In C++, a "node" typically refers to an element in a data structure (like a linked list or tree) that contains data and may link to other nodes, enabling efficient data organization and traversal.
Here's a simple example of a node structure in a linked list:
struct Node {
int data; // Data part
Node* next; // Pointer to the next node
Node(int value) : data(value), next(nullptr) {} // Constructor
};
What is Node C++?
Node C++ refers to the practice of using C++ to create native Node.js modules, which allows developers to harness the performance and capabilities of C++ within a Node.js environment. This combination is particularly powerful for applications that require high performance or need to interface with existing C++ libraries or systems.
Node C++ has diverse applications in various domains, including web servers, real-time data processing, and performance-sensitive tasks. Compared to other programming environments, Node C++ offers the advantage of leveraging C++’s speed while maintaining Node.js's asynchronous, event-driven programming model.
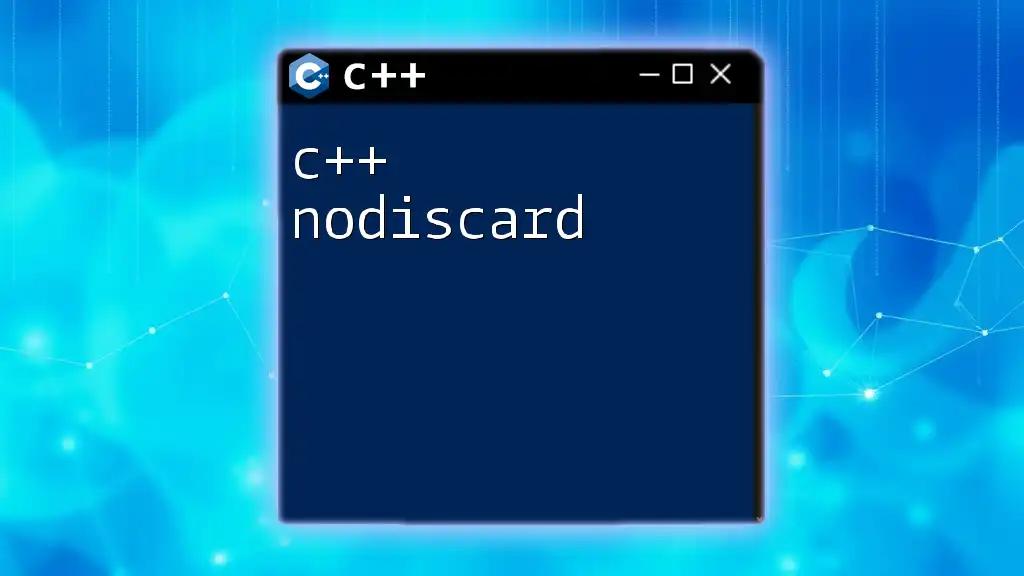
Setting Up Your Environment for C++ Node Development
Required Tools and Software
To develop with C++ Node, you will need a few essential tools:
- C++ Compilers: Popular choices include GCC (GNU Compiler Collection), Clang, and Visual Studio C++. Each compiler has its strengths and setup preferences depending on your operating system.
- Node.js: This is the backbone of your Node application; ensure you have the latest version installed.
- Text Editors and IDEs: Tools such as Visual Studio Code or JetBrains CLion are ideal for coding. They provide many useful features like syntax highlighting, debugging, and version control integrations.
Installation Guide
To get started with C++ Node development, you need to install the tools mentioned above. Begin by downloading and installing Node.js from the official website. Follow the instructions specific to your operating system.
Next, set up the C++ development environment based on the compiler of your choice:
- For GCC, ensure your system has it installed and set up the necessary environment variables.
- For Clang, download it according to the prompts for your platform.
- For Visual Studio, use the installer to select the C++ components you need.
After your environment is set up, you will link C++ libraries with Node.js applications, which can usually be done via npm or manual linking.
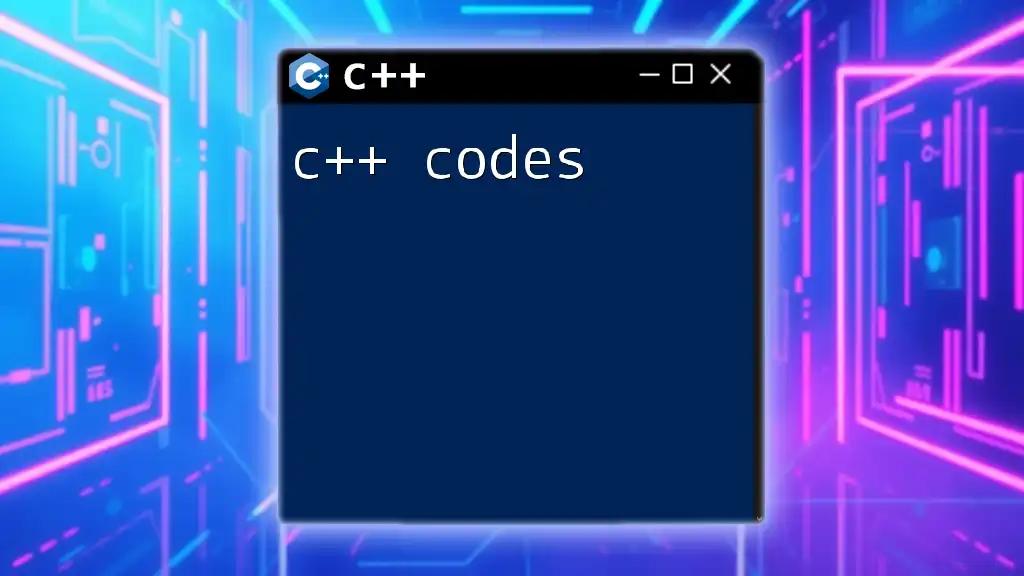
Understanding Node C++ Basics
Structure of Node C++ Code
A standard Node C++ program comprises several key components – libraries, functions, and the module initialization process. Familiarizing yourself with the fundamental structure is crucial for effective coding.
Consider this simple example of a C++ Node module that returns "Hello, World!" when called:
#include <node.h>
#include <v8.h>
void HelloWorld(const v8::FunctionCallbackInfo<v8::Value>& args) {
v8::Isolate* isolate = args.GetIsolate();
args.GetReturnValue().Set(v8::String::NewFromUtf8(isolate, "Hello, World!").ToLocalChecked());
}
void Initialize(v8::Local<v8::Object> exports) {
exports->Set(v8::String::NewFromUtf8(exports->GetIsolate(), "hello").ToLocalChecked(),
v8::FunctionTemplate::New(exports->GetIsolate(), HelloWorld)->GetFunction());
}
NODE_MODULE(NODE_GYP_MODULE_NAME, Initialize)
In this code snippet, `HelloWorld` is a simple function that takes parameters, and the `Initialize` function binds this to a JavaScript callable object.
Understanding V8 and Node's JavaScript Engine
At the core of Node.js is the V8 engine, which compiles JavaScript directly to native machine code. Understanding V8 is essential for C++ Node development, as it facilitates the interaction between C++ and JavaScript.
C++ interacts with JavaScript objects through V8's API, which allows developers to manipulate and exchange data between the two languages seamlessly. This interplay is vital for performance-oriented applications where efficiency is crucial.
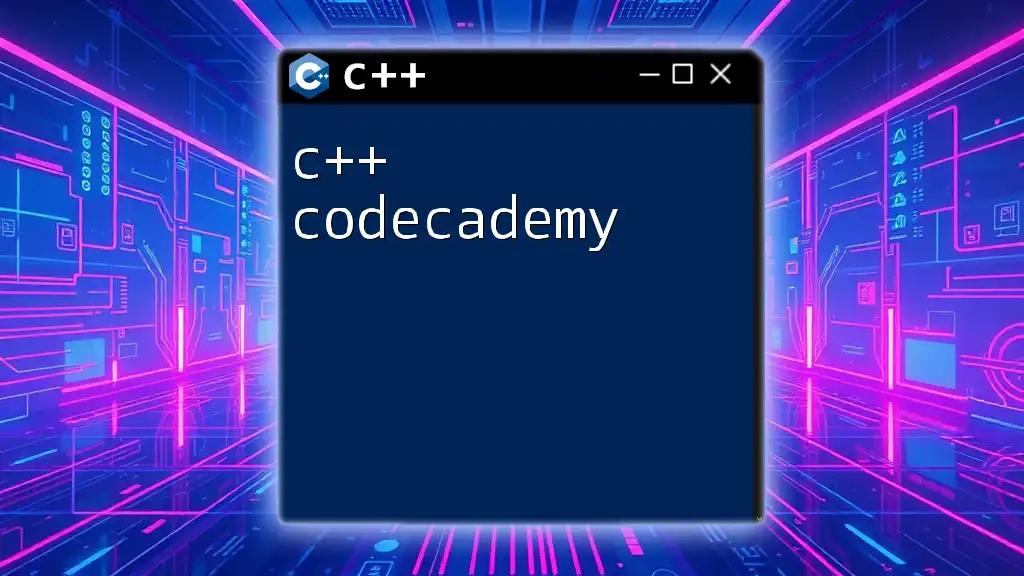
Key Concepts in C++ Node Development
Creating a Native Node Module
Creating your first native Node module using C++ is straightforward. Start by organizing your project structure neatly. Here's how you can create a simple module:
- Create a folder for your project and navigate into it.
- Initialize a new Node.js project:
npm init -y
- Create a C++ file (e.g., `hello.cc`) and write your code.
- Create a binding file (e.g., `binding.gyp`) to configure the build process.
Here is an example of a `binding.gyp` file:
{
"targets": [
{
"target_name": "hello",
"sources": ["hello.cc"]
}
]
}
After that, use `node-gyp` to configure and build your module.
Compiling and Building the Module
node-gyp is a powerful tool that automates the building of native add-ons for Node.js. It manages the compilation of your C++ code. To compile your code, run the following commands in your project's directory:
node-gyp configure
node-gyp build
This process will create a build directory and compile your C++ code into a .node file, which you can then require in your JavaScript code just like any other Node module.
Error Handling in Node C++
Effective error handling is critical in C++ Node applications, especially because unmanaged errors can lead to crashes or unexpected behavior.
Node provides mechanisms to handle errors gracefully. Below is how to throw an error if a certain condition fails in your C++ code:
if (someErrorCondition) {
isolate->ThrowException(v8::Exception::Error(v8::String::NewFromUtf8(isolate, "An error occurred").ToLocalChecked()));
return;
}
This code will send an error back to the JavaScript context, allowing you to handle it appropriately on the Node side.
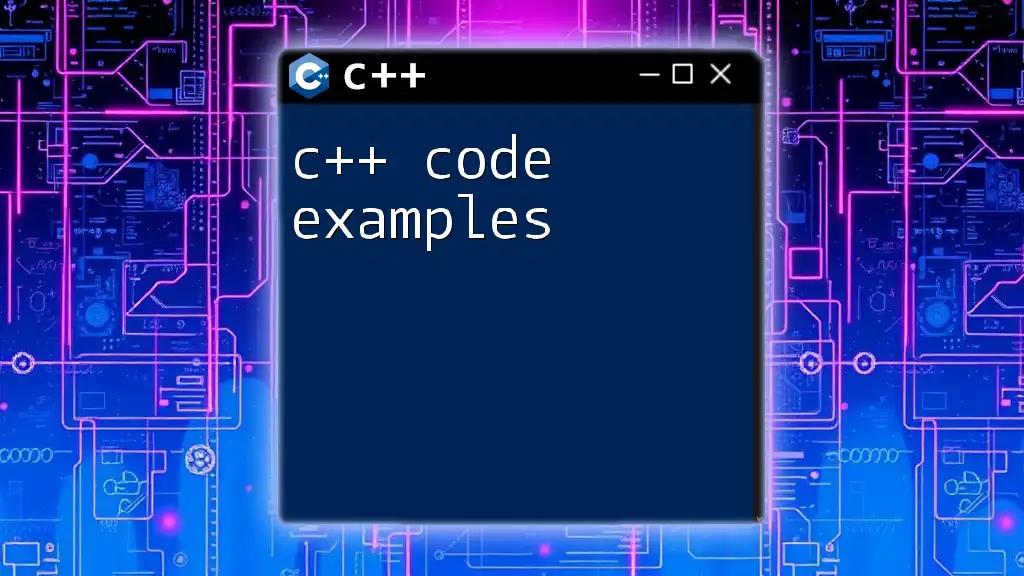
Real-World Applications of C++ Node
Performance-Intensive Applications
Certain applications necessitate the efficiency that C++ brings to the table. Examples include:
- Real-time data processing: Processing vast amounts of data without significant delays.
- Image processing: Utilizing libraries such as OpenCV for advanced graphics tasks.
- Game development: Enhancing Node.js-based game servers with high-performance routines.
Using C++ in these contexts dramatically improves performance, making it an indispensable part of the architecture.
Integrating C++ with External Libraries
Integrating existing C++ libraries enables you to harness their functionality without reinventing the wheel. A common use case is interfacing with libraries like OpenCV.
For example, to use OpenCV in your project, you need to modify your `binding.gyp` to link against the OpenCV libraries, and then you can create bindings that allow you to call library functions from your Node.js code.
Here's an example of writing a function that uses OpenCV to process an image, maintaining the same structure as before and adapting it for a specific library.
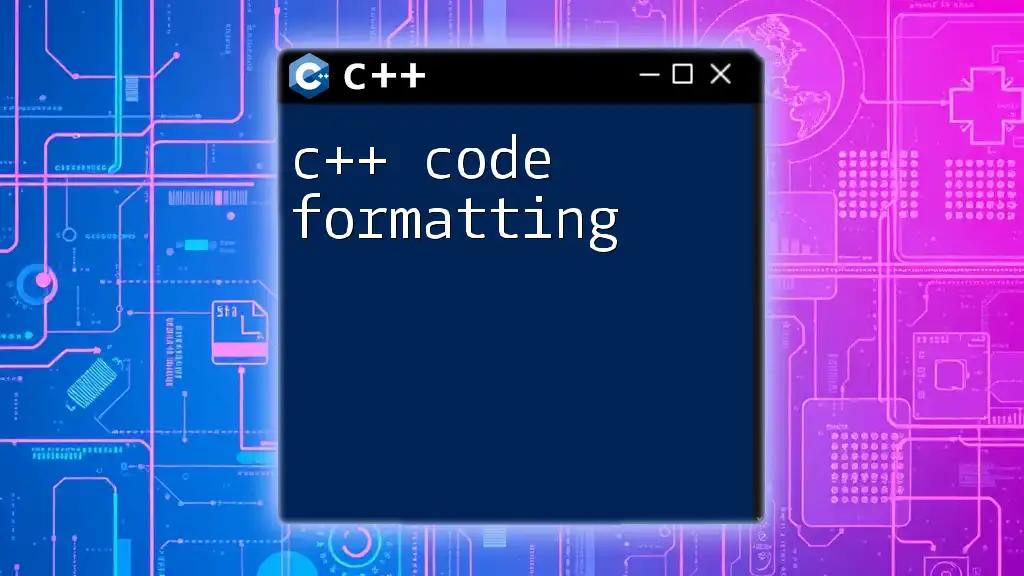
Best Practices for C++ Node Development
Code Organization and Module Structure
Maintaining a clear project structure is crucial. A typical layout could look like:
/my-node-module
|-- package.json
|-- binding.gyp
|-- src/
| |-- hello.cc
|-- include/
| |-- hello.h
This organization facilitates better code management and enhances collaboration among team members.
Debugging Techniques
Debugging C++ within Node can be challenging, but effective tools can aid significantly. gdb (GNU Debugger) is one such tool that allows you to debug your C++ code.
When debugging, consider using console logging in both your C++ and JavaScript files to trace the flow of execution and quickly identify where things go wrong. Additionally, tools like Valgrind can help identify memory leaks, which is a common issue in C++ applications.
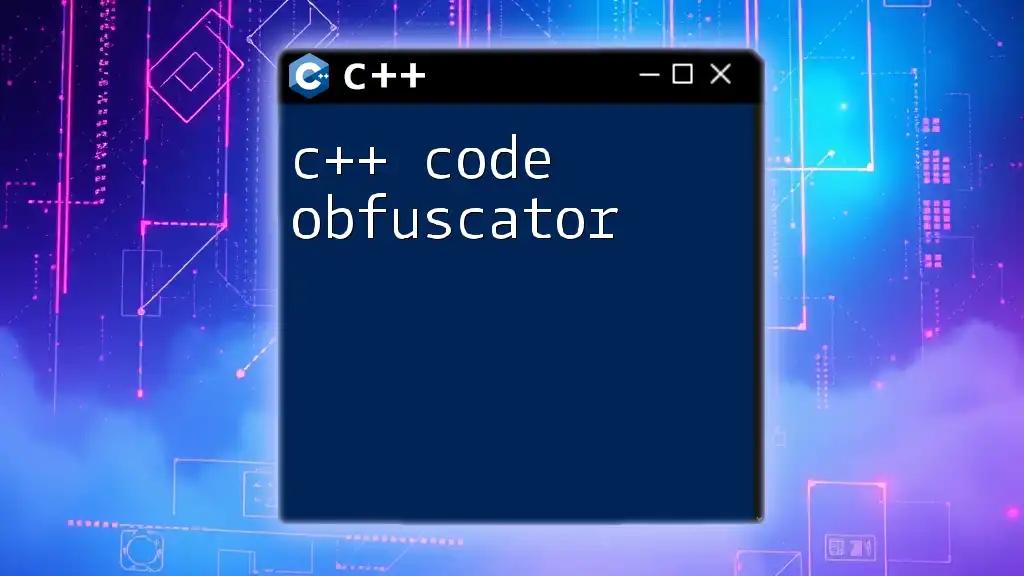
Conclusion
The synergy between C++ and Node.js opens up exciting possibilities for developing high-performance applications. By understanding how to create and manage C++ Node modules, you can significantly enhance the efficiency of your JavaScript applications. Armed with the knowledge of V8, error handling, and integration techniques, you are well on your way to mastering C++ Node development.
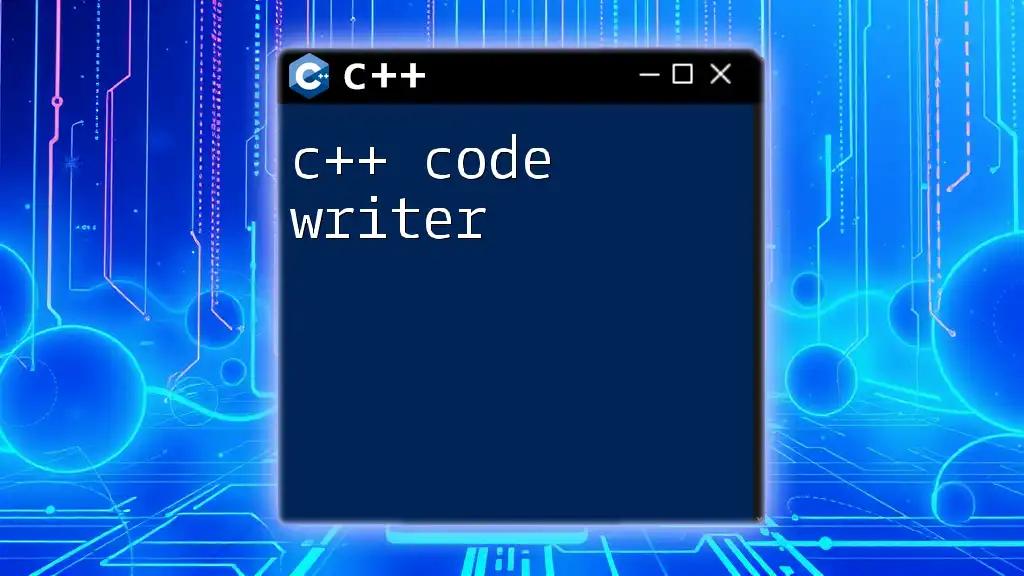
Additional Resources
For those eager to continue their learning journey, consider exploring recommended books such as C++ Primer or Professional Node.js. Additionally, online courses offer structured pathways for skill enhancement. Engage with documentation and participate in community forums for support and guidance.
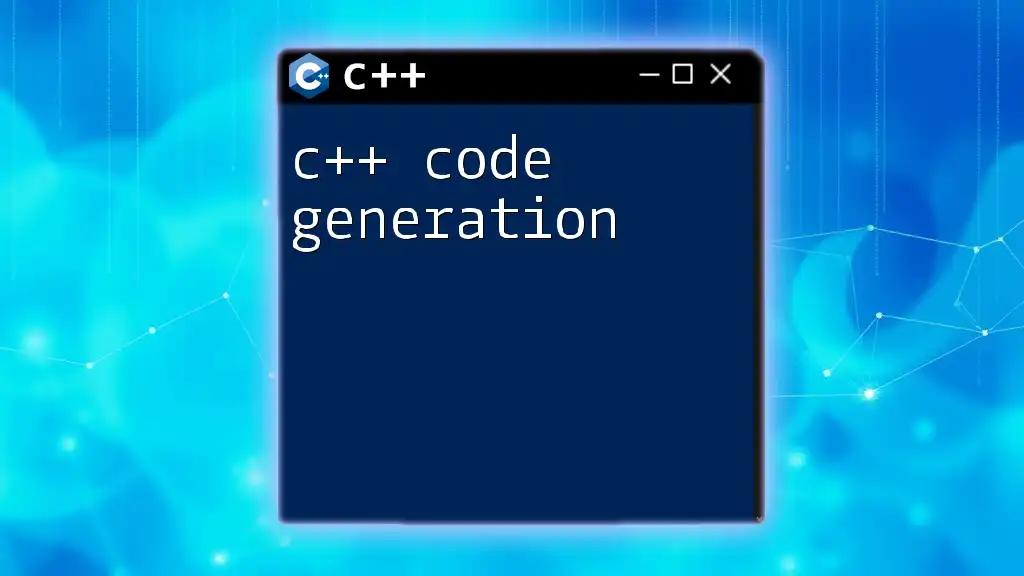
Call to Action
We invite you to share your experiences with C++ Node. What projects are you working on? Have you faced challenges that we haven’t covered? Your insights and questions can help foster a thriving community of C++ Node enthusiasts!