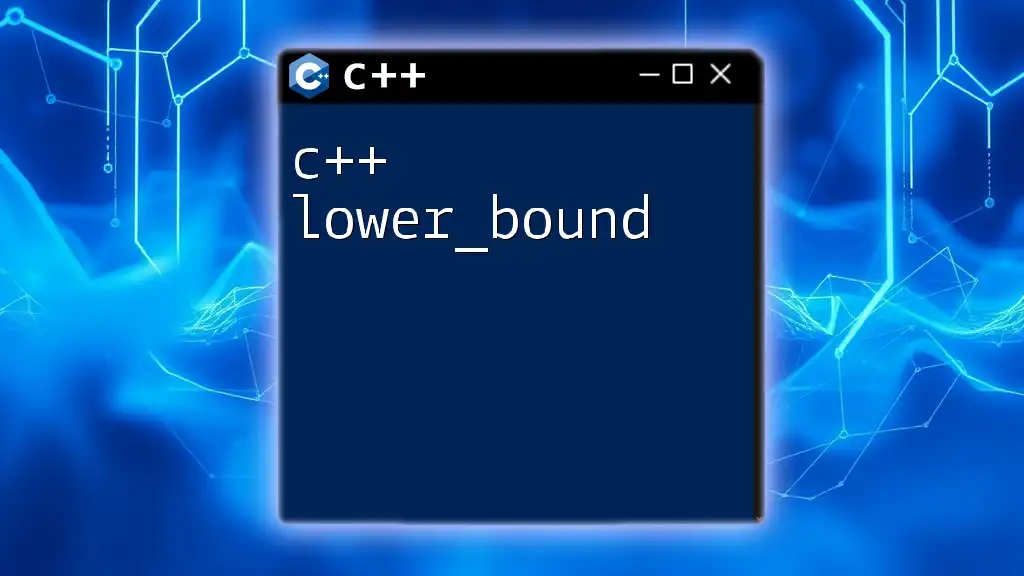
The `lower_bound` Function
Definition and Purpose
The `c++ map lower_bound` function is utilized to find the first element in a map that is not less than a given key. This function is an essential tool for searching within the map efficiently, especially when dealing with large datasets, as it leverages the underlying binary search mechanism.
Syntax of `map::lower_bound`
The function is defined with the following syntax:
iterator lower_bound(const Key& key);
const_iterator lower_bound(const Key& key) const;
- The `iterator` is used to traverse the elements in the map, while `const_iterator` is used for read-only access.
- The `Key` parameter is the key for which you want to search.
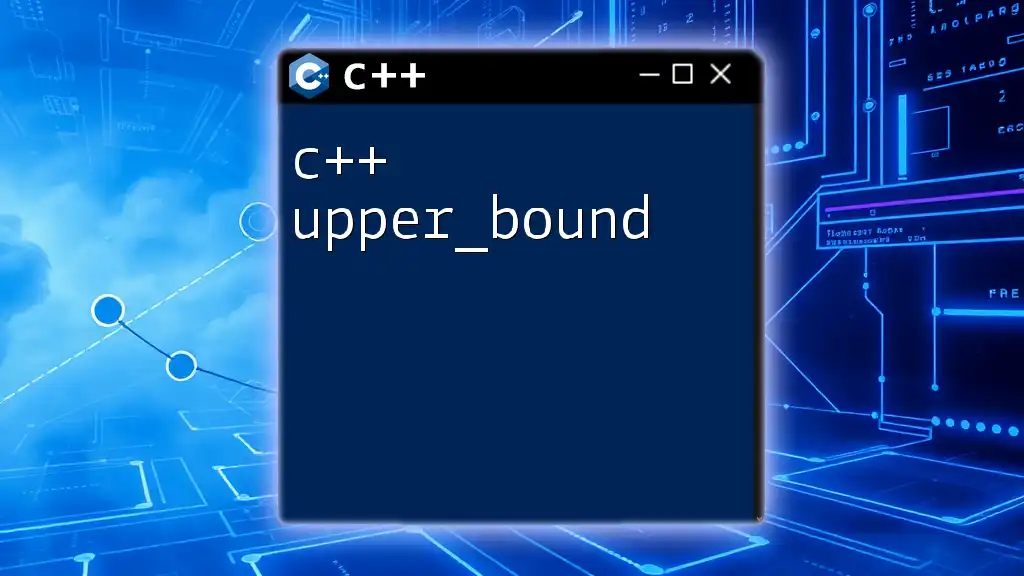
How `lower_bound` Works
Mechanism of Action
The `lower_bound` function performs a binary search to locate the position of the first element that is not less than the provided key. This mechanism allows you to achieve efficient searching, with a time complexity of O(log n).
Return Values
The return value of `lower_bound` is an iterator:
- If there is at least one element in the map that is not less than the provided key, `lower_bound` returns an iterator pointing to this element.
- If all elements in the map are less than the key, it returns an iterator pointing to the end of the map.
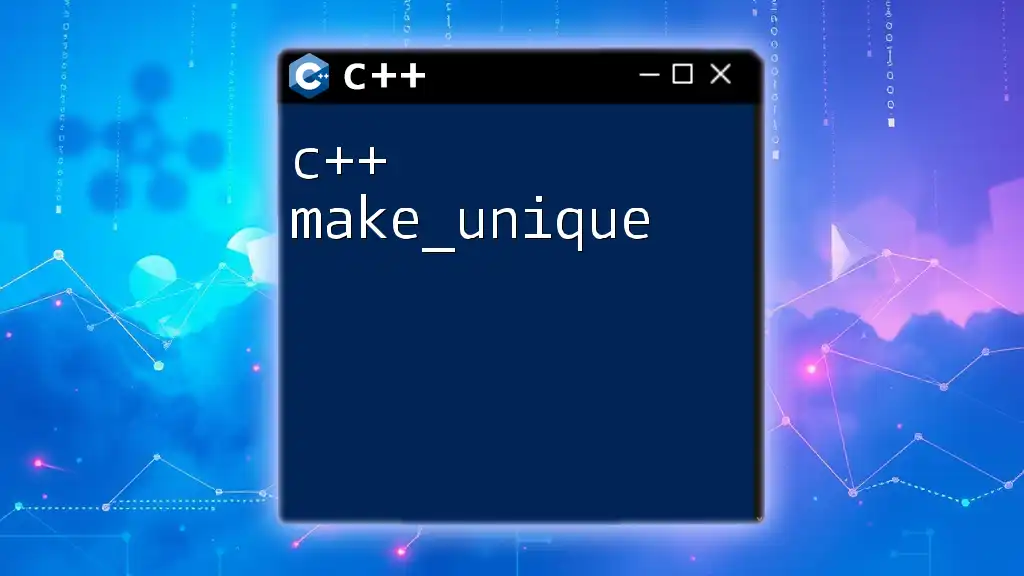
Practical Usage of `lower_bound`
Basic Example
Here's a simple example demonstrating how `c++ map lower_bound` works:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "Apple"},
{3, "Banana"},
{5, "Cherry"}
};
auto it = myMap.lower_bound(3);
if (it != myMap.end()) {
std::cout << it->first << ": " << it->second << std::endl; // Outputs: 3: Banana
}
}
In this example, we created a map called `myMap` with three key-value pairs. When we used `lower_bound` with the key `3`, the returned iterator points to the element with key `3`, which is `"Banana"`. If we were to query a key that did not exist in the map but was less than `3`, such as `2`, it would return an iterator pointing to `3`.
Advanced Example with User Input
Let’s explore a more dynamic example that prompts user input:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "Apple"},
{3, "Banana"},
{5, "Cherry"},
{7, "Date"},
{9, "Fig"}
};
int key;
std::cout << "Enter a key: ";
std::cin >> key;
auto it = myMap.lower_bound(key);
if (it != myMap.end()) {
std::cout << it->first << ": " << it->second << std::endl;
} else {
std::cout << "No elements greater than or equal to " << key << std::endl;
}
}
In this code, the user is prompted to enter a key. The program then calls `lower_bound` with this key and displays the corresponding value or a message indicating that no elements are greater than or equal to the input key.
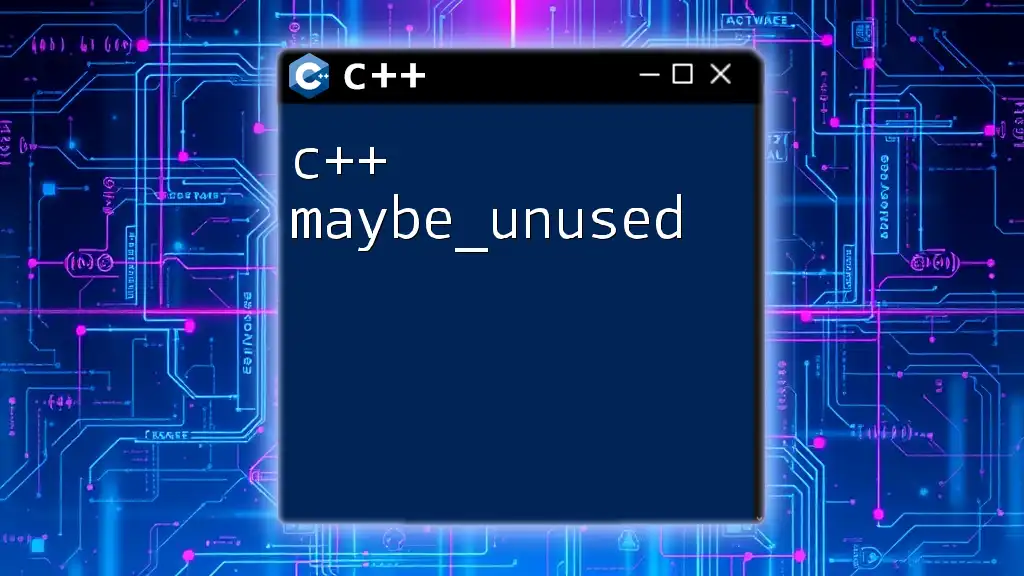
Common Errors and Troubleshooting
Misunderstanding Return Values
One common mistake when working with `c++ map lower_bound` is misunderstanding the return values. Developers often assume it returns a pointer to the exact match. However, it points to the first element that is not less than the specified key, which can lead to unexpected results if not properly understood.
Off-By-One Errors
Another frequent issue is the off-by-one error, where users forget about the possibility of the iterator pointing to the end of the map. Always check the iterator against `myMap.end()` to avoid dereferencing a null iterator.
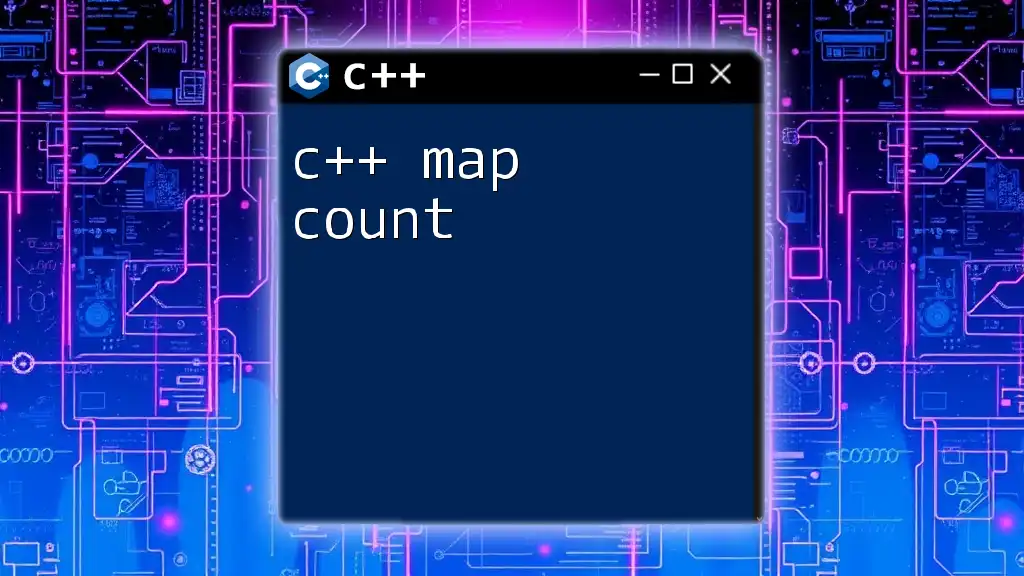
Conclusion
The `c++ map lower_bound` function is an invaluable tool in the C++ Standard Template Library, serving as a means to efficiently locate elements in a map. By practicing its use in various scenarios, developers can harness its full potential for effective key-based searches within maps.
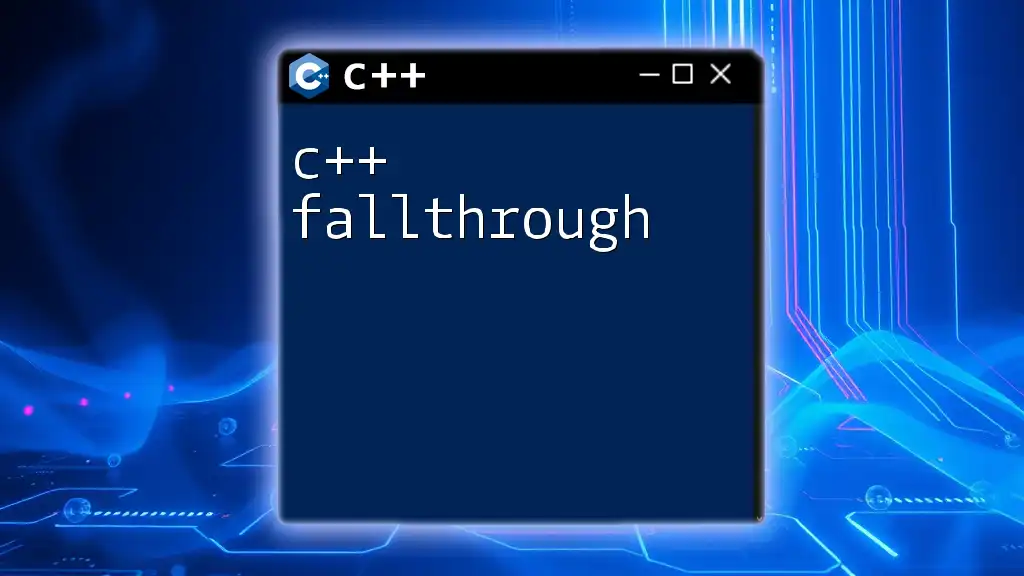
Additional Resources
For further reading, consider exploring the official [C++ documentation](https://en.cppreference.com/w/cpp/container/map/lower_bound) or take on exercises to solidify your understanding of `lower_bound`.
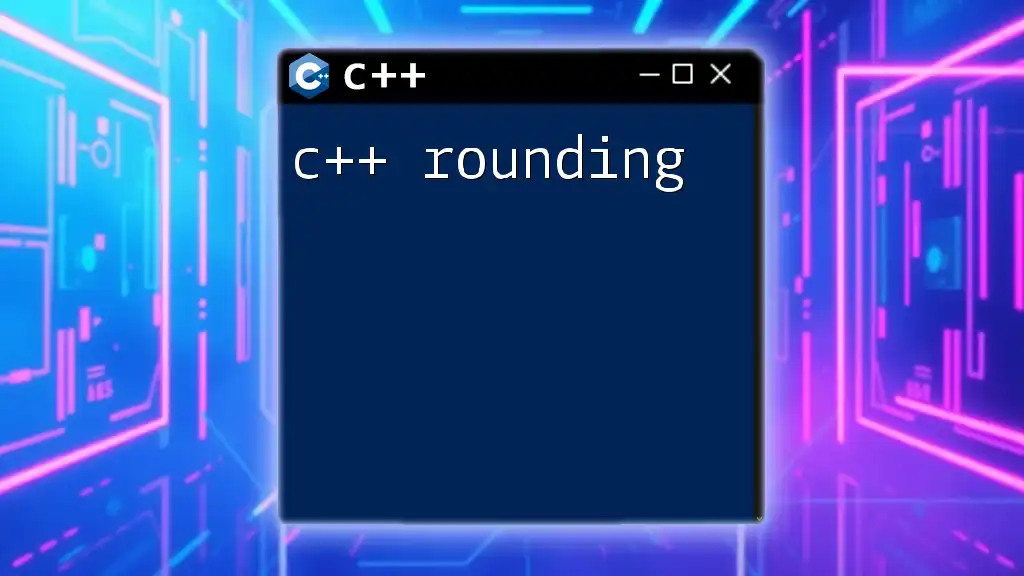
FAQ
What is the difference between `lower_bound` and `upper_bound`?
While `lower_bound` returns the iterator to the first element that is not less than the provided key, `upper_bound` gives you the iterator to the first element that is strictly greater than the key. Understanding this distinction is crucial for correctly applying these functions in various situations.
Can `lower_bound` be used with other STL containers?
Yes, the `lower_bound` function can also be used with other STL containers, such as `set`. Like maps, sets are ordered, and `lower_bound` efficiently finds elements according to the same principles.