C++ in the browser allows developers to run C++ code directly in web applications using tools like WebAssembly, enabling high-performance applications that can be executed on various devices without the need for native installations.
Here's a simple example of C++ code that can be compiled to WebAssembly:
#include <iostream>
extern "C" {
int add(int a, int b) {
return a + b;
}
}
The Evolution of C++
C++ has long been a favored language for system-level programming and high-performance applications. Traditionally, developers relied on it for building native applications that run on desktops and servers. However, the growing demand for interactive web applications has initiated a significant transformation. Developers are now exploring how to leverage C++ in the browser, enabling its powerful features to be utilized in a web context.
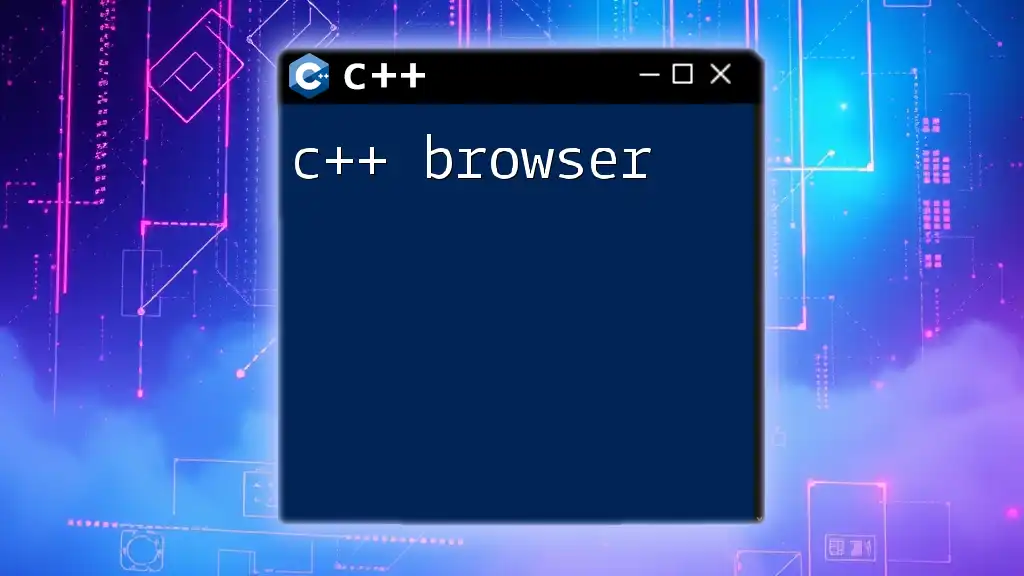
What Does "C++ in the Browser" Mean?
Understanding WebAssembly
At the core of running C++ in the browser is WebAssembly (Wasm), a binary instruction format that allows low-level languages, like C++, to be compiled and executed in web environments.
WebAssembly provides a way for code written in languages such as C, C++, and Rust to run efficiently alongside JavaScript. This capability enables a new class of applications that require fast execution times and efficient memory usage.
Example: The Wasm binary format is designed to be executed by the web browser's virtual machine, boasting significant portability and performance advantages over standard JavaScript.
Compiling C++ to WebAssembly
To harness the power of C++ in the browser, developers can use the Emscripten toolchain. Emscripten compiles C++ code into WebAssembly, making it runnable in the browser environment.
Step-by-Step Guide to Set Up Emscripten:
- Download and install Emscripten application from the official website.
- Set up the development environment by configuring the necessary paths and dependencies.
- Once Emscripten is configured, you can compile your C++ code easily.
To compile a simple C++ program into WebAssembly, you can use the following command:
emcc hello.cpp -o hello.html
This command converts the `hello.cpp` file into an executable HTML file, containing both the JavaScript glue and the WebAssembly binary code, enabling it to run directly in a web browser.
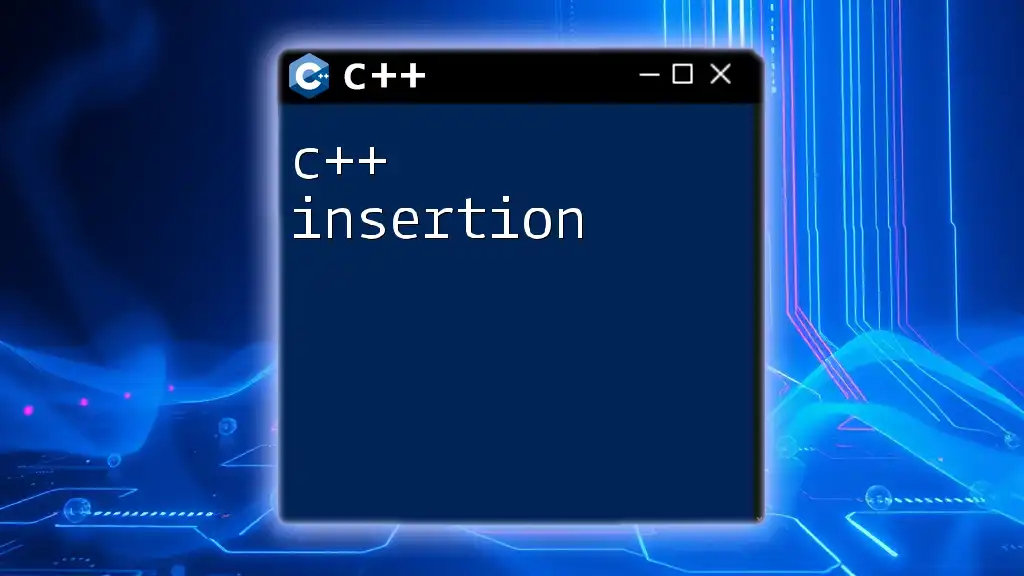
Setting Up Your Development Environment
Required Tools and Software
Before you can start coding, ensure you have the necessary tools installed:
- Emscripten: The primary tool for compiling C++ to WebAssembly.
- Node.js: Optional but useful for server-side runtime environment.
Install these tools following the detailed guides provided on their respective official sites to ensure compatibility and stability.
Writing Your First C++ Program for the Browser
Let's kick off your journey into C++ in the browser with a simple “Hello, World!” program.
#include <iostream>
extern "C" {
void say_hello();
}
void say_hello() {
std::cout << "Hello, World!" << std::endl;
}
In this code:
- The `extern "C"` block ensures that the C++ compiler doesn’t perform name mangling, allowing the function to be called from JavaScript.
- The `say_hello` function outputs “Hello, World!” to the console.
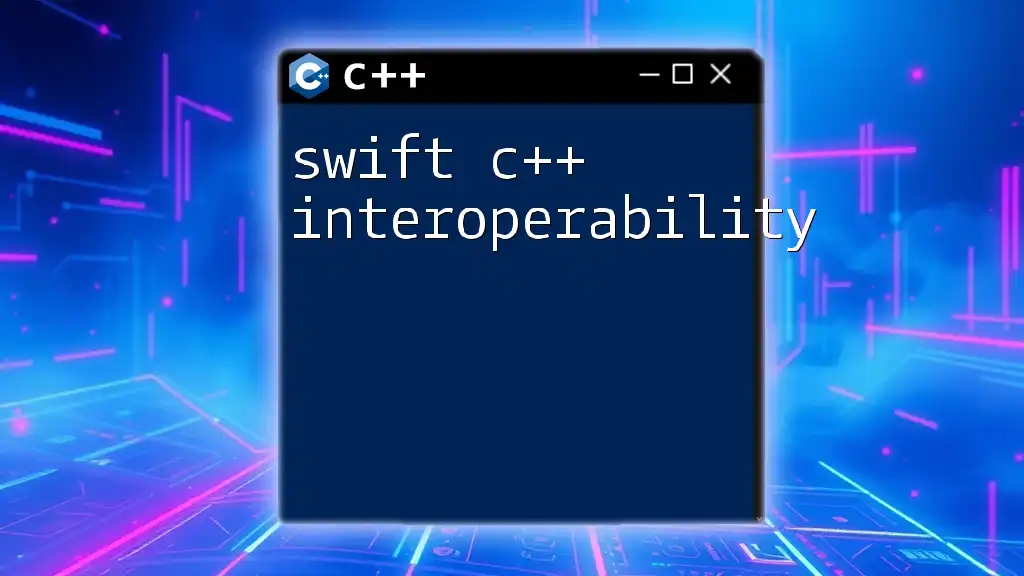
Running C++ Code in Your Browser
Loading Your WebAssembly Module
To see your C++ code in action, you need to load the WebAssembly module into an HTML document:
<!DOCTYPE html>
<html>
<head>
<title>C++ in the Browser</title>
<script src="hello.js"></script>
</head>
<body>
<script>
var Module = {
onRuntimeInitialized: function() {
Module._say_hello();
}
};
</script>
</body>
</html>
In this example, as soon as the runtime is initialized, the `say_hello` function is called, and you'll see “Hello, World!” displayed in the browser's console.
Debugging C++ in the Browser
Debugging is an essential skill for developers working with C++ in the browser. Although WebAssembly can be somewhat opaque, modern browsers provide debugging tools that allow you to inspect code execution.
- Use DevTools to set breakpoints and examine variable states in both C++ and JavaScript code.
- Source Maps: When compiled with Emscripten using the `-g` flag, source maps are created to help map the compiled code back to your original C++ code, simplifying the debugging process.
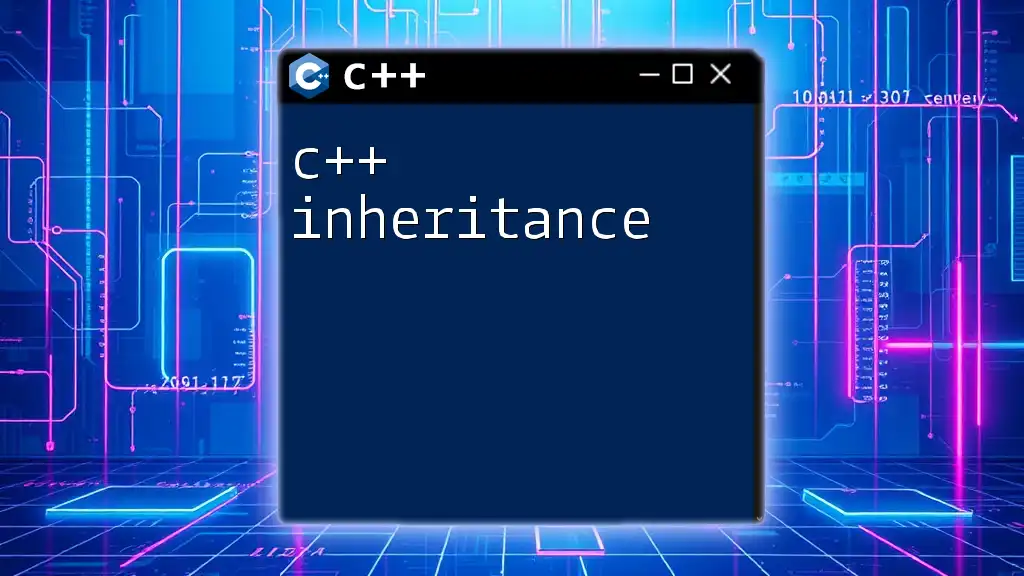
Use Cases of C++ in the Browser
Gaming Applications
C++ shines brightly in the world of game development, especially for browser-based games. Game engines like Unity and Unreal Engine utilize C++ for rendering graphics and managing game physics, and their capabilities can now be extended to the browser.
By using C++ to create high-performance games, developers can achieve smoother gameplay experiences without sacrificing speed or resource management.
Performance-Critical Applications
In addition to gaming, C++ is vital for applications requiring intense computational tasks—like scientific simulations, image processing, and graphics rendering. The power of C++ and its ability to be compiled into Wasm makes it an ideal choice for handling such performance-critical needs.
Consider a simulation of a complex system or a tool for data visualization; using C++ allows developers to optimize their algorithms and data structures for maximum efficiency when deployed in the browser.
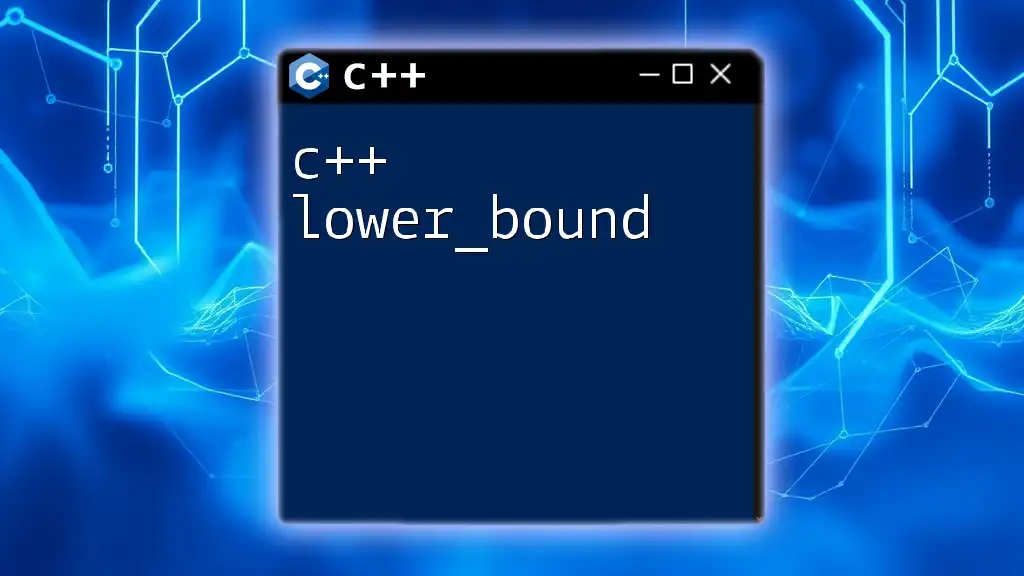
Advantages of Using C++ in the Browser
Performance Benefits
When comparing C++ to JavaScript, WebAssembly provides a considerable performance advantage. The binary format of Wasm is much closer to machine code, allowing it to execute faster than JavaScript’s interpreted code. This advantage is particularly crucial for applications that require rapid computations.
Code Reusability
One of the most significant benefits of utilizing C++ in the browser is the ability to leverage existing codebases. Developers can reuse libraries and tools already built in C++, which saves time and ensures consistency across applications. This cross-platform capability enhances productivity and can lead to faster iterations and a more extensive set of features in web applications.
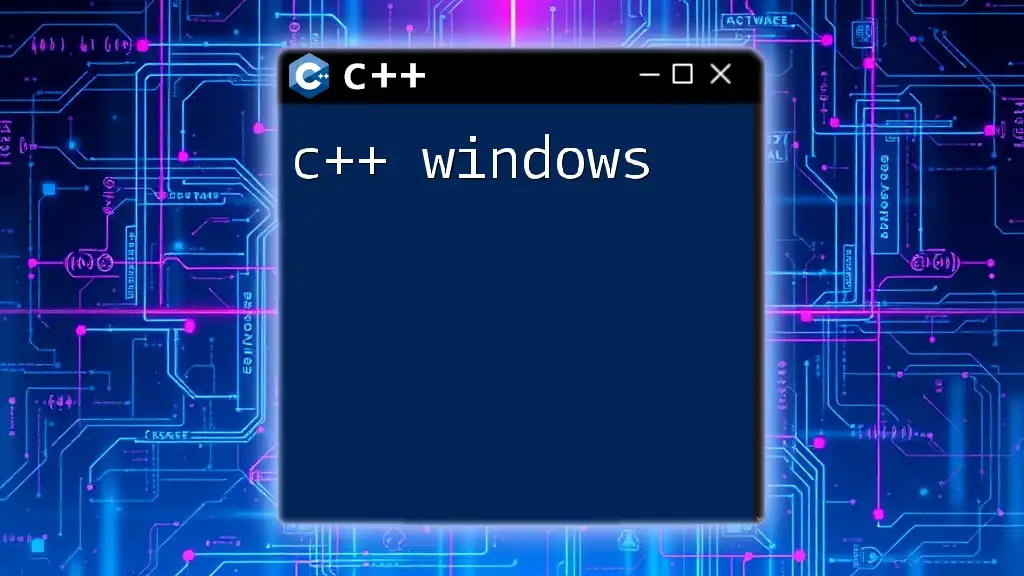
Limitations and Challenges
Compatibility Issues
While WebAssembly and C++ open exciting doors for development, compatibility with older browsers can be a challenge. Developers must consider the browser support for WebAssembly and ensure that their applications function seamlessly even on different platforms.
Debugging and Development Complexity
While tools are improving, debugging C++ code in the browser can be more complex than working solely with JavaScript. Issues like differences in memory management and resource allocation can lead to unexpected behaviors.
To mitigate these challenges, developers should familiarize themselves with best practices, focusing on memory leaks and careful management of resources to ensure stability in their applications.
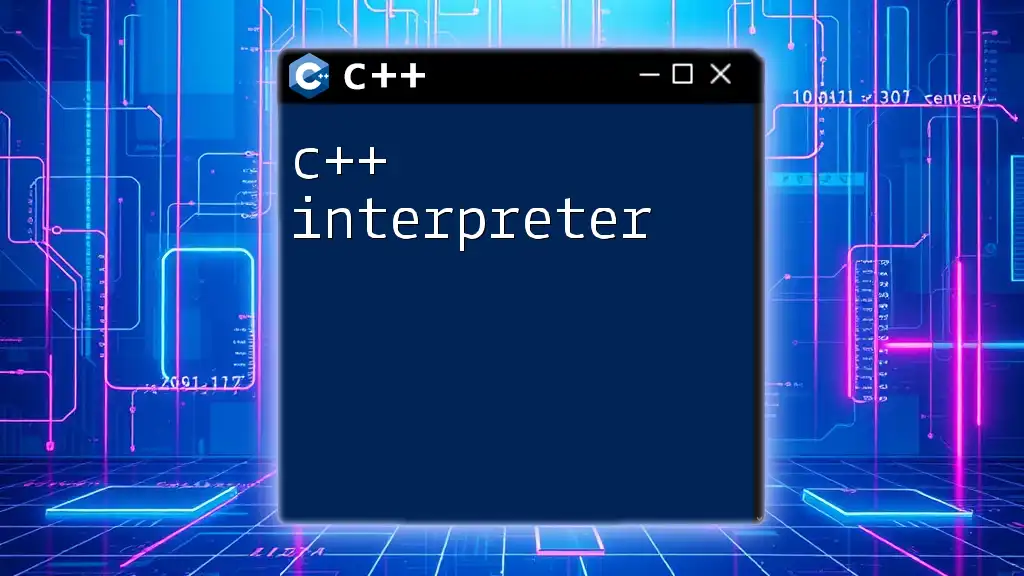
Resources for Further Learning
Online Tutorials and Documentation
For those interested in furthering their C++ in browser education, here are a few recommended resources:
- The official Emscripten documentation provides comprehensive guides and tutorials.
- Online platforms like Codecademy and Coursera offer courses focused on C++ and WebAssembly.
Community and Support Channels
Joining active communities can also provide valuable support; platforms like Stack Overflow, GitHub, and specific C++ forums can offer advice and solutions to common issues encountered during development.
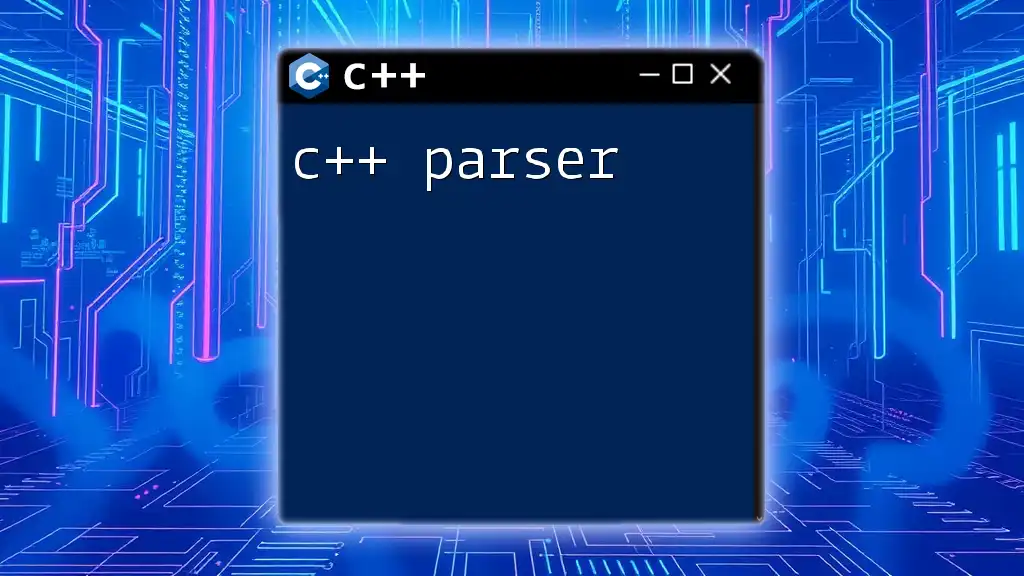
Conclusion
In conclusion, leveraging C++ in the browser opens up a world of possibilities for developers, allowing them to create fast, efficient web applications and games. With tools like Emscripten and the powerful capabilities of WebAssembly, the potential of C++ can now be harnessed for web development like never before.
As you embark on your journey into C++ for the web, don’t hesitate to explore, experiment, and engage with community resources. Start building remarkable applications and see the power of C++ come to life in a browser environment!
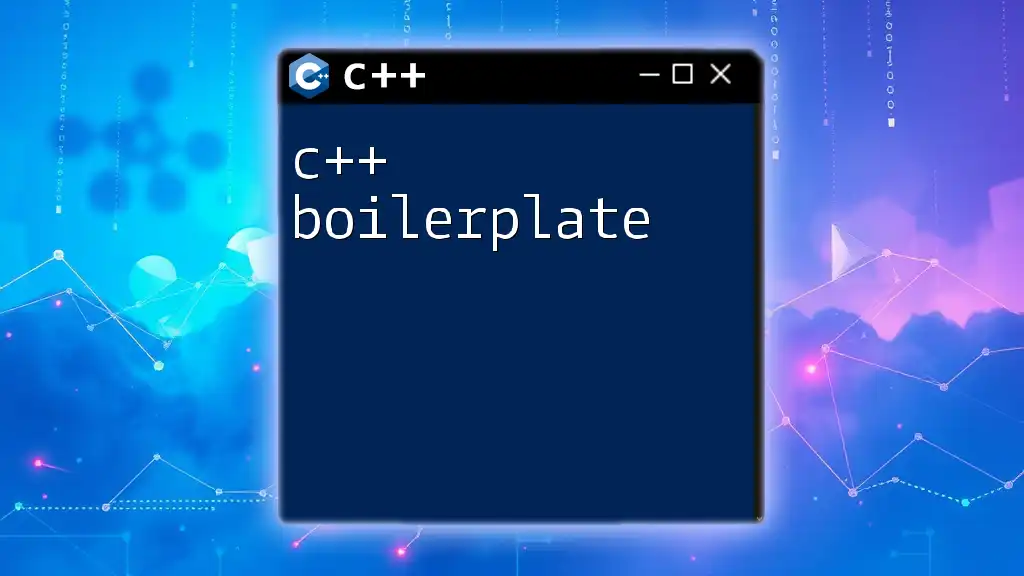
Call to Action
We invite you to subscribe to our newsletter for more insights into mastering C++, join a related course to deepen your understanding, or download our teaching resources to enhance your skillset. If you have any questions or seek further discussions about C++ and web development, feel free to reach out!