The `stod` function in C++ is used to convert a string to a `double`, which is particularly useful for parsing floating-point values from text data.
Here’s an example code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double num = std::stod(str);
std::cout << "The converted number is: " << num << std::endl;
return 0;
}
What is `std::stod`?
`std::stod` is a powerful function in C++ that belongs to the Standard Library, specifically designed to convert a string representation of a numeric value into a `double`. This function proves to be essential in scenarios involving data parsing and type conversion, helping developers manage user inputs, configuration files, or any data that comes as strings.
Importance in Data Conversion and Parsing
Data conversion is a common requirement when processing user inputs or data from external sources. When dealing with numeric data represented as strings, such as those read from a file or console input, using `std::stod` ensures that these values can be used in mathematical operations or stored as `double` for precision.
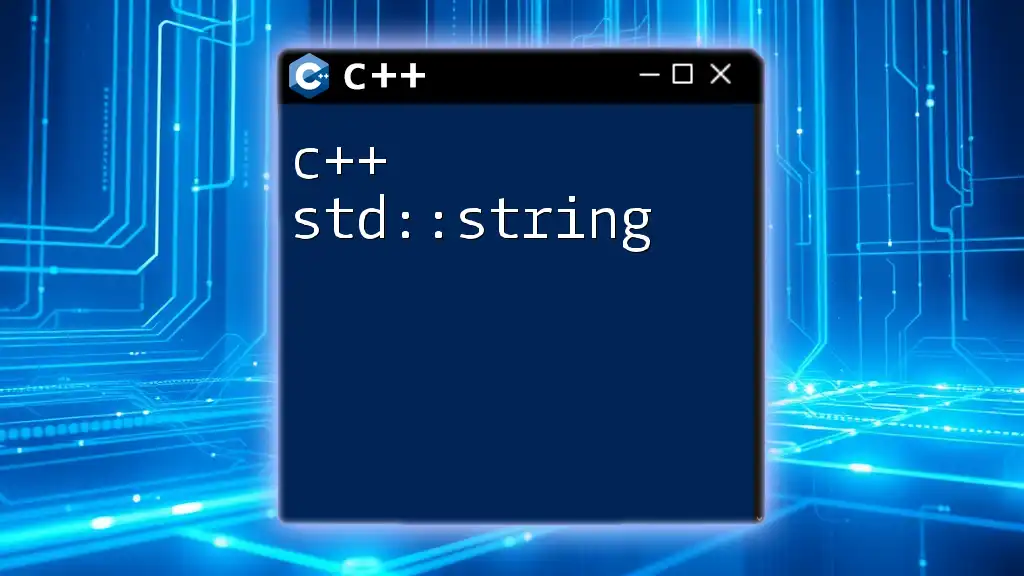
Syntax of `std::stod`
General Format
The syntax for `std::stod` is straightforward. It is defined as follows:
double stod(const std::string& str, std::size_t* pos = nullptr);
Parameters Explained
- str: This parameter is the string that you want to convert into a double. It should ideally contain a numeric value in standard format (e.g., "3.14", "0.0", "1.5e2").
- pos: This is an optional parameter that points to a `std::size_t` variable. If provided, it will store the position of the next character in the string that follows the number. This is useful for parsing multiple numeric values from a single string.
Return Value
`std::stod` returns the converted double value. If the input string is not a valid numeric representation, the function throws an exception. It's crucial for developers to handle these exceptions to prevent crashes or unexpected behavior in their applications.
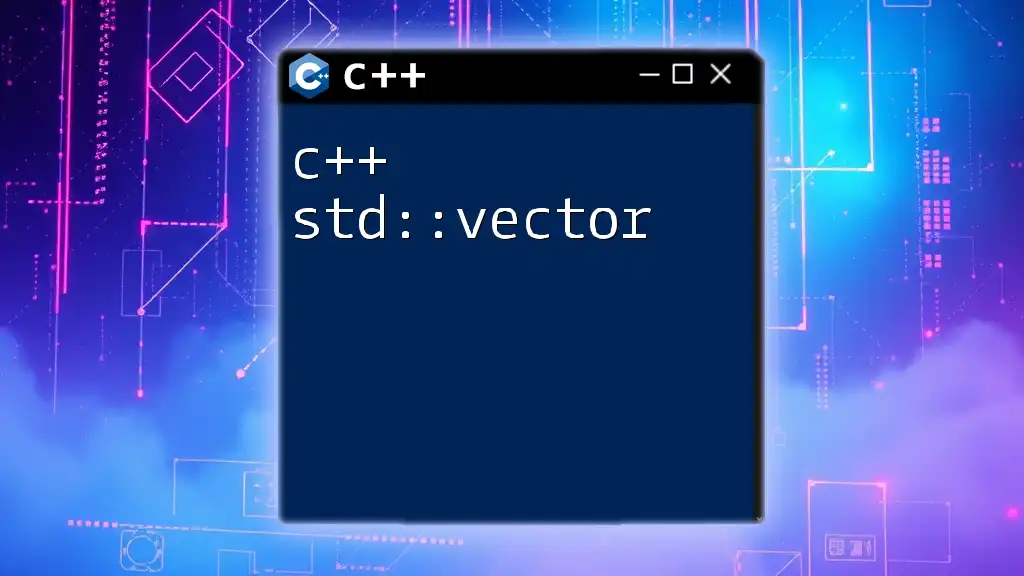
How to Use `std::stod`
Basic Example of `std::stod`
To illustrate the basic usage of `std::stod`, consider a simple program that converts a numeric string:
#include <iostream>
#include <string>
int main() {
std::string numStr = "3.14";
double num = std::stod(numStr);
std::cout << "The double value is: " << num << std::endl;
return 0;
}
In this example, the string "3.14" is successfully converted to a double, and the result is printed to the console.
Handling Invalid Input
Using Try-Catch Blocks
When converting strings that may not contain valid numeric values, Exception handling becomes vital. `std::stod` can throw two types of exceptions: `std::invalid_argument` if the input cannot be interpreted as a number, and `std::out_of_range` if the converted value falls outside the representable range of a double. Here’s how to handle these exceptions:
#include <iostream>
#include <string>
#include <stdexcept>
int main() {
try {
std::string invalidStr = "abc";
double result = std::stod(invalidStr);
} catch (std::invalid_argument& e) {
std::cout << "Invalid argument: " << e.what() << std::endl;
} catch (std::out_of_range& e) {
std::cout << "Out of range: " << e.what() << std::endl;
}
return 0;
}
In this code snippet, if the conversion fails due to an invalid string, the error message is printed instead of causing a program crash.
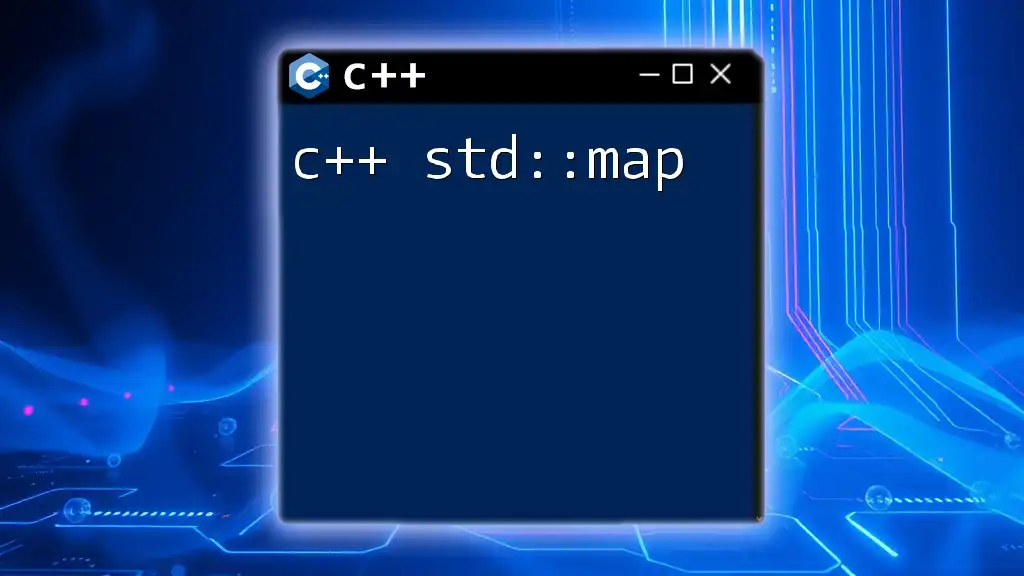
Advanced Usage of `std::stod`
Converting with Position Tracking
The `pos` parameter can be especially useful when parsing strings that contain multiple parts. It allows you to find out where the numeric conversion stopped. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string input = "123.45abc";
std::size_t pos;
double value = std::stod(input, &pos);
std::cout << "Converted value: " << value << ", next position: " << pos << std::endl;
return 0;
}
In this example, the `std::stod` function converts "123.45" to a double and stops at the character 'a', which is not part of a valid number. The position where the conversion stopped is stored in `pos`.
Working with Different Number Formats
`std::stod` also supports various numeric formats, including scientific notation. Here's an example that demonstrates this capability:
#include <iostream>
#include <string>
int main() {
std::string sciStr = "1.23e2"; // This represents 123 in scientific notation.
double sciValue = std::stod(sciStr);
std::cout << "Converted scientific value: " << sciValue << std::endl;
return 0;
}
In this snippet, the scientific notation "1.23e2" is effectively converted to the numeric value of 123.
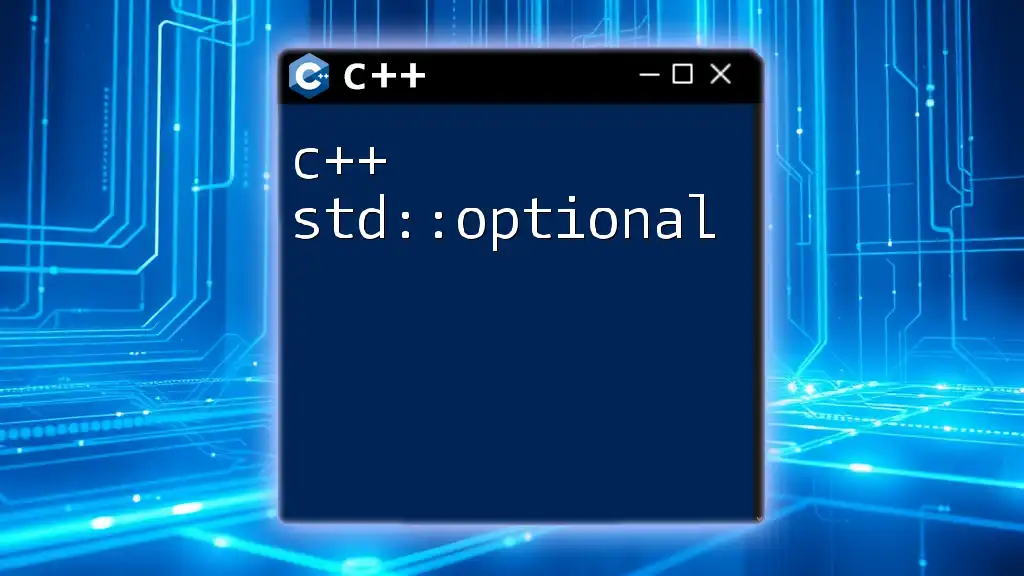
Common Pitfalls and Best Practices
Common Mistakes
One of the most frequent errors developers encounter is passing non-numeric strings to `std::stod`. This mistake can lead to exceptions that disrupt the application flow. Always ensure the string is properly validated before attempting conversion.
Best Practices
To write robust C++ programs, adhere to these best practices when using `std::stod`:
- Always Validate Input: Before conversion, check if the string truly represents a valid numeric value. This mitigates the risk of unexpected exceptions.
- Handle Exceptions: Always use try-catch blocks around your conversion code to manage potential errors gracefully. This adds robustness and improves user experience by providing meaningful feedback.
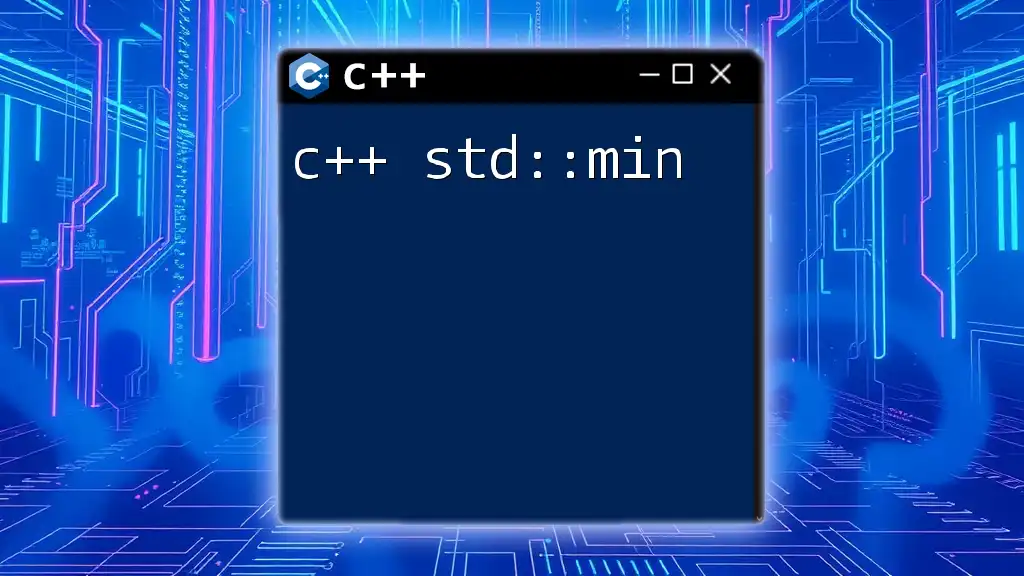
Performance Considerations
Performance is an important aspect of any function's utility. The `std::stod` function is generally efficient for converting strings to doubles, especially in scenarios where the input is well-formed. However, when considering performance-critical applications, compare `std::stod` with other conversion functions like `std::stof` (for float values) to determine the best choice for your specific use case.
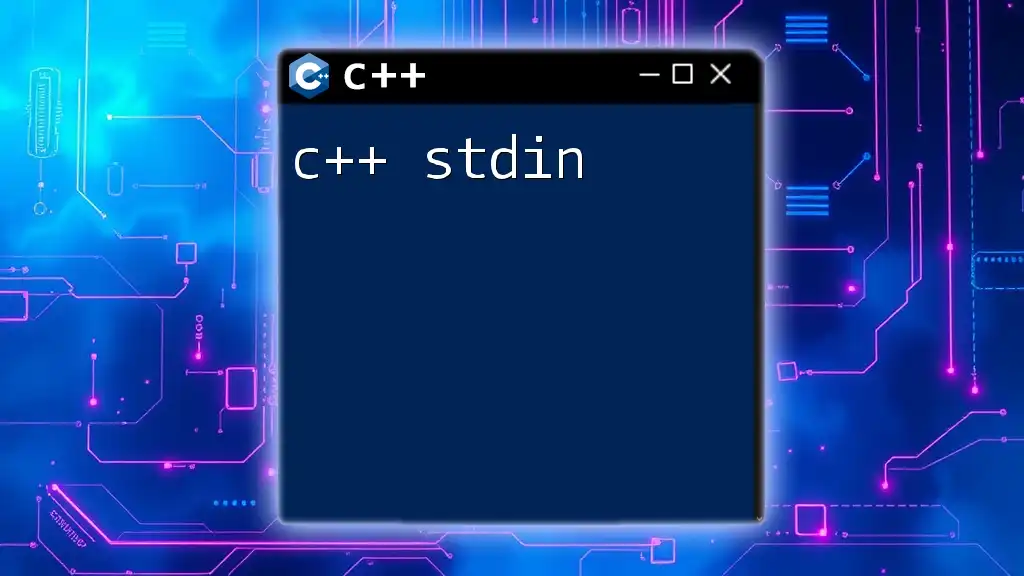
Conclusion
In summary, `std::stod` provides a vital service in C++ programming for converting strings into double precision floating-point numbers. Its functionality for error handling and position tracking enhances its utility when parsing and converting data. By mastering the use of `std::stod`, developers can confidently handle numerical data in string format while ensuring robust applications.
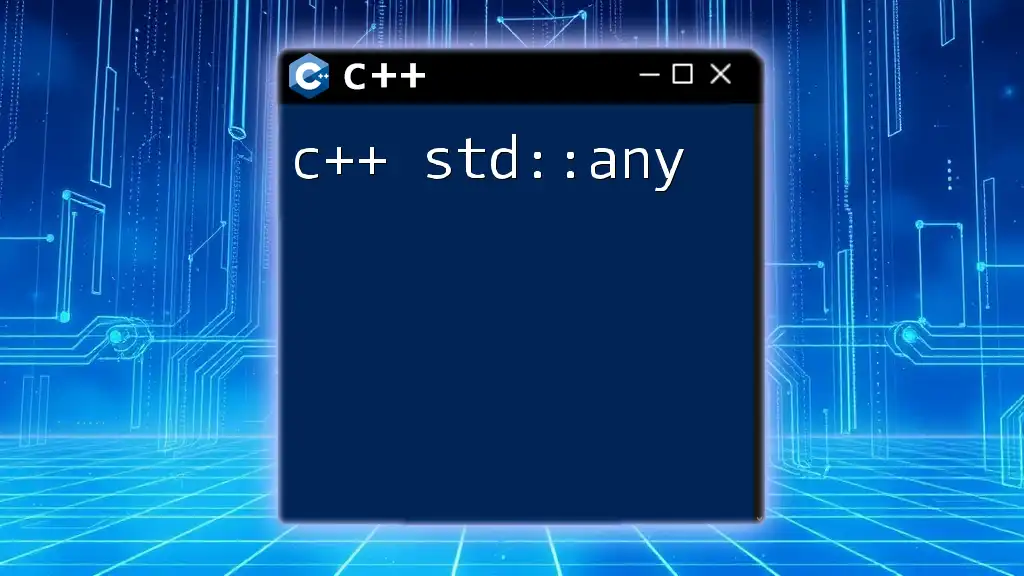
Call to Action
I encourage you to try writing your functions using `std::stod` and experiment with various input scenarios. It’s an excellent way to solidify understanding and proficiency in data conversion within C++. For further learning, don’t hesitate to explore additional resources on C++ programming.