The `std::any` type in C++ is a type-safe container for storing single values of any type, allowing you to hold and retrieve values without knowing their types at compile time.
#include <iostream>
#include <any>
int main() {
std::any value = 10; // Storing an integer
std::cout << std::any_cast<int>(value) << std::endl; // Retrieving the integer
return 0;
}
What is std::any?
std::any is a powerful and flexible type introduced in C++17 that allows you to store an instance of any type, effectively enabling type-erasure. This feature is crucial for applications that require dynamic type management, where the types of variables may not be known until runtime.
When compared to other types, such as std::variant (which restricts you to a fixed set of types) and std::optional (which can only hold a value or be empty), std::any's versatility shines. It allows the storage of any data type, making it especially useful in situations that require a high degree of flexibility.
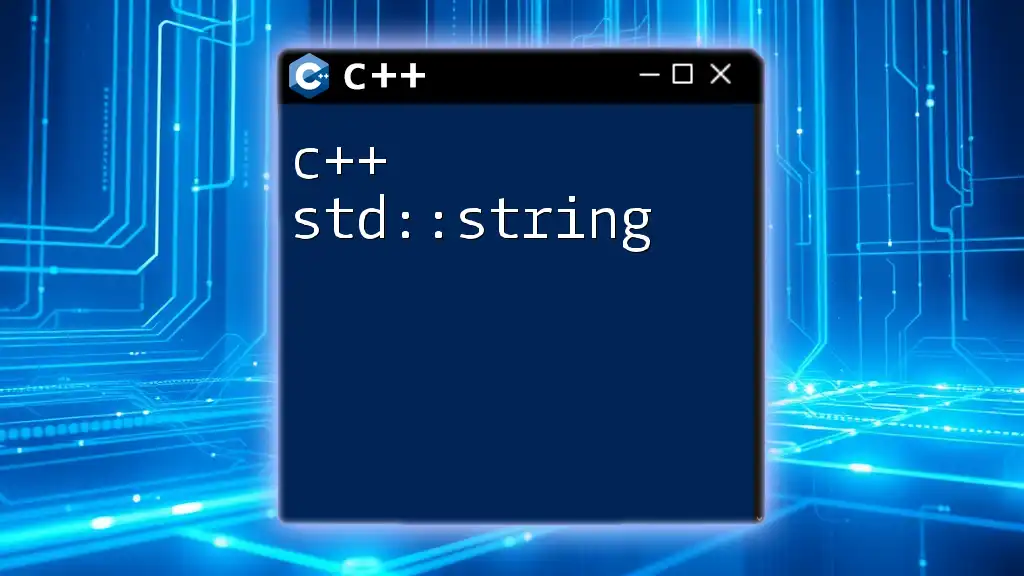
Why use std::any in C++?
The ability to hold different types is advantageous in many scenarios, including but not limited to:
- Dynamic Function Parameters: Functions can accept arguments of different types without the need to overload each function for specific types.
- Heterogeneous Collections: You can create collections (like vectors) that can hold any type in a single container.
How std::any Works
Internally, std::any leverages type-erasure to allow different types to be stored under a single type. It basically uses dynamic memory allocation to achieve this, which adds performance considerations that need to be accounted for.
Key Properties of std::any
One significant property of std::any is its size and alignment. When you store a type in std::any, it captures and manages that type's size and alignment characteristics. However, be cautious—using it carelessly can lead to performance bottlenecks due to frequent allocations and deallocations of memory.
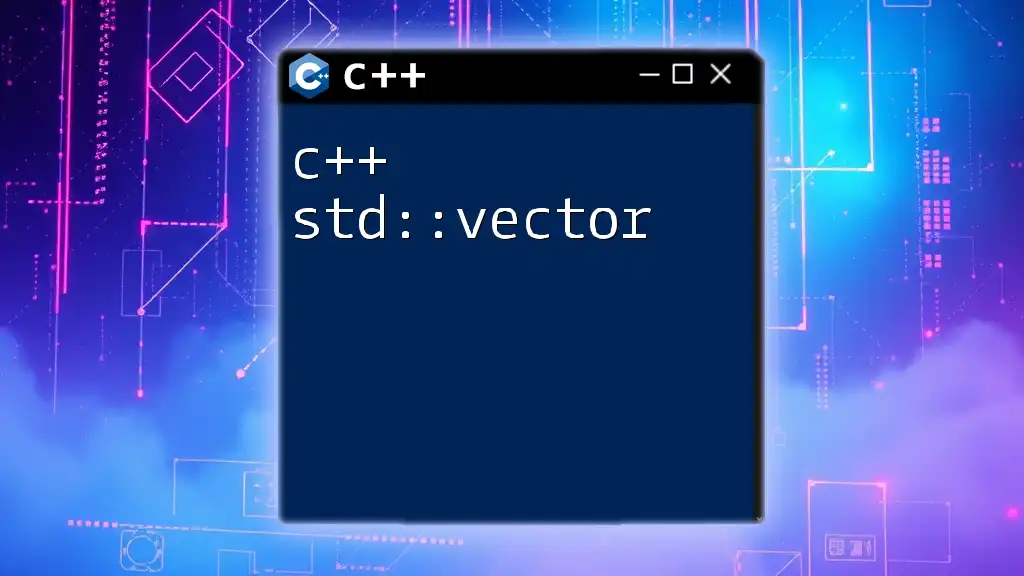
Using std::any: Basic Operations
Creating std::any Objects
Creating std::any instances is straightforward. You can initiate it by either default construction or assigning a value directly. For example:
std::any value = 42; // Storing an integer
You can also create it using its constructor:
std::any value1 = std::string("Hello");
std::any value2 = 3.14; // Storing a double
Assigning Values to std::any
One of the most beneficial features of std::any is its ability to hold different types over time. Assigning values is done quite simply, as shown here:
std::any value;
value = std::string("Hello, World!"); // Assign a string
value = 3.14; // Reassigning a different type
You can freely assign and reassign any type to std::any, though this may lead to memory management concerns.
Accessing Values in std::any
The most common way to retrieve values stored in std::any is through std::any_cast. It allows you to safely cast back to the original data type and retrieve the stored value.
try {
std::string str = std::any_cast<std::string>(value);
} catch (const std::bad_any_cast& e) {
// Handle casting error
}
If the type you are trying to cast to does not match the actual type contained within the std::any object, it throws a std::bad_any_cast exception.
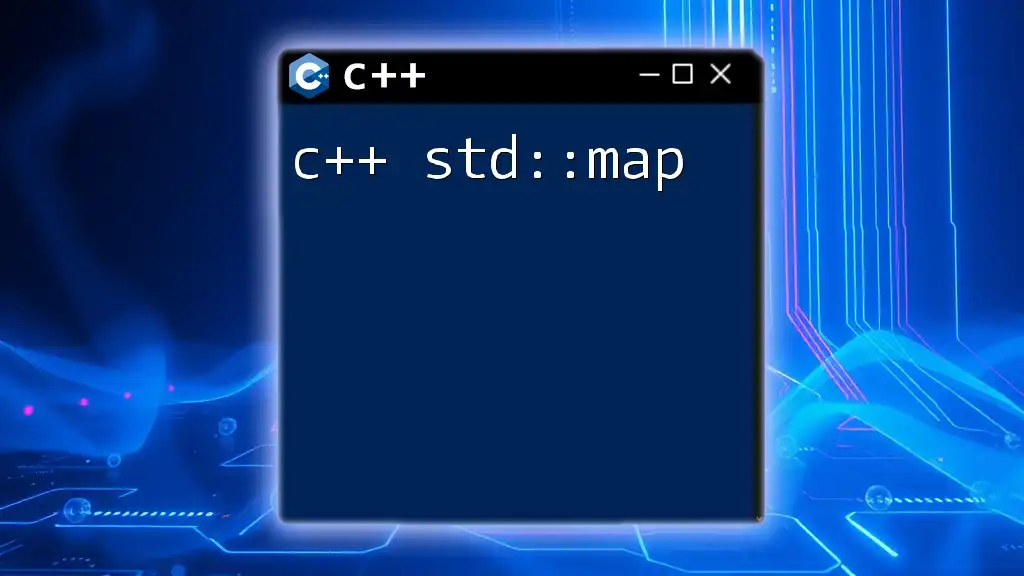
Advanced Features of std::any
Checking Types with std::any
std::any provides methods like std::any::type() and std::any::has_value() to help you manage your data more effectively. Checking if an std::any object contains a value is done using has_value():
if (value.has_value()) {
std::cout << "Value is present" << std::endl;
}
Swapping Values
Swapping values between two std::any objects can be accomplished easily using std::any::swap. This not only makes your code cleaner but also helps manage state without excessive copying:
std::any a = 10, b = 20;
a.swap(b); // Swap values between a and b
Resetting std::any
The reset() function allows you to clear the currently stored value inside an std::any object. This is useful for freeing up resources or preparing the object for new data:
value.reset(); // Cleans up the current stored value
This operation sets the std::any object back to its uninitialized state.
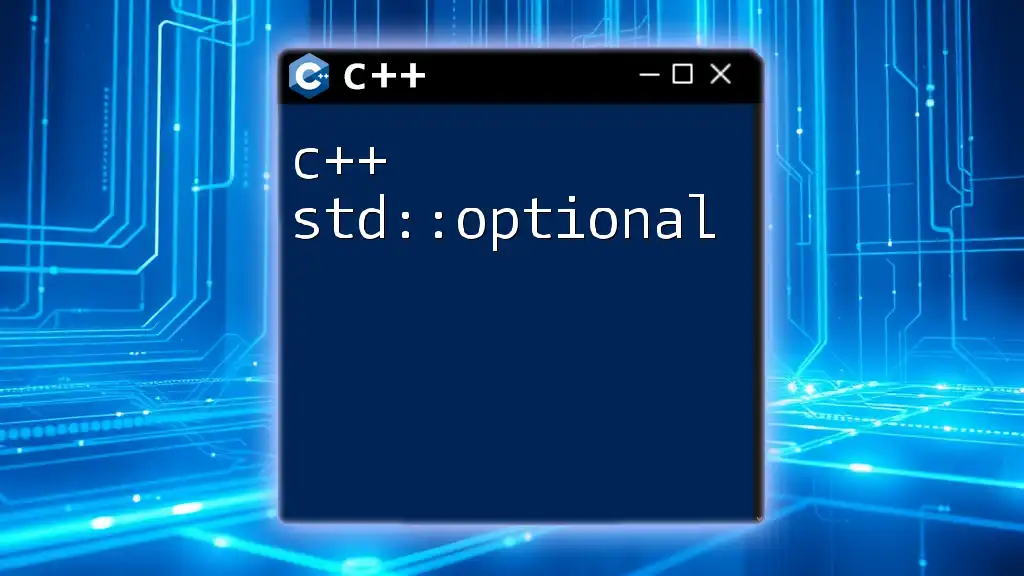
Practical Use Cases of std::any in C++
Dynamic Function Parameters
One common use case for std::any is accepting multiple argument types in a single function. This can help simplify function overloading and make your code cleaner. Below is a basic example of such a function:
void processValue(std::any value) {
if (value.type() == typeid(int)) {
int intValue = std::any_cast<int>(value);
std::cout << "Integer: " << intValue << std::endl;
} else if (value.type() == typeid(std::string)) {
std::string strValue = std::any_cast<std::string>(value);
std::cout << "String: " << strValue << std::endl;
}
}
Storing Heterogeneous Collections
If you need to create collections that can hold different types, std::any can be a perfect fit. For instance, consider a vector that can hold multiple data types:
std::vector<std::any> myVec = {42, std::string("Hello"), 3.14};
Such collections enable dynamic programming while maintaining type safety during retrieval.
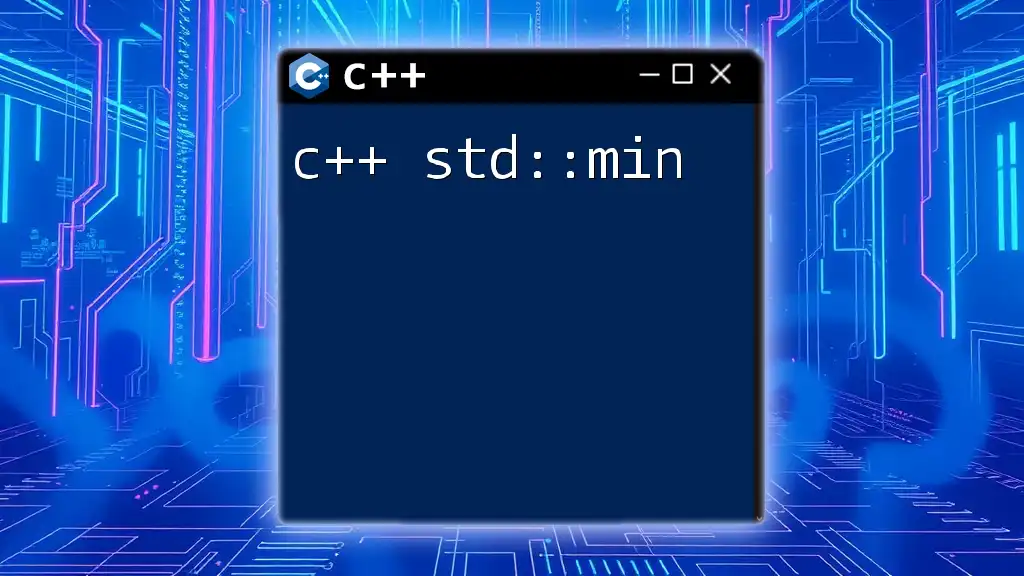
Best Practices and Common Pitfalls
Best Practices for std::any Usage
When using std::any, adhere to the following best practices:
- Use it when necessary: Avoid using std::any when you can achieve the same with a variant or optional; the overhead of dynamic memory could be avoided.
- Manage performance carefully: Since std::any may introduce memory allocation, analyze whether its use is justified in performance-critical sections of your code.
Common Mistakes to Avoid
The most frequent mistake occurs when using std::any_cast without prior type checking. Always ensure to check the type by using TypeInfo or has_value() before attempting to cast. Failure to do so results in unexpected exceptions and can crash your program.
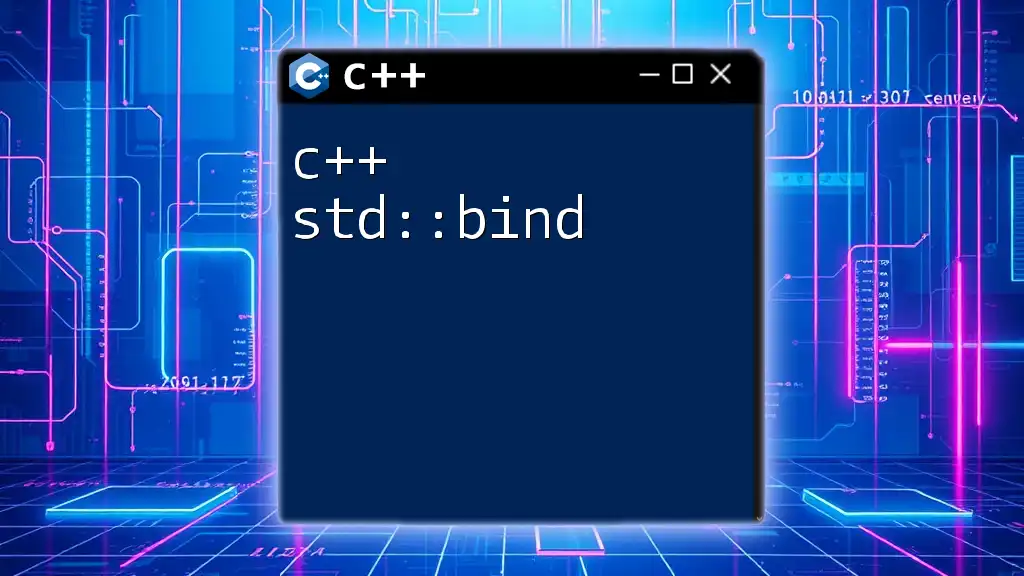
Conclusion
C++ std::any provides a versatile and powerful mechanism for handling heterogeneous data in a type-safe manner. By understanding and leveraging its features, developers can create flexible and dynamic applications.
Future of std::any in C++ Development
As C++ continues to evolve, the utility and efficiency of std::any are likely to improve, and its integration into popular libraries and frameworks may increase. Staying updated on changes in the C++ standards will help developers utilize this feature effectively.
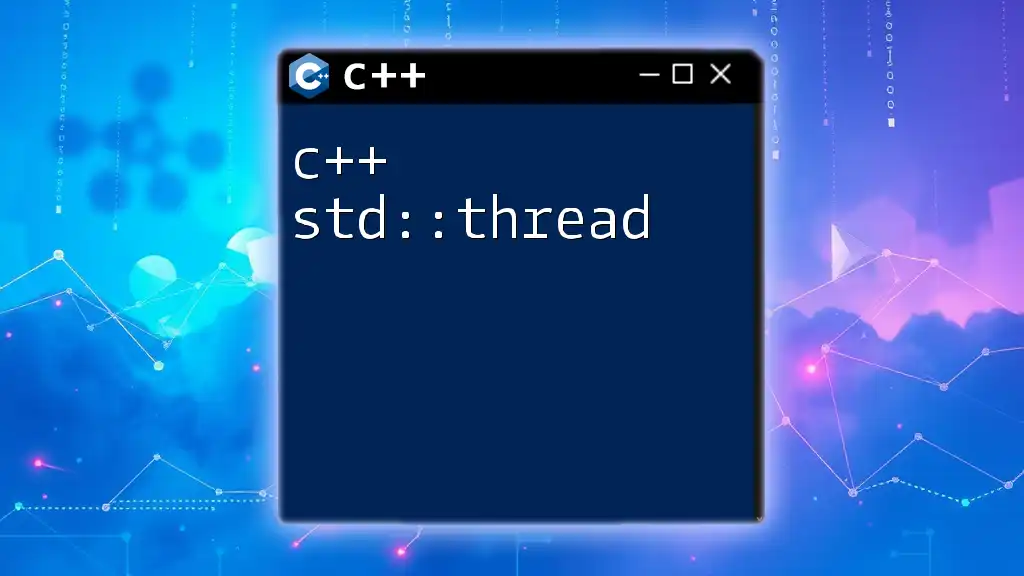
Additional Resources
While this guide covers the basics, diving deeper into the official C++ documentation and exploring community discussions can provide you with valuable insights into advanced usage and patterns.
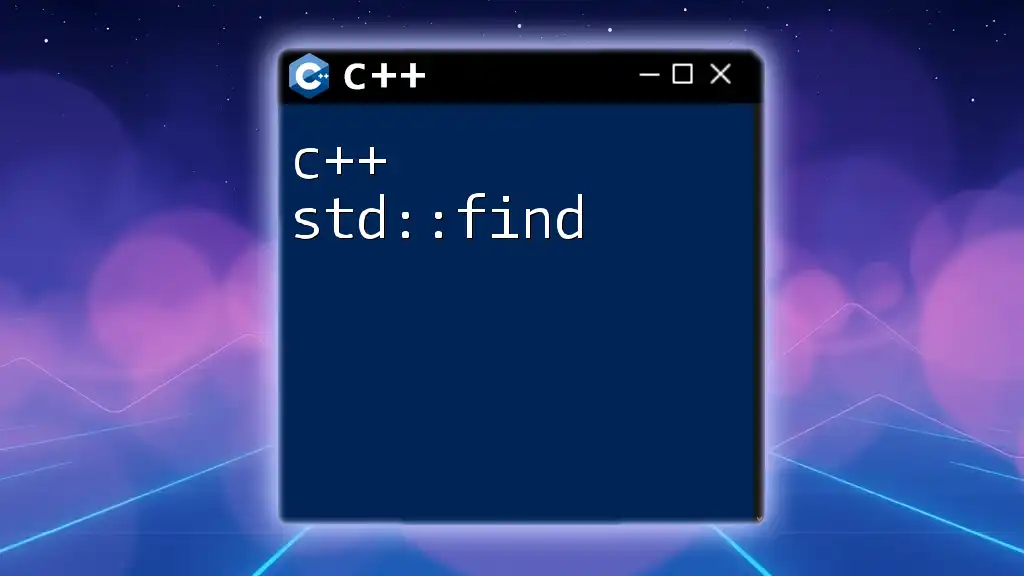
Call to Action
If you're ready to enhance your C++ coding skills, join our community. Engage with others who share your passion and learn how to use features like std::any effectively in real projects!