AWS CPP refers to the use of C++ commands and SDKs to interact with Amazon Web Services for cloud-based application development. Here’s a simple example of how to use the AWS SDK for C++ to create an S3 bucket:
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <aws/s3/model/CreateBucketRequest.h>
int main() {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
Aws::S3::S3Client s3_client;
Aws::S3::Model::CreateBucketRequest request;
request.SetBucket("my-new-bucket");
auto outcome = s3_client.CreateBucket(request);
if (outcome.IsSuccess()) {
std::cout << "Bucket created successfully!" << std::endl;
} else {
std::cerr << "Error creating bucket: " << outcome.GetError().GetMessage() << std::endl;
}
}
Aws::ShutdownAPI(options);
return 0;
}
Understanding AWS Fundamentals
What is AWS?
Amazon Web Services (AWS) is a comprehensive cloud platform that offers a variety of services such as computing power, storage options, and networking capabilities. By providing a global infrastructure and a pay-as-you-go pricing model, AWS has become an industry leader in cloud computing.
The major components of AWS include:
- Compute Services: Such as EC2, Lambda, and Elastic Beanstalk
- Storage Options: Including S3, EBS, and Glacier
- Database Solutions: Like RDS, DynamoDB, and Redshift
- Networking Services: VPC, Route 53, and CloudFront
Benefits of Using AWS
Utilizing AWS for your application needs comes with several advantages:
- Scalability: AWS automatically adjusts resources based on traffic and workloads, allowing applications to grow seamlessly.
- Cost-effectiveness: With its pay-as-you-go model, you only pay for what you use, eliminating the need for hefty upfront investments.
- Flexibility: AWS offers a vast array of services, allowing developers to choose the right tools for their specific applications.
- Global Reach: With data centers around the globe, AWS ensures low latency and high availability for users everywhere.
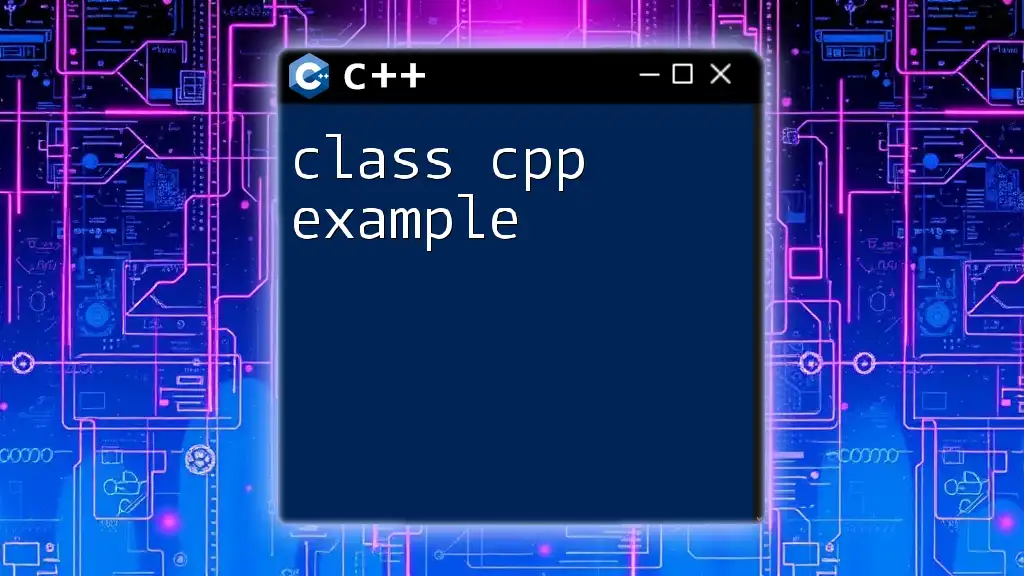
Getting Started with C++ on AWS
Setting Up Your Development Environment
To start using AWS with C++, you must set up your development environment. Here’s a simple guide to get you going:
-
Necessary Tools and IDEs: Choose a C++ IDE like Visual Studio, CLion, or Code::Blocks. Ensure you have a build system like CMake installed.
-
Installing AWS SDK for C++: The SDK provides APIs for various AWS services. You can install it via the following command (assuming you have CMake configured):
git clone https://github.com/aws/aws-sdk-cpp.git cd aws-sdk-cpp mkdir build cd build cmake .. && make sudo make install
-
Example Code Snippet: Here’s a basic setup to initialize the AWS SDK:
#include <aws/core/Aws.h> int main() { Aws::SDKOptions options; Aws::InitAPI(options); { // Your code goes here } Aws::ShutdownAPI(options); return 0; }
Overview of AWS SDK for C++
The AWS SDK for C++ simplifies the usage of AWS services in your C++ applications. It provides a set of APIs that abstract the complexities of interacting with the service endpoints and allows you to perform actions like creating a bucket in S3, sending messages to SQS, etc.
Key Features of the AWS SDK include:
- Comprehensive set of service APIs
- Cross-platform support for Linux, Windows, and macOS
- Easy integration with other C++ libraries
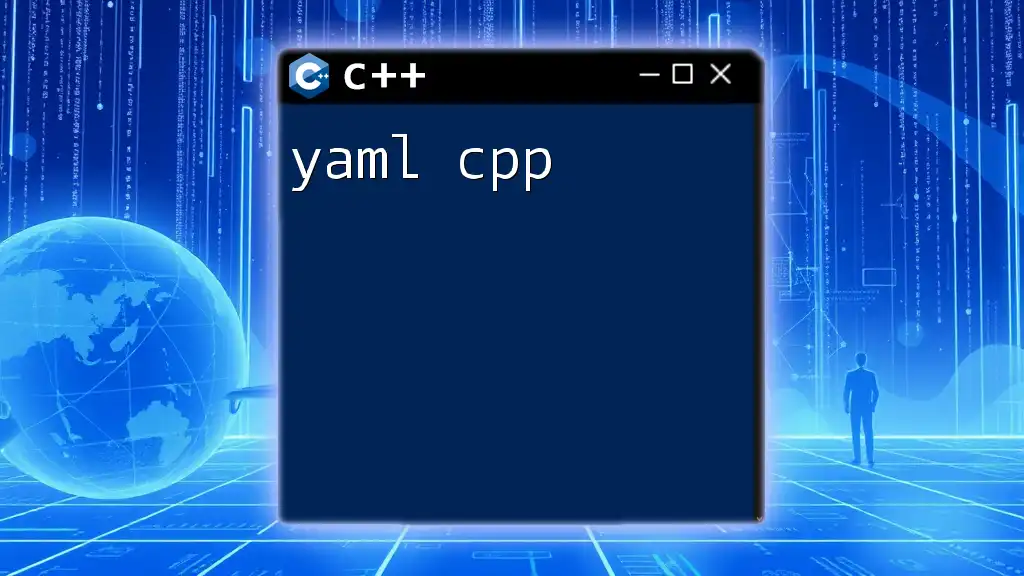
Key AWS Services and Their C++ Implementations
AWS S3 (Simple Storage Service)
What is AWS S3?
Amazon S3 is an object storage service that offers 99.999999999% durability, providing an ideal solution for backup and archival of data. Use cases range from hosting static websites to storing large datasets for analytics.
Using AWS S3 with C++
To interact with S3 using AWS SDK for C++, you can follow this example to upload a file.
Code Example: Uploading a File to S3
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <aws/s3/model/PutObjectRequest.h>
#include <fstream>
int main() {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
Aws::S3::S3Client s3;
Aws::S3::Model::PutObjectRequest object_request;
object_request.SetBucket("your-bucket-name");
object_request.SetKey("your-object-key");
std::shared_ptr<Aws::IOStream> input_data =
Aws::MakeShared<std::fstream>("SampleAllocation", "path/to/your/file", std::ios_base::in | std::ios_base::binary);
object_request.SetBody(input_data);
auto put_object_outcome = s3.PutObject(object_request);
if (put_object_outcome.IsSuccess()) {
std::cout << "Successfully uploaded file to S3." << std::endl;
} else {
std::cout << "Error uploading file: "
<< put_object_outcome.GetError().GetMessage()
<< std::endl;
}
}
Aws::ShutdownAPI(options);
return 0;
}
Explanation: This code initializes the AWS SDK, creates an S3 client, and uploads a file to a specified bucket. Note how the file is streamed into the request body and how error handling is implemented.
AWS EC2 (Elastic Compute Cloud)
What is AWS EC2?
Amazon EC2 provides scalable computing capacity in the cloud. It allows developers to launch virtual servers on demand to host web applications, databases, or any backend services.
Launching EC2 Instances with C++
To launch an EC2 instance programmatically, here’s how you can do it with AWS SDK for C++.
Code Example: Starting and Stopping EC2 Instances
#include <aws/core/Aws.h>
#include <aws/ec2/EC2Client.h>
#include <aws/ec2/model/RunInstancesRequest.h>
#include <aws/ec2/model/StopInstancesRequest.h>
int main() {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
Aws::EC2::EC2Client ec2;
// Start EC2 instance
Aws::EC2::Model::RunInstancesRequest run_instances_request;
run_instances_request.SetImageId("ami-0abcdef1234567890"); // Replace with your AMI ID
run_instances_request.SetInstanceType(Aws::EC2::Model::InstanceType::t2_micro);
run_instances_request.SetMinCount(1);
run_instances_request.SetMaxCount(1);
auto run_instances_outcome = ec2.RunInstances(run_instances_request);
if (run_instances_outcome.IsSuccess()) {
std::cout << "Successfully started EC2 instance." << std::endl;
} else {
std::cout << "Error starting instance: "
<< run_instances_outcome.GetError().GetMessage()
<< std::endl;
}
// Stopping an instance can similarly be done with StopInstancesRequest
}
Aws::ShutdownAPI(options);
return 0;
}
Detailed Walkthrough of the Code: After initializing the SDK, this code creates an EC2 client and sends a request to start an EC2 instance, specifying the AMI ID and instance type. Upon success, it prints a confirmation; otherwise, it shows any errors encountered.
AWS Lambda
What is AWS Lambda?
AWS Lambda lets you run code without provisioning or managing servers, often referred to as serverless computing. It’s especially useful for event-driven architectures or microservices.
Invoking Lambda Functions from C++
To invoke a Lambda function with C++, you would use the following example:
Code Example: Calling a Lambda Function
#include <aws/core/Aws.h>
#include <aws/lambda/LambdaClient.h>
#include <aws/lambda/model/InvokeRequest.h>
int main() {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
Aws::Lambda::LambdaClient lambda_client;
Aws::Lambda::Model::InvokeRequest invoke_request;
invoke_request.SetFunctionName("your_lambda_function_name");
invoke_request.SetPayload("{\"key1\":\"value1\"}"); // Example JSON payload
auto invoke_outcome = lambda_client.Invoke(invoke_request);
if (invoke_outcome.IsSuccess()) {
std::cout << "Lambda function invoked successfully." << std::endl;
} else {
std::cout << "Error invoking Lambda function: "
<< invoke_outcome.GetError().GetMessage()
<< std::endl;
}
}
Aws::ShutdownAPI(options);
return 0;
}
Explanation of Input and Output Handling: This example explains how to set up and send an invocation request to a defined Lambda function, showcasing error handling and payload management.
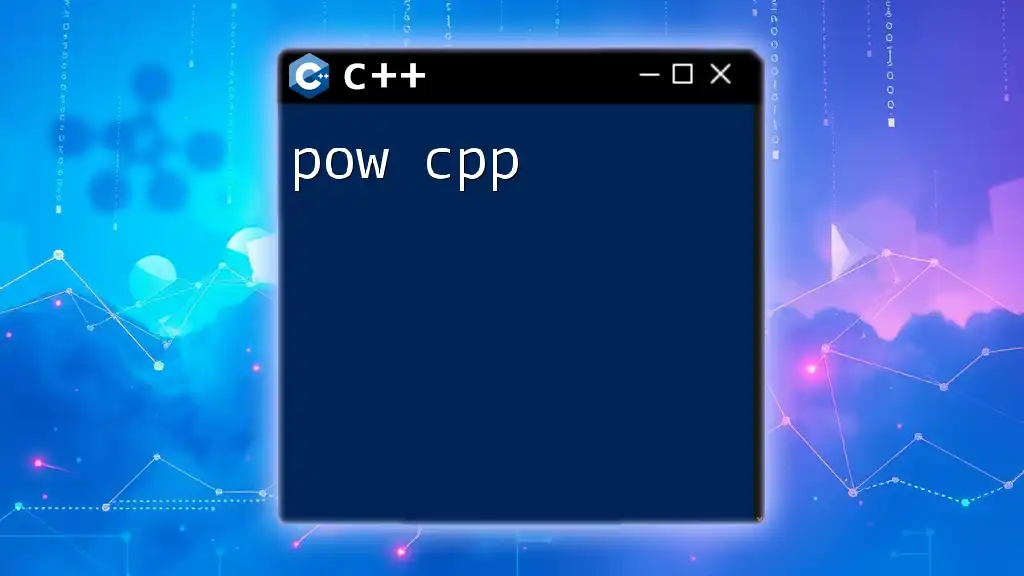
Best Practices for Developing C++ Applications in AWS
Efficient Resource Management
When developing applications in AWS with C++, it’s crucial to manage resources efficiently. This includes:
- Optimizing Usage of AWS Services: Scale your resources based on the workload. Use tools like AWS Auto Scaling to adjust your EC2 instances automatically.
- Error Handling Example: Always include comprehensive error handling to identify issues quickly. This improves reliability and user experience.
Security Practices
Security should always be a priority in any cloud application. Key practices include:
- Best Practices for Securing AWS Resources: Implement IAM roles carefully and always follow the principle of least privilege.
- Code Example: Using IAM Roles with C++: Be sure to assign appropriate IAM roles for your services, enabling them to interact securely with other AWS resources.
Performance Optimization
Achieving optimal performance is vital for any application. Some tips include:
- Monitoring and Logging Best Practices: Use AWS CloudWatch for monitoring application performance and setting up alerts for various metrics.
- Code Snippet: Utilizing AWS CloudWatch: Here's how to log custom metrics:
#include <aws/cloudwatch/CloudWatchClient.h>
#include <aws/cloudwatch/model/PutMetricDataRequest.h>
void LogCustomMetric(Aws::CloudWatch::CloudWatchClient& client) {
Aws::CloudWatch::Model::PutMetricDataRequest request;
request.SetNamespace("YourNamespace");
Aws::CloudWatch::Model::MetricDatum datum;
datum.SetMetricName("YourMetricName");
datum.SetValue(1.0); // Example value
request.AddMetricData(datum);
auto outcome = client.PutMetricData(request);
if (!outcome.IsSuccess()) {
std::cerr << "Failed to publish metrics: "
<< outcome.GetError().GetMessage() << std::endl;
}
}
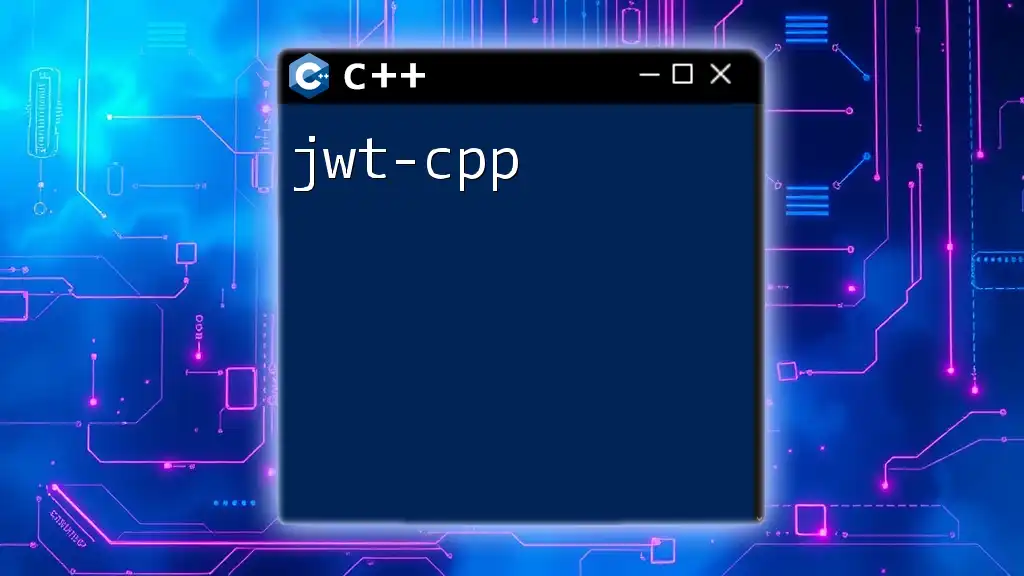
Deploying and Managing C++ Applications on AWS
Continuous Integration and Continuous Deployment (CI/CD)
Implementing CI/CD practices helps streamline deployment processes. AWS offers several tools such as:
- AWS CodePipeline: for automating your release pipelines.
- AWS CodeBuild: for building and testing your C++ applications.
Monitoring and Troubleshooting
As you develop your C++ applications on AWS, always include strategies for monitoring and troubleshooting:
- Tools for Monitoring C++ Applications on AWS: Combine AWS CloudWatch with custom logging to track performance and issues.
- Debugging Tips and Techniques: Utilize logging liberally. Capture both expected and unexpected flow of execution, allowing you to trace issues back effectively.
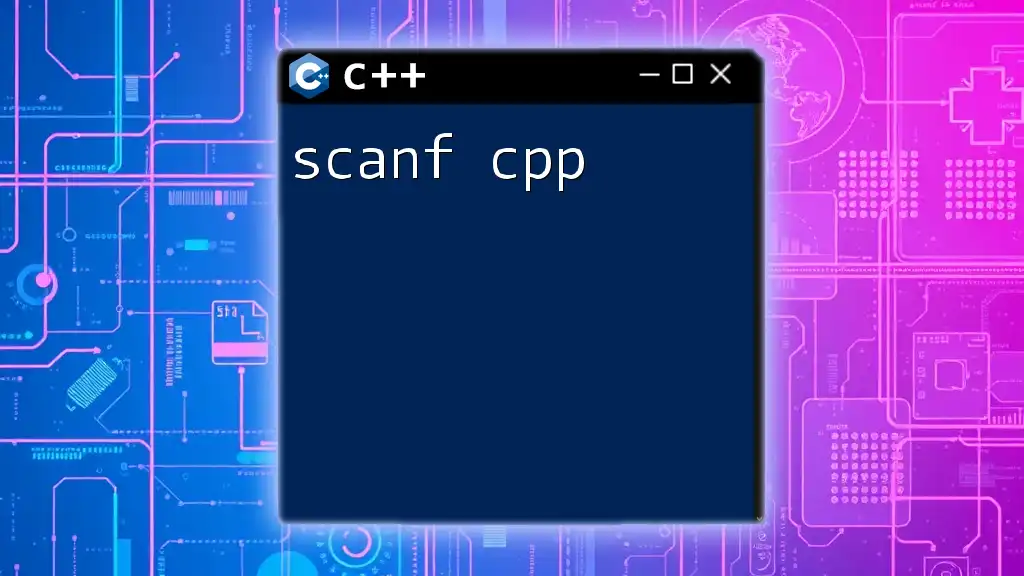
Real-world Use Cases of C++ in AWS
Case Study 1: High-frequency Trading
In a high-frequency trading application, C++ was utilized for its speed and efficiency. The application architecture involved:
- Leveraging AWS Lambda for event-driven architecture due to its responsiveness.
- Storing vast datasets in Amazon S3, with rapid access via C++ code for analytics.
Case Study 2: Game Development
Game developers traditionally use C++ due to its performance capabilities. A game development project utilized AWS to host back-end services:
- Real-time player data was processed using AWS DynamoDB.
- Management of server instances was handled via AWS EC2, scaling automatically based on player activity.
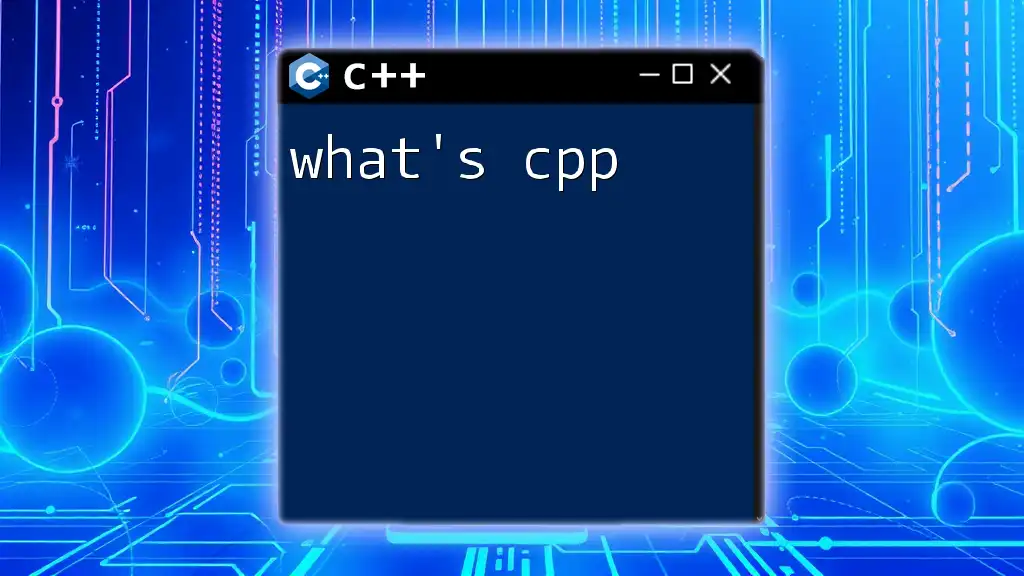
Conclusion
Summarizing our exploration of AWS CPP, we have seen how C++ integrates effectively with various AWS services, enhancing application performance and potential scalability. Significant advantages stem from the cloud resources that AWS provides, allowing developers to focus on building high-quality applications without the burden of infrastructure management.
As you dive deeper into AWS with C++, remember to leverage the comprehensive suite of tools and follow the best practices outlined above. Continued exploration and experimentation will lead you to new insights and improved application performance as you harness the power of AWS.
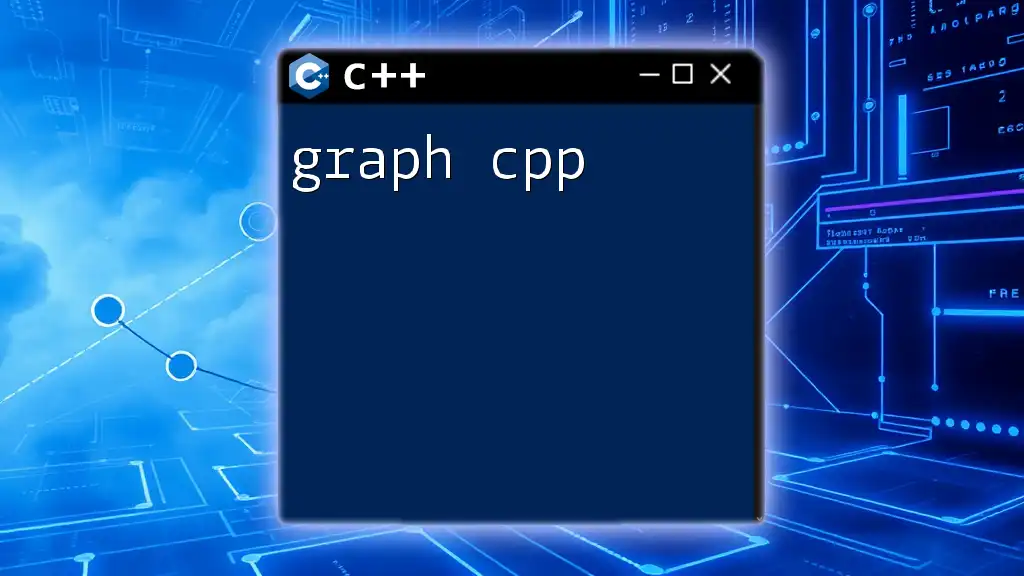
FAQs
Common Questions Regarding AWS and C++
-
What’s the Learning Curve for Using AWS SDK with C++? The learning curve can vary but is generally manageable for those with a foundational knowledge in C++. The SDK is well-documented, making it accessible.
-
How Can C++ Applications Take Advantage of AWS Scalability? By designing applications with cloud-native services such as AWS Auto Scaling, developers can adjust resource allocation based on demand dynamically, allowing applications to efficiently handle variable workloads.