In C++, the `insert` function of the `std::list` class allows you to add elements at a specified position within the list.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3};
auto it = myList.begin();
myList.insert(it, 0); // Inserts 0 at the beginning
for (int value : myList) std::cout << value << " "; // Outputs: 0 1 2 3
return 0;
}
Understanding C++ Lists
What is a List in C++?
A list in C++ is a sequence container that allows non-contiguous memory allocation, providing dynamic size management. Unlike vectors, which store elements contiguously, lists store their elements as nodes. This results in better performance for insertions and deletions as these operations do not require the shifting of elements, which is necessary in vector structures.
Using lists can be advantageous when you anticipate frequent insertions and deletions. This data structure excels in scenarios where maintaining a sorted sequence of elements is critical, or when you require frequent updates to your dataset.
How to Include the List Library
Before using lists in your C++ program, ensure that you include the necessary header file at the beginning of your code:
#include <list>
This inclusion grants access to the extensive functionalities that the list container provides.
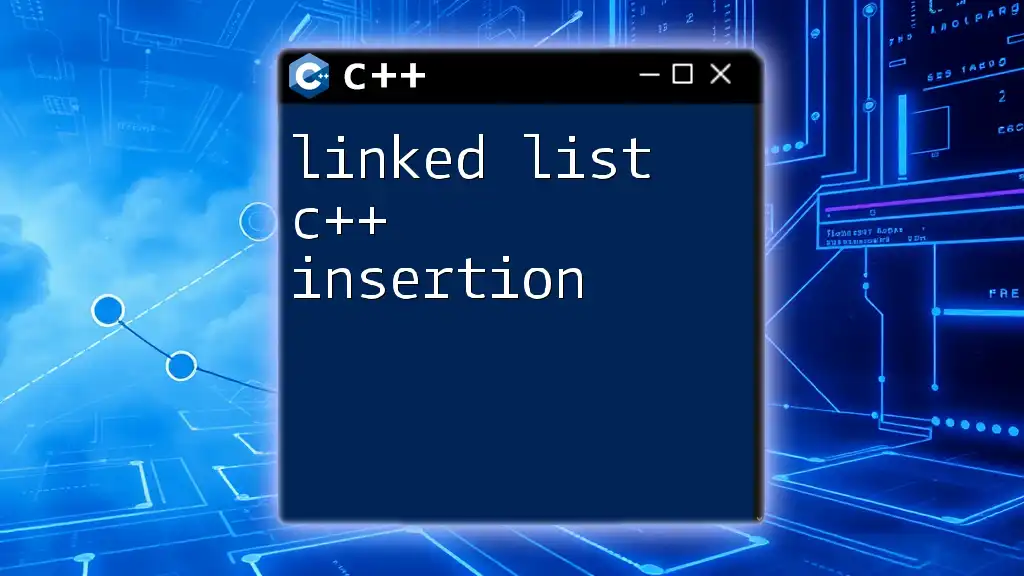
The Insert Function in C++
Overview of the List Insert Function
The `insert()` function plays a crucial role in modifying a C++ list. It allows you to insert elements into the list at specified positions. Understanding the syntax of the `insert()` function is vital:
list.insert(position, element);
Here:
- position: This parameter is an iterator that indicates the location where the new element will be inserted.
- element: This parameter represents the value that is to be inserted.
Parameters of the Insert Function
Besides the basic insert operation, `insert()` can take advantage of optional parameters:
- count: This specifies the number of times to insert the element.
- first and last: These iterators define a range of elements to be copied and inserted.
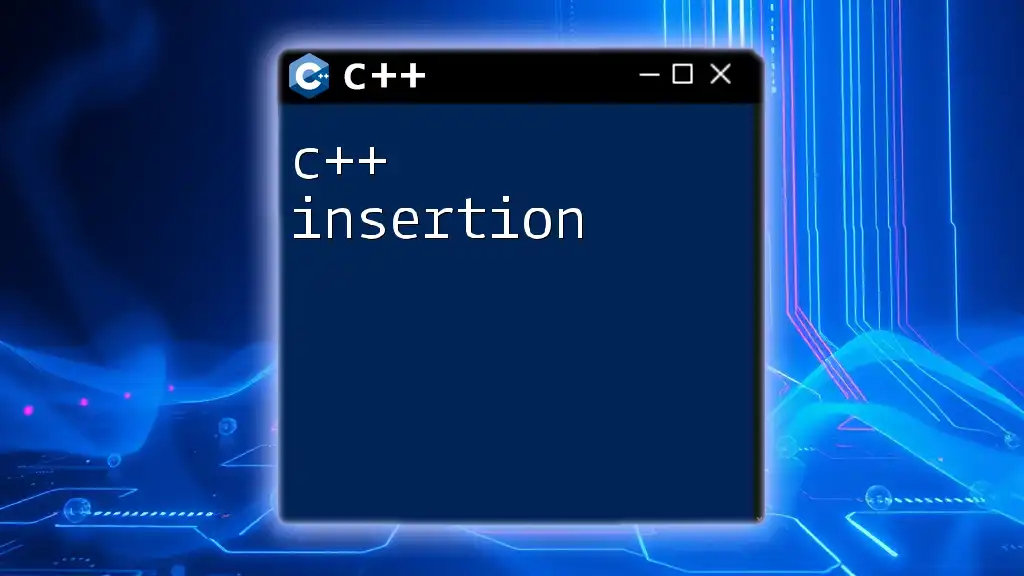
Using List Insert in C++
Basic Insert Operations
Inserting a Single Element
Inserting a single element into a list is straightforward. Here's a quick example:
std::list<int> myList;
myList.push_back(10);
myList.insert(myList.begin(), 5); // Inserting at the beginning
In this example, we create a list `myList`, add an element `10` to the back, and then insert `5` at the beginning of the list. The final content of `myList` will be `5, 10`.
Inserting Multiple Elements
You can also insert multiple copies of an element using `insert()`. Here’s how:
std::list<int> myList = {1, 2, 3};
myList.insert(myList.end(), 3, 0); // Inserting three zeros at the end
In the above code, we start with a list containing `1, 2, 3` and insert three `0`s at the end. The list would now look like `1, 2, 3, 0, 0, 0`.
Inserting Elements from Another List
You can seamlessly insert elements from another list into your list. Here’s an example:
std::list<int> sourceList = {4, 5, 6};
myList.insert(myList.end(), sourceList.begin(), sourceList.end());
Here, all elements from `sourceList` are inserted at the end of `myList`. If `myList` initially contains `1, 2, 3, 0, 0, 0`, the final result will be `1, 2, 3, 0, 0, 0, 4, 5, 6`.
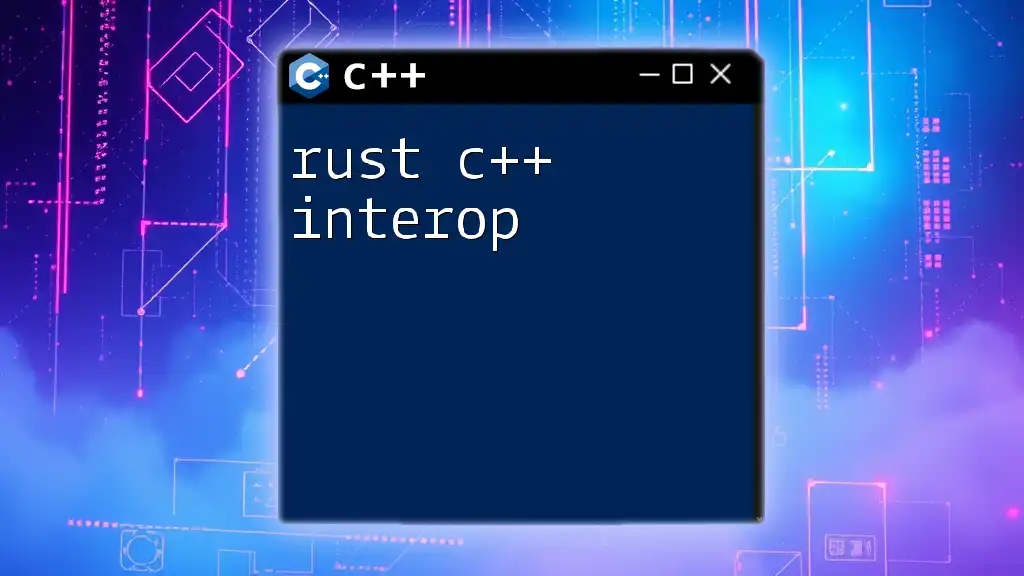
Advanced Usage of List Insert
Inserting an Element at a Specified Position
Inserting an element at a specific position involves understanding iterators. For example:
std::list<int> myList = {1, 2, 3, 4};
auto it = myList.begin();
std::advance(it, 2); // Move iterator to the third position
myList.insert(it, 10); // Insert 10 before position 3
In this snippet, we advance the iterator `it` to the third position of `myList` and insert `10` there. The list's new contents become `1, 2, 10, 3, 4`, demonstrating how iterators can effectively navigate and manipulate list structures.
Performance Considerations for Insertions
It is important to note the time complexity associated with insertions. Inserting an element in a list can typically be performed in constant time, O(1), if the position is known. This is a major advantage over array-like structures such as vectors, where insertions can take O(n) time because of the need to shift elements.
Conversely, if repeatedly inserting at random positions, consider the performance implications carefully, as the iterator must traverse parts of the list, impacting efficiency. Strategically choosing where and when to insert can yield significant performance benefits, especially in larger datasets.
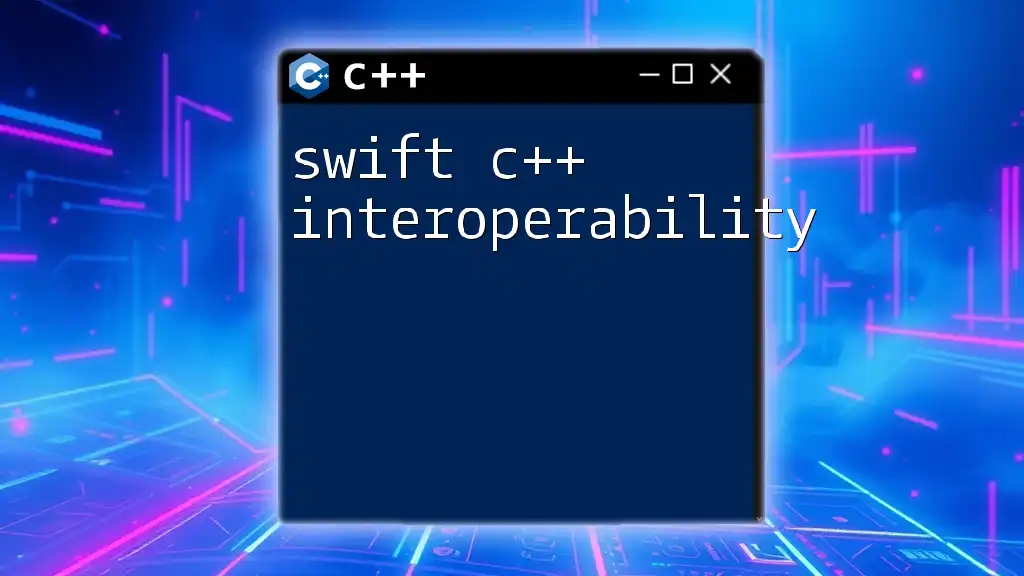
Common Mistakes and Best Practices
Common Mistakes When Using Insert
One common mistake developers make is forgetting to include the list header file. Another frequent error is attempting to insert at positions beyond the current boundaries of the list without proper iterator management, leading to runtime errors.
Best Practices for Using Insert in C++
To ensure smooth operations when using `insert()`, always check list boundaries. If you use iterators for positional insertions, it can lead to errors if the iterator is invalid or points to an inappropriate location.
Another best practice to consider is to prefer `emplace()` over `insert()` when you need to insert elements in-place. `emplace()` constructs the element in the location directly rather than inserting a copy, often resulting in better performance.
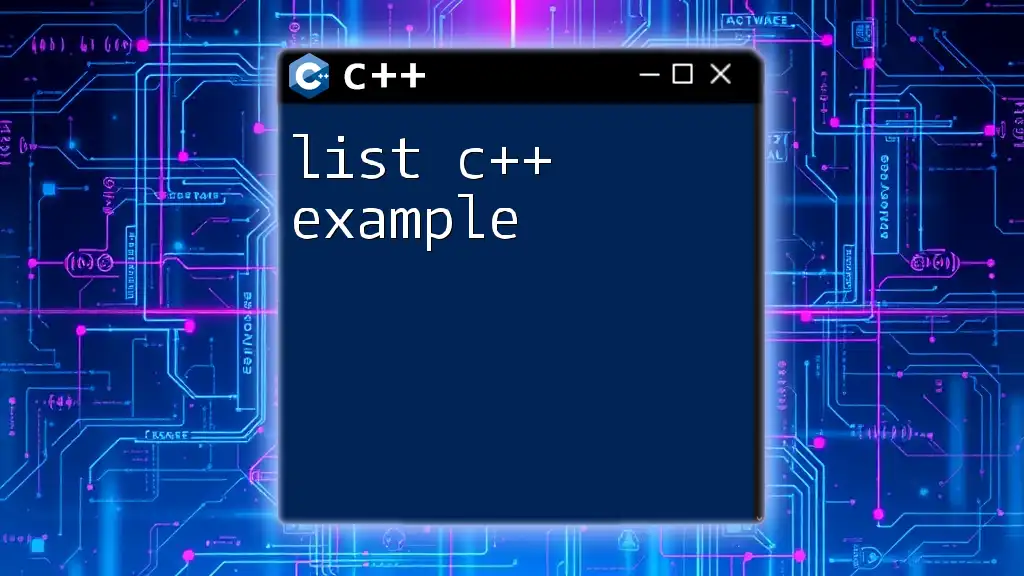
Conclusion
This guide has provided a comprehensive overview of the list C++ insert functionality. From inserting single and multiple elements to advanced techniques involving iterators and performance considerations, understanding how to manipulate lists in C++ can enhance your coding efficiency significantly.
We encourage you to practice and explore further with examples and exercises. If you have experiences or unique uses of the `insert` function, share your insights!