The `std::set` in C++ is a container that stores unique elements in a specific order, providing fast retrieval and automatic sorting.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {3, 1, 2}; // Elements are inserted and sorted automatically
for (int num : mySet) {
std::cout << num << " "; // Output: 1 2 3
}
return 0;
}
Understanding std::set
What is a Set?
A set in programming is a data structure that embodies a collection of distinct elements, ensuring that each element is unique. Unlike arrays or vectors, where duplicates are permissible, a set intrinsically forbids repeated entries. This property makes sets particularly advantageous in situations where ensuring uniqueness is critical, such as filtering out duplicate items from a dataset.
The Role of std::set in C++
In the realm of C++, `std::set` is a pivotal component of the Standard Template Library (STL). It is designed to store data in a sorted manner, using a binary tree structure (typically a red-black tree) under the hood. One of the key advantages of `std::set` is its automatic sorting—elements are stored in sorted order based on their values, which simplifies searching and removes the need for manual sorting.
By utilizing `std::set`, programmers can implement algorithms that require efficient lookups, insertions, and deletions due to its logarithmic time complexity for these operations. It outshines other containers, such as vectors, when it comes to maintaining order and uniqueness.
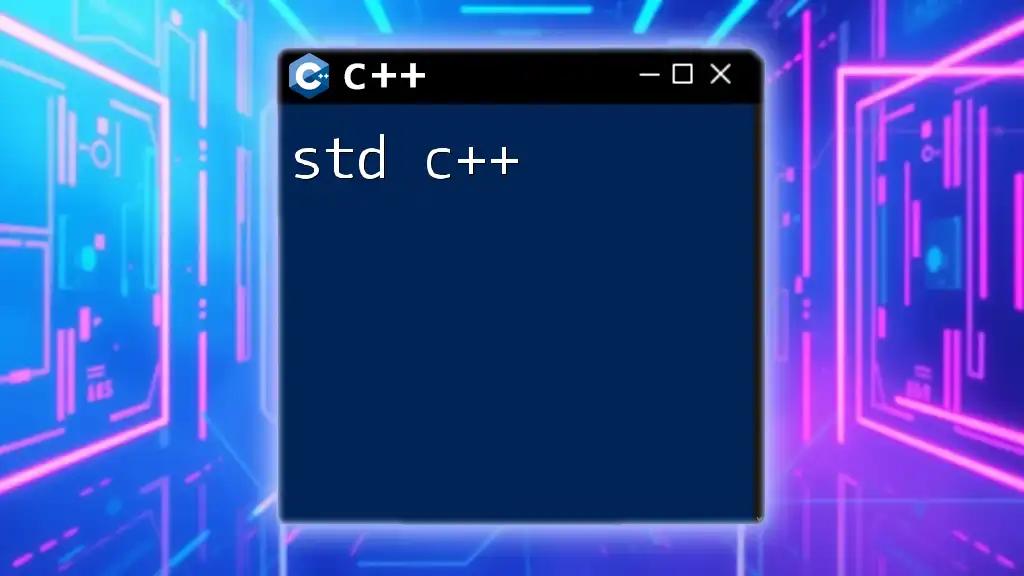
Key Features of std::set
Automatic Sorting
A defining characteristic of `std::set` is its ability to automatically sort elements upon insertion. When you add an item to a set, it finds the appropriate position in the underlying structure to maintain this order. This feature makes it an excellent choice for scenarios where data needs to be frequently accessed in a sorted manner without additional overhead.
Unique Elements
The set inherently enforces uniqueness. If you attempt to insert a duplicate item, the set will ignore this insertion silently. This behavior is useful in situations where duplicates could lead to erroneous data processing or analytics. For example, you can observe the following code snippet:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet;
mySet.insert(1);
mySet.insert(2);
mySet.insert(2); // This will not be added
for (const auto& elem : mySet) {
std::cout << elem << " "; // Output: 1 2
}
}
In this example, the number 2 is only stored once, confirming the set's emphasis on uniqueness.

Declaring and Initializing std::set
Basic Declaration
To declare a set in C++, use the following syntax, specifying the type of elements it will hold:
std::set<int> mySet;
This line creates an empty set that can only accommodate integer values.
Initializing with Values
You can also initialize a set with predefined values using an initializer list, which saves time and makes your code cleaner. Consider the example:
std::set<int> mySet = {1, 2, 3, 4, 5};
This initializes `mySet` with the integers provided, maintaining automatic sorting and uniqueness.

Commonly Used Operations
Inserting Elements
To add elements to a `std::set`, you utilize the `insert()` method. This method not only adds an element but also ensures that the set stays sorted. For example:
mySet.insert(6);
If `6` is not already present, it will be included in the set.
Finding Elements
Finding an element within a set can be accomplished using the `find()` method. This method returns an iterator pointing to the element if found, or `end()` if the element is absent. Here's an example:
auto it = mySet.find(3);
if (it != mySet.end()) {
std::cout << "Found 3";
}
This code snippet demonstrates how to check for the existence of an integer in the set.
Erasing Elements
The `erase()` function lets you delete specific elements from a set. If the specified item is in the set, it will be removed. Check out this example:
mySet.erase(2);
After running this line, the number `2` will no longer exist in the `mySet`.
Iterating Over a Set
You can traverse a set using iterators or range-based for loops. Here’s how you can do it:
for (auto it = mySet.begin(); it != mySet.end(); ++it) {
std::cout << *it << " ";
}
Alternatively, a more modern approach leverages range-based for loops:
for (const auto& elem : mySet) {
std::cout << elem << " ";
}
Both snippets allow you to access each element in the set sequentially.

Advanced Features
Custom Sorting with Comparators
While `std::set` uses the default sorting method (less than operator), you can customize the sorting criteria by implementing a comparator. For instance:
struct CustomComparator {
bool operator()(int a, int b) const {
return a > b; // Descending order
}
};
std::set<int, CustomComparator> customSet;
In this example, `customSet` will sort integers in descending order instead.
Set Operations
Operating on sets allows for powerful manipulations, including:
- Union: The union of two sets produces another set containing all unique elements present in either set.
std::set<int> set1 = {1, 2, 3};
std::set<int> set2 = {3, 4, 5};
std::set<int> resultSet;
std::set_union(set1.begin(), set1.end(), set2.begin(), set2.end(), std::inserter(resultSet, resultSet.begin()));
- Intersection: This operation returns another set with elements present in both sets.
std::set<int> intersectionSet;
std::set_intersection(set1.begin(), set1.end(), set2.begin(), set2.end(), std::inserter(intersectionSet, intersectionSet.begin()));
- Difference: The difference of two sets results in a new set containing elements that are in the first set but not in the second.
std::set<int> differenceSet;
std::set_difference(set1.begin(), set1.end(), set2.begin(), set2.end(), std::inserter(differenceSet, differenceSet.begin()));

Real-World Applications
Use Cases for std::set
`std::set` finds application in numerous real-world scenarios. Its primary use cases include:
-
Data Deduplication: Efficiently storing unique items, such as customer IDs or transaction numbers.
-
Sorted Data Management: Maintaining ordered data for applications that frequently require sorted lists, such as leaderboard systems or score tracking.
-
Efficient Searching: Quick lookups in applications like spell-checkers or game state management where element uniqueness is crucial.

Best Practices
When to Use std::set
Employ `std::set` when you require:
- Uniqueness: When elements must remain distinct.
- Automatic Sorting: If you require ordered collections and need quick lookups or insertion.
- Efficiency: In cases involving frequent insertions and deletions compared to alternative data structures.
Performance Considerations
When evaluating the efficiency of `std::set`, remember that its lookup, insertion, and deletion operate at logarithmic complexity O(log n). For use cases requiring constant-time complexity, consider alternatives like `std::unordered_set`, which, although quicker for lookups, do not maintain order and do not enforce uniqueness in the same manner.
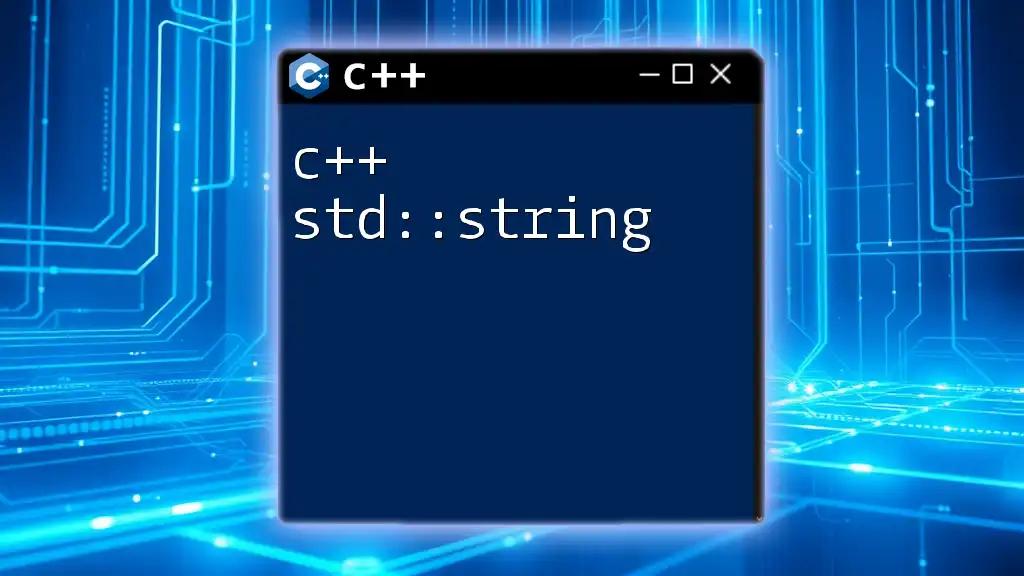
Conclusion
The `std::set` in C++ is a powerful tool for managing collections of unique elements in a sorted manner. By understanding its features, operations, and suitable use cases, programmers can harness the full potential of this container to improve code efficiency and maintain data integrity. Leveraging `std::set` appropriately can make a significant difference in applications requiring rigorous data handling and analysis.