In C++, the maximum value for a double precision floating-point variable can be obtained using the constant `DBL_MAX` defined in the `<cfloat>` library.
#include <iostream>
#include <cfloat>
int main() {
std::cout << "Maximum double value: " << DBL_MAX << std::endl;
return 0;
}
Understanding the Double Data Type in C++
What is a Double in C++?
In C++, the double data type is used to represent floating-point numbers, offering a higher precision than the float type. A double typically uses 64 bits of memory, which allows for a wider range of values and significant digits. This makes it ideal for computations that require greater accuracy, such as scientific calculations, financial modeling, and simulations.
Size and Precision of Double
A double provides approximately 15 to 17 decimal digits of precision. This means it can store very large or very small numbers with a reasonable degree of accuracy. While this is generally sufficient for most applications, it’s crucial to remember that floating-point arithmetic may introduce rounding errors during calculations.

c++ Double Max Value
What is the Maximum Double Value in C++?
The maximum value a double can hold is defined in the C++ Standard Library as `DBL_MAX`. This constant represents the largest finite value that a double-type variable can store, helping developers avoid errors from exceeding limits during computation.
How to Access the Maximum Double Value
To access the maximum double value in your C++ program, you first need to include the header file `<cfloat>`. Here’s how to implement it:
#include <iostream>
#include <cfloat>
int main() {
std::cout << "Maximum double value: " << DBL_MAX << std::endl;
return 0;
}
When you run this code, it will output the maximum value that a double can represent, specifically 1.7976931348623157 × 10^308.
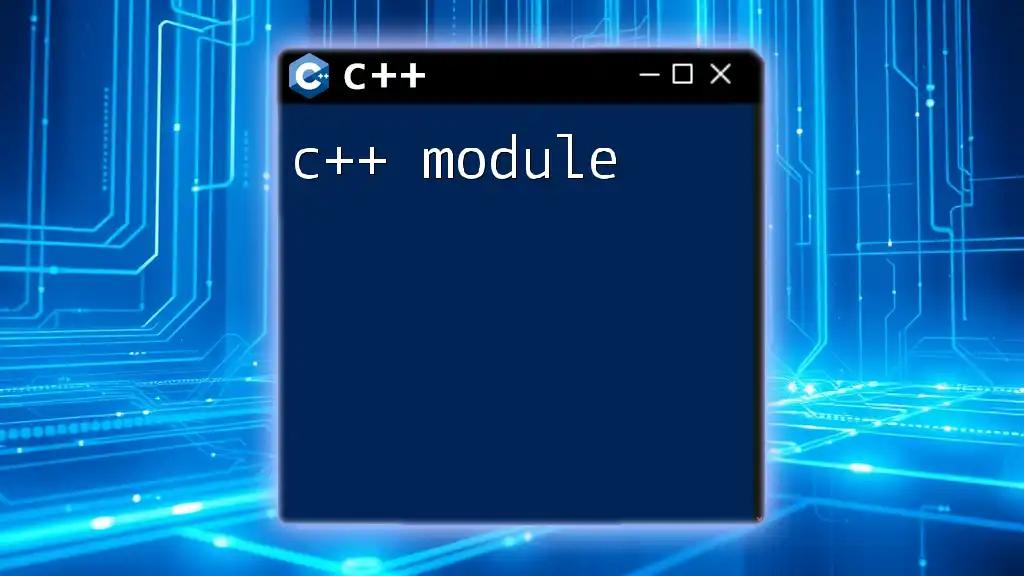
Practical Applications of Maximum Double Value
Usage in Real-World Scenarios
Understanding the c++ max double is essential in many real-world scenarios. For instance:
- Scientific Applications: When conducting experiments or simulations that involve highly precise measurements, knowing the limits of double values ensures calculations remain valid.
- Financial Applications: In fields like banking or finance, calculations involving large sums often require the double type to handle high precision effectively.
Preventing Overflow Using Max Double
Overflow occurs when an operation results in a value exceeding the maximum representable limit of a data type. In the case of doubles, using `DBL_MAX` can prevent inconvenient and unpredictable outcomes.
Consider the following example where an overflow occurs:
#include <iostream>
#include <cfloat>
int main() {
double largeValue = DBL_MAX;
std::cout << "Current value: " << largeValue << std::endl;
// This will cause an overflow
double newValue = largeValue * 2.0;
std::cout << "Updated value: " << newValue << std::endl; // This may result in 'inf'
return 0;
}
In this code, attempting to double `DBL_MAX` leads to infinite value outputted as `inf`, clearly illustrating the danger of overflow.

Double Max Value c++: Common Mistakes and Best Practices
Mistakes When Dealing with Double Max Values
When working with doubles, developers often make common mistakes:
- Ignoring Boundaries: Failing to check the value range can lead to unexpected behaviors and inaccuracies.
- Using the Wrong Data Type: Mixing float and double types may result in precision loss due to float's lower precision capabilities.
Best Practices
To prevent errors and enhance code reliability:
- Always Check Boundaries: Before performing calculations, ensure that values do not exceed the limits defined by `DBL_MAX`.
- Use Constants from `<cfloat>`: Adopting predefined constants like `DBL_MAX` fosters better code readability and maintenance, making your code clearer to other developers.

Conclusion
In summary, understanding the c++ max double and its applications is essential for efficient programming. Utilizing concepts like `DBL_MAX` can significantly enhance the reliability of your code while preventing critical errors associated with floating-point arithmetic. Take the knowledge gained here and apply it to your projects to ensure accuracy and dependability in your calculations.
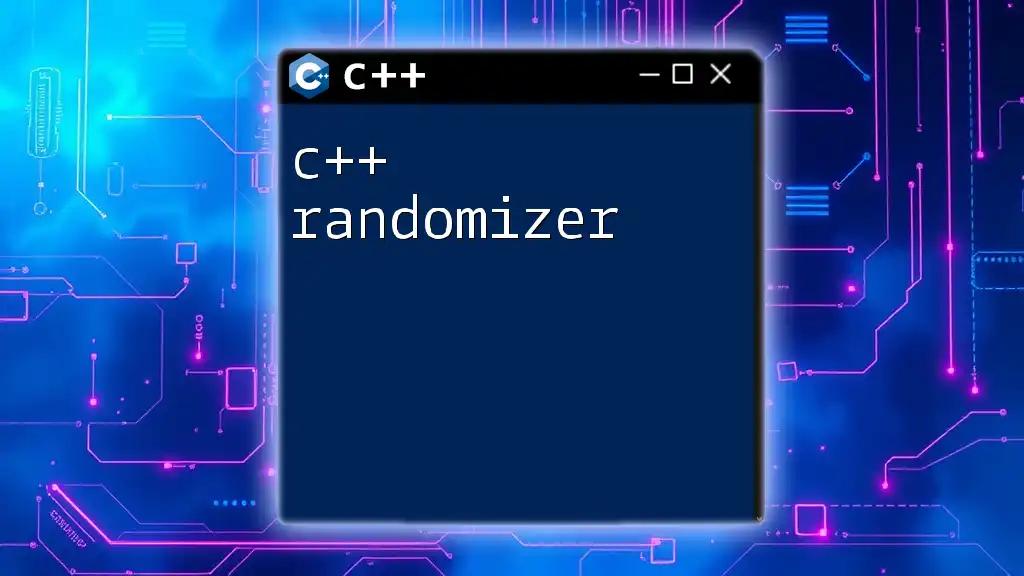
Frequently Asked Questions (FAQs)
What is the max value of double in C++?
The maximum value of a double in C++ is represented by the constant `DBL_MAX`, which is approximately 1.7976931348623157 × 10^308.
How can I check if a double exceeds its max value?
You can implement a simple function to check if a double exceeds its maximum permissible value:
#include <iostream>
#include <cfloat>
bool exceedsMaxValue(double value) {
return value > DBL_MAX;
}
int main() {
double value = 1e308;
std::cout << (exceedsMaxValue(value) ? "Exceeds max double value" : "Within range") << std::endl;
return 0;
}
Can double values be negative?
Yes, double values can indeed be negative, ranging from -DBL_MAX to DBL_MAX.
How should I handle double comparisons?
When comparing doubles, be aware of potential precision issues. A good practice is to use a threshold for comparison, allowing you to account for numerical inaccuracies rather than relying on direct equality checks. This can prevent misleading outcomes during calculations.