"JSON for Modern C++ is a popular library that facilitates easy parsing and serialization of JSON data in C++ applications."
Here’s a simple example of how to parse a JSON string using the library:
#include <nlohmann/json.hpp>
#include <iostream>
int main() {
// Example JSON string
std::string jsonString = R"({"name": "Alice", "age": 30})";
// Parse the JSON string
nlohmann::json jsonData = nlohmann::json::parse(jsonString);
// Access data
std::cout << "Name: " << jsonData["name"] << ", Age: " << jsonData["age"] << std::endl;
return 0;
}
Overview of json-cpp
What is json-cpp?
json-cpp is an open-source C++ library used for parsing and serializing JSON data. It provides a simple API that enables C++ developers to work seamlessly with JSON. As JSON has become a common format for data interchange in web services and APIs, having an efficient library like json-cpp enhances productivity in C++ applications.
Installing json-cpp
To use json-cpp in your projects, you need to install it. You can do this via several methods:
-
Using package managers like `vcpkg`, `Conan`, or `Homebrew`:
- For example, using `vcpkg`:
vcpkg install jsoncpp
- For example, using `vcpkg`:
-
Alternatively, you can clone the repository from GitHub and build it manually:
git clone https://github.com/open-source-parsers/jsoncpp.git cd jsoncpp mkdir build cd build cmake .. make
Once installed, ensure that your project is configured to include json-cpp. Include the library in your C++ files:
#include <json/json.h>
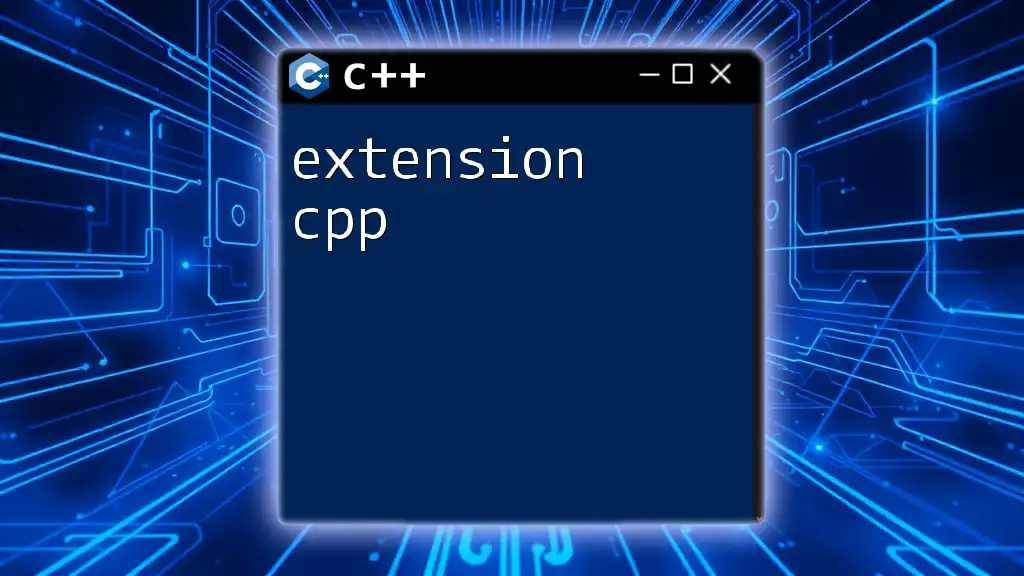
Getting Started with json-cpp
Basic Structure of a JSON Object in C++
Creating a JSON object is straightforward with json-cpp. Here’s how you can create a simple JSON object:
Json::Value jsonData;
jsonData["name"] = "John Doe";
jsonData["age"] = 30;
jsonData["city"] = "New York";
This snippet initializes a `Json::Value` object and adds some key-value pairs, which represent a basic JSON structure.
Reading JSON from a File
Reading JSON data from a file is a common task. Here's how to do it with json-cpp:
Json::Value jsonData;
Json::CharReaderBuilder readerBuilder;
std::ifstream jsonFile("data.json", std::ifstream::binary);
std::string errors;
if (!Json::parseFromStream(readerBuilder, jsonFile, &jsonData, &errors)) {
std::cout << "Failed to parse JSON: " << errors << std::endl;
}
In this code, we utilize a `CharReaderBuilder` to read from a file. The `parseFromStream` method handles the parsing and populates the `jsonData` variable.
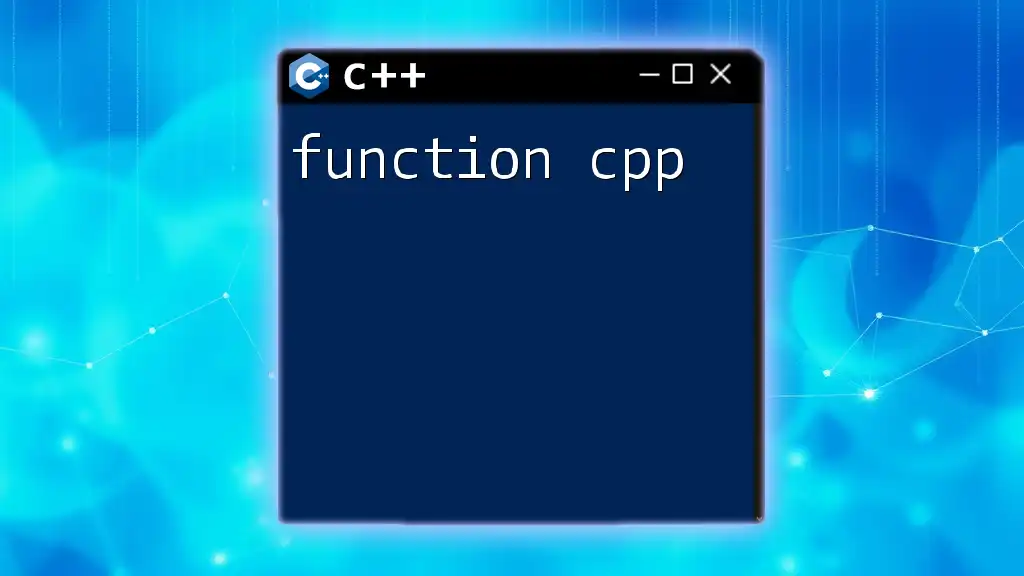
Manipulating JSON Data
Creating JSON Objects and Arrays
json-cpp allows you to create nested structures, which is essential for complex JSON data. Here’s how to create JSON objects and arrays:
Json::Value root;
root["employees"][0]["name"] = "Alice";
root["employees"][0]["role"] = "Engineer";
root["employees"][1]["name"] = "Bob";
root["employees"][1]["role"] = "Manager";
This example initializes a JSON object containing an array of employees.
Accessing JSON Values
Accessing values in a JSON object can be done easily with json-cpp. For instance:
std::string name = root["employees"][0]["name"].asString();
int age = root["employees"][0]["age"].asInt();
Here, we retrieve the name and age of the first employee. The `asString()` and `asInt()` methods convert JSON values to C++ types.
Modifying Existing JSON Data
You might find the need to modify existing JSON data. This can be achieved by simply reassigning values:
root["employees"][0]["role"] = "Senior Engineer";
This line updates Alice's role in the JSON structure.
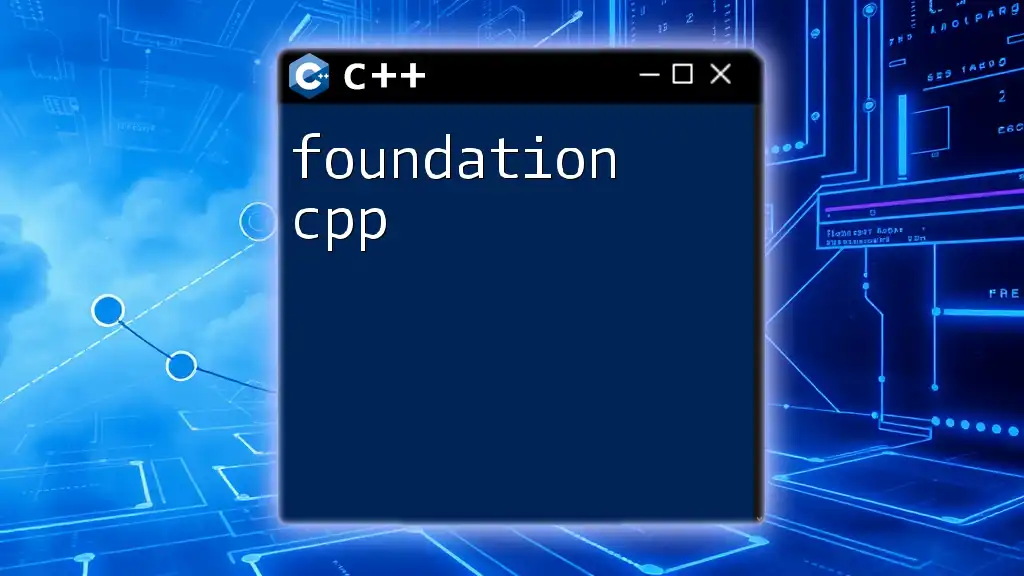
Serializing and Deserializing JSON
Understanding Serialization and Deserialization
Serialization is the process of converting a C++ data structure into JSON format. Conversely, deserialization is converting JSON data back into a C++ object or structure.
Converting C++ Data Structures to JSON
Here’s an example of how to serialize a custom C++ structure:
struct Employee {
std::string name;
int age;
};
void serializeEmployee(const Employee& emp, Json::Value& jsonData) {
jsonData["name"] = emp.name;
jsonData["age"] = emp.age;
}
// Usage
Employee emp = {"John", 29};
Json::Value empJson;
serializeEmployee(emp, empJson);
In this example, we define a function that converts an `Employee` struct into a JSON object.
Deserializing JSON into C++ Objects
Similarly, converting JSON data back to C++ objects can be done as follows:
void deserializeEmployee(const Json::Value& jsonData, Employee& emp) {
emp.name = jsonData["name"].asString();
emp.age = jsonData["age"].asInt();
}
// Usage
Employee emp;
deserializeEmployee(empJson, emp);
With these steps, you can take structured JSON data and recreate C++ objects easily.
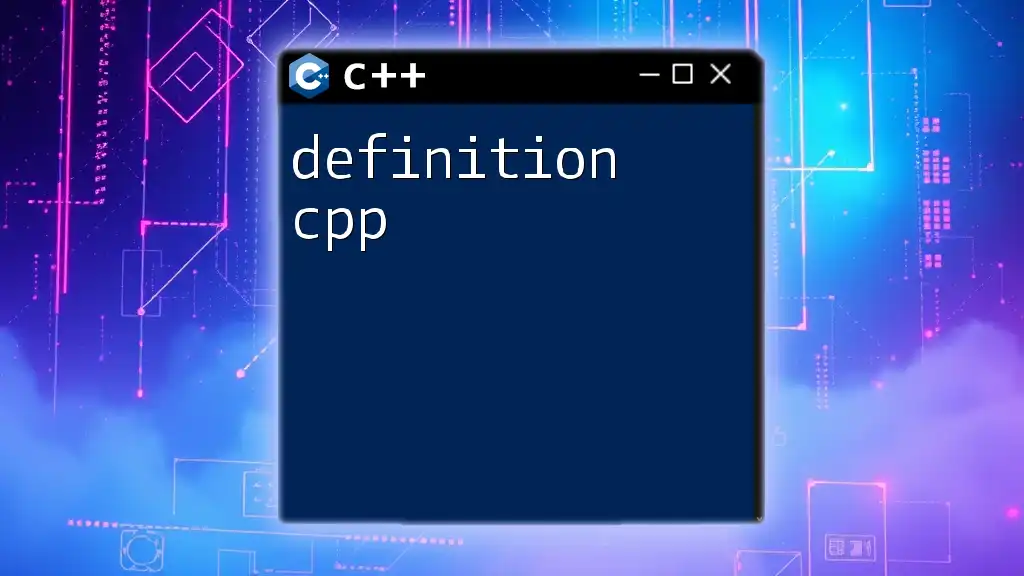
Error Handling and Validation
Handling JSON Parsing Errors
It is crucial to handle errors when parsing JSON, as invalid data can lead to unexpected behavior:
if (!Json::parseFromStream(readerBuilder, jsonFile, &jsonData, &errors)) {
std::cerr << "Error parsing JSON: " << errors << std::endl;
}
This example illustrates how to check for parsing errors and output a message if the JSON is invalid.
Validating JSON Data
To ensure the integrity of your JSON data, validating it is essential. Here is a simple validation routine:
bool validateJson(const Json::Value& jsonData) {
return jsonData.isMember("employees") && jsonData["employees"].isArray();
}
This function checks if the JSON contains the expected structure and types.
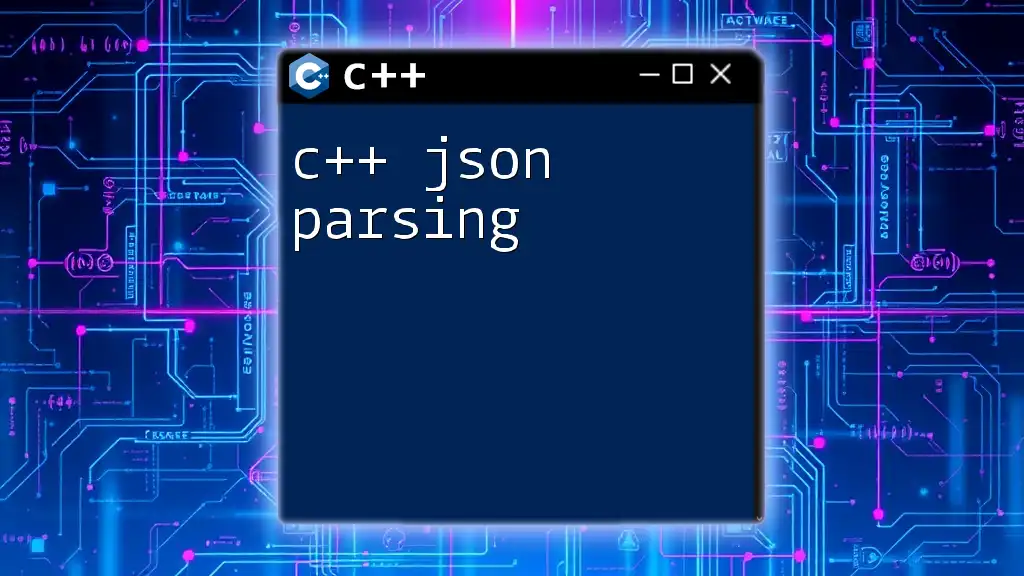
Advanced Features of json-cpp
Using json-cpp with STL Containers
json-cpp integrates well with C++ Standard Template Library (STL) containers. For example, filling a JSON array with a vector of strings:
std::vector<std::string> names = {"Alice", "Bob", "Charlie"};
Json::Value jsonArray;
for (const auto& name : names) {
jsonArray.append(name);
}
This snippet demonstrates how to convert a vector into a JSON array easily.
Performance Considerations
While json-cpp is efficient, there are strategies to optimize performance further. For instance, minimizing memory allocations and reusing `Json::Value` instances can enhance object handling speed. Additionally, profiling and benchmarking your JSON processing can identify bottlenecks.
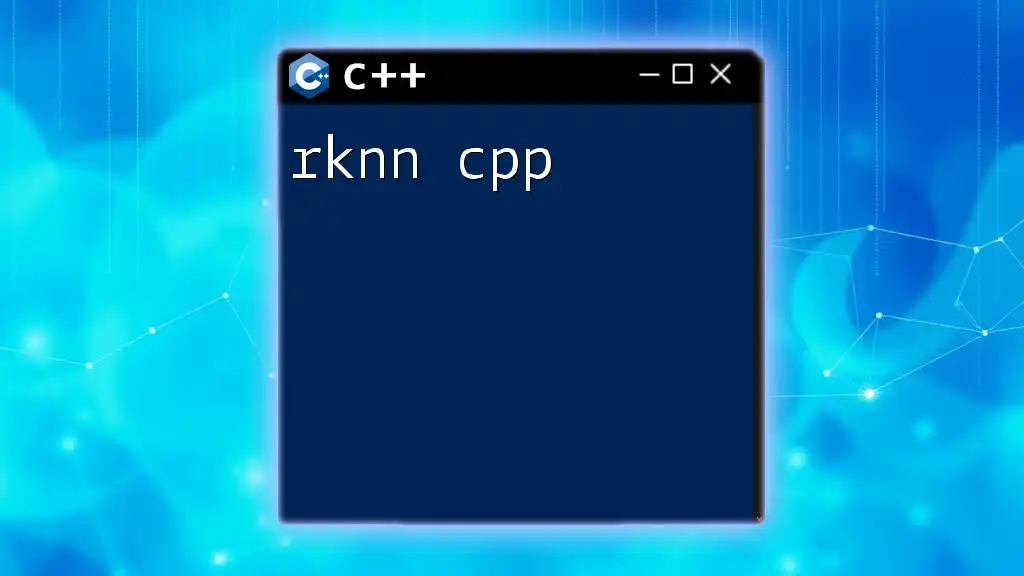
Conclusion
In summary, json-cpp provides a powerful and flexible toolset for C++ developers working with JSON data. By understanding how to create, access, modify, serialize, and deserialize JSON data, you can effectively integrate JSON handling into your applications. The library's ease of use and broad functionality make it an excellent choice for managing data interchange formats in performance-oriented software. Experiment further with json-cpp, and expand your understanding of JSON handling in C++.
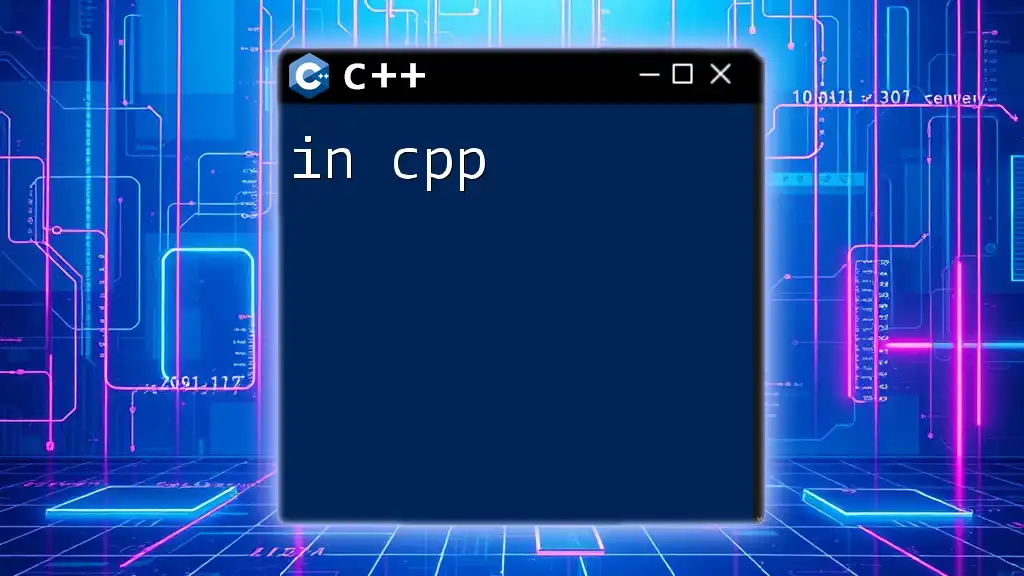
Additional Resources
-
Official Documentation
You can find the official documentation and source files on the [json-cpp GitHub page](https://github.com/open-source-parsers/jsoncpp). -
Tutorials and Examples
For more in-depth tutorials and examples, explore community resources that can help you become proficient in both C++ and JSON handling.