In C++, `cin` is used for input operations, allowing users to read data from standard input, while `cout` is used for output operations, enabling users to display data to standard output.
Here’s a simple example demonstrating the use of `cin` and `cout`:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
What is cin?
Definition of cin in C++
`cin` is an object of the `istream` class and is used to receive input from the standard input stream, which is usually the keyboard. When you use `cin`, you effectively allow your program to read user input during execution.
How cin Works
`cin` takes input in the form of data types such as integers, floating-point numbers, or strings depending on how you define the variable receiving the input. This is done using the extraction operator (`>>`), which reads formatted input.
Example of Using cin
Here’s a simple illustration of how to use `cin`:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this example, the program prompts the user to enter their age. The input taken from the user is stored in the variable `age`, and then it is displayed back using `cout`. This demonstrates a basic input-output cycle.
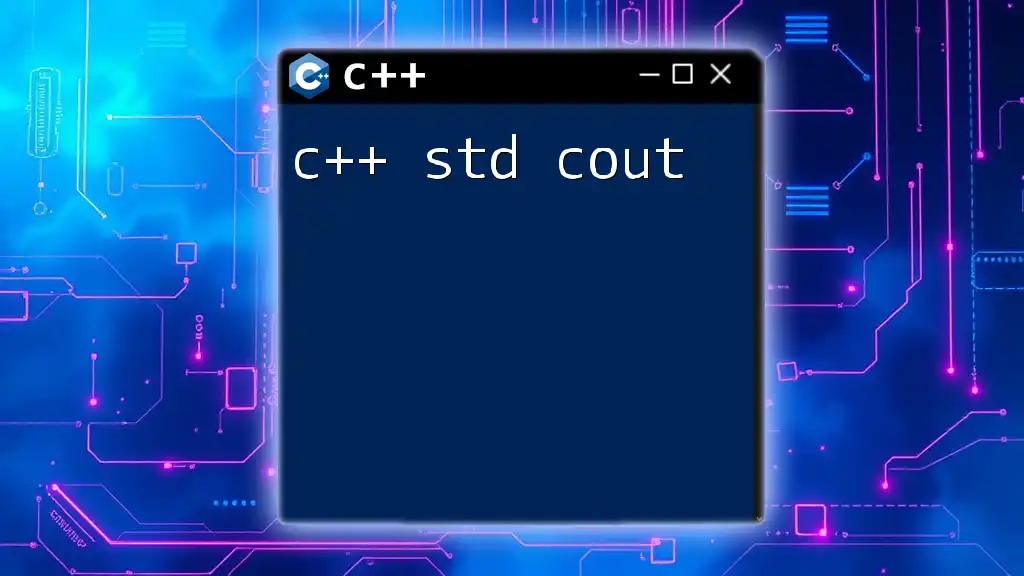
What is cout?
Definition of cout in C++
`cout` is an object of the `ostream` class, and it is used for outputting data to the standard output stream, typically the console.
How cout Works
Similar to `cin`, `cout` uses the insertion operator (`<<`) to send data to the output stream. You can send multiple items in a single statement, and `endl` can be used to insert a new line.
Example of Using cout
Here’s a straightforward example of using `cout`:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this case, the program outputs "Hello, World!" to the console. The `endl` ensures that the output cursor moves to the next line after printing.
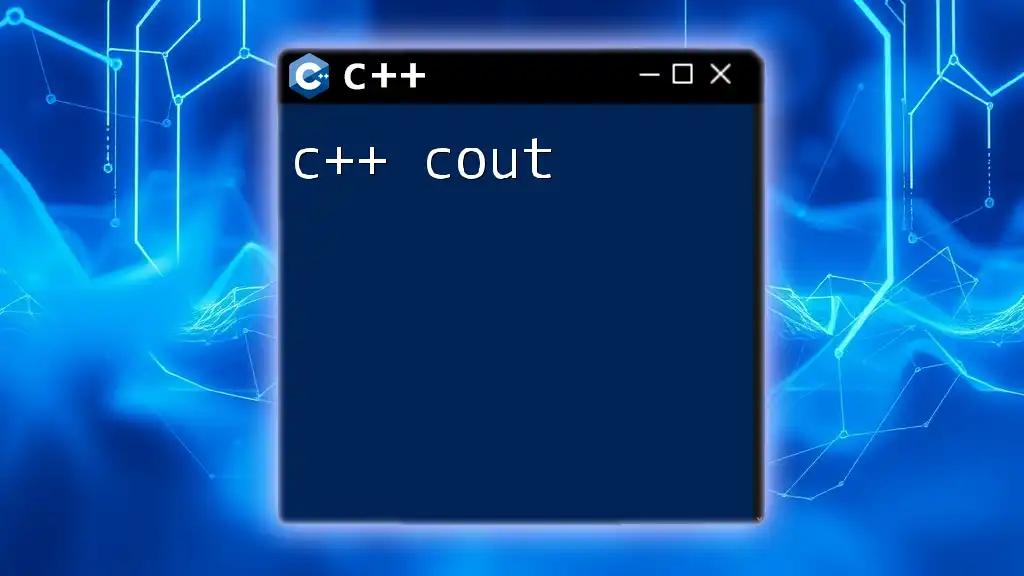
Working with cin and cout Together
Combined Usage of cin and cout
The power of C++ programming becomes more apparent when combining `cin` and `cout` for interactive programs. You can ask for user input and then provide immediate feedback.
Example: User Input and Response
Here’s an example that blends both concepts:
#include <iostream>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Hello, " << name << "!" << endl;
return 0;
}
In this code snippet, the program first asks for the user’s name, takes input using `cin`, and then greets the user by name with `cout`. It showcases how to create a simple interactive experience.
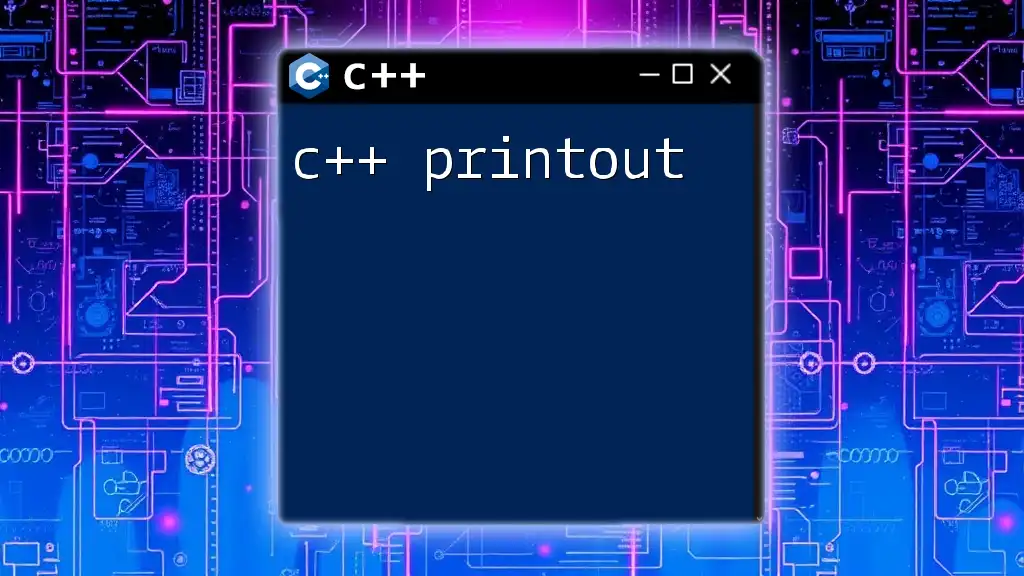
Formatting Output with cout
Using Format Manipulators
C++ offers manipulators that can enhance the way you present your output. Common manipulators include `endl` for new lines, `setw` for setting the width of output, and `setprecision` for controlling decimal places when dealing with floating-point numbers.
Example: Formatting Numbers
Here’s a demonstration of using format manipulators:
#include <iostream>
#include <iomanip> // for setprecision
using namespace std;
int main() {
double num = 5.6789;
cout << "Formatted number: " << fixed << setprecision(2) << num << endl;
return 0;
}
In this example, the output will display the number formatted to two decimal places due to the use of `fixed` and `setprecision`. This improves the readability of numerical output, which is often critical in applications involving finance or measurements.
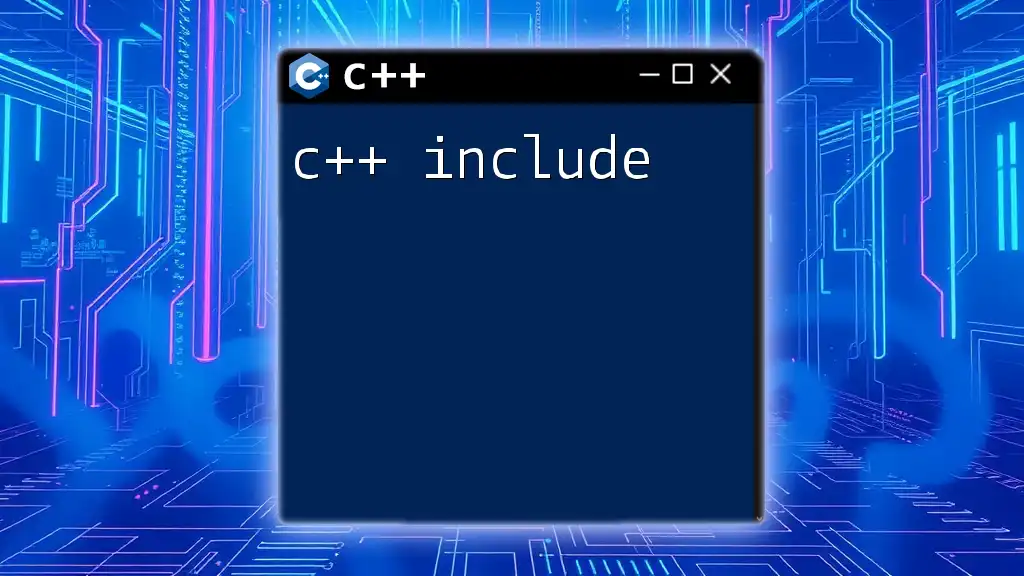
Error Handling with cin
Common Errors When Using cin
While `cin` is powerful, it is not infallible. Users might provide input that doesn't match the expected data type. This can lead to failure in reading input, resulting in an error state.
Example: Checking Input Validity
Here’s how you can handle errors when using `cin`:
#include <iostream>
#include <limits> // for numeric_limits
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
while (!(cin >> num)) {
cout << "Invalid input. Please enter a number: ";
cin.clear(); // clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // discard the invalid input
}
cout << "You entered: " << num << endl;
return 0;
}
In this snippet, the program continues prompting the user until a valid number is entered. If an invalid input is detected, the error state is cleared using `cin.clear()`, and the invalid input is discarded with `cin.ignore()`. This ensures that the program does not enter an infinite loop or crash due to unexpected input.
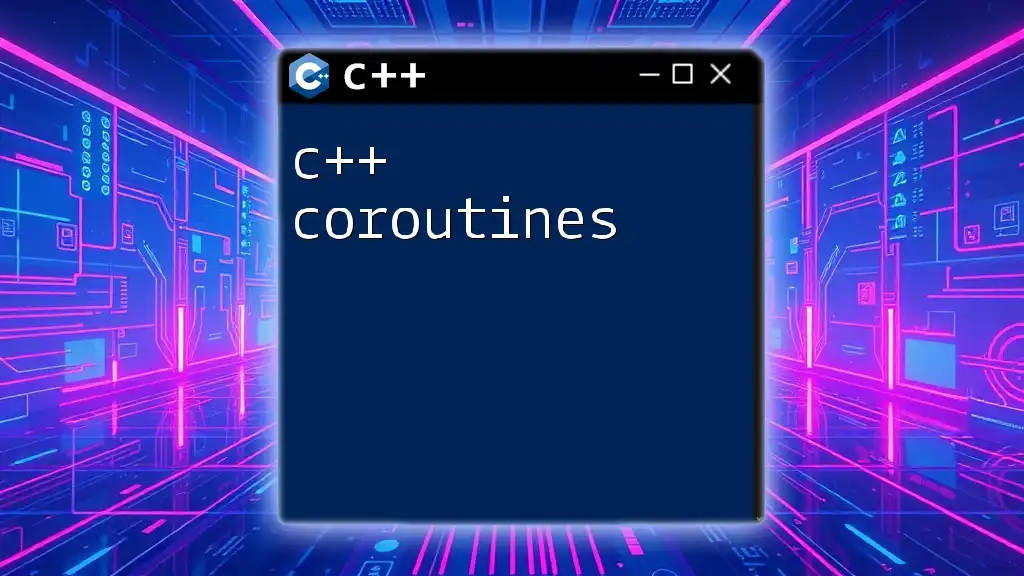
Conclusion
In summary, understanding c++ cin cout is essential for any C++ programmer aiming to create interactive applications. By mastering input and output methods, you equip yourself with the foundational skills necessary for building more complex programs.
Utilizing `cin` allows for flexible user input while `cout` enables effective display of information. Error handling with `cin` ensures that your program can gracefully manage unexpected input, making your applications robust.
As you practice using `cin` and `cout`, experiment with various scenarios to deepen your understanding. Share your experiences, questions, or unique challenges related to C++ I/O!