Code blocks in C++ are a way to group statements together, usually enclosed in curly braces `{}`, allowing for controlled scope and execution within functions, loops, or conditional statements.
Here's a simple example of a code block in C++:
#include <iostream>
int main() {
// This is a code block
{
int x = 5;
std::cout << "The value of x is: " << x << std::endl;
}
return 0;
}
Understanding Code Blocks
What is a Code Block?
A code block is a group of statements or declarations that are enclosed within curly braces `{}`. In C++, code blocks play a crucial role in defining scopes, managing variable lifetimes, and organizing code.
The syntax for a simple code block is straightforward:
{
// This is a code block
}
The significance of code blocks becomes evident when considering the scope of variables. Variables declared within a code block are only accessible within that block, helping to prevent naming conflicts and reducing errors.
Structure of a Code Block
Basic Syntax
As mentioned, code blocks are defined by the presence of opening `{` and closing `}` braces. This structure creates a scope where variables can be declared and used without affecting the global context. For example:
{
int x = 10;
std::cout << x; // Output will be 10
}
Variables declared here, like `x`, cannot be accessed outside the braces, which ensures that they do not interfere with other parts of your program.
Nested Code Blocks
Nested code blocks refer to code blocks within code blocks. This encapsulation allows for improved organization and better management of variable scopes. Consider the following example:
{
int x = 10;
{
int y = 5;
std::cout << x + y; // Accessing variables from the outer block will output 15
}
// std::cout << y; // Error: y is not accessible here
}
The ability to create nested structures enhances clarity in your code. However, developers must be cautious with visibility and scope, as trying to access an inner variable from outside its block will result in an error.
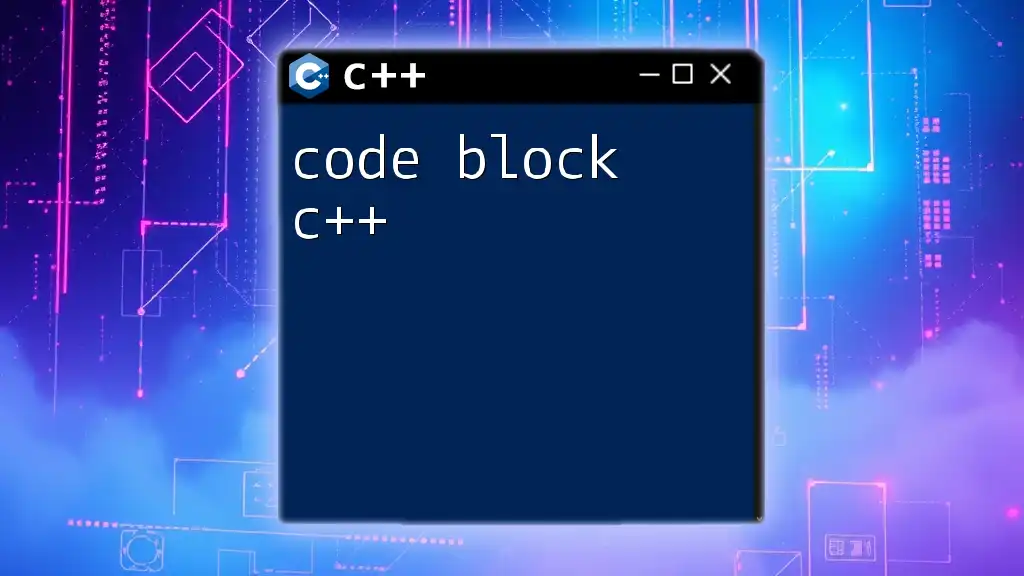
Types of Code Blocks in C++
Function Code Blocks
Every function in C++ operates within its own code block. This block encompasses all the statements that make up the function. Here’s a simple example:
void myFunction() {
// This is a function code block
std::cout << "Hello, World!";
}
In the above example, every time `myFunction()` is called, it executes the statements in the block. Variables declared inside the function cannot be accessed from outside, effectively isolating their scope.
Control Structure Code Blocks
If Statements
If statements provide a way to conditionally execute code blocks. Here is how you could structure an if statement:
if (condition) {
// This code block executes if the condition is true
std::cout << "Condition is true!";
}
This flexibility allows you to structure your code workflows based on specific conditions, making your programs dynamic and responsive.
Loops
Loops are another critical application of code blocks. They allow for repetitive execution of code based on a specified condition. Here is how code blocks function within various loops:
for (int i = 0; i < 5; i++) {
// Loop code block
std::cout << i; // This will print numbers from 0 to 4
}
In this example, the block within the for loop executes repeatedly until the condition `i < 5` is no longer satisfied.
Class Code Blocks
C++ classes also utilize code blocks to define their member functions and data. Each class has its own block that encapsulates all its properties and behaviors. Here’s an example:
class MyClass {
public:
void myMethod() {
// Method code block
std::cout << "Inside MyClass method!";
}
};
The use of code blocks within classes allows for organizing related functions and variables, promoting encapsulation and better code management.
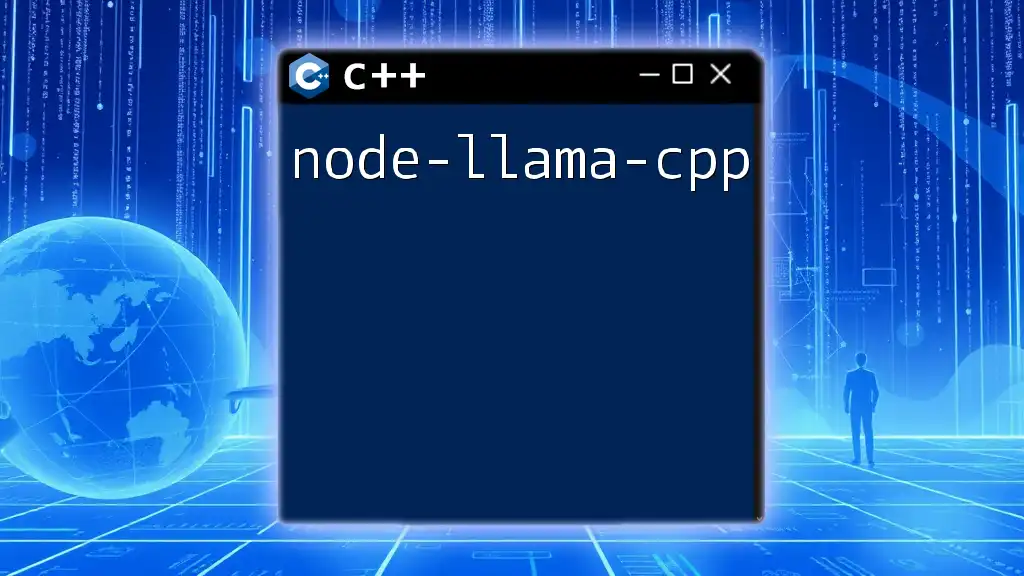
Best Practices for Using Code Blocks
Code Readability
Maintaining clear and consistent formatting within code blocks is vital for readability. Proper indentation and spacing can significantly improve how easily another programmer (or even you at a later date) can understand your code. For instance:
if (condition) {
// Properly indented code block
std::cout << "Condition is true!";
} else {
// An alternative code block
std::cout << "Condition is false!";
}
Minimizing Scope
To reduce potential errors, limit the scope of your variables. By declaring variables in the smallest possible code block, you minimize the chances of conflicts with other variables. For example:
{
int x = 5;
{
int y = 10;
std::cout << x + y; // Safe to access only within this nested block
}
}
// std::cout << y; // Error: y cannot be accessed here
Code Blocks in Multithreading
When utilizing code blocks in a multithreaded environment, it is crucial to consider thread safety. Proper synchronization methods must be applied to prevent multiple threads from interfering with each other when accessing shared data.
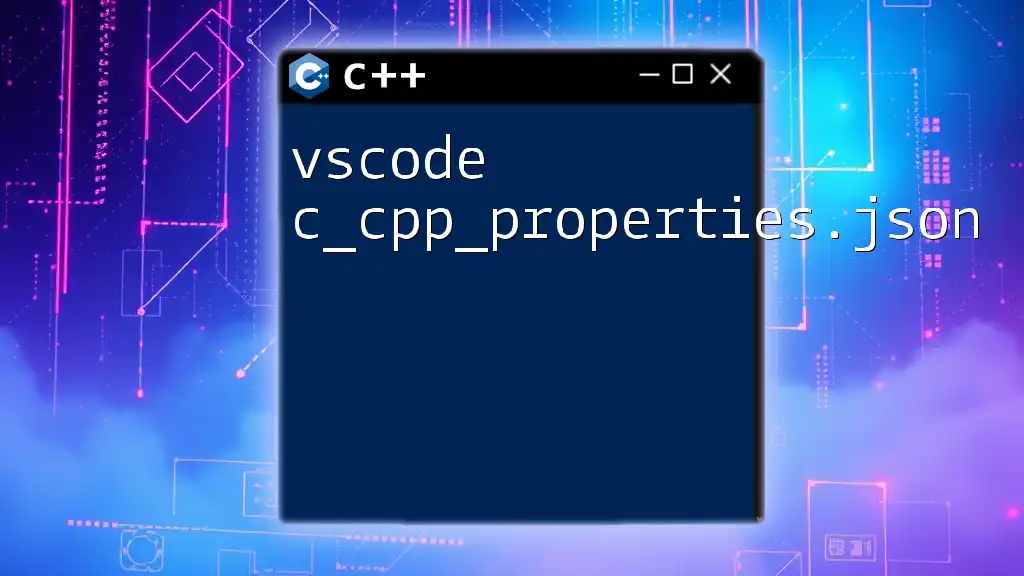
Error Handling within Code Blocks
Exceptions
Error handling is an essential aspect of any robust application. In C++, you can use try-catch blocks for managing exceptions:
try {
// Code block that may throw an exception
throw std::runtime_error("An error occurred");
} catch (const std::exception &e) {
// Handle exception
std::cout << e.what();
}
This structure allows you to manage errors gracefully, ensuring that your program can handle unexpected situations without crashing.
Returning Values
When functions exit, they often need to return values, which are determined by the code blocks within them. Here's how you might structure a function returning a value:
int add(int a, int b) {
return a + b; // Returning the result of the addition
}
In this case, the code block inside `add` determines what value is returned based on the computations completed within it.
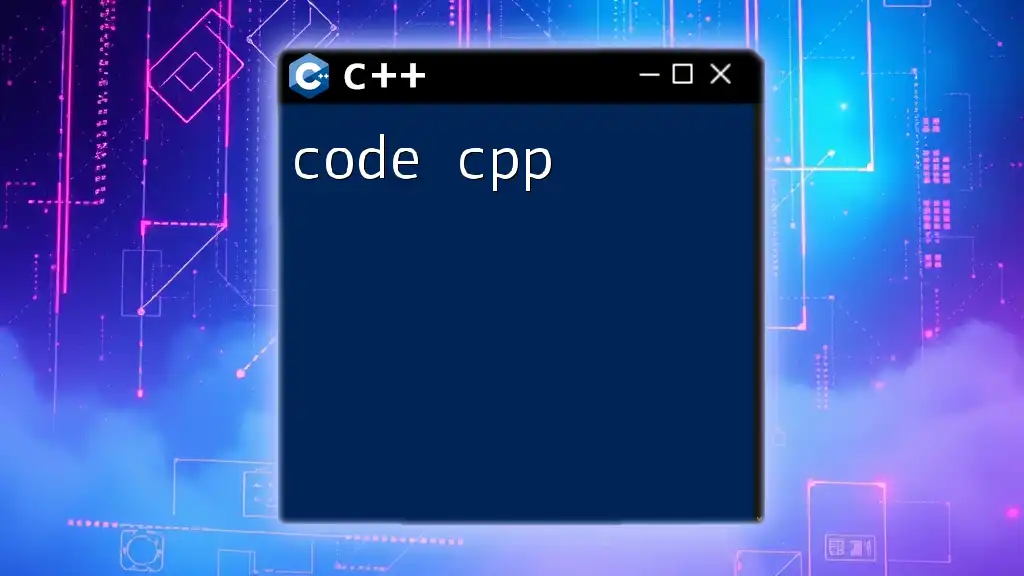
Conclusion
Code blocks in C++ are fundamental for defining scope, managing visibility, and organizing your code. Effective use of code blocks enhances readability, minimizes errors, and supports numerous programming structures. By mastering code blocks, you will significantly improve your coding skills and your ability to create efficient, maintainable software applications.
Engaging with various coding challenges and projects will further deepen your understanding. Embrace the use of code blocks in every C++ program you write and watch how your proficiency grows. For additional resources, consider exploring official C++ documentation, community forums, and online tutorials to further sharpen your skills in this versatile programming language.