In C++, "std" refers to the standard namespace that contains all the functionalities of the C++ Standard Library, allowing developers to use common features without prefixing them with "std::".
Here's an example of using the `std::cout` command to print a message:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Does std Stand For in C++?
In C++, std stands for Standard. It is part of the std namespace, which houses all the standard functions and classes provided by the C++ Standard Library. The use of the namespace allows developers to avoid naming conflicts, especially in larger projects where multiple libraries might be utilized.
The Origin of std
The std namespace originated with the Standard Template Library (STL), which was introduced as part of the C++98 standard. The STL provides a powerful collection of algorithms and data structures, making C++ a more versatile and user-friendly language.

The Significance of the std Namespace
Why Use std in C++?
Using std serves several purposes:
- Avoid Naming Conflicts: When variable names or functions conflict, the std namespace helps segment and clarify which elements belong to which library.
- Code Readability: When using standard library elements, it becomes easier to identify the source of functions and objects, enhancing overall code readability.
Common std Implementations
The std namespace includes a plethora of libraries that cater to various programming needs. Here’s a brief overview of frequently used libraries:
- I/O Functionalities: The `iostream` library for input and output operations.
- Collections: Standard containers like `vector`, `list`, and `map` facilitate data management.
- Algorithms: Libraries providing essential algorithms such as `sort`, `find`, and `reverse`.
Example Code Snippet: Basic I/O with std
To illustrate how to utilize `std`, consider the following example showcasing basic input and output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `std::cout` uses the standard output stream defined within the std namespace.

How to Use std in Your Code
Syntax for Using std
To use elements from the std namespace, there are two main approaches:
- Always Prefix with std: This method involves prefixing each standard library function or object with `std::`. It's clear and prevents conflicts.
- Using the 'using' Keyword: By declaring `using namespace std;`, you permit direct access to standard library names without the std prefix.
What is std:: and When to Use It?
Using `std::` has the advantage of highlighting that a particular function originates from the standard library. This practice promotes clarity, especially in larger files which may involve numerous custom libraries.
Example Code: Using vs. Not Using std
To demonstrate both methods, here’s a simple program utilizing `cout`:
Using `std::`:
#include <iostream>
int main() {
std::cout << "Using std:: prefix" << std::endl;
return 0;
}
Using `using namespace std;`:
#include <iostream>
using namespace std;
int main() {
cout << "Using namespace std" << endl;
return 0;
}
While the second approach improves convenience, it may pose risks of name collisions, especially in libraries or larger codebases.

The Broader Context of std in C++
Evolution of std in C++ Standards
The std namespace has evolved significantly from C++98 to C++20, with several new features and functionalities introduced. Each update enhances existing libraries or introduces new paradigms, such as lambda expressions and smart pointers, broadening the scope of what can be achieved with standard tools.
Importance of std in C++ Programming Culture
The adherence to the std namespace is vital within the C++ community. Developers strive to use standard library components to ensure code portability and maintainability across various platforms. The integration of std further encourages best practices among C++ developers, fostering a culture of innovation and proper usage.

Best Practices When Using std
When to Avoid std Namespace
While using `using namespace std;` can simplify code, it is advisable to refrain from this practice in larger projects. Potential naming conflicts may hinder code clarity and maintainability. Being explicit about where a function or type originates contributes to a clearer understanding, especially when working in teams.
Implications for Performance
From a performance perspective, there is generally negligible overhead associated with using std. However, being mindful of namespace usage can lead to better structured and optimized code, particularly in large applications where clarity and maintainability translate to improved performance over time.
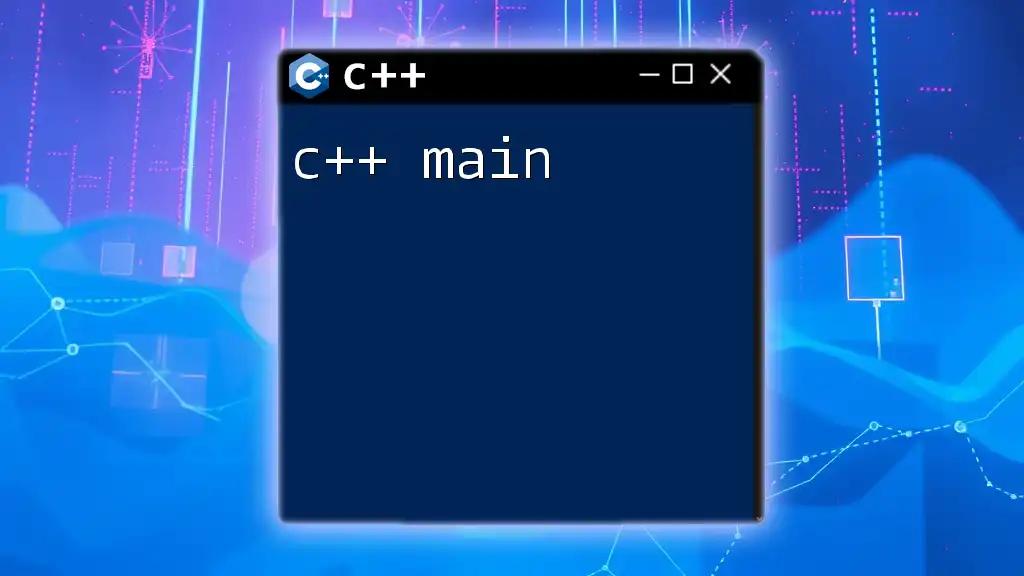
Conclusion
In summary, the std c++ meaning extends beyond mere terminology; it encapsulates a powerful framework that facilitates effective programming practices. Recognizing the significance of the std namespace, its historical context, and its importance in the broader programming culture empowers developers to leverage this invaluable resource in crafting efficient and maintainable C++ applications.

Additional Resources
For those eager to delve deeper, consider exploring C++ tutorials, official documentation, and engaging with C++ programming communities. Practicing the use of the std namespace in your projects will solidify your understanding and improve your proficiency in C++.
FAQs About std in C++
Some frequently asked questions regarding std in C++ include:
- What does std mean in C++?: It signifies the Standard namespace for the C++ Standard Library.
- Can I avoid using std altogether?: It’s possible, but not advisable, as you would miss out on the robust features provided by the standard library.
- Is it safe to use `using namespace std;`?: Use it with caution, preferably in smaller projects, to avoid naming conflicts in larger codebases.
By embracing the std namespace, you not only streamline your programming practice but also align with the principles of C++ as a modern, effective programming language.