A C++ machine learning library provides developers with tools and functions to create, train, and evaluate machine learning models efficiently using the C++ programming language.
Here's a simple code snippet demonstrating the usage of a hypothetical C++ machine learning library for linear regression:
#include <mlpack/core.hpp>
#include <mlpack/methods/linear_regression/linear_regression.hpp>
using namespace mlpack;
using namespace mlpack::regression;
int main() {
// Load dataset
arma::mat X; // Input features
arma::rowvec y; // Target variable
data::Load("data.csv", X, true); // Load data from CSV
data::Load("labels.csv", y, true); // Load labels
// Train the linear regression model
LinearRegression lr(X, y);
// Make a prediction
arma::rowvec prediction;
lr.Predict(X, prediction);
return 0;
}
This code snippet loads data, trains a linear regression model, and makes predictions with it using the mlpack library, a popular C++ machine learning library.
Understanding Machine Learning and Its Importance in C++
What is Machine Learning?
Machine learning (ML) is a subfield of artificial intelligence (AI) that focuses on the development of algorithms that allow computers to learn from and make predictions or decisions based on data. The concept emerged in the 1950s but has gained substantial traction due to advancements in computational power and the vast amounts of data generated by modern technology.
Machine learning is crucial as it powers numerous applications, such as natural language processing, image recognition, recommendation systems, and autonomous vehicles, ultimately shaping how we interact with technology daily.
The Role of C++ in Machine Learning
C++ has established itself as a powerful language in the development of machine learning applications. It offers numerous advantages:
-
Performance: C++ is known for its high performance due to its efficient memory management and low-level system access, making it suitable for computationally intensive tasks in machine learning.
-
Control: Developers have more control over system resources and performance optimizations, often leading to faster execution times compared to higher-level languages like Python.
When contrasting C++ with other programming languages, it becomes evident that while Python is preferred for its simplicity and extensive libraries, C++ excels in contexts where performance and efficiency are paramount.
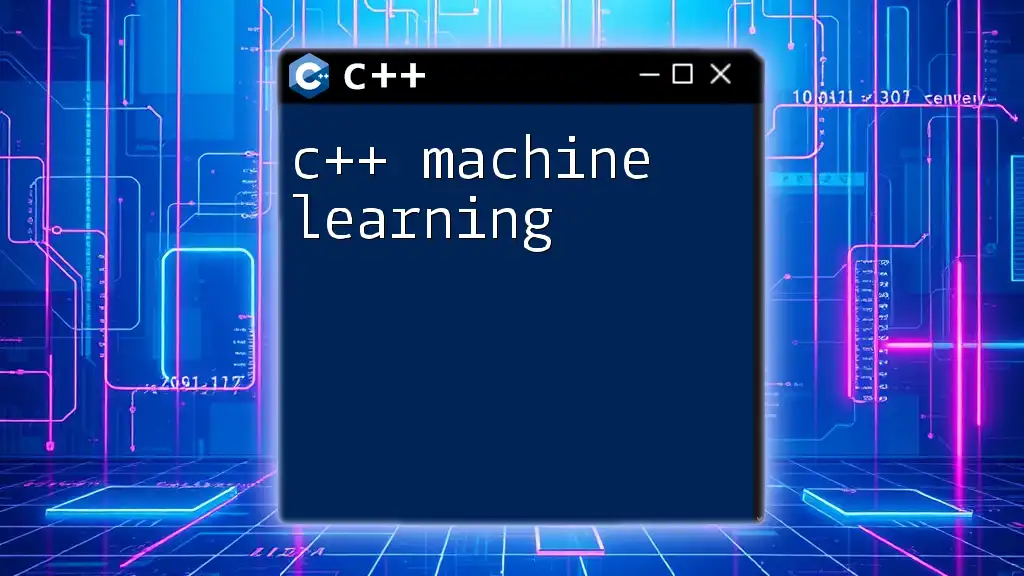
Overview of C++ Libraries for Machine Learning
What is a C++ Machine Learning Library?
A C++ machine learning library is a collection of precompiled code and functions specifically designed to facilitate machine learning development. These libraries provide ready-made algorithms, data structures, and utilities that accelerate the development process and reduce the time required to implement complex functionalities.
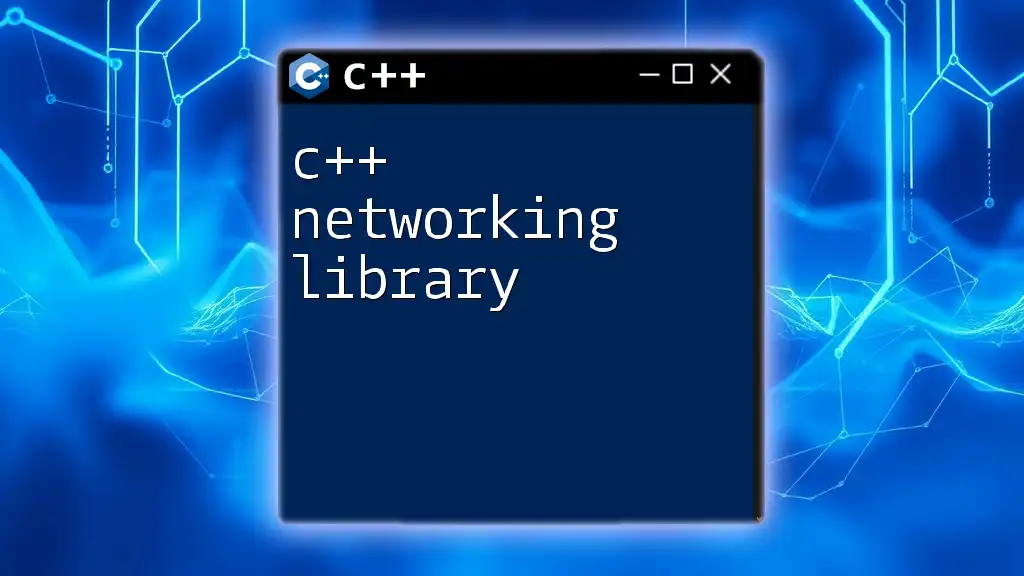
Popular C++ Libraries for Machine Learning
TensorFlow C++
TensorFlow is a renowned open-source machine learning library originally developed by Google. Its C++ API allows developers to leverage TensorFlow's powerful features in performance-sensitive applications.
To get started with TensorFlow C++, first ensure you have the necessary dependencies installed. Follow the official installation guide on the TensorFlow website. Here's a simple example of creating a linear regression model using TensorFlow C++:
#include "tensorflow/cc/client/client_session.h"
#include "tensorflow/cc/ops/standard_ops.h"
#include "tensorflow/core/framework/tensor.h"
using namespace tensorflow;
using namespace tensorflow::ops;
int main() {
// Define the inputs and outputs
Scope root = Scope::NewRootScope();
auto X = Placeholder(root.WithOpName("X"), DT_FLOAT);
auto Y = Placeholder(root.WithOpName("Y"), DT_FLOAT);
// Define the linear regression model (Y = W * X + b)
auto W = Variable(root.WithOpName("W"), {1}, DT_FLOAT);
auto b = Variable(root.WithOpName("b"), {}, DT_FLOAT);
auto Y_pred = Add(root.WithOpName("Y_pred"), MatMul(root, X, W), b);
// Define the loss and optimizer
auto loss = ReduceMean(root.WithOpName("loss"), Square(root, Subtract(root, Y_pred, Y)));
auto optimizer = ApplyGradientDescent(root, W, Const(root, 0.01), {loss});
// Initialize variables and run the session
ClientSession session(root);
session.Run({Assign(root, W, Const(root, {0.0})), Assign(root, b, Const(root, {0.0}))});
// Further training code would follow...
}
Dlib
Dlib is another powerful C++ library, known for its robustness in machine learning and image processing. It is particularly favored for its ease of use and comprehensive implementations of various machine learning algorithms.
To install Dlib, refer to its official documentation. Once installed, you can utilize it for tasks like face detection. Here's an example:
#include <dlib/image_processing.h>
#include <dlib/image_io.h>
int main() {
dlib::frontal_face_detector detector = dlib::get_frontal_face_detector();
dlib::array2d<dlib::rgb_pixel> img;
dlib::load_image(img, "face.jpg");
std::vector<dlib::rectangle> dets = detector(img);
std::cout << "Number of faces detected: " << dets.size() << std::endl;
}
Shark
The Shark library is recognized for its focus on optimization, machine learning, and statistical applications. It supports both supervised and unsupervised learning tasks.
To install Shark, download it from the official repository. Here's how you can create a supervised learning model using Shark:
#include <Shark/ObjectiveFunctions/Losses.h>
#include <Shark/Algorithms/Backpropagation.h>
int main() {
shark::Data<shark::RealVector> trainingData; // assume data is populated
// Create a neural network
shark::NeuralNetwork<shark::SigmoidActivationFunction> network;
// Train the model
shark::BackpropagationAlgorithm<> algo(network, trainingData);
algo.train();
}
mlpack
mlpack is designed for scalability and speed, making it a premium choice for implementing machine learning algorithms in C++. With a focus on performance, mlpack is especially adept at handling large datasets.
To get started with mlpack, follow its installation documentation. Below is an example of implementing the K-means clustering algorithm:
#include <mlpack/core.hpp>
#include <mlpack/methods/kmeans/kmeans.hpp>
int main() {
// Load dataset
arma::mat dataset;
mlpack::data::Load("data.csv", dataset);
// Define the number of clusters
const int clusters = 3;
arma::Mat<size_t> assignments;
arma::mat centroids;
// Run K-means
mlpack::kmeans::KMeans<> kmeans;
kmeans.Cluster(dataset, clusters, assignments, centroids);
}
OpenCV
OpenCV is a widely-used library that focuses on computer vision, which is an integral part of machine learning applications. Its robust set of features also extends to machine learning.
OpenCV can be installed through package managers or from source. Here's a simple application of image processing using OpenCV:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat img = cv::imread("image.jpg");
cv::Mat grayImg;
// Convert image to grayscale
cv::cvtColor(img, grayImg, cv::COLOR_BGR2GRAY);
// Display the image
cv::imshow("Gray Image", grayImg);
cv::waitKey(0);
}
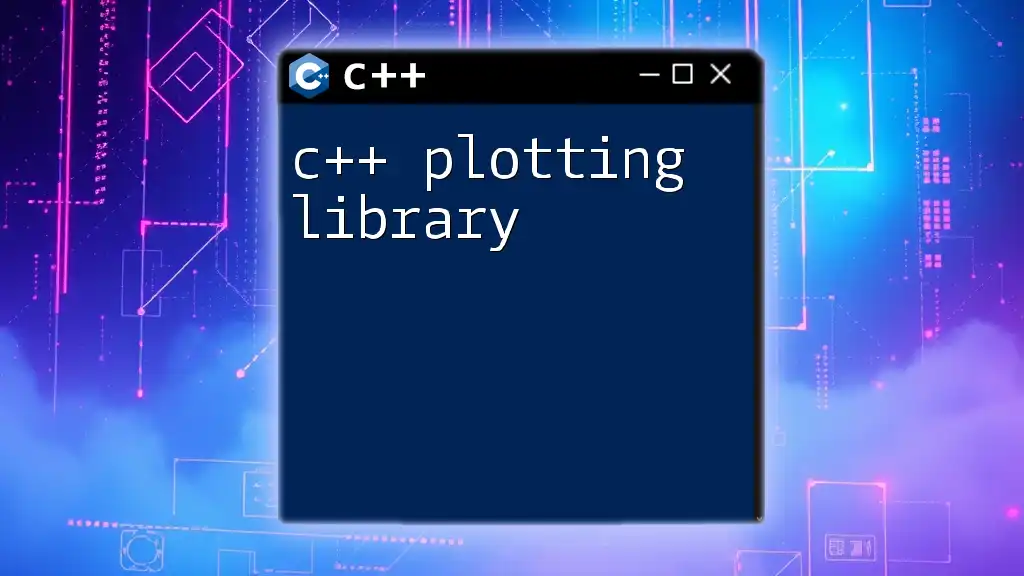
Choosing the Right C++ ML Library for Your Project
Factors to Consider
When selecting the best C++ ML library for your project, consider the following:
-
Project Size and Complexity: Determine if your project requires a light-weight library or something more comprehensive.
-
Community Support and Documentation: Look for libraries with active communities and extensive documentation for troubleshooting and learning.
-
Performance and Speed: Assess the performance benchmarks of the libraries with similar tasks you intend to implement.
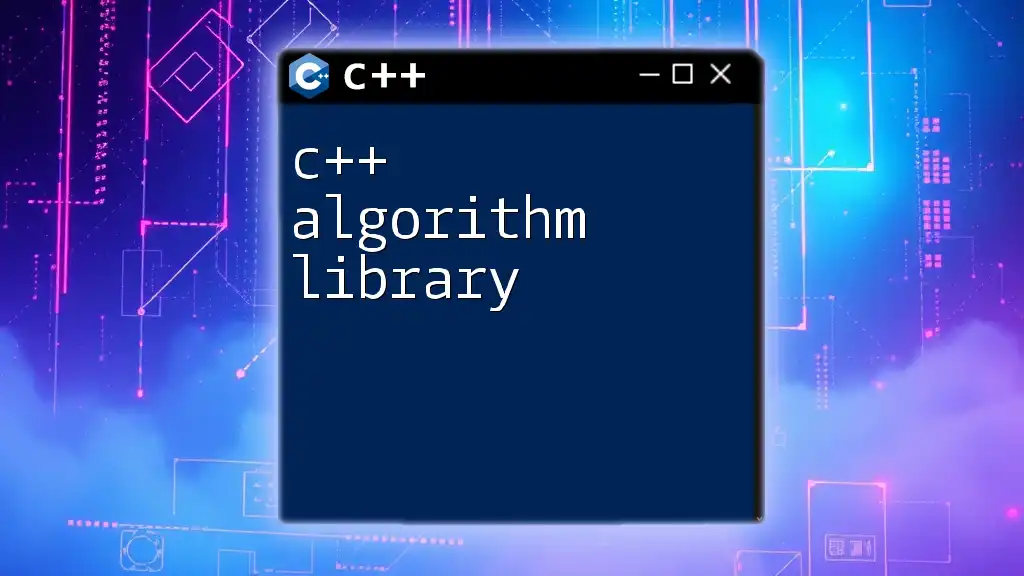
How to Get Started with C++ for Machine Learning
Setting Up Your Development Environment
For developing C++ machine learning applications, select an integrated development environment (IDE) that you are both comfortable with and that supports C++ development. Common choices include Visual Studio, Code::Blocks, and CLion. Ensure you have a C++ compiler installed, such as GCC or Clang.
Basic C++ Concepts for Machine Learning
Understanding fundamental C++ concepts is crucial for effective ML development. Memory management, templates, and the Standard Template Library (STL) are particularly beneficial. Mastery of these topics will enable you to create efficient, reusable, and scalable ML algorithms.
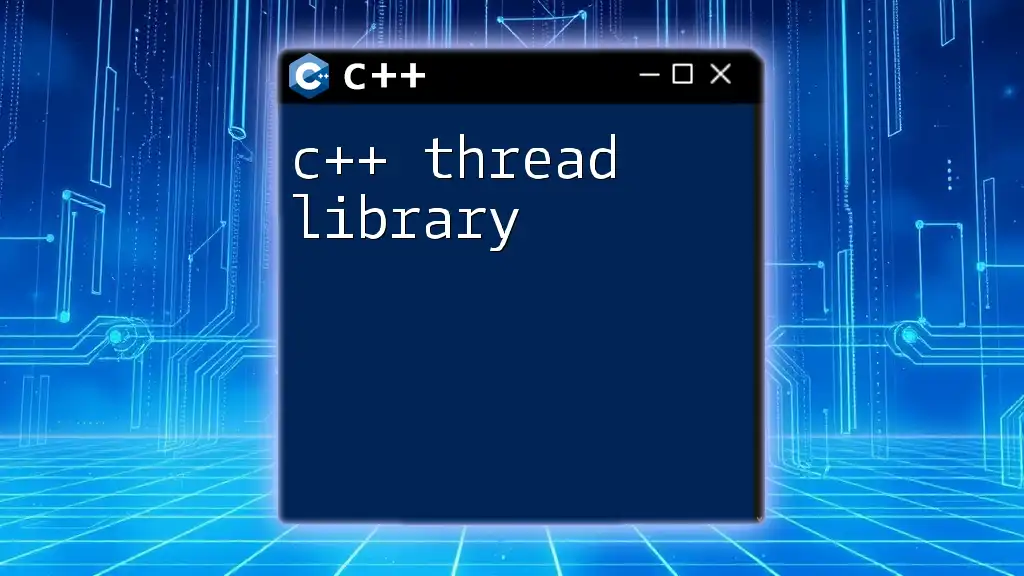
Building Your First Machine Learning Application in C++
Step-by-Step Guide to Creating a Simple ML Model
Start by selecting a well-known dataset, such as the Iris dataset or MNIST, frequently used in testing ML algorithms. First, load and preprocess the dataset to prepare it for training. Ensure that you handle input formatting and normalization.
An example training implementation can look like this:
#include <mlpack/core.hpp>
#include <mlpack/methods/logistic_regression/logistic_regression.hpp>
int main() {
// Load the Iris dataset
arma::mat dataset;
arma::Row<size_t> labels;
mlpack::data::Load("iris.csv", dataset, true);
// Split dataset into training and testing
auto train = dataset.submat(0, 0, dataset.n_rows - 1, 100);
auto test = dataset.submat(0, 100, dataset.n_rows - 1, dataset.n_cols - 1);
// Fit a logistic regression model
mlpack::regress::LogisticRegression<> lr(train, labels);
// Evaluate the model
arma::Row<size_t> predictions;
lr.Classify(test, predictions);
}
Debugging and Optimization Tips
Debugging in C++ can be more challenging than in higher-level languages. Pay special attention to pointer management, memory leaks, and manage your resources effectively. Utilizing tools like Valgrind can help identify leaks.
For optimization, consider employing proper profiling techniques to identify bottlenecks and leverage data structures wisely for better performance and faster algorithms.
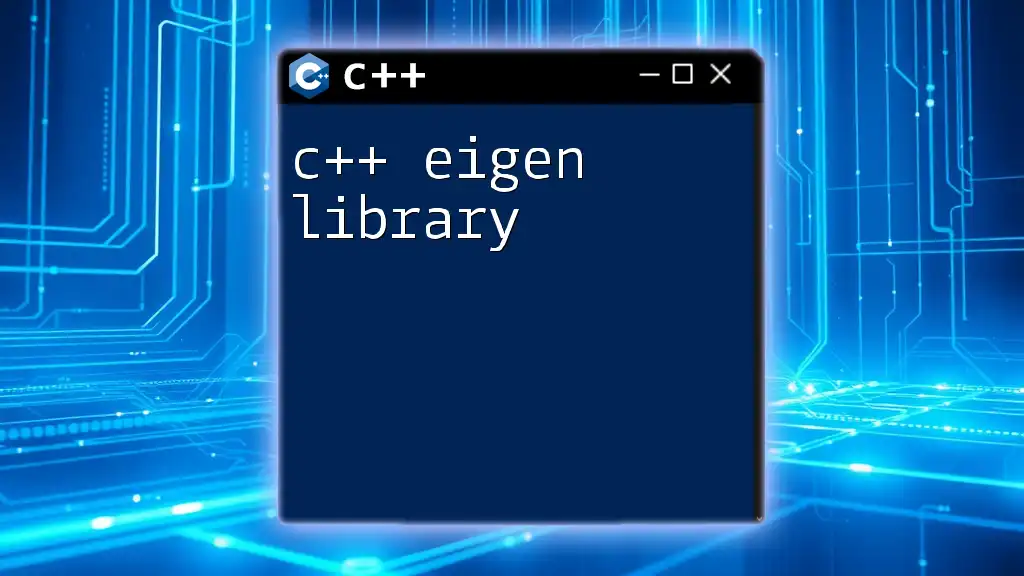
Conclusion
Recap of Key Points
In this guide, we covered essential aspects of C++ machine learning libraries, highlighting powerful options like TensorFlow C++, Dlib, Shark, mlpack, and OpenCV. Understanding the role of C++ in the machine learning domain will enable developers to choose the right tools for their projects and leverage them effectively.
Encouragement to Experiment
Machine learning is a continually evolving field, and C++ remains a vital tool for efficient computation. I encourage you to experiment with various libraries, build your projects, and share your learning experiences with the community!
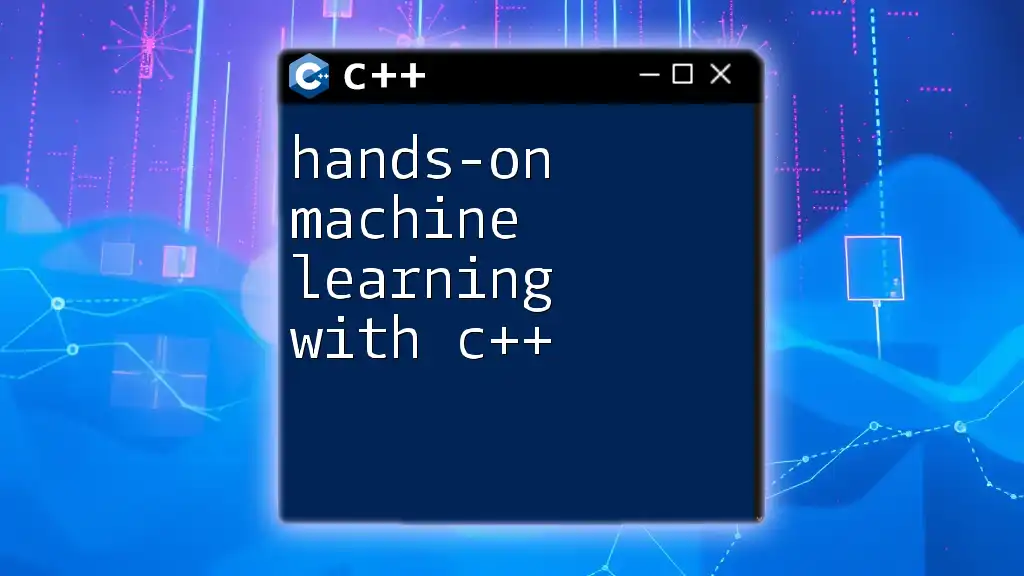
Additional Resources
Recommended Books and Online Courses
To further enhance your machine learning expertise in C++, explore a selection of recommended books and online courses tailored to expand your knowledge base and skills.
Community Forums and Platforms
Engage with other C++ machine learning enthusiasts on platforms like GitHub, Stack Overflow, and relevant forums to share ideas, troubleshoot issues, and keep up with the latest developments in the field.