"Concur CPP" refers to the use of concurrency features in C++, allowing for concurrent execution of tasks to enhance performance and responsiveness in applications.
Here’s a simple example using C++11's `<thread>` library to create two threads that run concurrently:
#include <iostream>
#include <thread>
void task1() {
std::cout << "Task 1 is running.\n";
}
void task2() {
std::cout << "Task 2 is running.\n";
}
int main() {
std::thread t1(task1);
std::thread t2(task2);
t1.join();
t2.join();
return 0;
}
What is `concur` in C++?
The `concur` library represents a modern approach to concurrency in C++. Unlike traditional threading models, `concur` provides an abstraction layer that simplifies the management of concurrent tasks. This allows developers to focus on the logic of their applications rather than the complexities of thread management. With `concur cpp`, tasks are treated as first-class citizens, providing a powerful way to handle multiple tasks running simultaneously without the intricacies associated with direct thread manipulation.
Utilizing `concur` offers several advantages:
- Readability: The syntax is designed to make concurrent code easier to read and maintain.
- Safety: It helps reduce common concurrency issues such as race conditions and deadlocks.
- Efficiency: `concur` manages resource allocation effectively, improving performance on multicore processors.
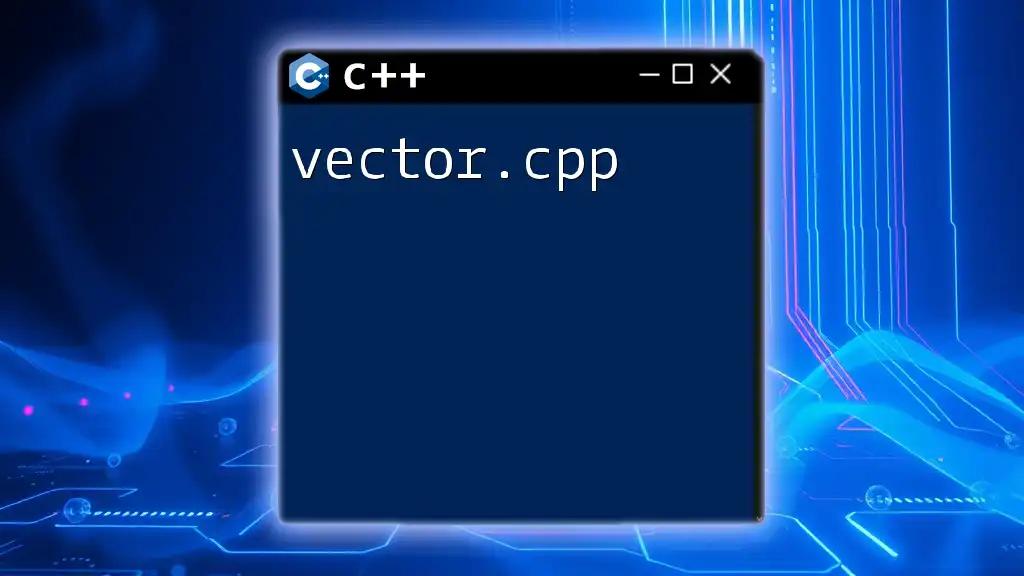
Getting Started with `concur`
Installing `concur`
To begin using `concur`, you need to install the library. Here’s how you can do it:
-
Package Manager: If you are using a package manager like `vcpkg` or `Conan`, you can install `concur` easily. For instance, with `vcpkg`, you can run:
vcpkg install concur
-
Direct Download: Alternatively, you can download the library from its official repository. Be sure to follow the instructions in the README for integrating it into your build system.
Before starting, ensure that your C++ environment is set up and compatible with the library, typically C++17 or higher is recommended for `concur`.
Basic Syntax and Structure
The construction of concurrency in `concur cpp` is user-friendly. At its core, you use the `run` function to define and execute tasks. Here's a simple example of a `concur` invocation:
#include <concur.h>
void helloWorld() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
concur::run(helloWorld);
return 0;
}
In this example, when the program runs, it calls the `helloWorld` function asynchronously, allowing for non-blocking execution.
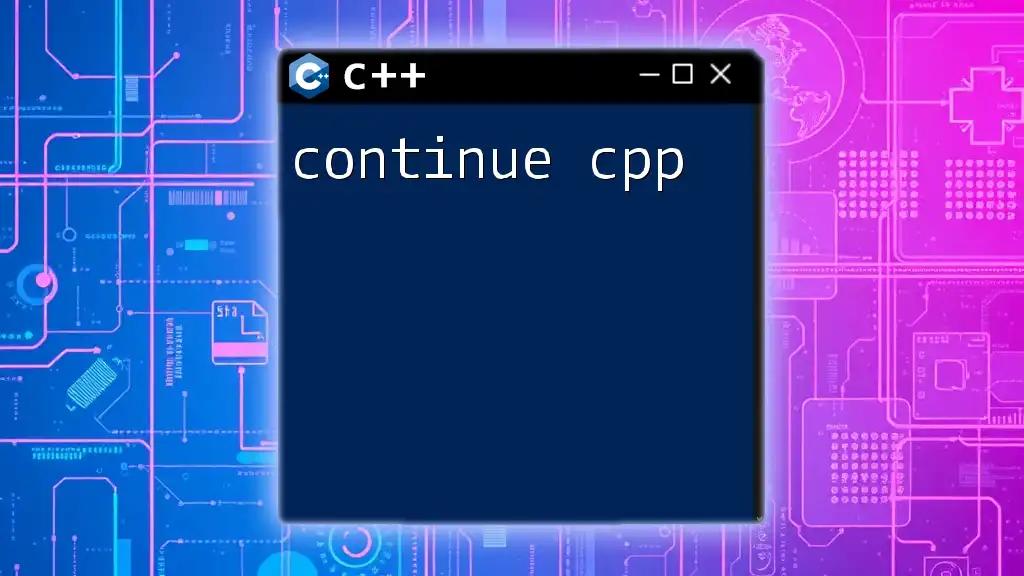
Understanding Tasks and Threads
Defining a Task
In `concur`, a task is a unit of work that can run concurrently. Tasks can be defined with lower overhead compared to traditional threads, allowing for rapid creation and destruction. This is especially useful for I/O-bound operations or when you need to execute many small tasks.
Here's an example of defining a task:
#include <concur.h>
void myTask() {
std::cout << "Executing my task." << std::endl;
}
int main() {
auto task = concur::task(myTask);
task.run();
return 0;
}
In this scenario, the `myTask` function is wrapped in a `task` object, which is then executed, demonstrating how easy it is to create concurrent tasks.
Managing Thread Lifecycle
Managing the lifecycle of tasks is crucial in concurrent programming. `concur` takes care of scheduling, allowing you to focus on the core functionality. For example, to pause and resume tasks, you can set conditions directly within your task logic. This contributes significantly to responsiveness in applications requiring real-time interactions.
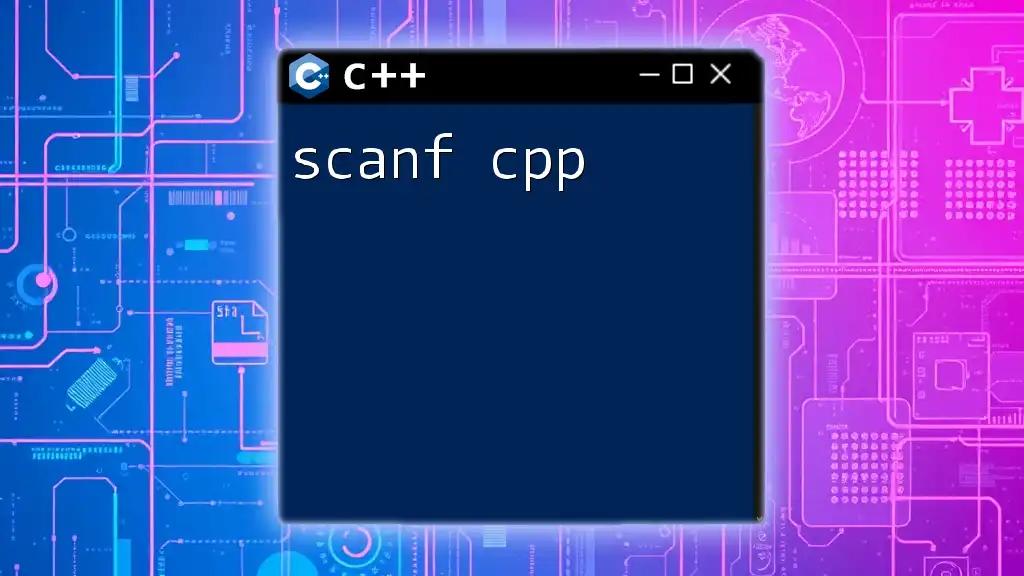
Synchronization in `concur`
Essential Synchronization Techniques
When multiple tasks operate on shared resources, synchronization becomes vital to avoid conflicts. `concur` provides built-in synchronization tools which prevent data races and ensure thread safety.
Using Locks in `concur`
Using locks to protect shared data is a common need in concurrency. The `concur` library incorporates mechanisms that allow easy implementation of locks. For example, consider this code snippet demonstrating how to safely increment a shared variable:
#include <concur.h>
#include <mutex>
std::mutex mtx;
void safeIncrement(int &counter) {
std::lock_guard<std::mutex> lock(mtx);
++counter;
}
int main() {
int counter = 0;
concur::run([&]() { safeIncrement(counter); });
return 0;
}
Here, the `std::lock_guard` ensures that the `mtx` mutex is locked during the execution of `safeIncrement`, preventing concurrent modifications to `counter`.

Advanced Features of `concur`
Futures and Promises
Futures and promises offer a powerful way to handle asynchronous results, enabling you to write efficient and responsive applications. In `concur`, you can easily create a promise and retrieve its value later with a future.
Error Handling in Concurrent Tasks
Concurrency introduces unique challenges, especially regarding error handling. Unlike single-threaded applications, exceptions thrown from one task may not propagate correctly to others. It is important to manage error handling carefully in `concur`.
For instance, you can wrap task execution in a try-catch block to gracefully handle any exceptions:
concur::run([]() {
try {
// Task that might throw an exception
throw std::runtime_error("An error occurred.");
} catch (const std::exception &e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
});
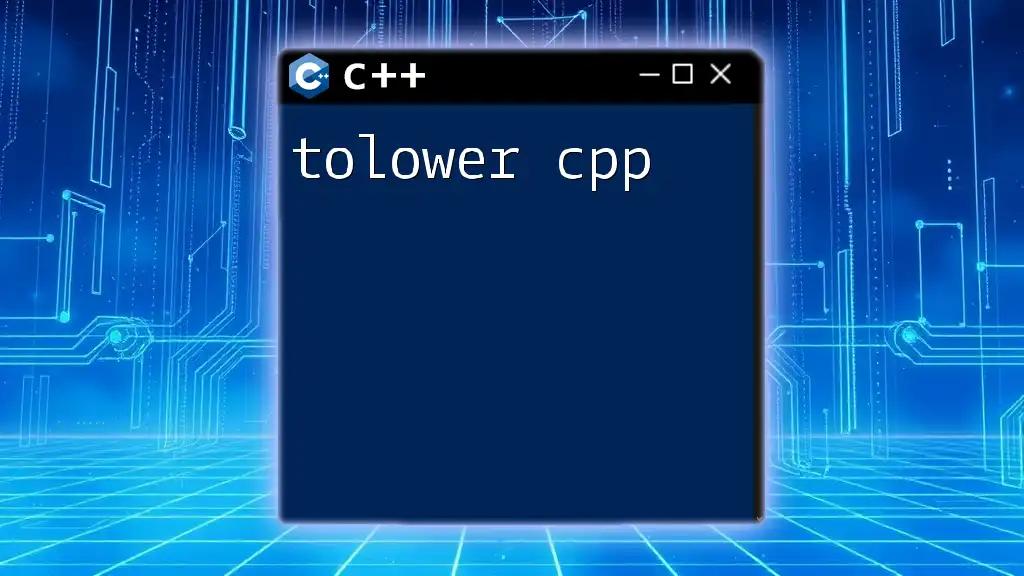
Performance Considerations
Measuring Efficiency
Performance measurement tools such as profilers can help identify bottlenecks in concurrent applications. Using `concur`, you should also be aware of thread contention and avoid unnecessary locking, which can slow down program execution.
Best Practices for Writing Concurrent Code
To make your concurrent code efficient and maintainable, consider the following best practices:
- Minimize shared state wherever possible to reduce synchronization overhead.
- Use higher-level abstractions like tasks instead of managing threads directly.
- Always handle exceptions thoughtfully to prevent unintended crashes.

Conclusion
In summary, `concur cpp` allows C++ developers to harness the power of concurrency in a straightforward and efficient manner. With its task-based model, synchronization tools, and easy integration, `concur` is an invaluable library for building modern applications that require responsiveness and performance. By practicing these tools and techniques, you can enhance your C++ skills and develop applications that fully utilize contemporary multicore architectures.

Additional Resources
For more in-depth knowledge, consider checking out the official `concur` documentation, exploring recommended books, and participating in community forums dedicated to C++ concurrency discussions. Engaging with the community can further bolster your understanding and expertise in this critical area of modern software development.