A REST API in C++ allows developers to create web services that communicate over HTTP using the C++ programming language, enabling interaction with web clients in a lightweight and structured manner.
Here's a simple example of a C++ REST API using the Crow microcontroller framework:
#include "crow_all.h"
int main(){
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, World!";
});
app.port(18080).multithreaded().run();
}
Understanding RESTful APIs
What is REST?
REST, or Representational State Transfer, is an architectural style that defines a set of constraints and properties based on HTTP. It emphasizes a stateless, client-server communication model where interactions between the client and server happen through dedicated endpoints. The primary principles of REST include:
- Statelessness: Every request from the client must contain all the information needed to process that request. The server does not store any session-based information, making the API scalable and easier to manage.
- Client-Server Architecture: This principle separates the user interface from the data storage system. It allows different clients to interact with the same API without depending on the server implementation.
- Resources: In REST, everything is considered a resource, which is identified by a unique URL. This allows for a structured way to access data.
REST vs. SOAP
When comparing REST to SOAP (Simple Object Access Protocol), REST offers key advantages in terms of simplicity and flexibility. While SOAP requires a more rigid set of standards and protocols, REST allows the use of different formats and is easier to understand and implement with modern web technologies. REST is generally more suitable for web applications and services, particularly when developing APIs with C++.
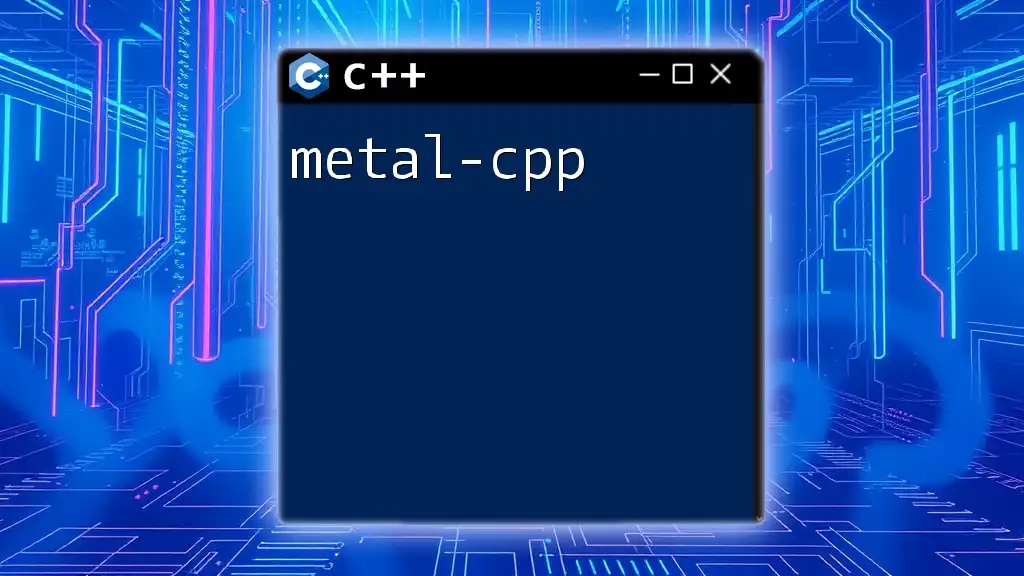
Getting Started with C++ REST API Development
Setting Up Your Development Environment
To create a REST API in C++, you'll need to set up a development environment that includes:
- A robust IDE: Consider using Visual Studio, Code::Blocks, or CLion for better code management and debugging.
- A suitable compiler: G++ (part of the GCC project) and Clang are both excellent choices for compiling your C++ code.
Common Libraries for C++ REST API
A few libraries have emerged as popular choices for building REST APIs in C++:
-
Boost.Beast: A part of the Boost library, it provides HTTP and WebSocket functionality. It's a robust choice for developers who are already familiar with Boost.
-
Pistache: A lightweight HTTP and REST framework that’s easy to set up and supports modern C++ standards.
-
Crow: A C++ microframework designed for building web applications. It has a simple routing interface and is suitable for smaller projects.
Consider the pros and cons of each based on your project needs—ease of use versus comprehensive features.
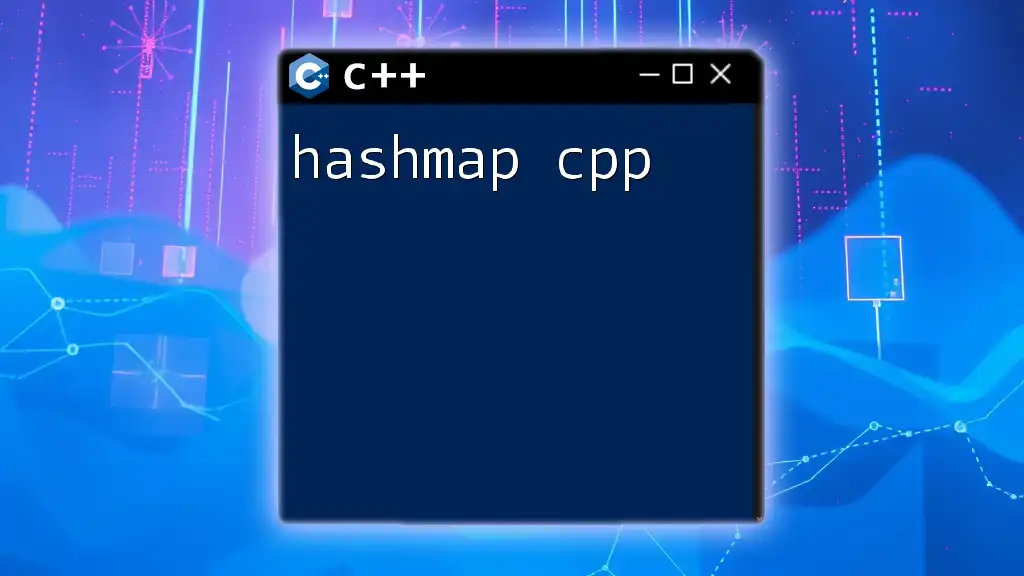
Building a Simple REST API with C++
Designing Your API
Before diving into coding, it’s crucial to design your API. This includes defining what resources you'll expose and how clients will interact with them. Well-designed APIs often follow REST conventions by utilizing meaningful URLs, resources, and proper HTTP methods.
Implementing Your First REST API
Let's build a basic REST API that responds to GET requests. Here’s an example of how you might set it up using the Crow library:
#include "crow_all.h"
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/welcome")
([]() {
return "Welcome to the REST API!";
});
app.port(18080).multithreaded().run();
}
This framework allows you to easily define routes and functionality. When you navigate to `http://localhost:18080/welcome`, you'll see the response "Welcome to the REST API!"
Handling HTTP Methods
As REST APIs depend on different HTTP methods, you must implement the following:
- GET: Retrieve resources.
- POST: Create new resources.
- PUT: Update existing resources.
- DELETE: Remove resources.
Here’s an example demonstrating how to handle both POST and GET methods using Crow:
#include "crow_all.h"
#include <json/json.h>
std::vector<std::string> items;
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/items")
.methods("GET"_method)([](const crow::request& req) {
crow::json::wvalue json_response;
json_response["items"] = items;
return json_response;
});
CROW_ROUTE(app, "/items")
.methods("POST"_method)([](const crow::request& req) {
auto json_body = crow::json::load(req.body);
if (!json_body) {
return crow::response(400);
}
std::string item = json_body["item"].s();
items.push_back(item);
return crow::response(201);
});
app.port(18080).multithreaded().run();
}
Error Handling and Logging
Error handling is critical in any API. Implement a systematic approach to catch and log errors:
try {
// ... API logic
} catch (const std::exception& e) {
CROW_LOG_ERROR << "Error: " << e.what();
return crow::response(500); // Internal Server Error
}
Logging requests and errors not only helps during development but also enhances the maintenance and debugging process in production.
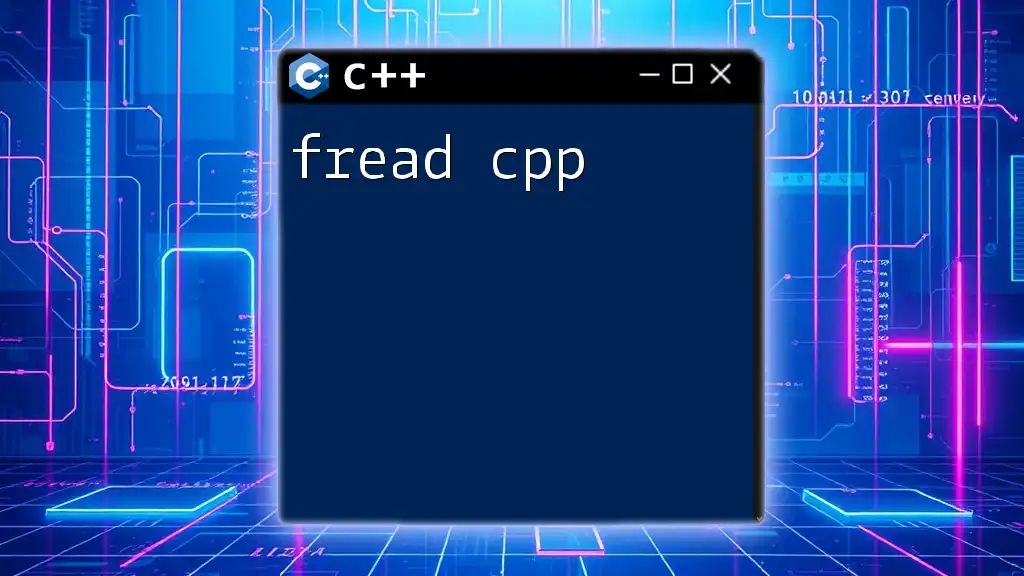
Securing Your C++ REST API
Authentication and Authorization
In a world where security is paramount, implementing authentication and authorization is essential. OAuth and JWT (JSON Web Tokens) are common methods.
With JWT, a client retrieves a token after successful authentication, which can then be used in subsequent requests for authorization. This approach streamlines access to various endpoints without exposing sensitive data.
Best Practices for API Security
To protect your REST API:
- Validate Input: Always validate user inputs to prevent injection attacks.
- Use HTTPS: Ensure encryption of all traffic to secure data in transit.
- Rate Limiting: Implement rate limiting to prevent abuse and denial-of-service attacks.
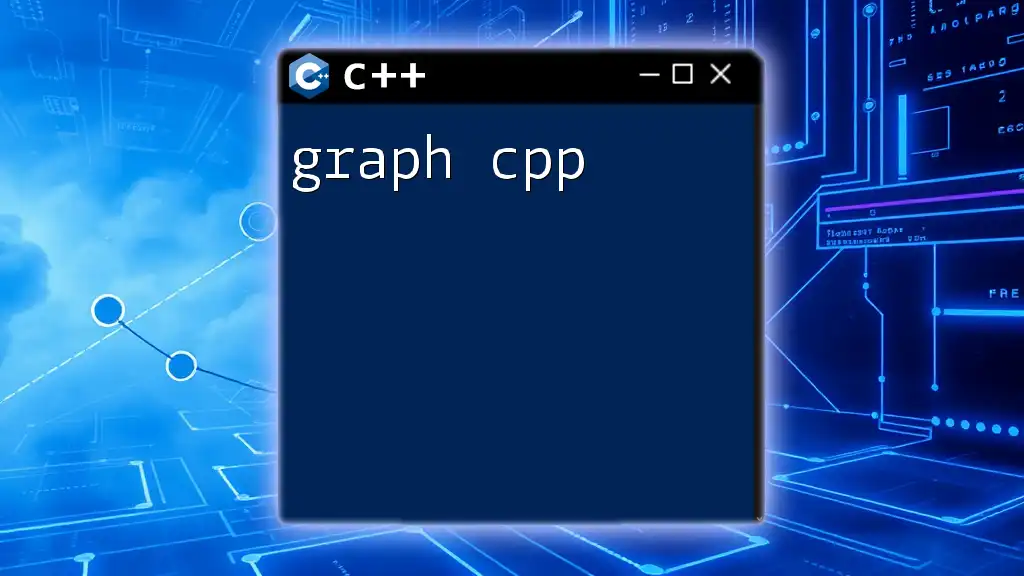
Testing Your C++ REST API
Importance of Testing
Testing your API is crucial to ensure reliability, performance, and correctness. It helps identify potential issues before they affect users.
Tools and Techniques for Testing
Popular tools for testing REST APIs include Postman and CURL. These tools enable you to simulate requests and observe responses without needing a front end.
To write test cases for your endpoints, consider using a testing framework like Catch or Google Test. Here's a simple example of a test case using Google Test:
#include <gtest/gtest.h>
TEST(ItemTest, AddItem) {
// Your logic to test adding an item
ASSERT_EQ(items.size(), 1); // Check that an item has been added
}
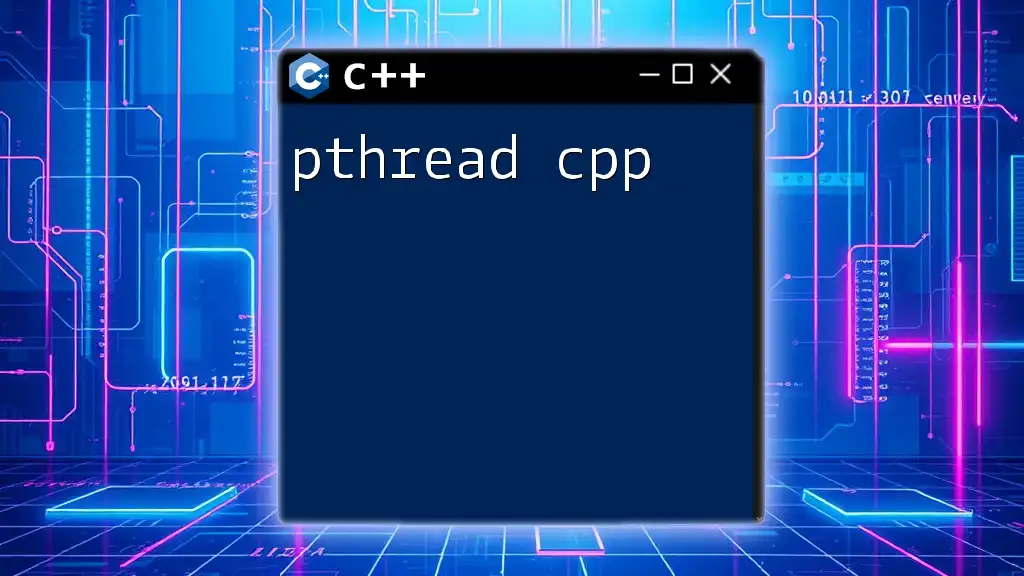
Documentation and Best Practices
Writing Clear Documentation
Good documentation is essential for the usability of your API. It should include clear instructions on endpoint usage, parameters, and examples. Tools like Swagger and Doxygen can automatically generate documentation from your code.
Best Practices for REST API Development
As you develop your REST API in C++, consider implementing the following best practices:
- Use meaningful HTTP status codes: Communicate the success or failure of requests effectively.
- Versioning: Maintain versioning in your API to manage future changes without breaking existing clients.
- Consistent Naming Conventions: Use clear and consistent naming for endpoints to enhance usability.
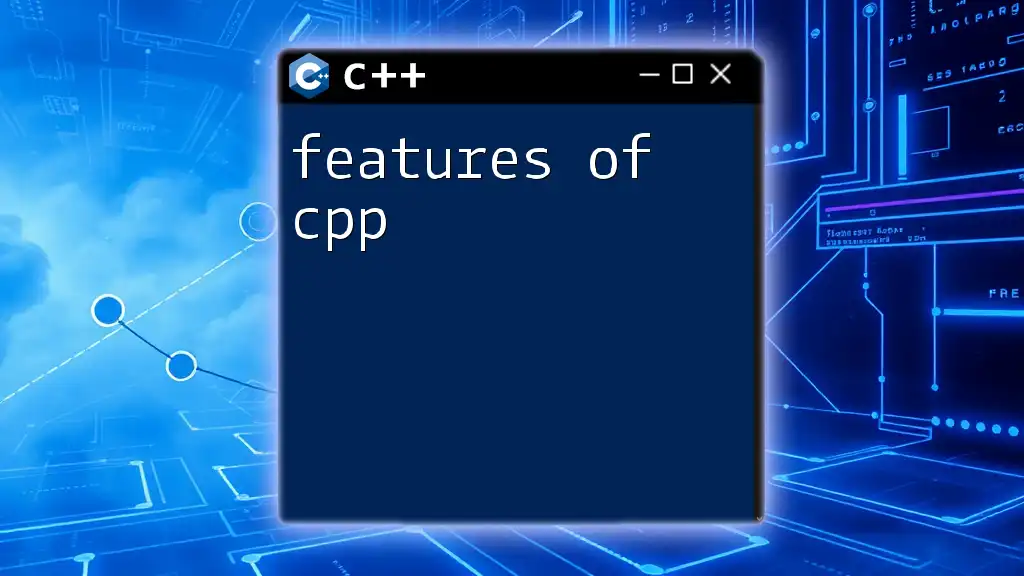
Conclusion
Building a REST API C++ is a rewarding endeavor that allows you to leverage the power of C++ while implementing flexible and scalable web services. By following the guidelines and practices outlined in this article, you can develop APIs that are robust, secure, and easy to use. Embrace the challenge, continue learning, and experiment with different libraries and tools to advance your skills in C++ RESTful API development.
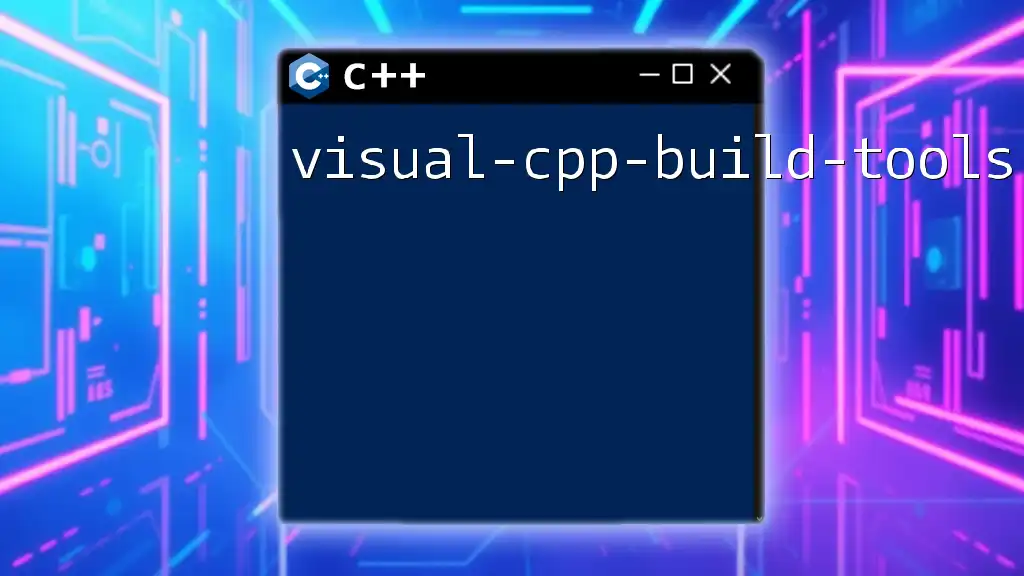
Resources and References
- Books on C++ Software Design
- C++ REST libraries documentation (Boost, Pistache, Crow)
- Tutorials on REST API development
With this knowledge, you are well-equipped to dive into the world of C++ REST APIs. Happy coding!