C++ machine learning involves leveraging the C++ programming language to implement algorithms and frameworks that facilitate the development of machine learning models efficiently and with high performance.
Here’s a simple example demonstrating the implementation of a linear regression algorithm in C++:
#include <iostream>
#include <vector>
class LinearRegression {
public:
void fit(const std::vector<double>& x, const std::vector<double>& y) {
// Simplified implementation of fitting a linear regression model
double x_mean = 0, y_mean = 0, numerator = 0, denominator = 0;
size_t n = x.size();
for (size_t i = 0; i < n; ++i) {
x_mean += x[i];
y_mean += y[i];
}
x_mean /= n;
y_mean /= n;
for (size_t i = 0; i < n; ++i) {
numerator += (x[i] - x_mean) * (y[i] - y_mean);
denominator += (x[i] - x_mean) * (x[i] - x_mean);
}
slope = numerator / denominator;
intercept = y_mean - slope * x_mean;
}
double predict(double x) {
return slope * x + intercept;
}
private:
double slope = 0;
double intercept = 0;
};
int main() {
LinearRegression lr;
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {2, 3, 5, 7, 11};
lr.fit(x, y);
std::cout << "Predicted value for x=6: " << lr.predict(6) << std::endl;
return 0;
}
What is C++?
C++ is a widely-used programming language that combines both high-level and low-level language features, allowing for robust system-level programming while also supporting object-oriented and generic programming paradigms. It is known for its performance and versatility, making it a popular choice in various domains, particularly where resource management and efficiency are critical.
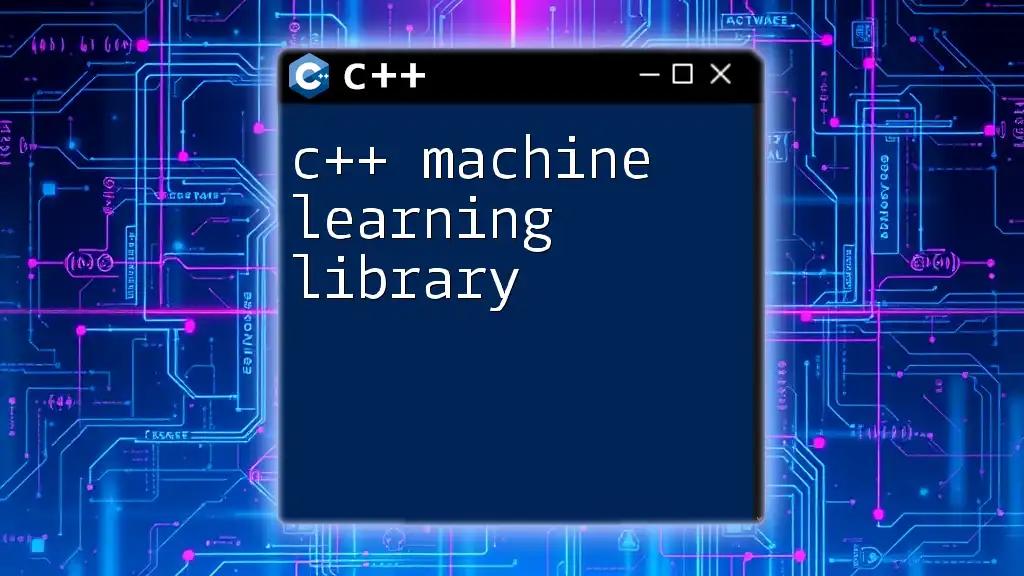
Understanding Machine Learning
Machine learning (ML) refers to the field of study that enables computers to learn from data and make predictions or decisions without being explicitly programmed. There are three primary types of machine learning:
-
Supervised Learning: Involves training a model on labeled data, where the outcome is known.
-
Unsupervised Learning: Uses input data without labeled responses to find patterns or groupings.
-
Reinforcement Learning: Focuses on making decisions sequentially, learning to achieve a goal through trial and error.
Machine learning is increasingly vital in today's technology landscape, driving innovations in diverse applications ranging from medical diagnostics to natural language processing.
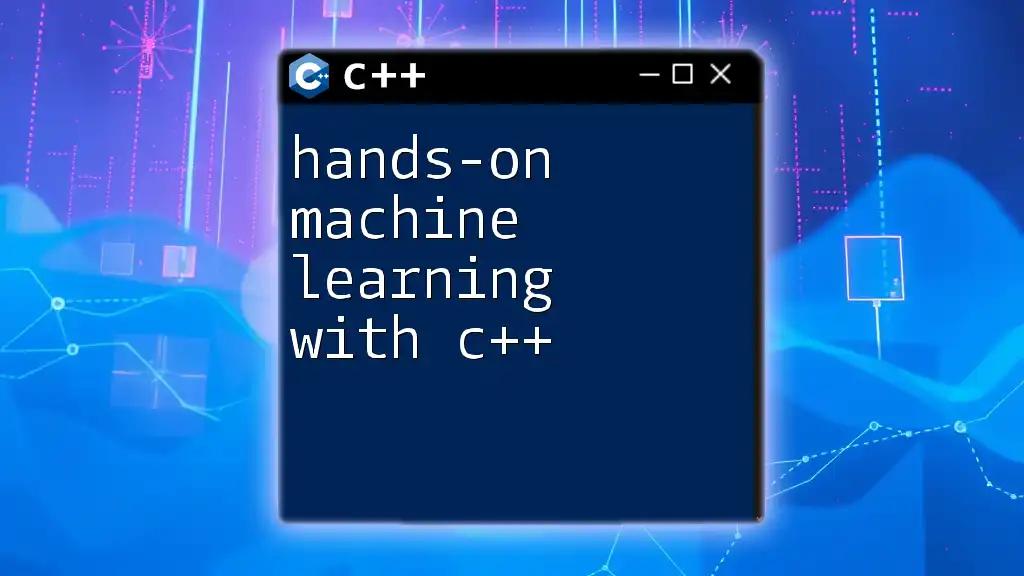
Why C++ for Machine Learning?
C++ offers several advantages for machine learning applications. Its performance and efficiency allow for the processing of vast datasets quickly, making it ideal for tasks that require low latency and high throughput. C++ also grants developers fine control over system resources, which is particularly beneficial when optimizing memory and CPU usage. Additionally, the existence of powerful C++ libraries for machine learning simplifies development and enhances productivity.
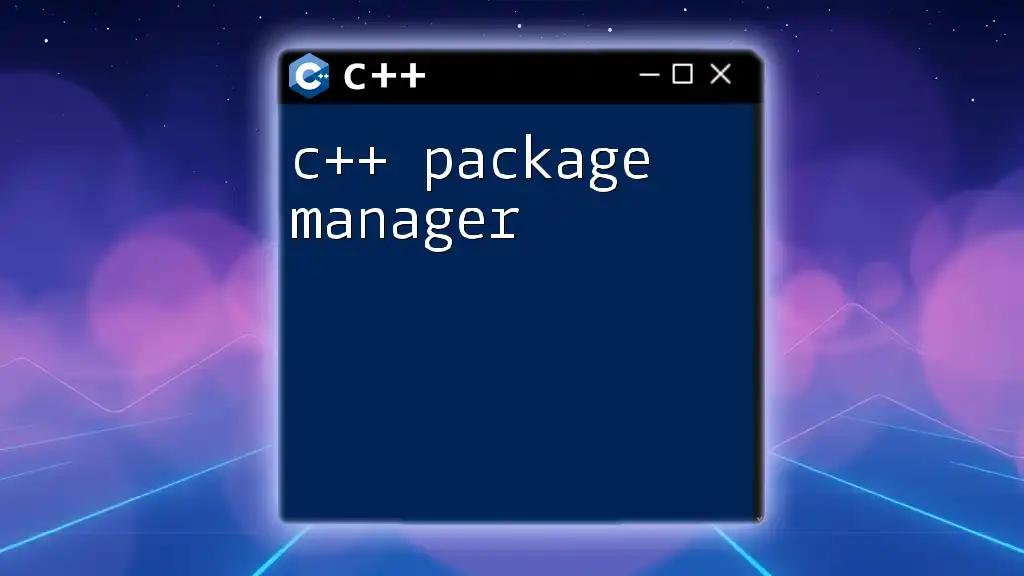
Setting Up Your C++ Environment for Machine Learning
Installing a C++ Compiler
To start developing in C++, you will need a reliable compiler. Popular options include:
-
GCC: The GNU Compiler Collection is widely supported across different platforms.
-
Clang: Known for its fast compilation times and excellent diagnostics.
-
MSVC: The Microsoft Visual C++ compiler is ideal for Windows users.
Follow the respective guides for installation based on your operating system to get your development environment up and running.
Choosing an Integrated Development Environment (IDE)
The right Integrated Development Environment can streamline your development process significantly. Recommended IDEs include:
-
Visual Studio: Feature-rich with support for debugging and version control.
-
Code::Blocks: An open-source IDE that is lightweight and easy to configure.
-
CLion: JetBrains' powerful cross-platform IDE known for its productivity features.
Once you've installed an IDE, set it up for machine learning projects using the libraries and compiler you've chosen.
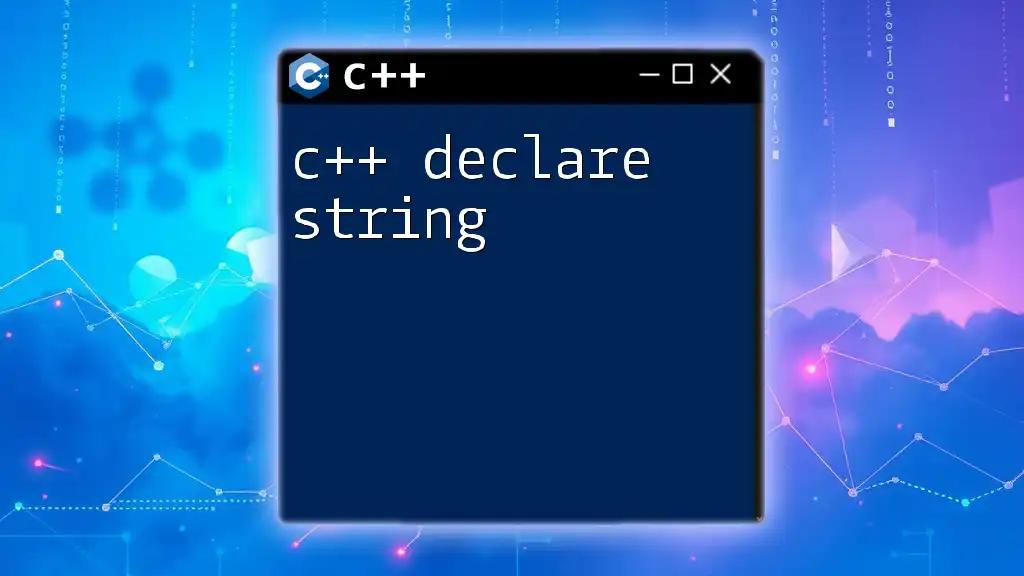
Key Libraries for Machine Learning in C++
Introduction to Machine Learning Libraries
Utilizing existing libraries can profoundly accelerate your development process. They provide pre-built implementations of algorithms and data structures, allowing you to focus on solving problems rather than implementing complex math from scratch. Popular C++ libraries for machine learning include dlib, mlpack, and Shark.
dlib Library
dlib is a modern C++ toolkit containing machine learning algorithms and tools for creating complex software in C++.
To install dlib, follow the instructions on its [official website](http://dlib.net/).
Here’s a simple example of building a linear regression model:
#include <dlib/matrix.h>
using namespace dlib;
int main() {
matrix<double> x(3, 2);
matrix<double> y(3, 1);
x(0, 0) = 1; x(0, 1) = 1;
x(1, 0) = 1; x(1, 1) = 2;
x(2, 0) = 2; x(2, 1) = 2;
y(0, 0) = 1; y(1, 0) = 2; y(2, 0) = 2;
// Fit your model here...
}
mlpack Library
mlpack is a fast, flexible machine learning library that provides simple and efficient APIs.
To get started with mlpack, follow the installation guide on its [official website](https://www.mlpack.org/).
Here’s an example of implementing K-means clustering:
#include <mlpack/core.hpp>
#include <mlpack/methods/kmeans/kmeans.hpp>
int main() {
// Load dataset and initialize KMeans
mlpack::kmeans::KMeans<> kmeans;
// Your clustering code here...
}
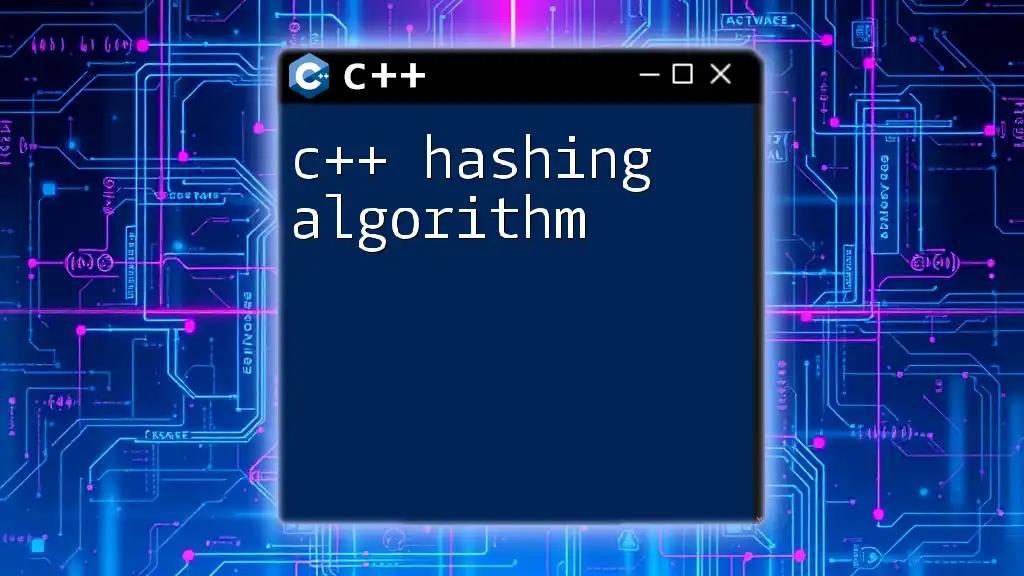
Implementing Machine Learning Algorithms in C++
Supervised Learning Algorithms
Linear Regression
Linear regression is a fundamental algorithm in supervised learning used to model the relationship between a dependent variable and one or more independent variables. The simplicity of linear regression makes it a go-to model for prediction tasks. Below is the implementation:
// Linear regression code implementation
Decision Trees
Decision trees partition data into subsets based on the value of input features, making decisions at each node. This structure allows for easy visualization and interpretability.
// Decision tree code implementation
Unsupervised Learning Algorithms
Clustering Algorithms
K-means clustering is one of the simplest and most commonly used clustering algorithms. It groups data points based on feature similarity into a predetermined number of clusters.
Pseudocode for K-means could look like this:
// K-means clustering pseudocode in C++
Principal Component Analysis (PCA)
PCA is used for dimensionality reduction by transforming the dataset to a new set of variables, which are orthogonal and capture the most variance. The mathematical foundation involves eigenvalue decomposition.
// PCA code implementation
Reinforcement Learning
Reinforcement learning is specialized for scenarios where an agent learns to make decisions by interacting with an environment. Q-learning is a popular algorithm where the agent learns to take actions that maximize cumulative reward.
// Q-learning pseudocode in C++
void qLearning() {
// State-action value initialization
// Update rules...
}
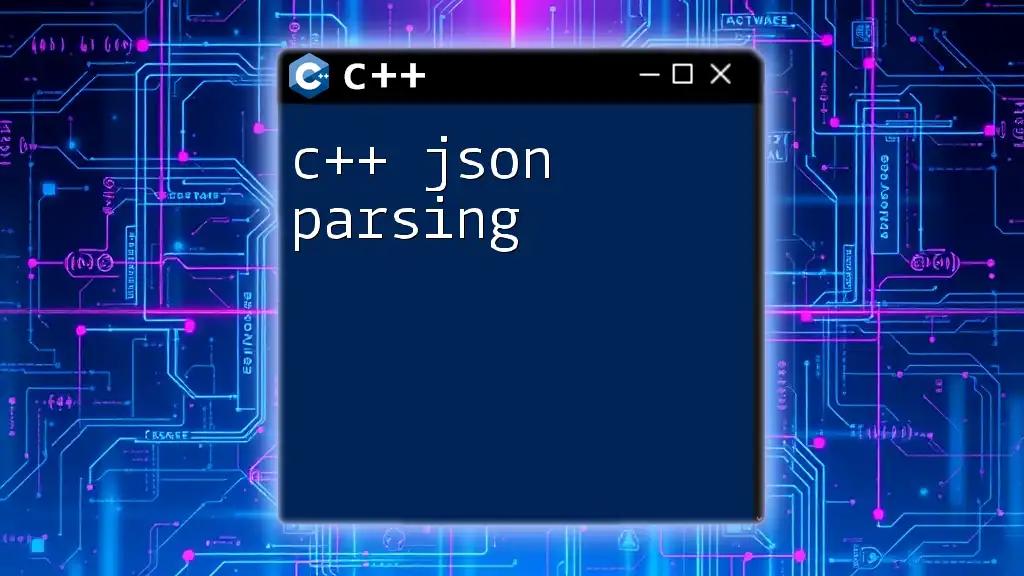
Advanced Topics in C++ and Machine Learning
Parallel Processing with C++
C++ allows for leveraging parallel processing capabilities, which can drastically speed up machine learning algorithms. Libraries like OpenMP facilitate multi-threading.
Here’s an example of how to use OpenMP for parallel execution:
#include <omp.h>
int main() {
#pragma omp parallel
{
// Parallel code here...
}
}
Optimizing Performance of Machine Learning Models
Optimal performance in machine learning applications involves multiple strategies, including efficient memory management, choosing the right data structures, and profiling application performance. Techniques such as caching and data preprocessing can also improve speed.
Integrating C++ with Python for Machine Learning
Combining C++ and Python opens up compelling opportunities to harness the best of both worlds. By using libraries such as Pybind11, you can expose C++ code to Python, facilitating the development of performance-critical components while still leveraging Python's simplicity for high-level orchestrations.
Below is a basic example of exposing a C++ function:
// Example of Pybind11 integration
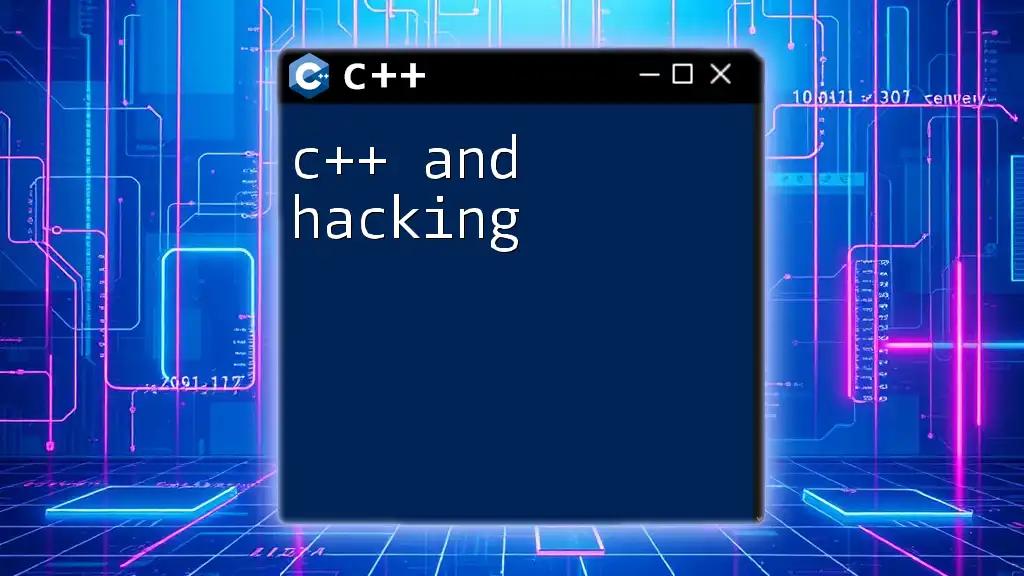
Case Studies and Real-world Applications
Machine learning paved the way for advancements in various industries. In finance, C++ models analyze extensive datasets to predict market trends. In healthcare, it aids in diagnostics by processing medical images rapidly.
Projects employing C++ in their machine learning implementations have demonstrated significant success, pushing the boundaries of what's possible in real-time analytics and autonomous systems.

Conclusion
As machine learning continues to evolve, the role of C++ remains crucial due to its performance characteristics and control over system resources. The future of C++ in machine learning looks promising, with a growing ecosystem that enables developers to explore and implement advanced algorithms more effectively.

Additional Resources
There is a wealth of information available regarding C++ and machine learning. Books, online courses, and community forums are excellent places to broaden your understanding and seek assistance in your journey. Engaging with the community and continuous learning will undoubtedly enhance your ability to leverage C++ for machine learning applications effectively.