The `c_cpp_properties.json` file in Visual Studio Code is used to configure the IntelliSense engine for C++ projects, specifying include paths, defines, and the C++ standard version.
{
"configurations": [
{
"name": "Win32",
"includePath": [
"${workspaceFolder}/**",
"C:/path/to/your/include/files"
],
"defines": ["_DEBUG", "UNICODE", "_UNICODE"],
"compilerPath": "C:/path/to/your/compiler/g++.exe",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "gcc-x64"
}
],
"version": 4
}
Understanding `c_cpp_properties.json`
What is `c_cpp_properties.json`?
The `vscode c_cpp_properties.json` file is a critical component of your C++ development environment when using Visual Studio Code (VSCode). It serves as a configuration file that helps manage the settings required for the C/C++ extension by Microsoft. This powerful extension offers features such as IntelliSense, code navigation, and code formatting, all of which rely on the settings defined in this file.
Key Components of `c_cpp_properties.json`
The file consists of several crucial sections, including `configurations`, `version`, and `includePath`. Understanding these components is essential for effective configuration.
- Configuraions: This section is where you define different settings for diverse build environments. You can specify options for various platforms or compilers here.
- Version: This indicates the version of the configuration format. It helps the extension understand how to interpret the settings correctly.
- IncludePath: This is one of the most important fields. It tells the IntelliSense engine where to find header files for your project. Without proper paths, IntelliSense may not function correctly.
Example Structure of `c_cpp_properties.json`
Understanding the typical structure can help you create your own configurations. Here’s an example of a basic `c_cpp_properties.json` file:
{
"configurations": [
{
"name": "Win32",
"includePath": [
"${workspaceFolder}/**",
"C:/path/to/headers"
],
"defines": [],
"compilerPath": "C:/MinGW/bin/g++.exe",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "gcc-x64"
}
],
"version": 4
}
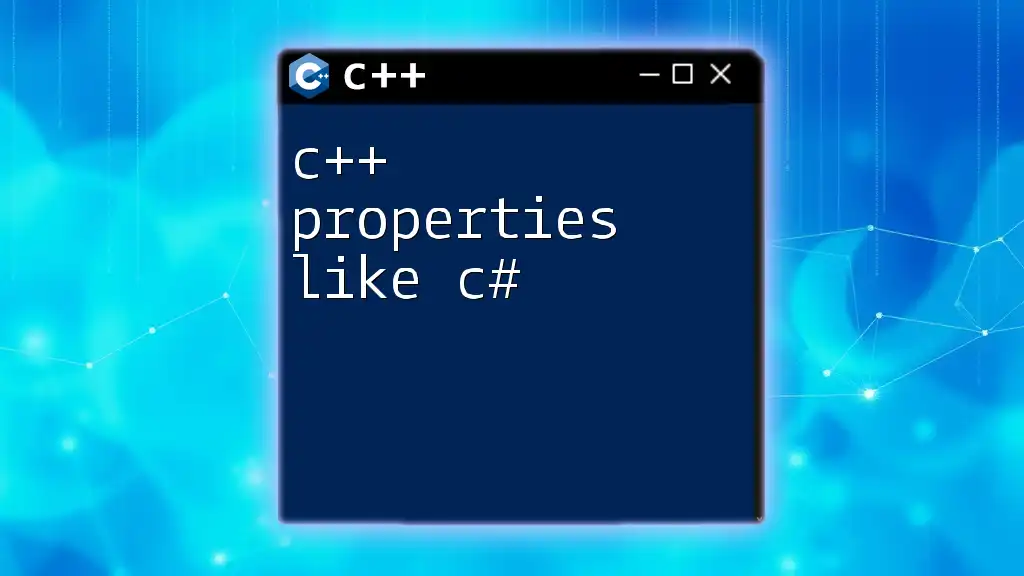
Configuring Include Paths
What are Include Paths?
In C/C++, include paths provide the compiler and IntelliSense engine with locations to look for header files. Proper configuration of these paths is essential for successful builds and accurate code completion and error reporting during development.
Setting Up Include Paths in `c_cpp_properties.json`
To set include paths in your `vscode c_cpp_properties.json`, modify the `includePath` field. You can specify individual paths or use wildcards to make sure all relevant folders are included. This flexibility helps accommodate various project structures.
Example of Adding Include Paths
Here’s how you might configure include paths:
"includePath": [
"${workspaceFolder}/**",
"/usr/include/**",
"C:/SomeLibrary/include"
]
This setup allows the IntelliSense engine to search through all subdirectories of your workspace and the specified library paths for header files.
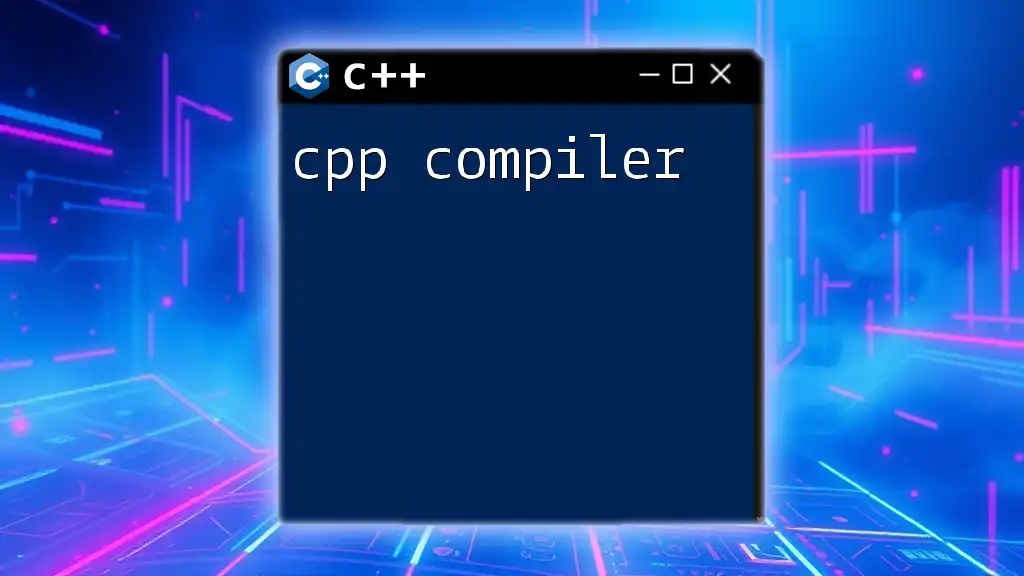
Defining Compiler Options
Importance of Compiler Options
Compiler options significantly influence the behavior of your build process and IntelliSense experience. Specifying the correct compiler path and standards ensures compatibility with different codebases and libraries.
Configuring Compiler Path
The `compilerPath` field in the `c_cpp_properties.json` tells VSCode which compiler to use for IntelliSense. This setting is important for both Windows and Linux users.
Example of Setting Compiler Path
To specify a compiler, your configuration may look like this:
"compilerPath": "C:/Path/To/GCC/g++.exe"
Setting C and C++ Standards
The fields `cStandard` and `cppStandard` allow you to specify which versions of the C and C++ languages your code adheres to. This is particularly useful when your codebase requires specific features or syntax found in newer versions of the languages.
Example of C++ Standard Configuration
Here’s an example of how to set it:
"cppStandard": "c++17"
This example indicates that the project will utilize features from the C++17 standard.
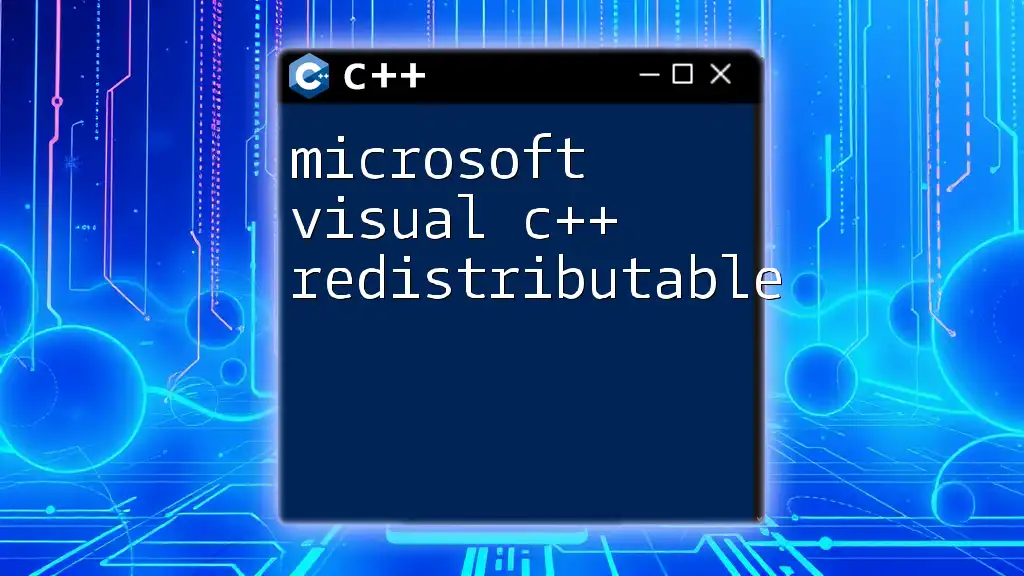
IntelliSense Configuration
What is IntelliSense?
IntelliSense is a key feature of the C/C++ extension in VSCode that provides automatic code completion, parameter info, quick info, and member lists. This feature enhances productivity and code quality by reducing errors and speeding up the coding process.
Configuring IntelliSense Modes
The `intelliSenseMode` determines how IntelliSense will behave based on the compiler and platform. Correctly configuring it ensures that you get the most relevant suggestions and diagnostics.
Examples of IntelliSense Modes
Common IntelliSense modes include:
- `gcc-x64` for GCC on 64-bit Linux
- `clang-x64` for Clang on 64-bit systems
- `msvc-x64` for Microsoft Visual C++ on 64-bit Windows
Configuring the mode might look like this:
"intelliSenseMode": "gcc-x64"
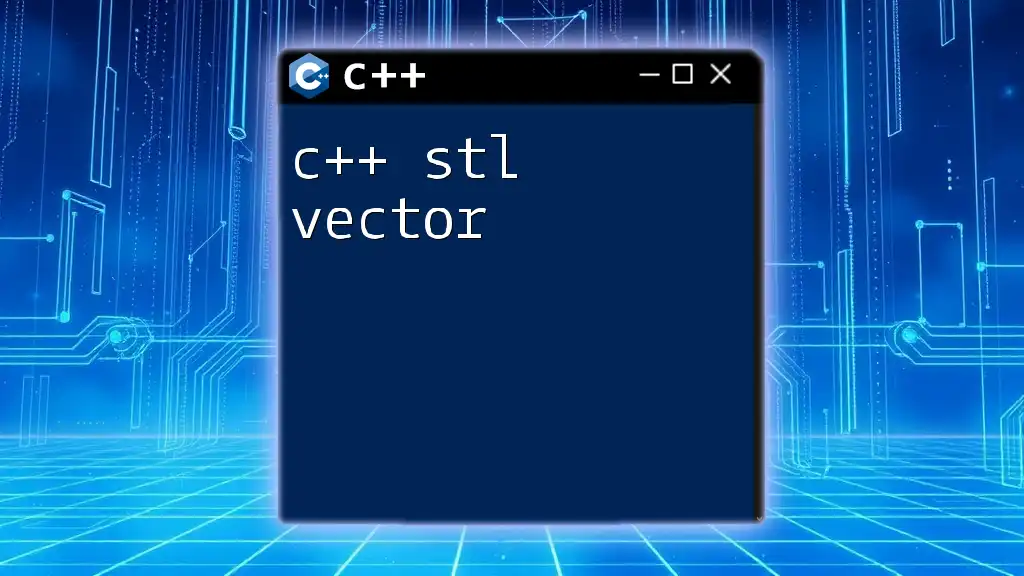
Advanced Configuration
Using Defines
The `defines` field allows you to specify preprocessor directives that the compiler can use during the build process. This is particularly useful for defining macros that might be essential for different parts of your code.
Example of Adding Defines
For example, to define `_DEBUG` and `UNICODE`, you could set it up like so:
"defines": [
"_DEBUG",
"UNICODE"
]
Managing Multiple Configurations
For larger projects, managing multiple configurations becomes vital. You can set up different configurations for various platforms, enabling you to switch quickly between them without modifying the file each time.
Example of Multiple Configurations
Here’s how you might structure your configurations:
"configurations": [
{
"name": "Win32",
// Windows-specific settings
},
{
"name": "Linux",
// Linux-specific settings
}
]
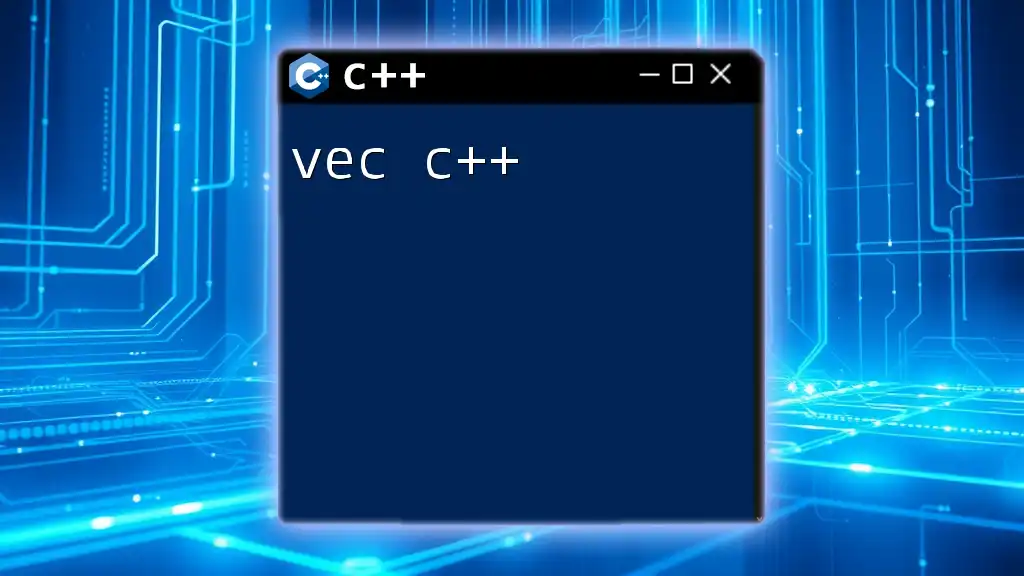
Troubleshooting Common Issues
IntelliSense Not Working
If you encounter issues with IntelliSense, it could be due to incorrect paths in your `c_cpp_properties.json`. Double-check the `includePath` and ensure that all critical directories are included.
Build Errors Related to Configuration
Incorrect configurations can lead to build failures. Ensure that your `compilerPath`, `defines`, and standards are compatible with your available libraries and source files.
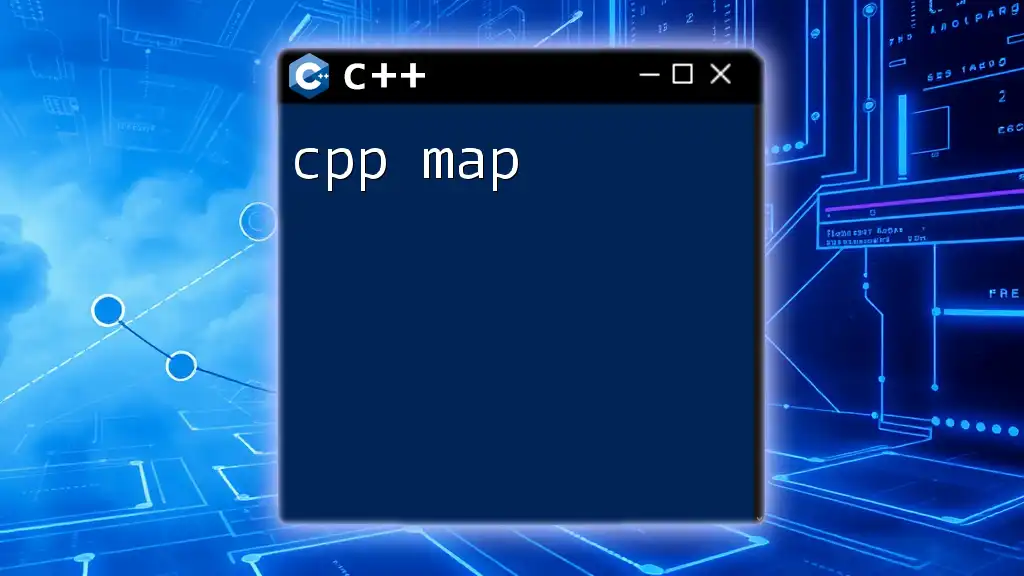
Conclusion
The `vscode c_cpp_properties.json` file is a powerful tool for managing your C++ development environment in Visual Studio Code. By properly configuring the various sections, you can enhance your development experience significantly. Whether you are new to C++ or a seasoned developer, understanding and optimizing this configuration file can lead to more efficient coding practices and smoother development workflows.
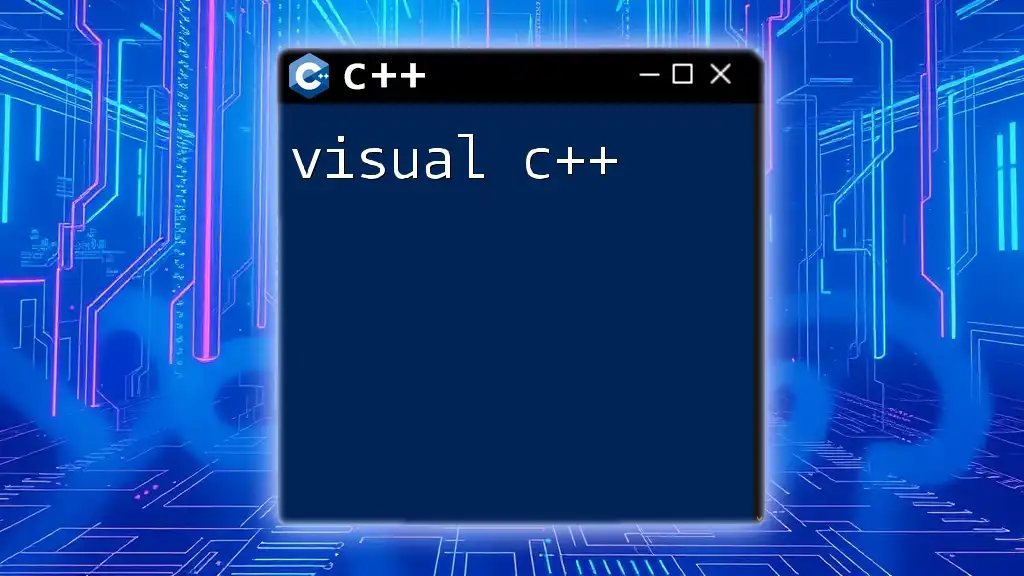
Additional Resources
For further reading and more in-depth understanding, refer to the official [C++ extension documentation](https://code.visualstudio.com/docs/cpp/cpp-ide) and explore additional tools and resources that could enhance your C++ development setup.