YAML (YAML Ain't Markup Language) is a human-readable data serialization format commonly used for configuration files, and when integrated with C++, it allows for easy management and parsing of complex data structures.
Here’s a simple example of using a YAML file with C++:
#include <iostream>
#include <yaml-cpp/yaml.h>
int main() {
YAML::Node config = YAML::LoadFile("config.yaml");
std::cout << "Host: " << config["host"].as<std::string>() << std::endl;
return 0;
}
Make sure to create a `config.yaml` file containing:
host: localhost
What is YAML?
YAML, which stands for YAML Ain't Markup Language, is a human-readable data serialization language that is primarily used for configuration files and data exchange between languages with different data structures. Its simple syntax makes it accessible, which is one of the reasons it has become popular in modern programming.
YAML supports complex data structures, such as lists and maps, allowing it to represent hierarchical data conveniently. This makes it an excellent choice for configuration files due to its clarity and ease of use.
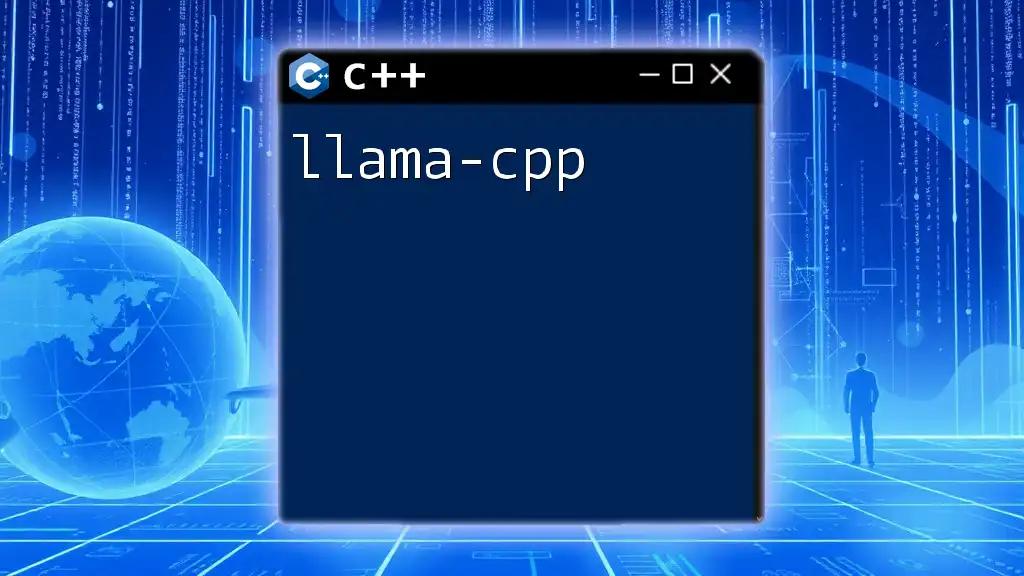
What is C++?
C++ is a powerful, versatile programming language that supports procedural, object-oriented, and generic programming paradigms. It is widely used in system/software, game development, and applications requiring high-performance graphics.
The combination of C++’s capabilities with YAML’s intuitive nature creates a compelling toolkit for developers, especially those who need to manage configuration settings dynamically.
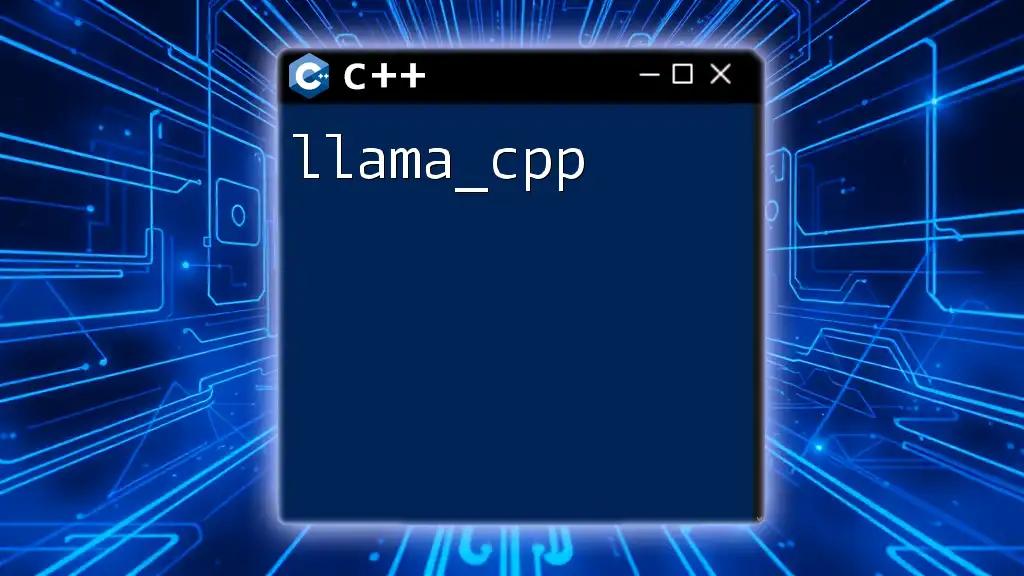
The Intersection of YAML and C++
Combining YAML and C++ empowers developers to manage application configurations effortlessly. Using YAML allows C++ applications to remain flexible and adaptable by simply updating a configuration file without the need for recompilation.
Why Combine YAML with C++?
- Human Readability: YAML files are easy to read and understand compared to other formats like JSON or XML. This readability improves maintainability.
- Structured Data: YAML supports complex data structures, which allows developers to organize configurations better.
- Dynamic Changes: With YAML, you can change configurations on the fly, enhancing the flexibility of applications.
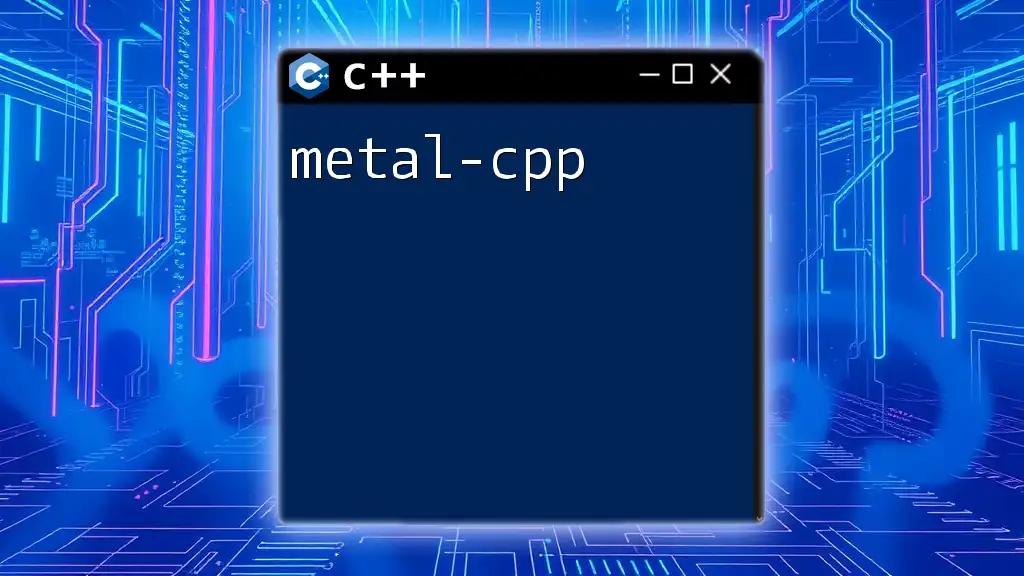
Setting Up Your Environment for Using YAML with C++
Required Libraries
To work with YAML in C++, you will primarily use yaml-cpp, an open-source YAML parser and emitter. It allows for easy reading and writing of YAML files directly in your C++ applications. You can explore the official repository for more details and documentation at [yaml-cpp GitHub](https://github.com/jbeder/yaml-cpp).
Installation of yaml-cpp
Step-by-Step Guide
Installing yaml-cpp can be accomplished using various methods, including package managers like vcpkg or Conan and building it from the source.
Here's a simple way to build it from source:
git clone https://github.com/jbeder/yaml-cpp.git
cd yaml-cpp
mkdir build && cd build
cmake ..
make
sudo make install
By following these commands, you will set up yaml-cpp and make it available for your C++ development.
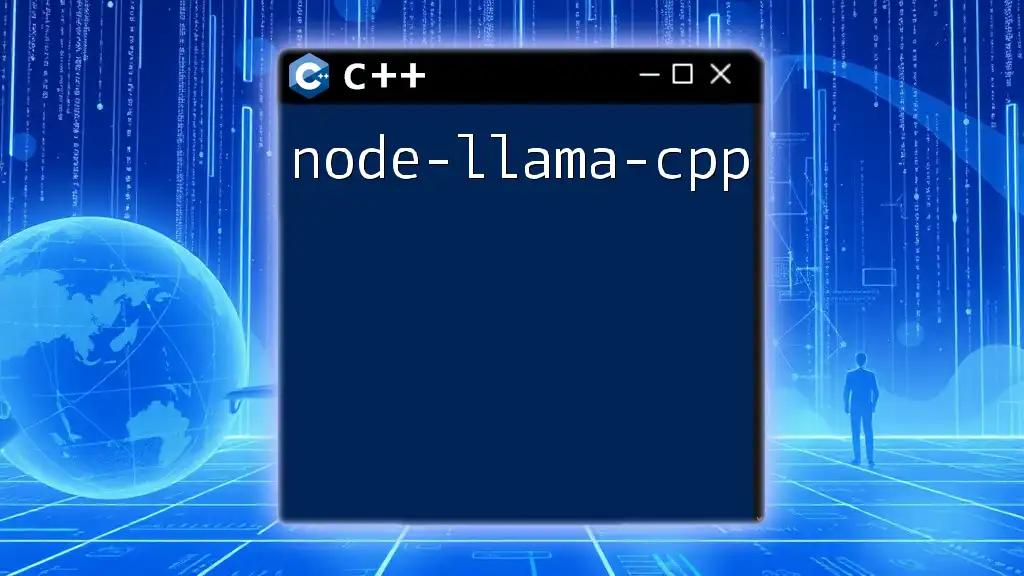
Core Concepts of YAML
YAML Syntax Basics
Understanding YAML syntax is essential for effective usage. The syntax is structured around key-value pairs, where indentation denotes hierarchy. Here’s what you need to know:
- Indentation: YAML uses white space for formatting, and consistent indentation is crucial.
- Data Types: YAML supports scalar values (strings, integers), sequences (arrays), and mappings (objects).
- Human-Friendly: YAML’s design prioritizes readability, making it an ideal choice for configuration files.
Example of YAML Syntax:
person:
name: John Doe
age: 30
hobbies:
- reading
- hiking
YAML Data Types Explained
YAML supports various data types, which cater to different programming needs:
- Scalars: These are simple values like strings or integers.
- Sequences: Represented by the dash (`-`), sequences allow the representation of lists or arrays.
- Mappings: Represented by key-value pairs, used for objects/dictionaries.
Example of Various Data Types in YAML:
fruits:
- apple
- banana
- orange
details:
weight: 1.5
isFresh: true

Integrating YAML with C++
Loading YAML in C++
Loading YAML files in C++ using yaml-cpp is straightforward. It allows you to access data by navigating the hierarchical structure of the YAML document.
Example Code for Loading a YAML File:
#include <yaml-cpp/yaml.h>
#include <iostream>
int main() {
YAML::Node config = YAML::LoadFile("config.yaml");
std::string name = config["person"]["name"].as<std::string>();
std::cout << "Name: " << name << std::endl;
return 0;
}
In this example, we load a YAML file named `config.yaml` and access the "name" key from the "person" mapping.
Accessing Data in YAML
Accessing data in YAML involves understanding the hierarchy of your YAML structure. You can use the `.as<Type>()` method to retrieve specific types, helping to ensure that your program receives the correct data.
Error Handling for Missing Keys: You should always add checks for existence to prevent runtime errors, providing a smoother experience.
Writing YAML in C++
Creating and saving YAML files in C++ is equally simple with yaml-cpp. Writing data is as easy as constructing a YAML node and outputting it to a file.
Example Code for Writing to a YAML File:
YAML::Node output;
output["person"]["name"] = "Jane Doe";
output["person"]["age"] = 28;
std::ofstream outFile("output.yaml");
outFile << output;
outFile.close();
This snippet constructs a YAML node with information about a person and saves it to `output.yaml`.

Advanced YAML Features for C++
Using Custom Data Types
Serializing and Deserializing Custom Classes: You can extend yaml-cpp to handle custom types. By defining how to convert your custom classes into YAML and vice versa, you maintain a seamless integration.
Example of Serializing a Custom Type:
struct Person {
std::string name;
int age;
};
YAML::Emitter& operator<<(YAML::Emitter& out, const Person& person) {
out << YAML::BeginMap
<< YAML::Key << "name" << YAML::Value << person.name
<< YAML::Key << "age" << YAML::Value << person.age
<< YAML::EndMap;
return out;
}
With this setup, the `operator<<` function defines how to serialize a `Person` object to YAML.
Best Practices for Using YAML with C++
- Structuring Larger YAML Documents: Maintain clarity by organizing complex data hierarchically and documenting structures in comments.
- Validating YAML Files: Use tools to validate your YAML syntax before parsing with C++ to prevent errors.
- Performance Considerations: Be mindful of the size of YAML files; large documents can impact performance.

Debugging Common Issues
Troubleshooting YAML Parsing Errors
Common YAML parsing errors typically relate to improper formatting or unexpected data types. Ensure that your YAML files have consistent indentation and correct data structures.
Logging Errors in C++
Logging parsing errors effectively can help in diagnosing issues quickly. A simple logging mechanism can be built to capture exceptions and unexpected behaviors during YAML parsing.
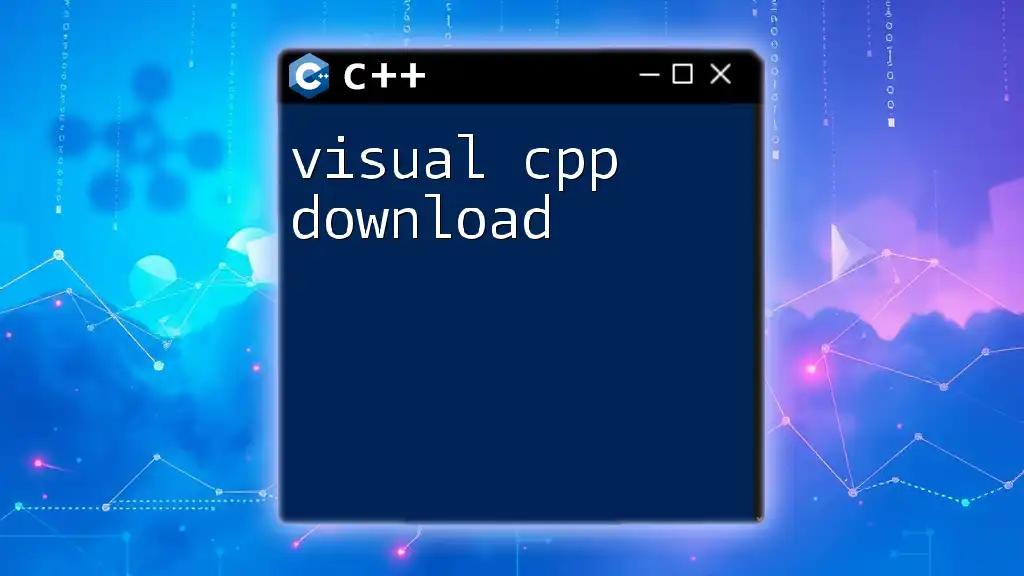
Conclusion
In summary, leveraging YAML with C++ provides an organized and dynamic approach to configuration management that enhances the flexibility of applications. Understanding how to load, process, and write YAML files effectively can transform how developers manage application settings and configurations.
As you explore the integration of YAML with C++, you’ll find that this powerful combination can significantly streamline your development workflow. The potential for using YAML in diverse applications continues to grow, solidifying its role in modern programming. Embrace this synergy and elevate your C++ projects with YAML today!