The `itoa` function in C++ is used to convert an integer value into a null-terminated string representation, allowing you to manipulate numerical data as text.
#include <iostream>
#include <cstdlib>
int main() {
int number = 123;
char buffer[20];
itoa(number, buffer, 10); // Convert integer to string using base 10
std::cout << "The string representation is: " << buffer << std::endl;
return 0;
}
What is itoa in C++?
The `itoa` function is primarily designed to convert an integer into its ASCII string representation. This means that if you have an integer, you can easily convert it into a string that represents that number, which is essential for displaying numbers in textual form or performing string manipulations. It is particularly useful in applications where numeric output is essential, such as in games or embedded systems programming.
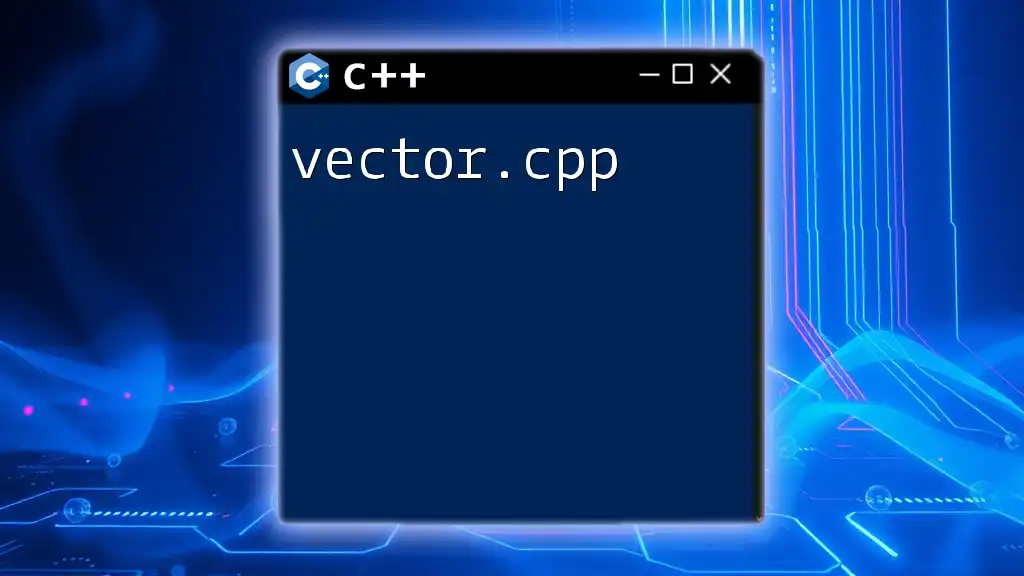
Syntax of itoa in C++
The syntax of the `itoa` function can be broken down as follows:
char* itoa(int value, char* str, int base);
Parameters Explained
- `int value`: The integer that you want to convert to a string.
- `char str`:* A pointer to a character array (buffer) where the resulting string will be stored. It's important to ensure that this buffer is large enough to hold the converted string.
- `int base`: The numerical base to use for the conversion. This can be binary (2), octal (8), decimal (10), or hexadecimal (16).
Example of itoa Syntax in Action
To illustrate the syntax in practice, consider the following example:
char buffer[20];
itoa(12345, buffer, 10); // Converts the integer 12345 to a string in base 10
In this example, `buffer` will hold the string "12345" after the conversion.
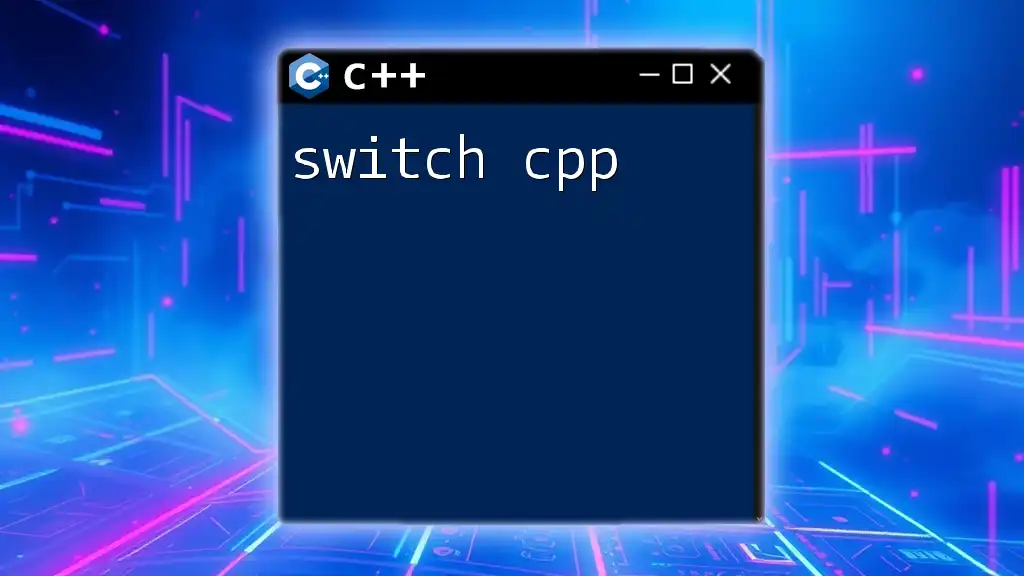
Implementing itoa in C++
Basic Implementation Example
Let’s look at a simple code snippet to see how `itoa` can be implemented.
#include <iostream>
#include <cstdlib> // include for itoa
int main() {
int number = 2023;
char buffer[20];
itoa(number, buffer, 10);
std::cout << "The number is: " << buffer << std::endl;
return 0;
}
In this code:
- We include the necessary header `<cstdlib>` to utilize the `itoa` function.
- We define an integer `number` with a value of 2023.
- The `itoa` function converts this number into a string stored in `buffer` using base 10.
- Finally, we print the resulting string to the console. The output will be: "The number is: 2023".
Handling Different Bases
The flexibility of `itoa` allows it to handle different number bases. For instance, you might need to convert a number to its binary, octal, or hexadecimal representation.
Consider this code:
#include <iostream>
#include <cstdlib>
int main() {
char buffer[20];
itoa(15, buffer, 2); // Converts to binary
std::cout << "Binary: " << buffer << std::endl;
itoa(15, buffer, 8); // Converts to octal
std::cout << "Octal: " << buffer << std::endl;
itoa(15, buffer, 10); // Converts to decimal
std::cout << "Decimal: " << buffer << std::endl;
itoa(15, buffer, 16); // Converts to hexadecimal
std::cout << "Hexadecimal: " << buffer << std::endl;
return 0;
}
In this code, the number `15` is converted into various bases:
- Binary: Outputs "1111"
- Octal: Outputs "17"
- Decimal: Outputs "15"
- Hexadecimal: Outputs "F"
Advantages of Using itoa
Using `itoa` provides many benefits:
- Performance and Efficiency: The conversion using `itoa` is generally faster and uses less memory than other string conversion alternatives, especially in scenarios where quick conversions are essential.
- Simplicity of Code: It helps keep the code clean and straightforward when converting integers to their string representation.
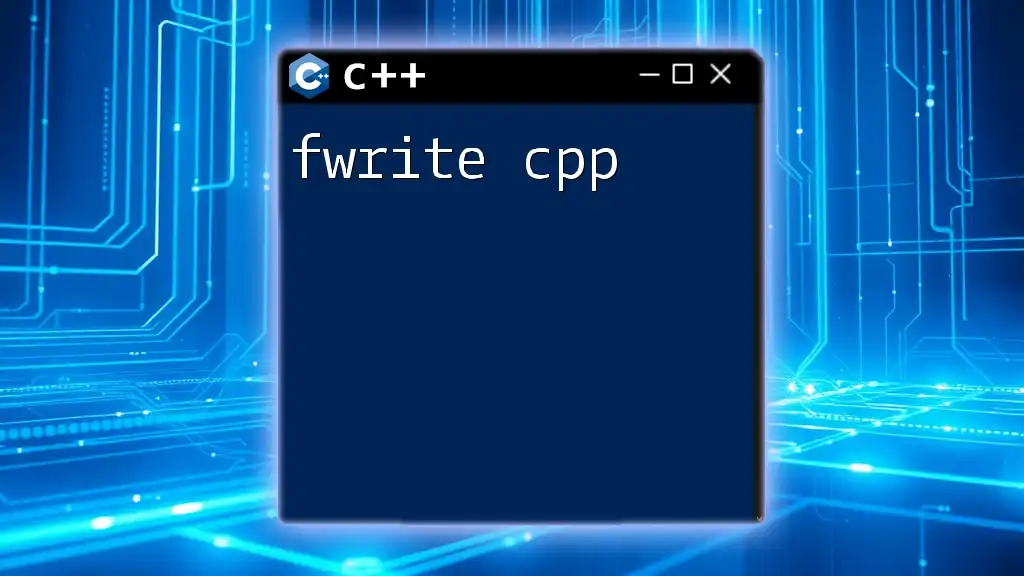
Limitations of itoa in C++
Despite its advantages, `itoa` has certain limitations:
- No Standard Definition in C++: While `itoa` is available in many compilers, it's not part of the C++ standard. This means your code might not be portable across different compilers or platforms.
- Buffer Size Management: You must ensure that the specified buffer is large enough to hold the resulting string, which can lead to potential buffer overflow issues if not managed properly.
- Portability Issues Across Different Compilers: Since `itoa` is non-standard, relying on it in your projects could lead to compatibility problems.
Alternatives to itoa
You can consider using alternatives like:
- `std::to_string`: Available in C++11 and later, it’s a safer and more portable method to convert integers to strings.
- `sprintf`: A traditional C-style function that formats and stores a series of characters and values into the buffer.
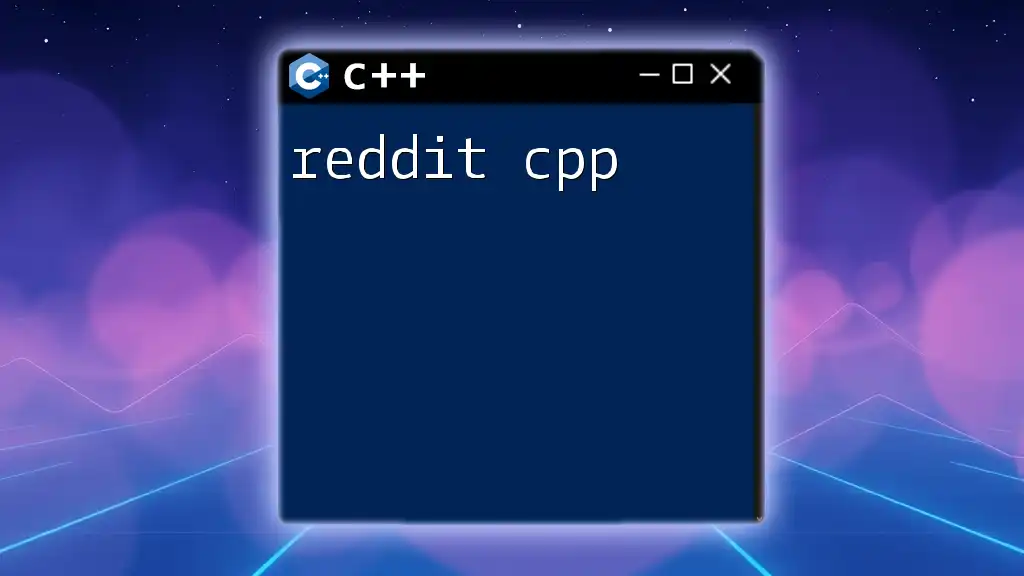
Best Practices for Using itoa in C++
To effectively use `itoa` in your code, keep these best practices in mind:
- Safe Buffer Management Techniques: Always ensure the buffer is appropriately sized. Use `snprintf` or similar functions if possible for better control over memory handling.
- Ensuring Compatibility Across Platforms: Test your code in multiple environments if you must use `itoa`, or consider using standard alternatives like `std::to_string`.
- When to Prefer itoa Over Other Methods: Use `itoa` when performance is critical, and you are confident about its implications on compatibility and memory management.
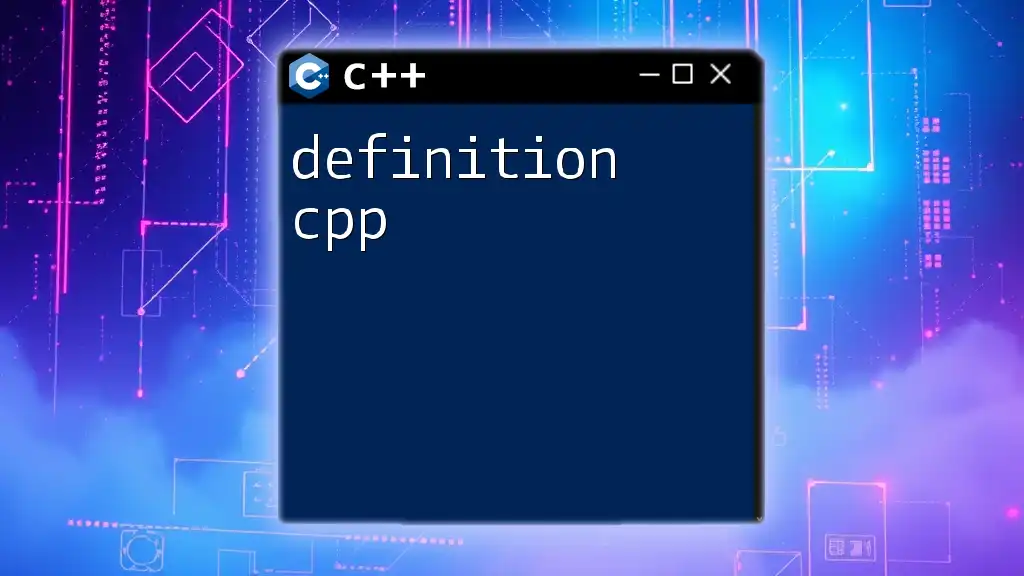
Conclusion
In summary, the `itoa` function is a powerful tool for converting integers to strings within C++. While it offers speed and efficiency, you should be aware of its limitations related to portability and buffer management. Exploring modern alternatives like `std::to_string` may provide a more robust solution for many applications. Embrace the capabilities of `itoa` in your C++ projects while adhering to best practices, and don't hesitate to experiment with this unique function to enhance your programming skill set.