The `scanf` function in C++ is used for inputting formatted data from the standard input (usually the keyboard) into specified variables.
Here’s a simple example of using `scanf` to read an integer and a floating-point number:
#include <stdio.h>
int main() {
int integer;
float floatingPoint;
printf("Enter an integer and a floating-point number: ");
scanf("%d %f", &integer, &floatingPoint);
printf("Integer: %d, Floating Point: %f\n", integer, floatingPoint);
return 0;
}
What is `scanf`?
`scanf` is a standard input function in C and C++ that allows you to read formatted data from the standard input, typically the keyboard. It plays a crucial role in input handling, enabling programmers to efficiently gather user inputs during program execution. While other methods, such as `cin`, exist for reading input in C++, `scanf` remains a popular choice due to its straightforward syntax and control over input formatting.
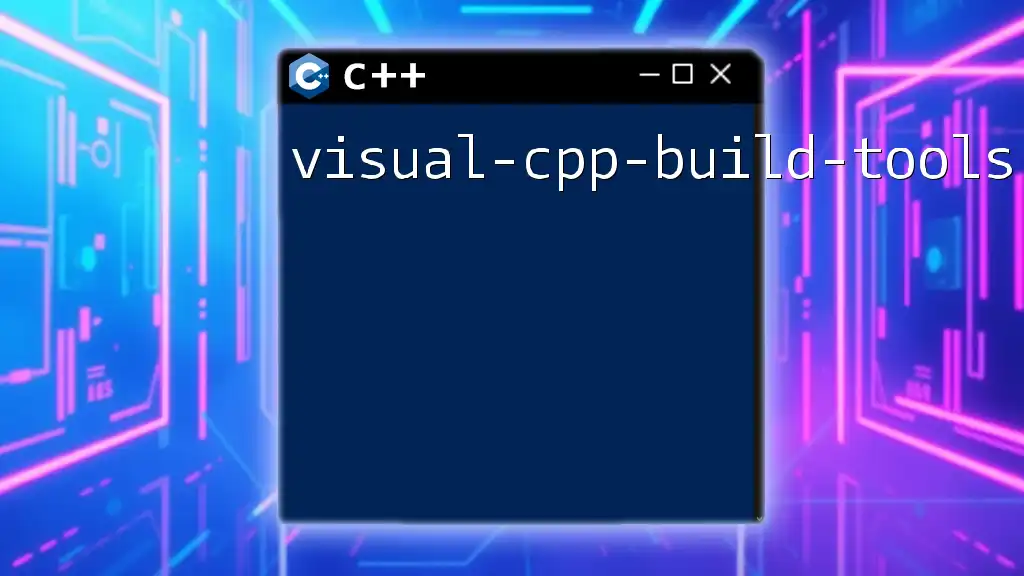
Why Use `scanf` in C++?
Using `scanf` in C++ can be advantageous in various situations:
- Performance: `scanf` can be more efficient than `cin` in some scenarios, especially where the input and output performance is critical.
- Formatted Input Control: `scanf` allows for fine-grained control over how input is read, using format specifiers to ensure the right data type is captured.
- Legacy Code: Many C programs still utilize `scanf`, making it useful to understand for transitioning between C and C++.
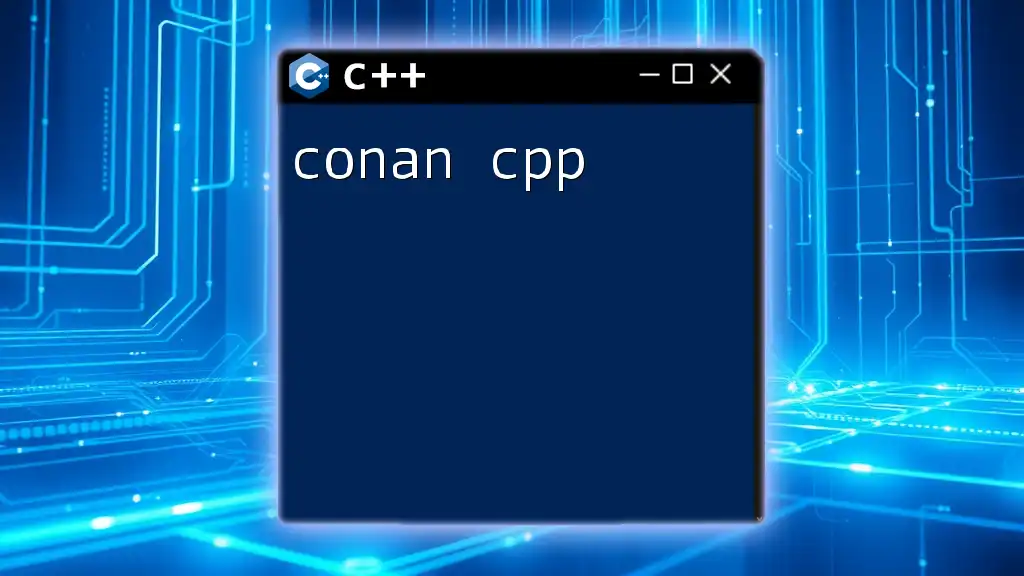
Basic Syntax of `scanf`
General Structure
The general structure of `scanf` involves a format string followed by a list of pointers to variables where the input will be stored. The syntax looks like this:
scanf("format_string", &variable1, &variable2, ...);
In this syntax:
- The `"format_string"` specifies the expected format of the input data (e.g., integers, floats, strings).
- The parameters listed after the format string are the addresses of the variables where the input values will be stored. The `&` operator is used to pass the address of a variable.
Format Specifiers
Format specifiers in `scanf` dictate the type of input expected. Here are some commonly used specifiers:
- `%d`: For reading integers
- `%f`: For reading floating-point numbers
- `%lf`: For reading double precision floating-point numbers
- `%s`: For reading strings (character arrays)
- `%c`: For reading a single character
Understanding these specifiers is essential for effectively using `scanf` to capture the correct type of data.
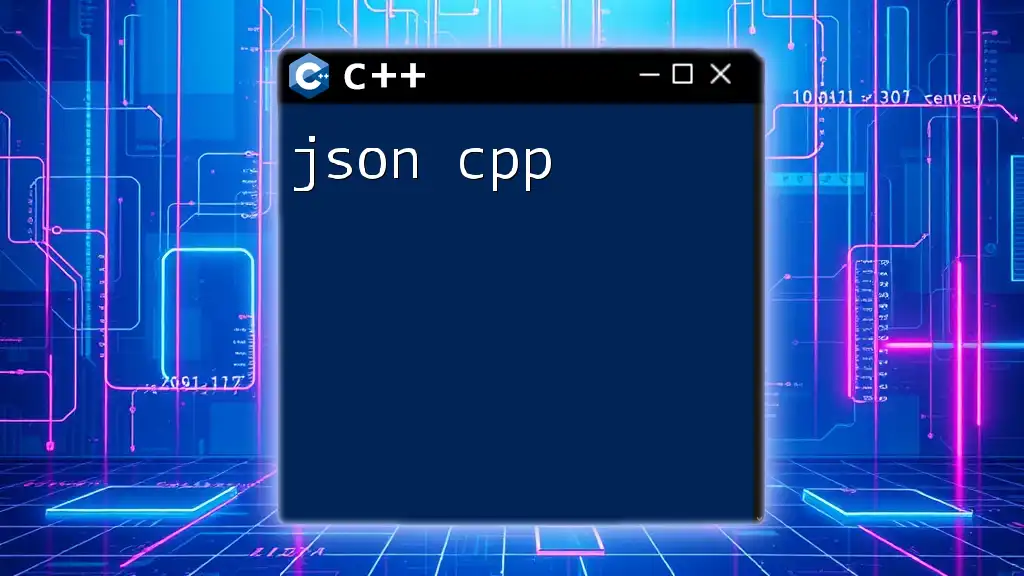
Reading Different Data Types with `scanf`
Reading Integers
To read an integer using `scanf`, you can use the `%d` format specifier. Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter an integer: ";
scanf("%d", &num);
cout << "You entered: " << num << endl;
return 0;
}
In this program, the user is prompted to enter an integer, which is then stored in the variable `num`.
Reading Floating Point Numbers
To capture a floating point number, use the `%f` specifier. Here’s how it works:
#include <iostream>
using namespace std;
int main() {
float num;
cout << "Enter a floating point number: ";
scanf("%f", &num);
cout << "You entered: " << num << endl;
return 0;
}
This snippet prompts the user to enter a floating point number and stores it in the variable `num`.
Reading Strings
When reading strings, you should use character arrays and the `%s` specifier. This example highlights the potential pitfalls, such as buffer overflow:
#include <iostream>
using namespace std;
int main() {
char str[100];
cout << "Enter a string: ";
scanf("%s", str);
cout << "You entered: " << str << endl;
return 0;
}
In this code, the user input is stored in the character array `str`. However, be cautious about buffer sizes to prevent overflow!
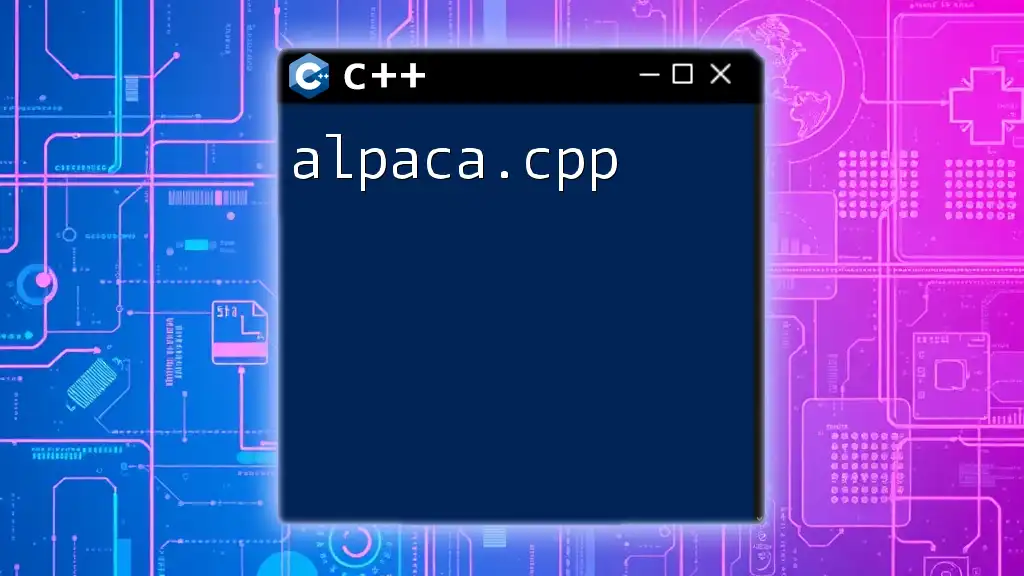
Handling Return Values from `scanf`
Understanding the Return Value
`scanf` returns the number of input items successfully matched and assigned. If input fails, it returns a value less than the number of arguments passed. This return value can be crucial for validating user input.
Example of Handling Input Errors
You can incorporate error handling to ensure the input was valid:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter an integer: ";
if (scanf("%d", &num) != 1) {
cout << "Invalid input!" << endl;
} else {
cout << "You entered: " << num << endl;
}
return 0;
}
In this example, the program checks whether the input was successful and provides an error message if it wasn't.
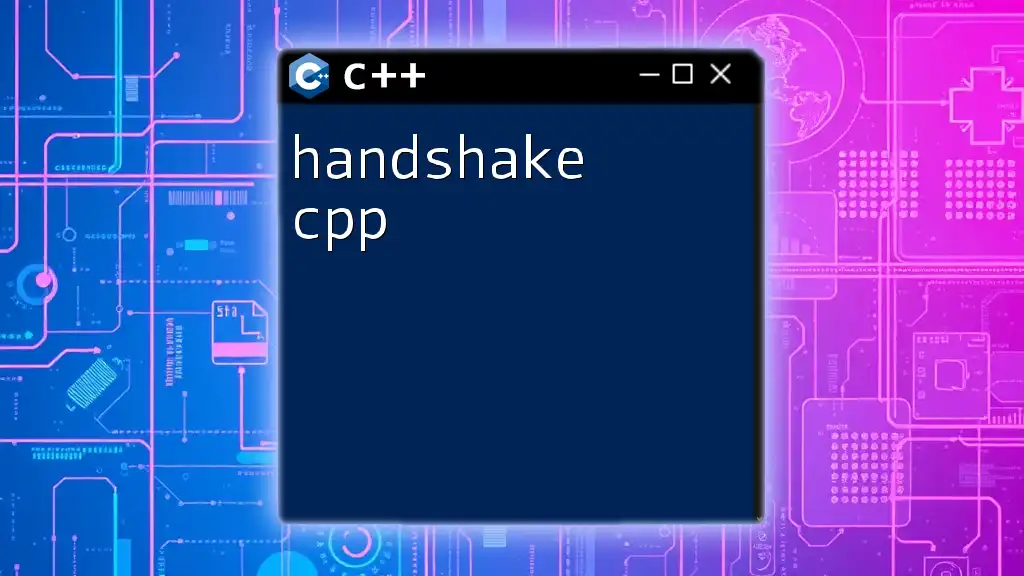
Common Pitfalls and Errors with `scanf`
Buffer Overflow Issues
A common pitfall when using `scanf` is buffer overflow, particularly when reading strings. If the user inputs more characters than the buffer can hold, it can lead to undefined behavior. Best practices include:
- Always define a fixed size for character arrays.
- Use `%ns` specifier (where `n` is the maximum number of characters to read) to limit the size of input.
Handling Whitespace in Input
`scanf` effectively ignores whitespace between inputs, which can be both a feature and a challenge. For instance, when reading multiple values, `scanf` can be quite efficient:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two integers: ";
scanf("%d %d", &a, &b);
cout << "You entered: " << a << " and " << b << endl;
return 0;
}
In this snippet, the user can input two integers separated by whitespace, and both values will be stored correctly in the respective variables.
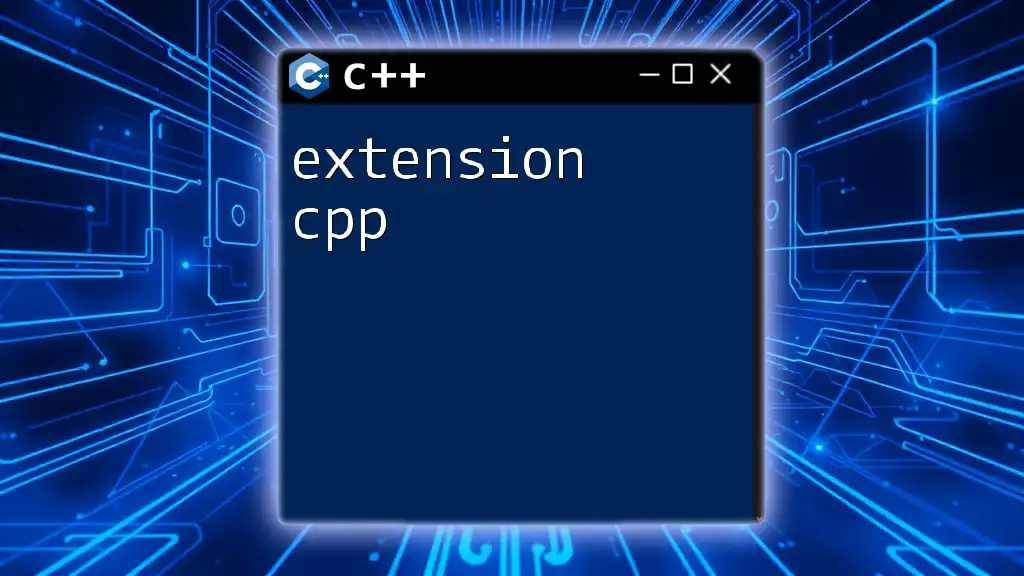
Alternatives to `scanf` in C++
Using `cin` for Input
While `scanf` is powerful, the C++ `cin` method can be a more robust option in many cases. `cin` utilizes operator overloading, allowing much easier handling of different data types without needing format specifiers.
Comparison of `scanf` vs `cin`
Understanding when to use `scanf` versus `cin` is important:
-
`scanf` Advantages:
- Performance-oriented for reading large inputs.
- Fine-grained control over the input format.
-
`cin` Advantages:
- Type safety and ease of use.
- Better handling of input errors with built-in mechanisms.
Ultimately, the choice depends on the specific requirements of the program and the developer's familiarity with the input methods.
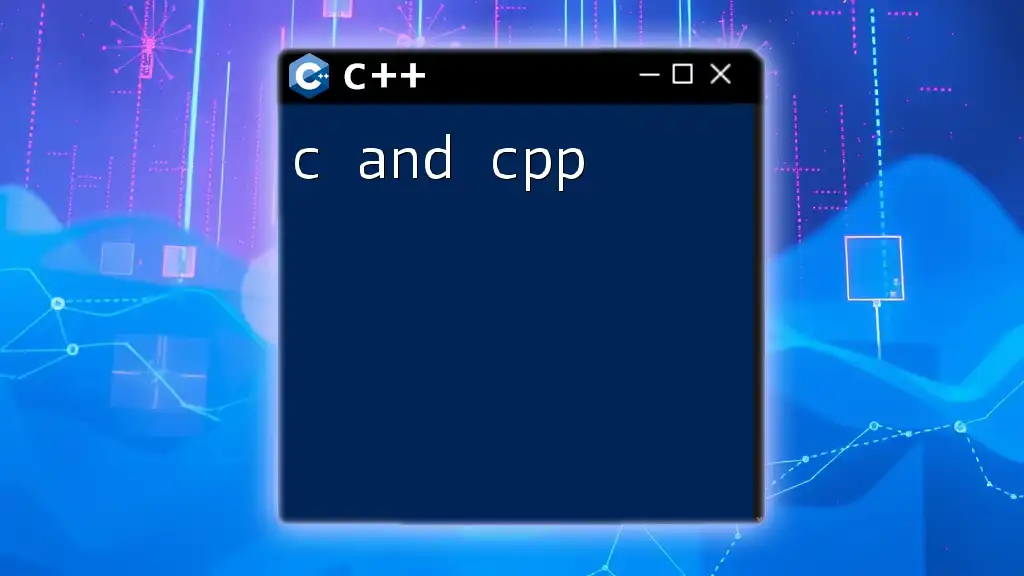
Conclusion
In this guide, we explored the `scanf` function in C++, covering its definition, syntax, usage with different data types, error handling, and common pitfalls. Understanding `scanf` in C++ is crucial for effective input handling and allows for more control over user interactions in your programs. By practicing the concepts discussed, you can enhance your programming skills and efficiently manage input in C++.
Encourage yourself to experiment with `scanf`, and supplement your learning with additional resources such as books and online tutorials to deepen your understanding of formatted input in C++.