"Graph CPP" involves utilizing C++ to represent and manipulate graph data structures efficiently, enabling operations like traversal and pathfinding. Here's a simple code snippet to create and display an undirected graph using an adjacency list:
#include <iostream>
#include <vector>
using namespace std;
class Graph {
int V; // Number of vertices
vector<vector<int>> adj; // Adjacency list
public:
Graph(int V) {
this->V = V;
adj.resize(V);
}
void addEdge(int v, int w) {
adj[v].push_back(w);
adj[w].push_back(v); // For undirected graph
}
void display() {
for (int v = 0; v < V; v++) {
cout << "Vertex " << v << ": ";
for (int w : adj[v]) {
cout << w << " ";
}
cout << endl;
}
}
};
int main() {
Graph g(5);
g.addEdge(0, 1);
g.addEdge(0, 4);
g.addEdge(1, 2);
g.addEdge(1, 3);
g.addEdge(1, 4);
g.addEdge(2, 3);
g.addEdge(3, 4);
g.display();
return 0;
}
What is a Graph?
Definition of Graph
A graph is a fundamental data structure in computer science that consists of a set of vertices (or nodes) and a set of edges that connect pairs of vertices. In a directed graph, edges have a direction, implying a one-way relationship, while in an undirected graph, edges imply a two-way relationship. Additionally, edges can be weighted or unweighted, where weights typically signify costs or distances associated with traversing between two vertices.
Applications of Graphs
Graphs can model various real-world scenarios effectively. For instance, in social networks, individuals are represented as vertices, and connections or friendships as edges. In navigation systems, locations are vertices and routes between them form the edges. Problems such as finding the shortest path, determining the minimum spanning tree, and maximizing network flows can be articulated and solved using graph structures.

Representing Graphs in C++
Adjacency Matrix
An adjacency matrix is a square matrix used to represent a graph. The matrix has dimensions \(N \times N\), where \(N\) is the number of vertices. Each cell, \(matrix[i][j]\), indicates whether there is an edge from vertex \(i\) to vertex \(j\).
Using an adjacency matrix can be more efficient for dense graphs; however, it consumes \(O(N^2)\) space, which might not be ideal for sparse graphs.
Code Example:
const int MAX = 100; // maximum number of vertices
int adjMatrix[MAX][MAX]; // adjacency matrix representation
Adjacency List
An adjacency list is another way to represent graphs more space-efficiently, especially in sparse graphs. Each vertex has a list that contains its neighboring vertices. The adjacency list can be implemented using arrays, vectors, and linked lists.
This method requires less memory, using \(O(V + E)\), where \(V\) is the number of vertices and \(E\) is the number of edges.
Code Example:
#include <vector>
#include <list>
class Graph {
public:
int numVertices;
std::vector<std::list<int>> adjList; // adjacency list representation
Graph(int v) : numVertices(v), adjList(v) {}
void addEdge(int u, int v);
};

Basic Graph Operations in C++
Adding Edges
To connect vertices in a graph, we can add edges. In an adjacency list, adding an edge involves adding a vertex to another vertex’s list.
Example of Adding an Edge:
void Graph::addEdge(int u, int v) {
adjList[u].push_back(v); // adds edge from u to v
// For an undirected graph, add the reverse edge
adjList[v].push_back(u);
}
Removing Edges
Removing edges is another essential operation, and similar to adding edges, the process varies between the adjacency matrix and list.
Example of Removing an Edge:
void Graph::removeEdge(int u, int v) {
adjList[u].remove(v); // remove edge from u to v
adjList[v].remove(u); // for undirected graphs
}
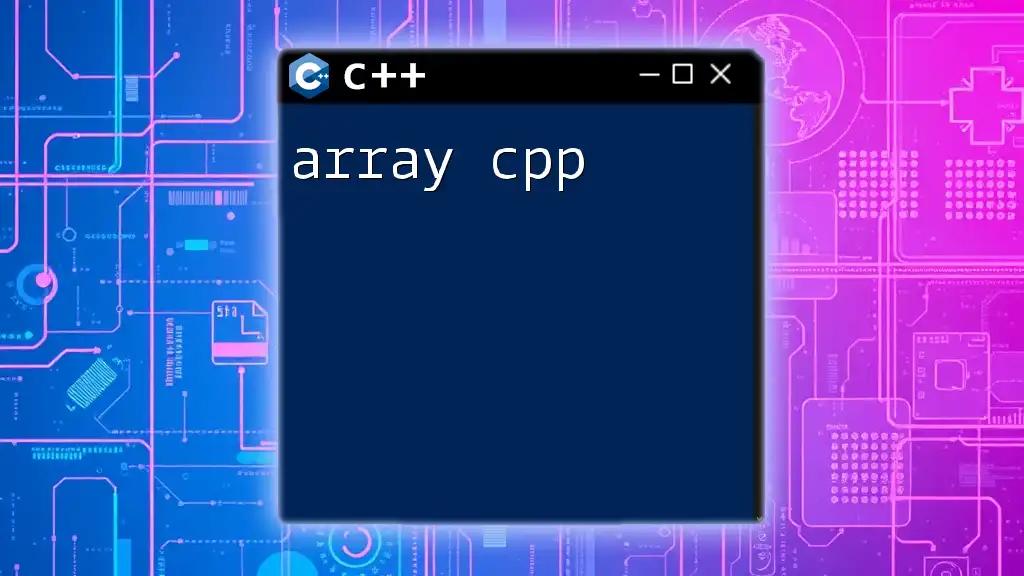
Traversing Graphs
Depth-First Search (DFS)
Depth-First Search (DFS) is an algorithm for traversing or searching tree or graph data structures. It explores as far as possible along each branch before backtracking, using a stack (either implicitly via recursion or explicitly).
Implementation in C++:
void DFS(int v, std::vector<bool>& visited) {
visited[v] = true;
for (int neighbor : adjList[v]) {
if (!visited[neighbor]) {
DFS(neighbor, visited); // Recursive call for further exploration
}
}
}
Breadth-First Search (BFS)
Breadth-First Search (BFS), on the other hand, explores the neighbor vertices before progressing to the next level of vertices. This approach uses a queue.
Implementation in C++:
void BFS(int start) {
std::vector<bool> visited(numVertices, false);
std::queue<int> q;
q.push(start);
visited[start] = true;
while (!q.empty()) {
int v = q.front();
q.pop();
for (int neighbor : adjList[v]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
q.push(neighbor); // Add unvisited neighbors to the queue
}
}
}
}
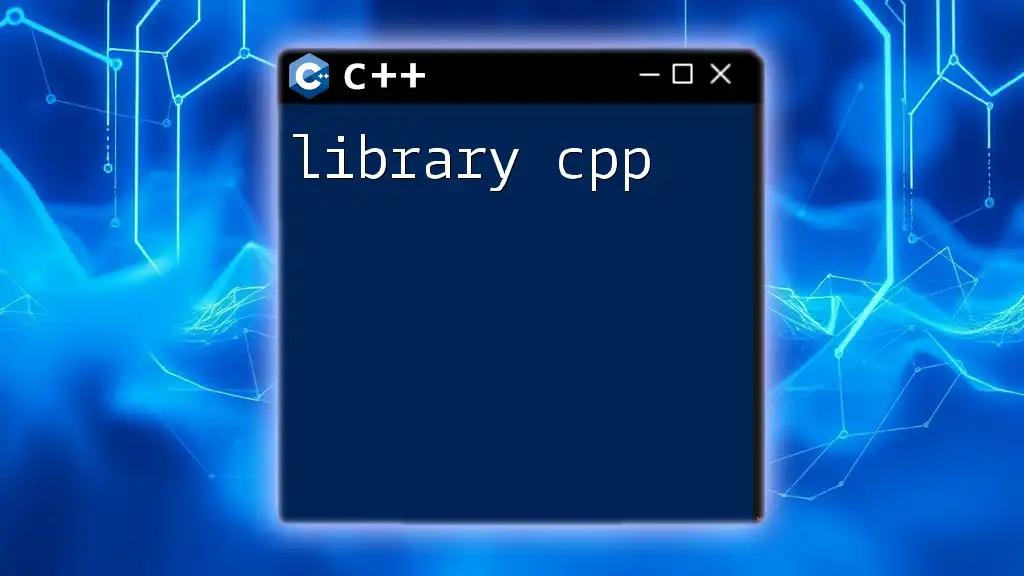
Advanced Graph Concepts
Shortest Path Algorithms
One of the most critical applications of graphs is finding the shortest path between two vertices. Dijkstra's Algorithm is designed for graphs with non-negative weights.
Implementation Example:
void dijkstra(int src) {
std::vector<int> dist(numVertices, INT_MAX);
dist[src] = 0;
std::priority_queue<std::pair<int, int>> pq;
pq.push({0, src});
// Implementation details (distances calculation, queue management, etc.)
}
Minimum Spanning Tree
Finding the Minimum Spanning Tree (MST) can be accomplished using Prim's Algorithm. This method connects all vertices together while minimizing the overall edge weight.
Implementation Example:
void primMST() {
// Implementation details involving priority queues and edge selections
}
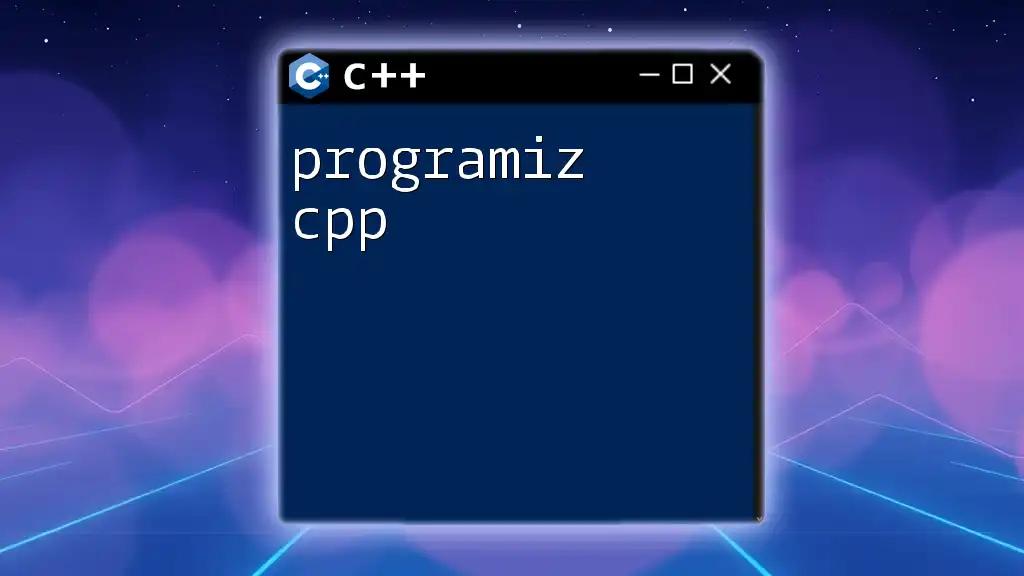
Common Graph Problems
Finding Cycles
Detecting cycles in a graph can be essential, particularly in directed graphs. Algorithms often use DFS with additional markers to gauge visiting states of vertices.
Graph Coloring
The graph coloring problem entails coloring the vertices of a graph such that no two adjacent vertices share the same color. Finding an optimal solution is a classic NP-hard problem, but greedy algorithms can yield acceptable solutions.
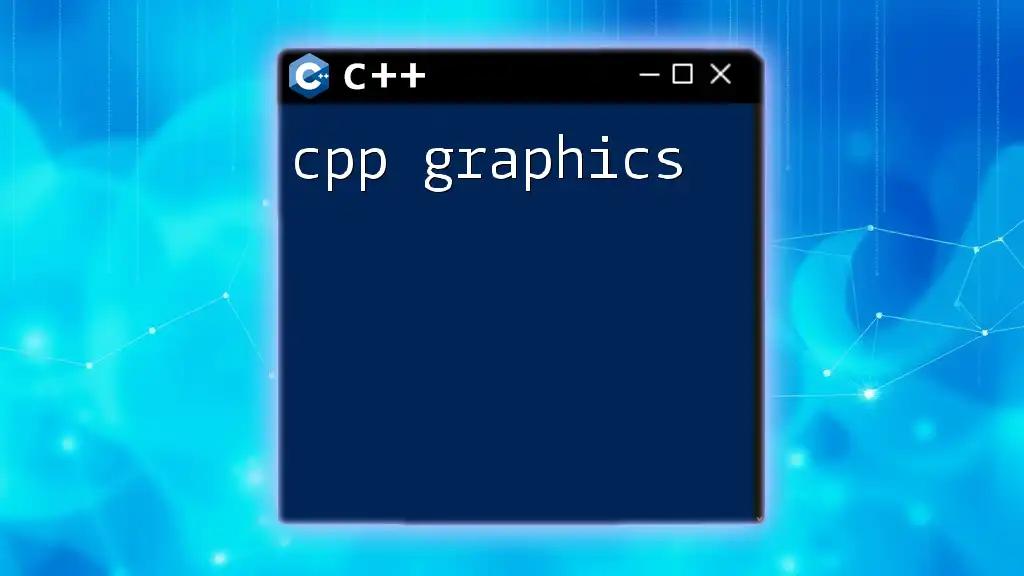
Conclusion
In summary, understanding graph cpp is crucial for any programmer tackling real-world problems involving networks or relationships. Mastering graph representations, basic operations, traversals, and advanced algorithms will empower you to efficiently address challenges in various domains.
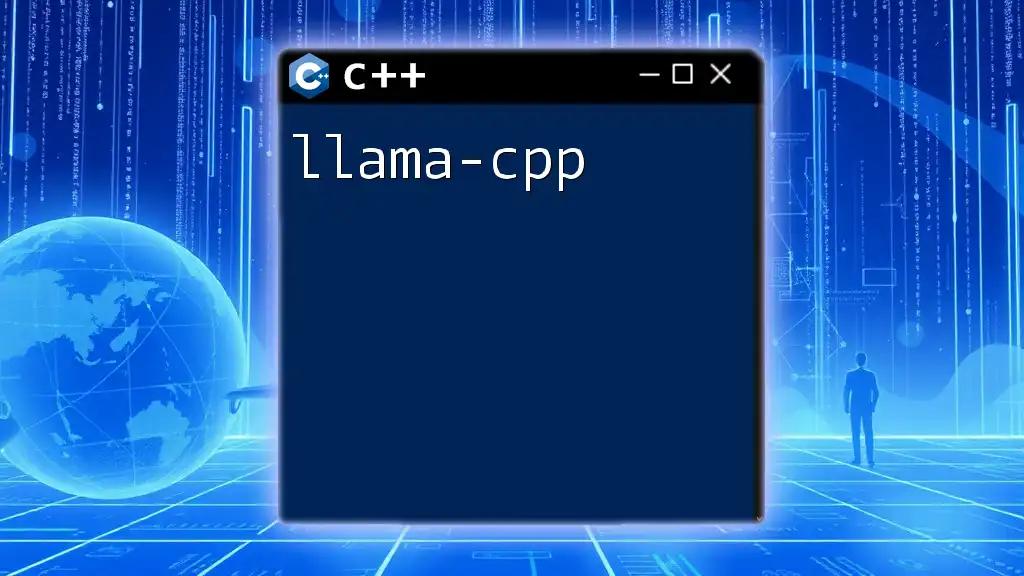
Resources
For further exploration into graph theory and applications in C++, consider checking reputable programming texts, online courses, and interactive coding platforms. Delve deeper into advanced algorithms to refine your skill set and enhance your understanding.
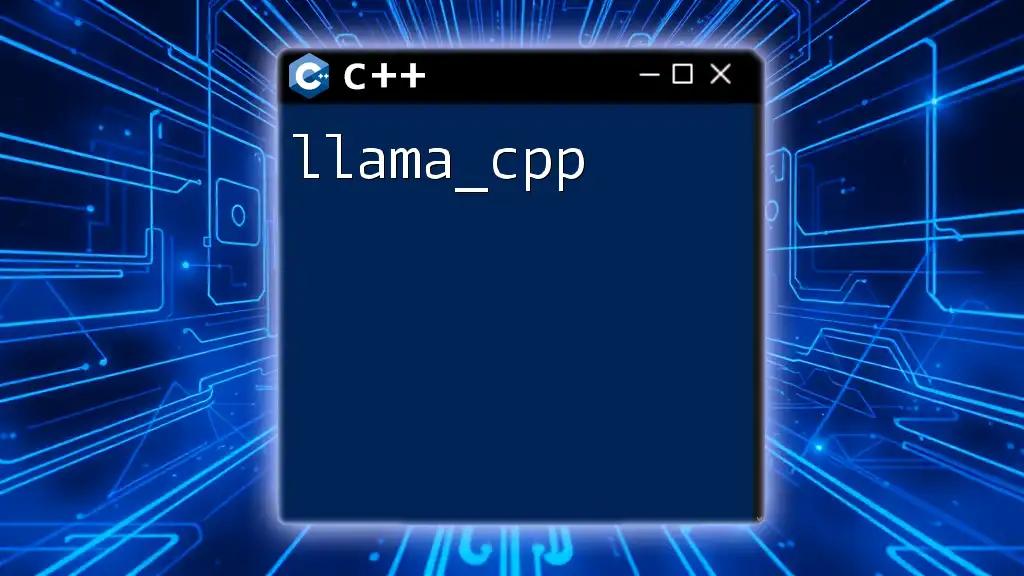
Call to Action
Join our upcoming courses to learn about C++ graph commands and discover the vast capabilities of graphs in programming. Embrace the challenge and elevate your coding skills!