Code::Blocks is an open-source Integrated Development Environment (IDE) that facilitates the writing, compiling, and debugging of C++ programs, making it an excellent choice for both beginners and experienced developers.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Code Structure
Basic Syntax of C++
C++ has a specific syntax that is fundamental to understanding how to write effective code. The main components include data types, variables, and constants.
Data types such as `int`, `float`, and `char` form the backbone of data representation in C++. For instance:
int age = 30;
float weight = 70.5;
char grade = 'A';
Variables are used to store data, while constants are fixed values that do not change during the execution of a program. To declare a constant, you might use:
const int MAX_AGE = 100;
Control Structures
Control structures are crucial in C++ as they dictate the flow of execution based on certain conditions. The most common control structures are if statements and switch statements.
If Statement: This allows the execution of code blocks based on whether a condition evaluates to true.
if (age > 18) {
cout << "You are an adult.";
}
Switch Statement: This provides an efficient way to execute different code blocks based on the value of a variable.
switch (grade) {
case 'A':
cout << "Excellent!";
break;
case 'B':
cout << "Well done!";
break;
default:
cout << "Grade not recognized.";
}
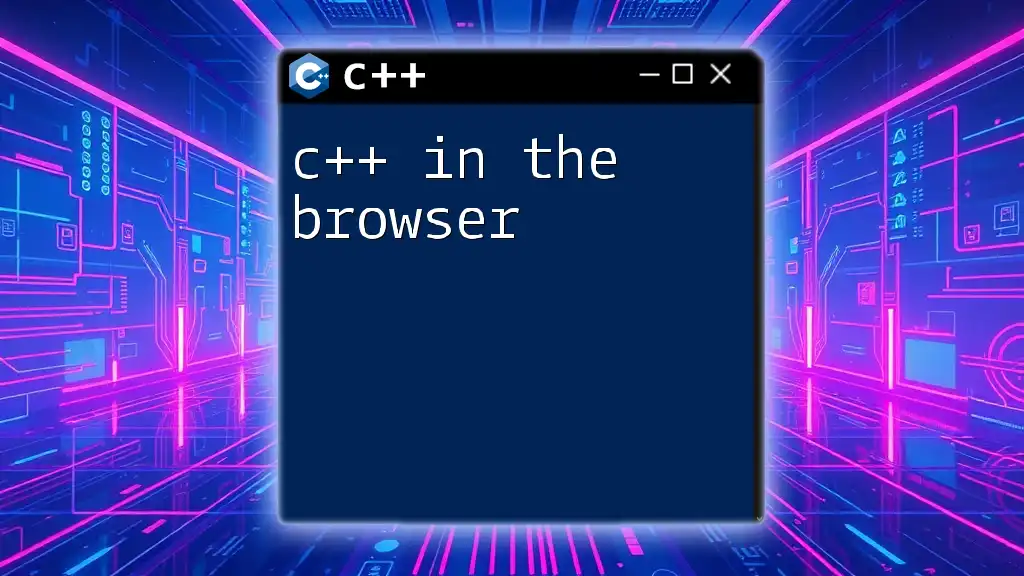
Code Blocks and Scope
What are Code Blocks?
Code blocks in C++ are defined sections of code surrounded by curly braces `{}`. They group multiple statements together, allowing a set of instructions to execute as a single unit. Each code block has its own scope, which defines the visibility of variables declared within it.
For example:
{
int localVariable = 10;
cout << localVariable; // This is accessible
}
// cout << localVariable; // This would cause an error due to scope
Nested Code Blocks
Nested code blocks occur when a code block is contained within another code block. This can lead to complex structures, and it's essential to ensure that variables do not leak into unintended scopes.
if (true) {
int outerVariable = 5;
{
int innerVariable = 10;
cout << outerVariable + innerVariable; // Output: 15
}
// cout << innerVariable; // Error: innerVariable is not accessible here
}
Understanding the rules of scoping in nested blocks is vital for maintaining the integrity of your variables and logic.
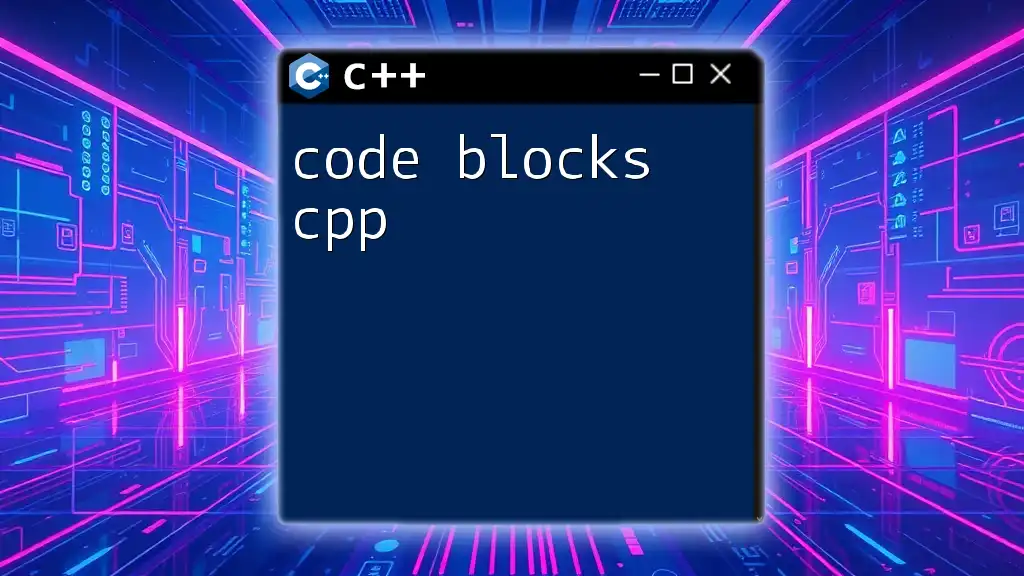
Functions and Code Blocks
Defining Functions in C++
Functions encapsulate a block of code that performs a specific task and can be reused throughout your program. They enhance modularity and make code easier to maintain.
A function in C++ can be defined as follows:
void greet() {
cout << "Hello, welcome to C++!";
}
Passing Parameters and Return Types
Functions can accept parameters, allowing you to pass data into them for processing. The syntax generally looks like this:
int add(int a, int b) {
return a + b;
}
This `add` function demonstrates how two integers can be passed in, and their sum is returned.
Example:
cout << add(5, 10); // Output: 15
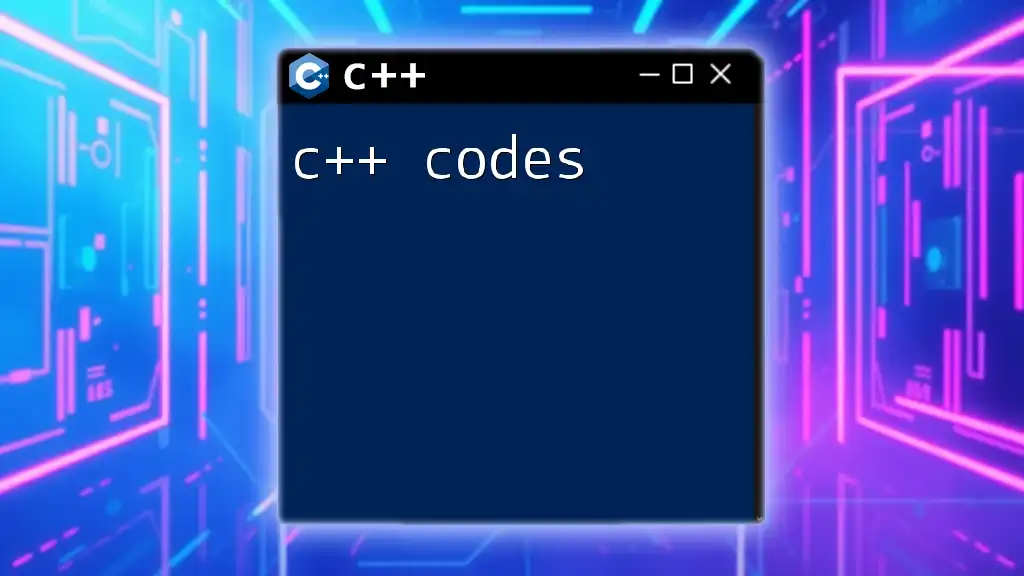
Using Code Blocks in Control Statements
Conditional Statements
Conditional statements utilize code blocks for executing different paths of logic. The if, else, and switch statements all benefit from well-structured code blocks to maintain clarity and functionality.
if (age < 18) {
cout << "Minor";
} else {
cout << "Adult";
}
Loop Structures
Loops are another area where code blocks shine in C++. The `for`, `while`, and `do-while` loops can execute a block of code repeatedly until a certain condition is met.
A classic example using a for-loop is:
for (int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
The block within the for-loop will run five times, each time printing the current iteration count.
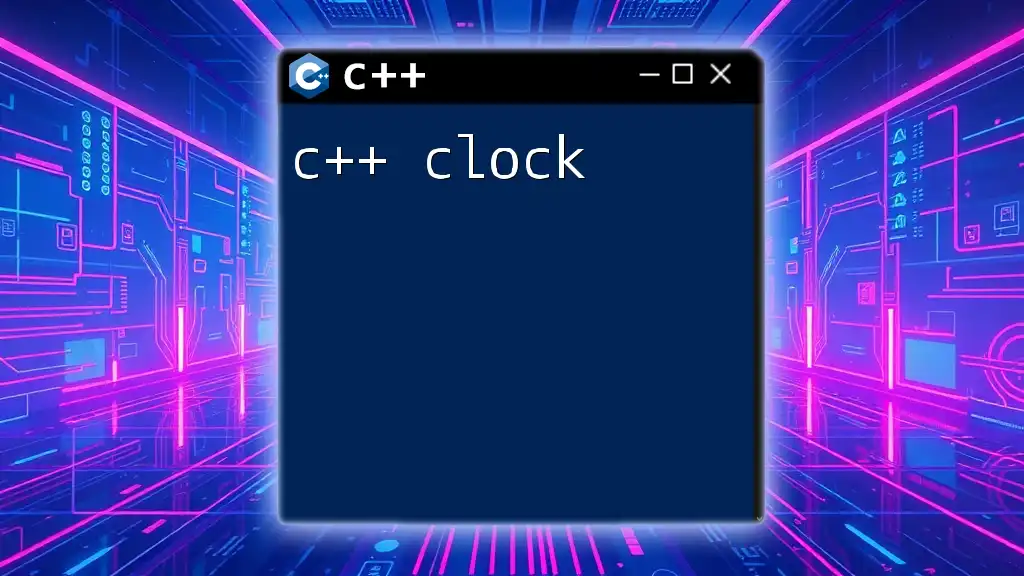
Best Practices for Writing Code Blocks
Readability and Maintenance
Writing readable code is just as important as making it functional. Proper indentation and spacing within code blocks improve readability, enabling anyone to understand the flow at a glance.
A well-structured code block looks like this:
if (condition) {
// Positive condition logic
executeTask();
} else {
// Negative condition logic
executeAlternativeTask();
}
Commenting Code Blocks
Comments play a crucial role in coding as they provide context and explanation to the code. Utilizing comments effectively can demystify complex code blocks for future reference or for other programmers.
Example:
// This function calculates the area of a rectangle
int calculateArea(int width, int height) {
return width * height; // Return the calculated area
}
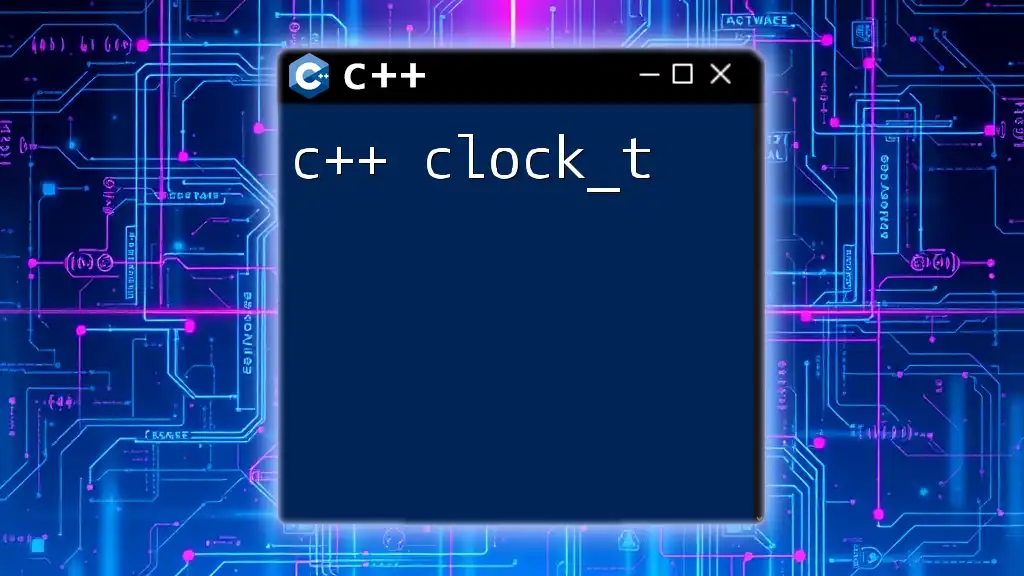
Debugging Code Blocks in C++
Common Issues with Code Blocks
When dealing with code blocks, some common issues can arise, such as using uninitialized variables or misunderstanding scope. An uninitialized variable may yield unpredictable results.
Example of an error:
int x; // Uninitialized
cout << x; // Output is undefined
To avoid these issues, ensure that variables are initialized before use, and be mindful of their scope.
Tools for Debugging Code Blocks
Debugging tools provide a powerful way to identify and resolve problems within your code. Popular tools include GDB and Visual Studio Debugger, which allow you to step through your code, examine variable values, and confirm logic flow.
Using these tools in conjunction with well-organized code blocks can streamline the debugging process significantly.
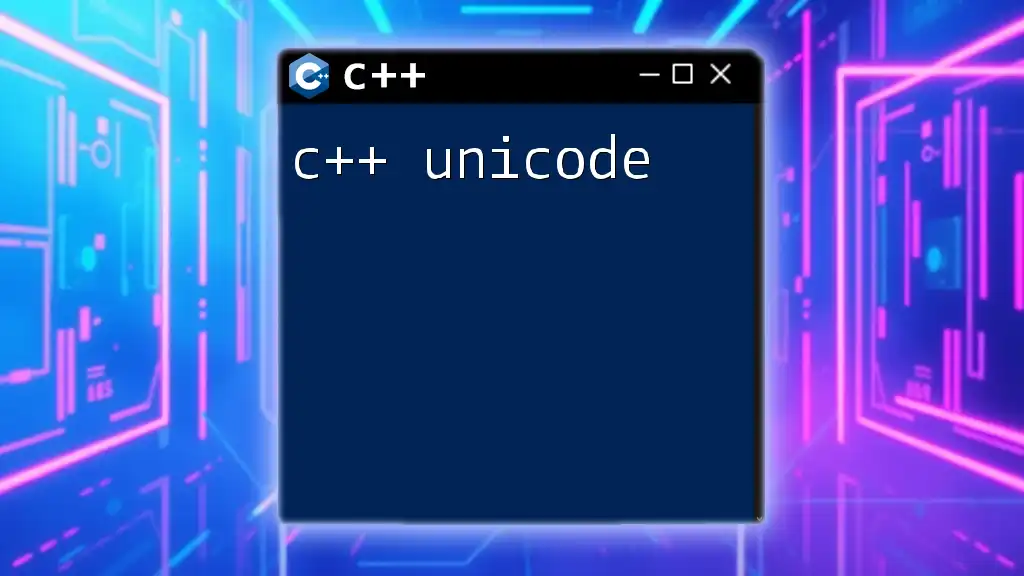
Conclusion
In conclusion, understanding C++ in code blocks is essential for writing clear, efficient, and maintainable code. By mastering the use of code blocks, control structures, functions, and best practices, you lay a strong foundation for greater programming success.
For those eager to enhance your skills further, consider joining a C++ learning community where you can collaborate, share ideas, and grow your proficiency in C++. Happy coding!
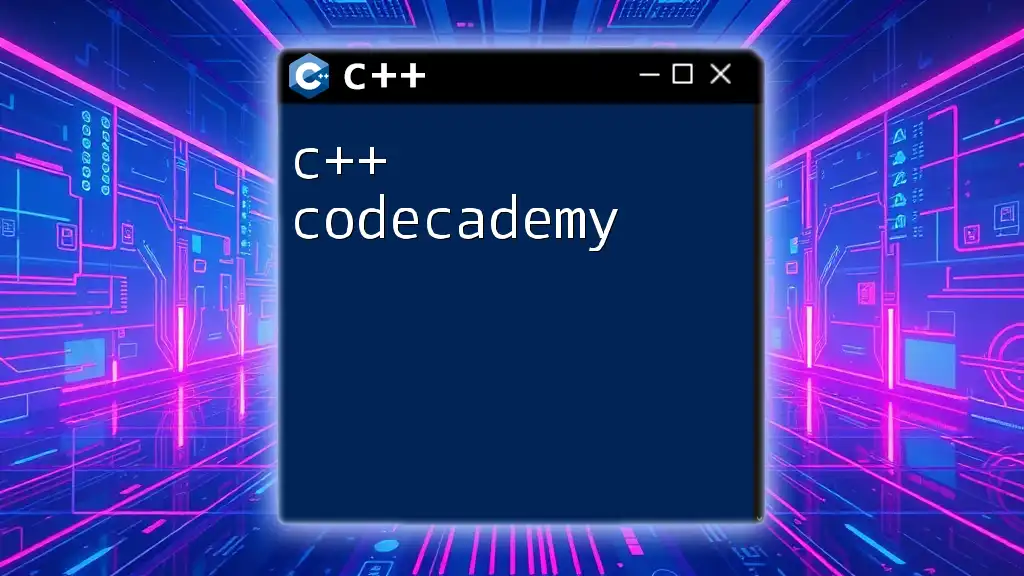
References and Further Reading
For a deeper dive into the intricacies of C++, consider exploring the following resources:
- Books: "The C++ Programming Language" by Bjarne Stroustrup
- Online Resources: Cplusplus.com, Codecademy C++ Course
- Tutorials: LearnCPP.com, C++ for C Programmers