The `cin` object in C++ is used to take input from the standard input (usually the keyboard) and can be used to read strings directly with the `>>` operator.
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a string: ";
std::cin >> input;
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding `cin` and C++ Strings
What is `cin`?
`cin`, short for "character input," is a standard input stream in C++ that allows for reading data from the standard input device, typically the keyboard. It is crucial for interactive applications where user input is necessary.
Why use `cin`? One of the main advantages of using `cin` is its ability to read formatted data, allowing you to quickly capture user inputs in conjunction with prompts. It can handle various data types, including integers, floating-point numbers, and strings, making it a versatile tool for developers.
What is a C++ String?
In C++, a string is an object of the `std::string` class, part of the C++ Standard Library. Unlike C-style strings that are essentially arrays of characters, C++ strings manage memory automatically and provide several built-in functions for manipulation.
Why use strings in C++? The advantages of using C++ strings include:
- Automatic memory management
- Built-in functions for string manipulation (like concatenation, comparison, and searching)
- Enhanced safety against common string manipulation errors
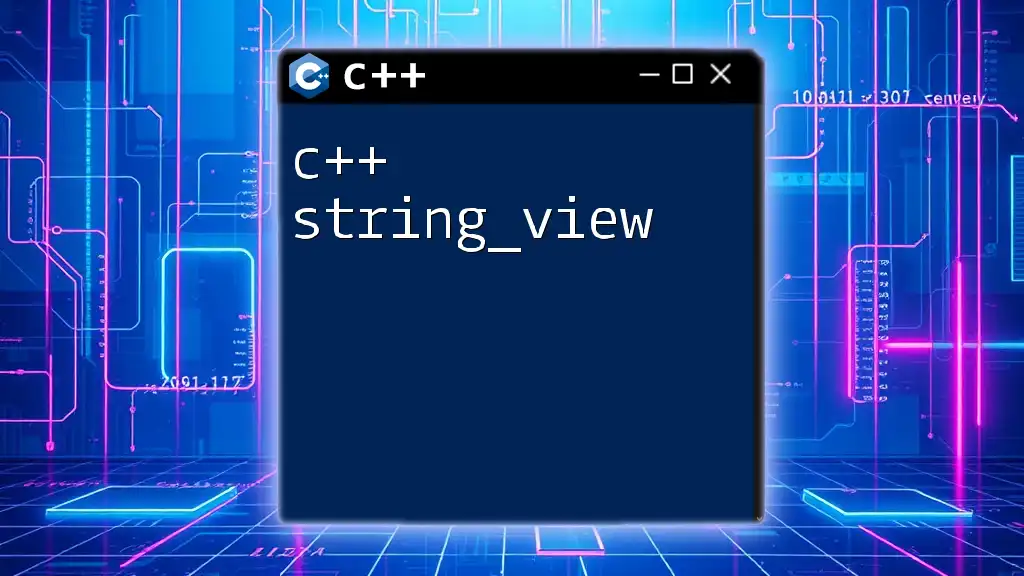
How to Use `cin` with C++ Strings
Basic Usage of `cin` with Strings
To input a string using `cin`, the syntax is straightforward. Here’s a simple example:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin >> name;
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
Explanation of the Code:
- `#include <iostream>` and `#include <string>` include the necessary headers for input/output and string manipulation.
- We declare a string variable `name`.
- The program prompts the user to enter their name. `cin` reads the input and stores it in the `name` variable, allowing for direct output.
Inputting Full Lines using `cin`
While `cin` suffices for single-word input, it struggles with full lines, particularly when spaces are involved. Instead, we can utilize the `getline` function to capture full lines of input effectively.
Here’s an example using `getline`:
#include <iostream>
#include <string>
int main() {
std::string fullName;
std::cout << "Enter your full name: ";
std::getline(std::cin, fullName);
std::cout << "Hello, " << fullName << "!" << std::endl;
return 0;
}
The primary difference is that `getline` reads the entire line until the newline character, allowing users to input names with spaces.
Comparison between `cin` and `getline`:
- Use `cin` for individual words or tokens and `getline` for sentences or phrases that include spaces.
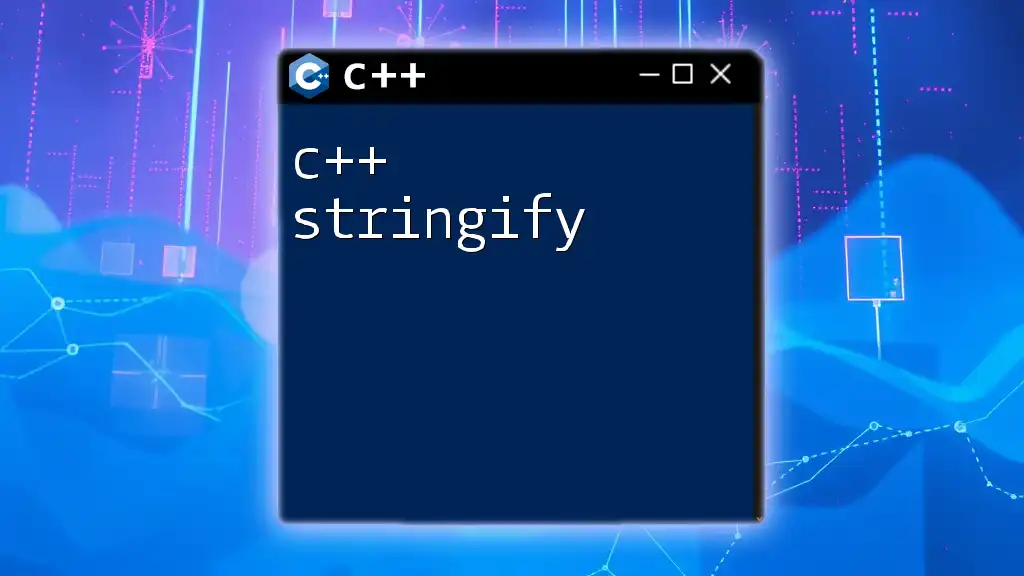
Common Issues and Solutions
Handling Whitespace in Input
One major issue with `cin` arises when users attempt to input multiple words separated by spaces. `cin` reads only the first word and truncates the rest.
Solution: Using `getline` resolves this problem, as it reads the entire line correctly, including spaces. Always prefer `getline` when you expect to receive full names or phrases.
Clearing the Input Buffer
Sometimes, after using `cin`, you may find it necessary to clear the input buffer, especially when expecting further input that could be affected by erroneous input or leftover characters.
How to clear the input buffer: You can accomplish this by using the following code snippet:
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
This line discards characters in the input buffer, ensuring that subsequent `cin` calls work as expected.
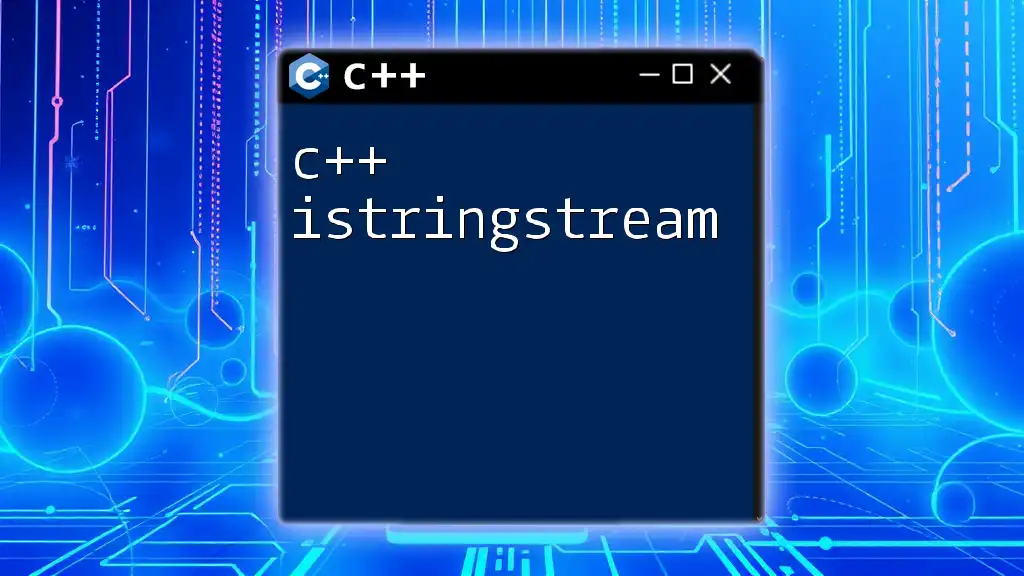
Advanced Concepts
String Manipulation after Input
Once you have your input stored as a string, you can easily manipulate it. Here are some basic string operations available in C++:
- Concatenation: This combines two or more strings into one.
- Length: Get the total number of characters in a string using `.length()` or `.size()`.
- Substring: Extract a part of a string using `.substr()`.
Here’s an example that demonstrates string manipulation:
std::string firstName = "John";
std::string lastName = "Doe";
std::string fullName = firstName + " " + lastName;
std::cout << "Full Name: " << fullName << std::endl;
This code combines `firstName` and `lastName` and prints the full name.
Using `cin` in Functions
Another great feature of C++ is the ability to use functions to encapsulate repetitive tasks. You can pass strings gathered via `cin` to functions seamlessly.
Here’s a function that greets a user based on their input:
void greetUser(const std::string& userName) {
std::cout << "Welcome, " << userName << "!" << std::endl;
}
int main() {
std::string name;
std::cin >> name;
greetUser(name);
return 0;
}
In this example, `greetUser` takes a string parameter and outputs a welcome message.
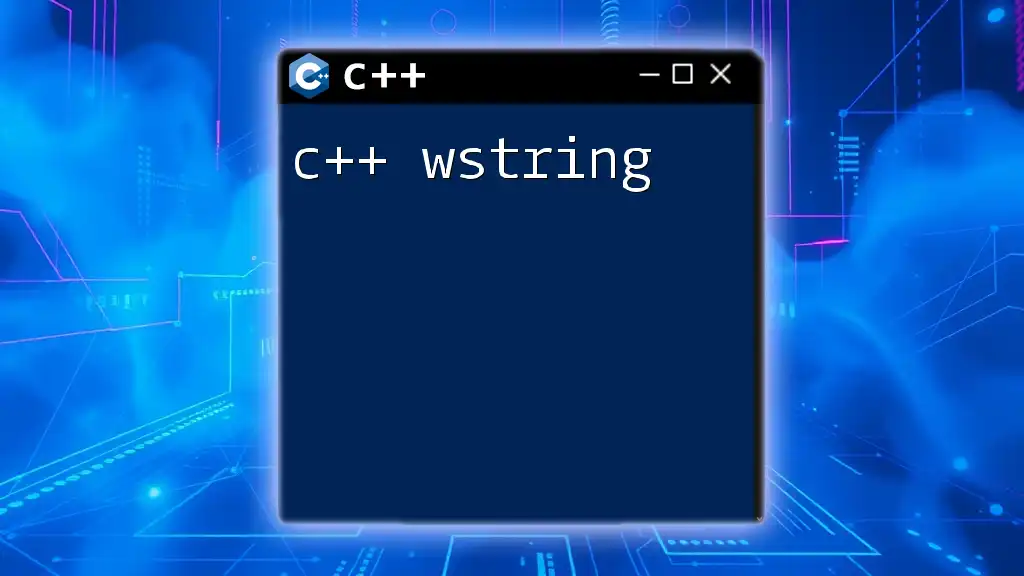
Best Practices for Using `cin` with Strings
Tips for Effective Input Handling
- Use `getline()` for Full Input: Always default to `getline()` when expecting multi-word strings or full sentences.
- Always Validate User Input: Check if the input meets expected criteria (e.g., ensuring no special characters in names).
- Clear the Input Buffer When Necessary: Ensure you manage the input buffer to avoid unexpected behavior in your program.
Common Mistakes to Avoid
- Overlooking Input Limits: Be mindful of the maximum size when reading strings to avoid buffer overflows or truncation.
- Not Clearing Input Buffers: Forgetting to clear the input buffer may lead to unintended consequences on subsequent `cin` calls.
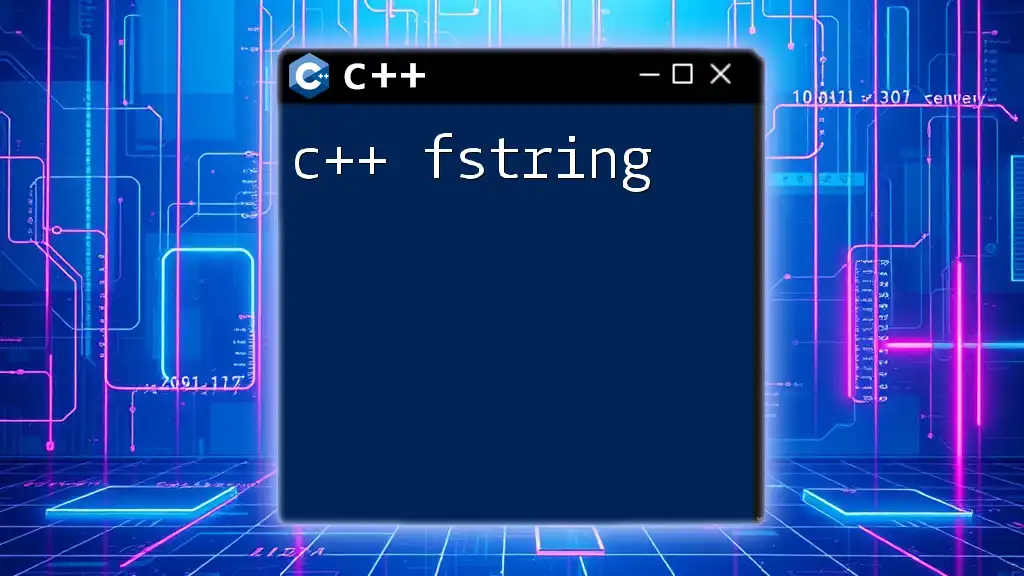
Conclusion
Mastering `cin` with C++ strings is essential for any developer who wishes to create interactive applications. Understanding the differences between `cin` and `getline`, along with how to handle input effectively, will significantly enhance your programming skills.
Take the time to experiment with the examples provided, and practice input validations and string manipulations to solidify your understanding of `cin c++ string`. Happy coding!