C++ is a numeric language that provides various data types and operators for performing arithmetic and logical calculations efficiently.
Here’s a simple code snippet demonstrating basic numeric operations in C++:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 3;
cout << "Addition: " << (a + b) << endl; // Output: Addition: 8
cout << "Subtraction: " << (a - b) << endl; // Output: Subtraction: 2
cout << "Multiplication: " << (a * b) << endl; // Output: Multiplication: 15
cout << "Division: " << (a / b) << endl; // Output: Division: 1
return 0;
}
Understanding Numeric Types in C++
What are Numeric Types?
In C++, numeric types represent data that can be expressed as numbers, allowing for mathematical calculations and operations. Numeric types can be broadly classified into two categories: integral types, which represent whole numbers, and floating-point types, which represent real numbers.
Categories of Numeric Types
Integral Types
Integral types are used to represent whole numbers without any decimal component. They include:
- `int`: The most commonly used type for integer values.
- `short`: Typically used for smaller integer values, requiring less memory.
- `long`: Used for larger integer values.
- `char`: Represents a single character, but it is stored as an integer.
Here's an example demonstrating the declaration and initialization of these types:
#include <iostream>
using namespace std;
int main() {
int age = 25; // Standard integer
short temp = -10; // Short integer
long population = 7000000000; // Long integer
char grade = 'A'; // Char representing a character
cout << "Age: " << age << endl;
cout << "Temperature: " << temp << endl;
cout << "Population: " << population << endl;
cout << "Grade: " << grade << endl;
return 0;
}
Floating-Point Types
Floating-point types are designed to represent numbers that require a decimal point. They include:
- `float`: A single precision floating-point type, suitable for most real number representations.
- `double`: A double precision floating-point type, which provides better precision for calculations.
- `long double`: An extended precision floating-point type, for cases where even more precision is needed.
The following code illustrates the initialization and usage of floating-point types:
#include <iostream>
#include <iomanip> // For setting precision
using namespace std;
int main() {
float pi = 3.14f; // Single precision
double e = 2.718281828459; // Double precision
long double goldenRatio = 1.618033988749895; // Extended precision
cout << fixed << setprecision(10); // Setting precision for output
cout << "Pi: " << pi << endl;
cout << "Euler's Number: " << e << endl;
cout << "Golden Ratio: " << goldenRatio << endl;
return 0;
}
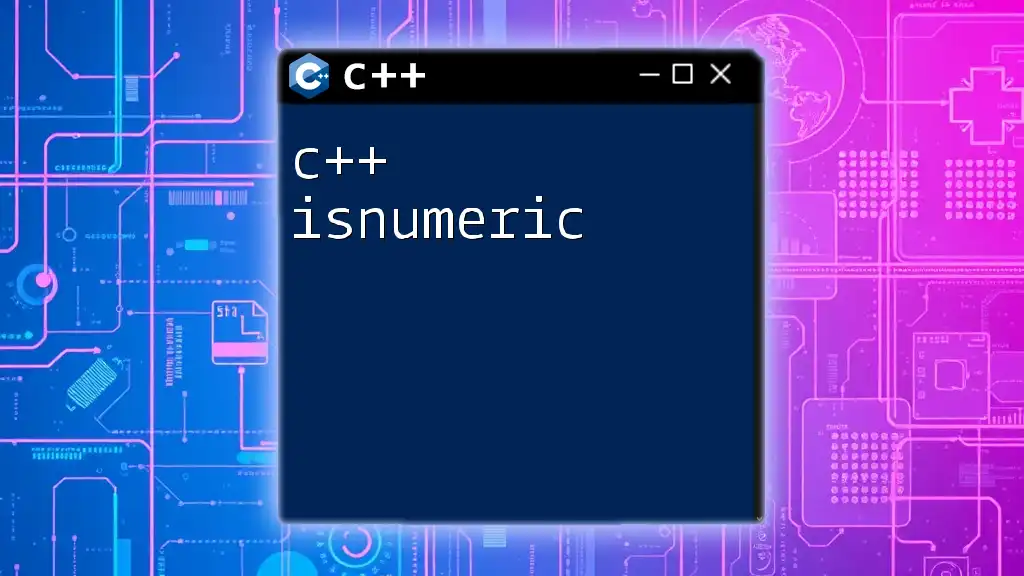
Working with Numeric Data in C++
Declaring Numeric Variables
In C++, declaring a variable of numeric type involves specifying the type followed by the variable name. Initialization can occur at the time of declaration or later in the code.
int a; // Declaration
a = 10; // Assignment
double weight = 65.5; // Declaration with initialization
Arithmetic Operations
C++ provides several fundamental arithmetic operators that can be used with numeric types:
- Addition (`+`)
- Subtraction (`-`)
- Multiplication (`*`)
- Division (`/`)
- Modulus (`%`)
Here's an example demonstrating these operations:
#include <iostream>
using namespace std;
int main() {
int x = 8, y = 3;
cout << "Addition: " << (x + y) << endl;
cout << "Subtraction: " << (x - y) << endl;
cout << "Multiplication: " << (x * y) << endl;
cout << "Division: " << (x / y) << endl; // Integer division
cout << "Modulus: " << (x % y) << endl;
return 0;
}
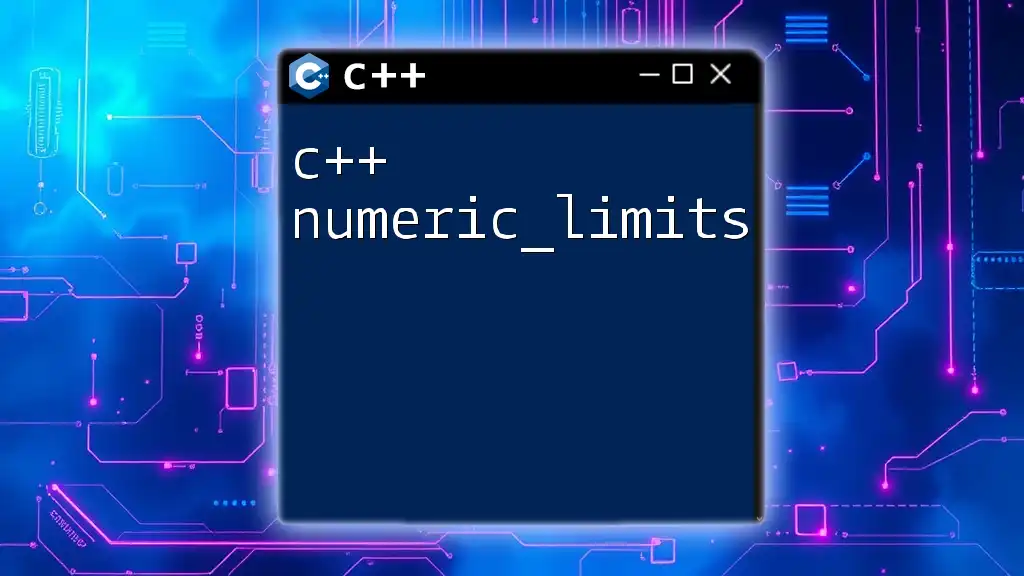
Numeric Constants and Literals
What are Numeric Constants?
Numeric constants are fixed values assigned to variables in a program. They can be categorized into integer constants, floating-point constants, and character constants. For example, `42` is an integer constant, while `3.14` is a floating-point constant.
Using Numeric Constants in Code
Defining constants can be crucial for maintaining code clarity. In C++, constants can be defined using `const` and `constexpr` declarations:
#include <iostream>
using namespace std;
const int daysInWeek = 7; // Constant variable
constexpr double pi = 3.14159; // Constexpr for compile-time evaluation
int main() {
cout << "Days in a week: " << daysInWeek << endl;
cout << "Pi: " << pi << endl;
return 0;
}
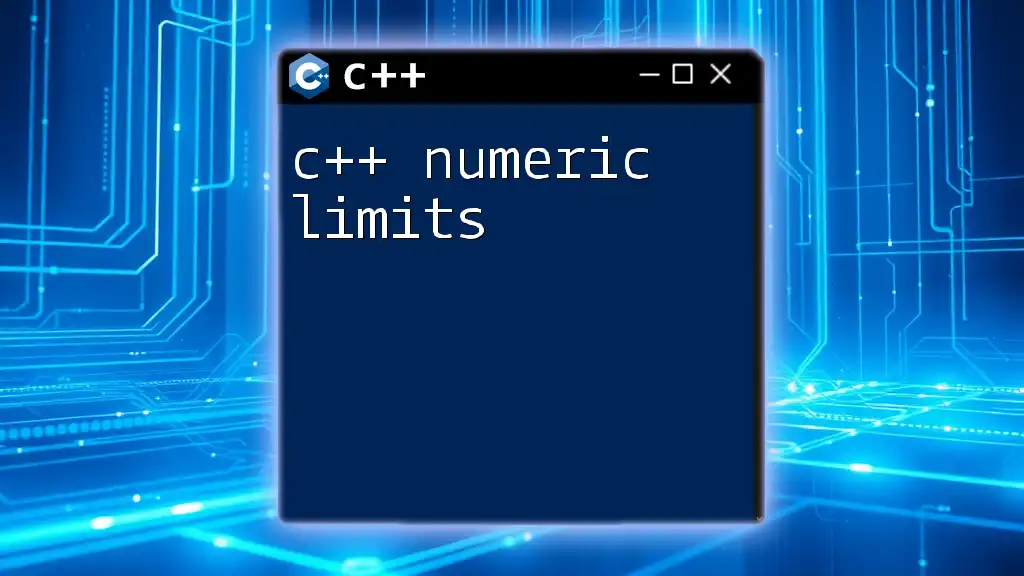
Type Casting in C++
Importance of Type Casting
Type casting allows one numeric type to be converted into another. This is particularly important for avoiding errors like loss of precision when performing arithmetic operations with mixed types.
Static and Dynamic Type Casting
In C++, you can use several casting techniques. Two of the most common are `static_cast` and `dynamic_cast`.
- `static_cast`: Used for standard conversions, such as between numeric types.
- `dynamic_cast`: Usually employed in inheritance hierarchies but also applicable for type safety.
Example of `static_cast`:
#include <iostream>
using namespace std;
int main() {
double price = 9.99;
int intPrice = static_cast<int>(price); // Converts double to int
cout << "Price as double: " << price << endl;
cout << "Price as int: " << intPrice << endl;
return 0;
}
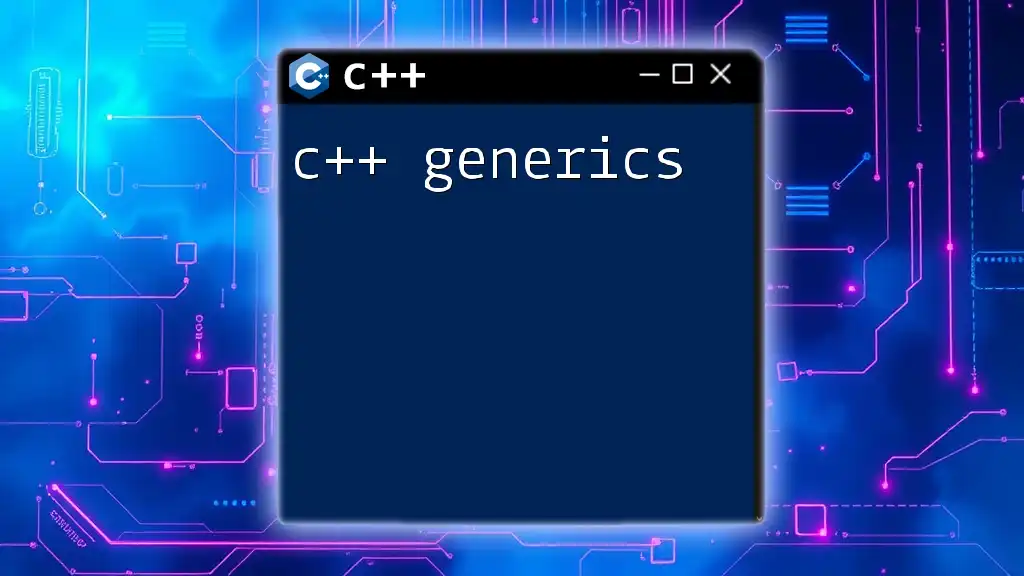
Common C++ Commands for Numeric Types
Input and Output
C++ uses the standard input/output streams `cin` and `cout` for reading and writing numeric data. Here's how you can read and print numeric values:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number; // Reading input
cout << "You entered: " << number << endl; // Printing output
return 0;
}
Math Functions from `<cmath>` Library
The `<cmath>` library provides several useful mathematical functions for numeric calculations such as `sqrt()`, `pow()`, and `abs()`.
#include <iostream>
#include <cmath> // Include cmath for mathematical functions
using namespace std;
int main() {
double value = -5.0;
cout << "Square root of 16: " << sqrt(16) << endl;
cout << "2 raised to the power of 3: " << pow(2, 3) << endl;
cout << "Absolute value of " << value << ": " << abs(value) << endl;
return 0;
}
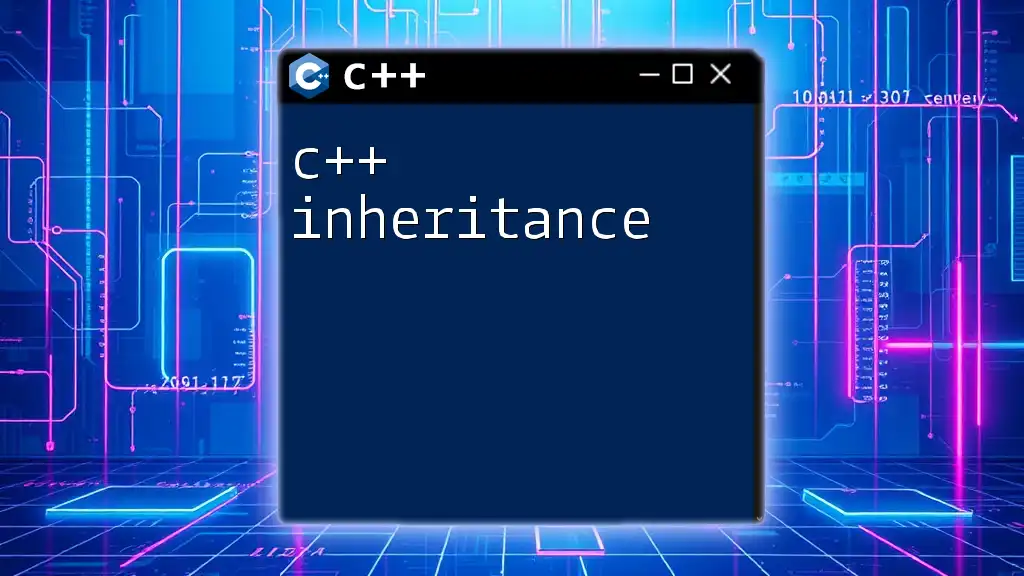
Best Practices for Working with Numeric Types
Choosing the Right Numeric Type
Selecting the most suitable numeric type is essential for optimizing both memory usage and performance. For instance, prefer `int` over `long` unless you specifically need the extended range. Likewise, use `float` when single precision suffices but switch to `double` for higher precision in calculations.
Avoiding Common Mistakes
When working with numeric types, be mindful of common pitfalls such as integer division or overflow. For example, be cautious when dividing two integers, as it truncates the decimal part:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 3;
cout << "Integer division: " << (a / b) << endl; // Results in 3, not 3.333...
return 0;
}
To avoid this, you can explicitly cast one of the operands to `float` to achieve floating-point division:
cout << "Floating-point division: " << (static_cast<float>(a) / b) << endl; // Results in 3.333...
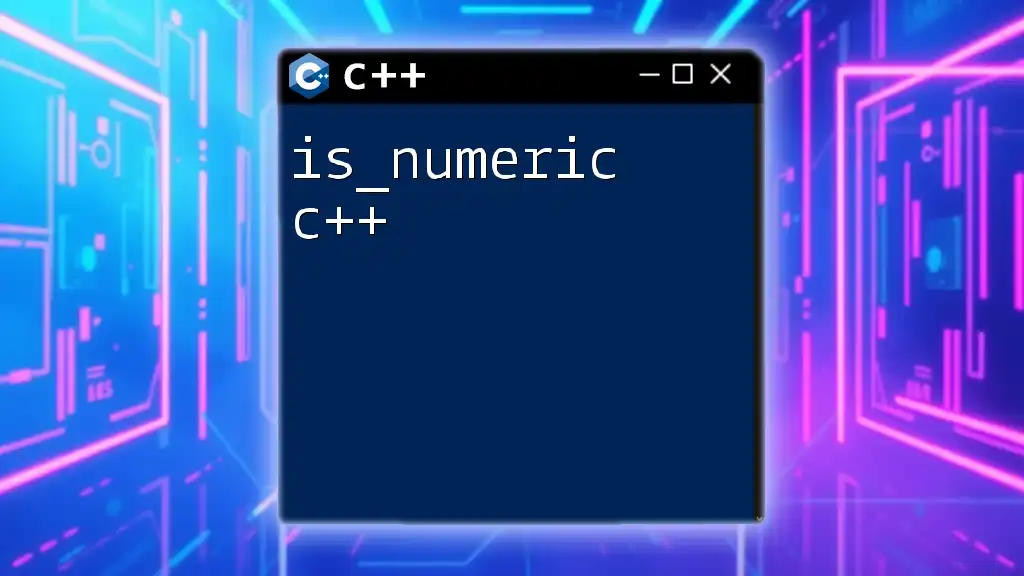
Conclusion
Understanding how C++ handles numeric types is fundamental to effective programming and performing arithmetic operations. By mastering numeric types, casting, and various C++ commands, you can elevate your programming skills and create more effective and efficient code. Embrace the learning process, experiment with different numeric types, and enhance your coding efficiency!
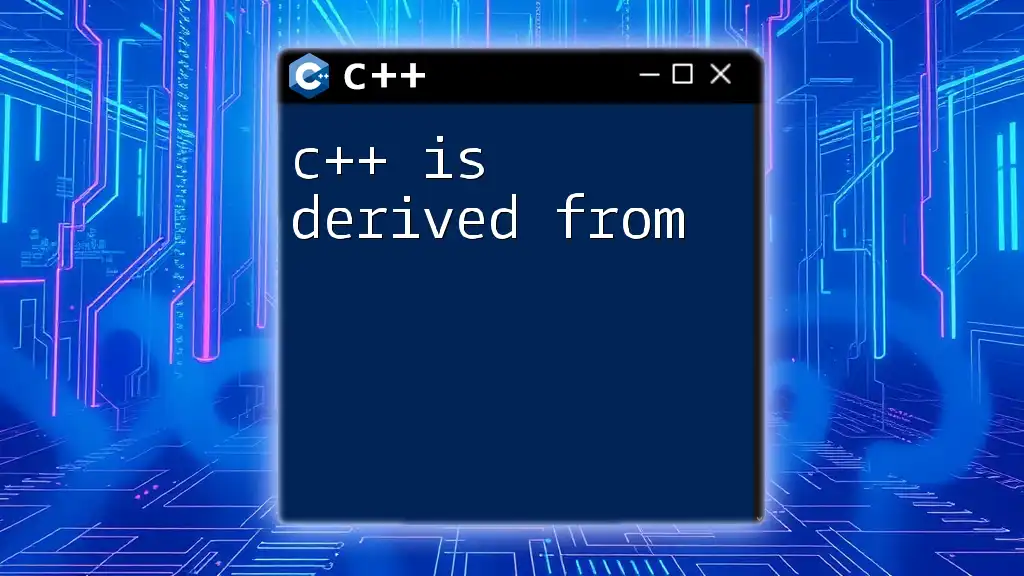
Additional Resources
For further exploration of numeric types in C++, consider reviewing the official documentation, as well as recommended programming books and online courses focused on C++ programming. Happy coding!