"Programiz C++ is an online platform that provides concise tutorials and examples to help learners quickly master C++ commands with practical coding snippets."
Here's a simple code snippet using C++ commands:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Getting Started with Programiz C++ Compiler
Programiz C++ Compiler is a powerful online tool that allows programmers of all skill levels to write, compile, and run their C++ code quickly and efficiently.
Introduction to the Compiler
The Programiz Compiler provides a user-friendly interface that caters to both beginners and experienced developers. With its rich feature set, users can focus on learning C++ without worrying about the complexities of setting up a local environment. Key features include:
- Simplicity: The interface is intuitive, making it easy to navigate.
- In-browser Execution: Run code directly in the browser without any installation.
- Output Section: See the output of your code instantly.
How to Access Programiz C++ Compiler
Accessing the Programiz C++ compiler is straightforward. Follow these steps:
-
Online Access: Simply visit the Programiz website, where the compiler interface can be accessed directly through the browser.
-
Installation: If available, you can download the compiler for offline use. However, the online tool is ideal for immediate practice.
Navigating the Programiz Compiler Interface
Upon entering the compiler interface, you’ll see:
- An editor area for typing your code.
- An output area where results are displayed after execution.
- Buttons for compiling and executing the code.
Understanding these elements will help maximize your coding efficiency and improve your learning experience.
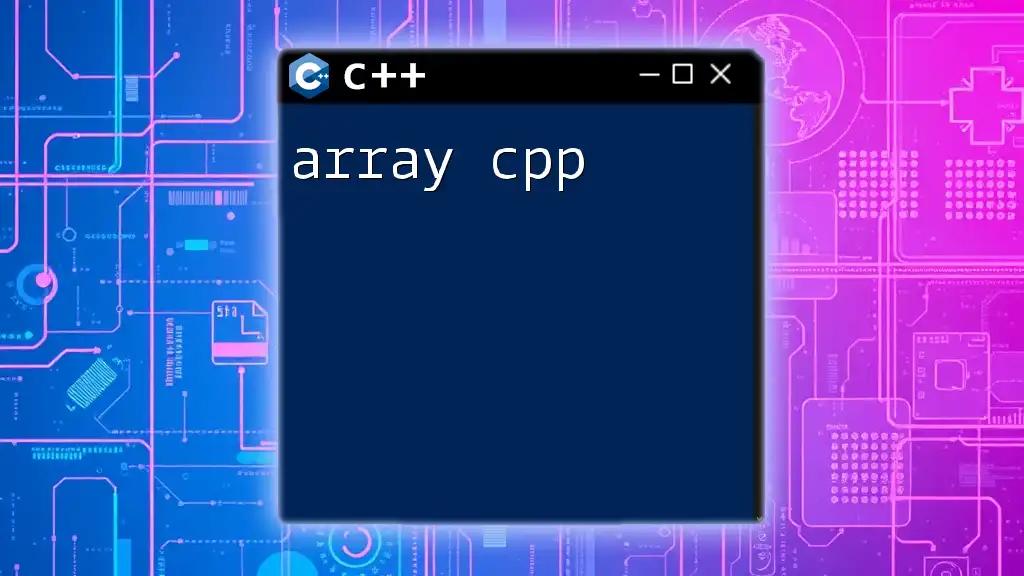
Basic Concepts of C++ through Programiz
Data Types in C++
In C++, data types are used to determine the type of data a variable can hold. The fundamental data types include:
- int: Represents integers.
- float: Represents floating-point numbers.
- char: Represents a single character.
- bool: Represents true or false values.
Example Code Snippet: Data Type Declaration
int number = 10;
float price = 99.99;
char grade = 'A';
bool isPassed = true;
Variables and Constants
Variables in C++ are used to store data that can change, while constants hold fixed values which cannot be altered once defined.
Example Code Snippet: Using Variables
int age = 25; // Variable
const float PI = 3.14; // Constant
Input and Output in C++
Input and output operations in C++ are commonly handled using `cin` and `cout`. This makes it easy for users to interact with their programs.
Example Code Snippet: Simple Input/Output
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "Your age is " << age << endl;
return 0;
}
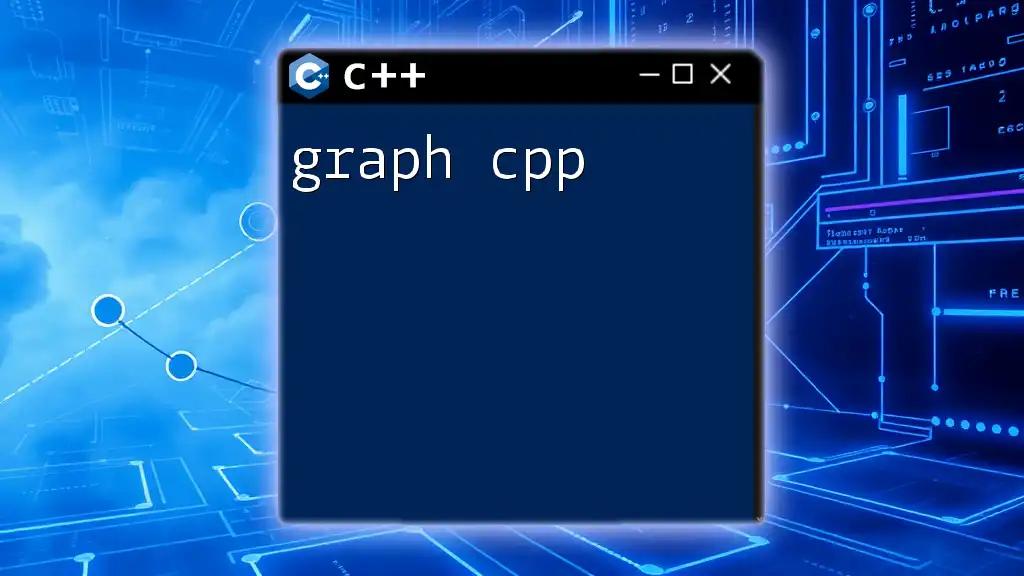
Control Flow in C++ Using Programiz
Conditional Statements
Conditional statements allow your program to take different paths based on certain conditions.
- if Statement: Executes a block of code if the condition is true.
- else Statement: Executes an alternative block if the condition is false.
- switch Statement: Adds multiple conditions through case checks.
Example Code Snippet: Conditional Logic
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (number > 0) {
cout << "Positive number" << endl;
} else if (number < 0) {
cout << "Negative number" << endl;
} else {
cout << "Number is zero" << endl;
}
return 0;
}
Loops in C++
Loops are essential for executing a block of code multiple times.
- for Loop: Used when the number of iterations is known.
- while Loop: Continues until a specified condition is false.
- do-while Loop: Executes the code block at least once before checking the condition.
Example Code Snippet: Looping Constructs
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; i++) {
cout << i << endl; // Prints numbers from 0 to 4
}
return 0;
}
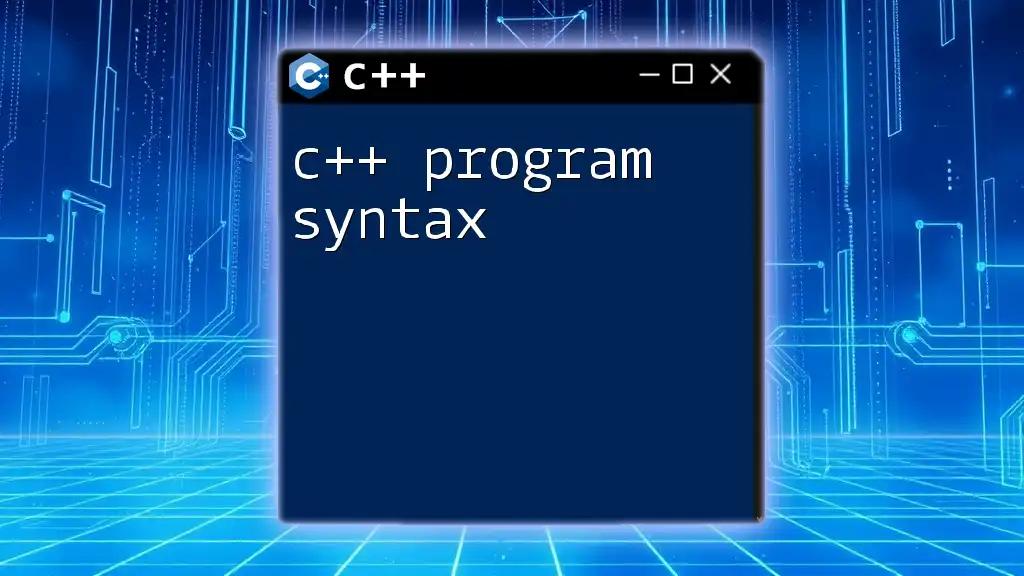
Functions in C++
Understanding Functions
Functions are blocks of code designed to perform a specific task and can be reused throughout your program. They enhance modularity and readability.
Example Code Snippet: Function Declaration and Definition
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, World!" << endl;
}
int main() {
greet(); // Calling the function
return 0;
}
Function Overloading
C++ allows multiple functions with the same name but with different parameters. This is known as function overloading.
Example Code Snippet: Demonstrating Function Overloading
#include <iostream>
using namespace std;
void display(int number) {
cout << "Number: " << number << endl;
}
void display(float number) {
cout << "Float number: " << number << endl;
}
int main() {
display(5); // Calls display(int)
display(5.5f); // Calls display(float)
return 0;
}
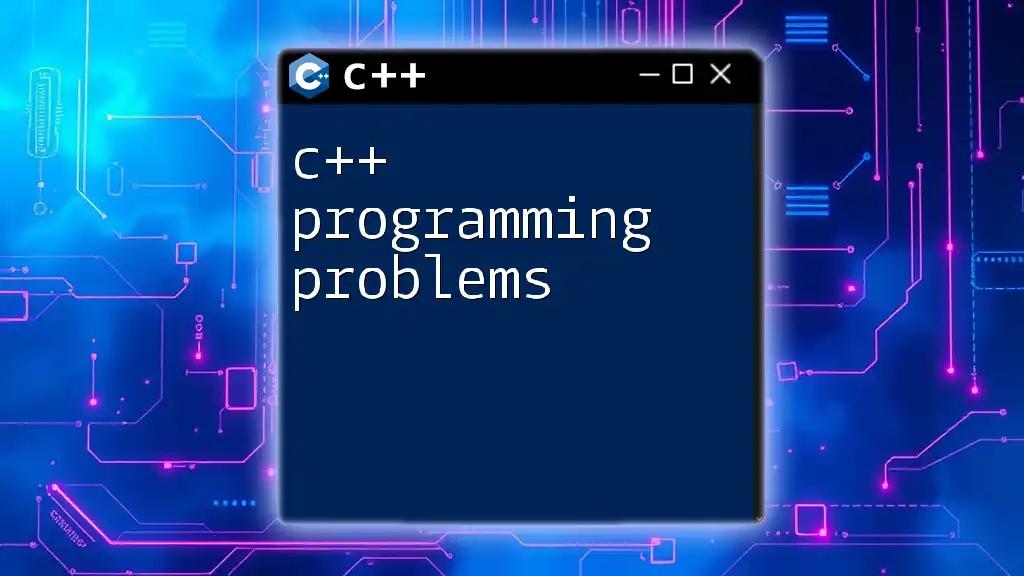
Object-Oriented Programming with C++
Introduction to OOP Concepts in C++
Object-Oriented Programming (OOP) is fundamental in C++. It promotes code reusability and organization and is built around concepts such as:
- Encapsulation: Keeping data private and providing public access.
- Inheritance: Deriving new classes from existing ones to implement shared behavior.
- Polymorphism: Allowing objects to be treated as instances of their parent class.
Classes and Objects
Classes serve as blueprints for creating objects. An object, in turn, is an instance of a class.
Example Code Snippet: Simple Class Implementation
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog; // Creating an object
myDog.bark(); // Calling the method
return 0;
}
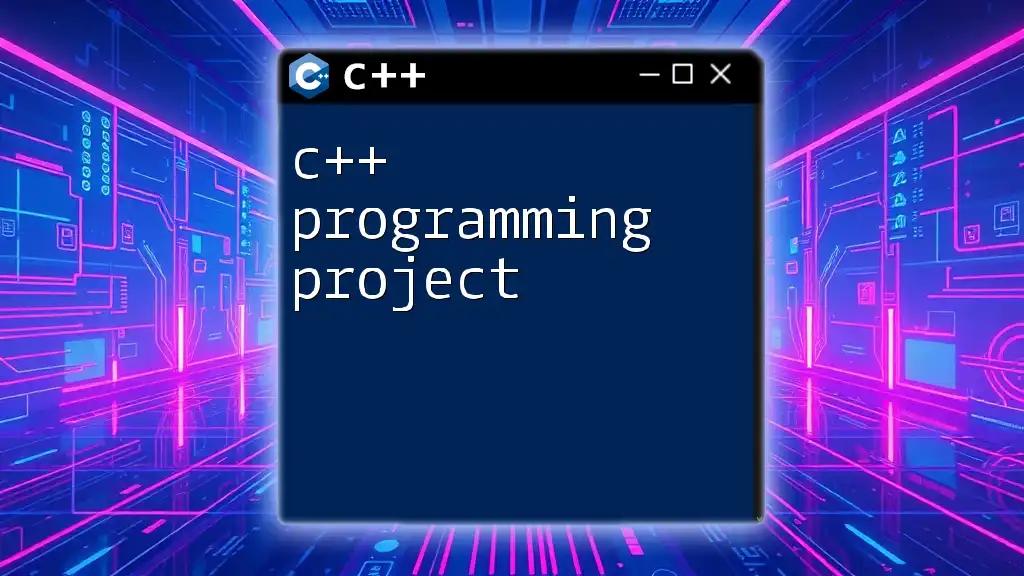
Advanced Concepts in C++ Using Programiz
Templates
Templates in C++ allow you to write generic and reusable code.
Example Code Snippet: Using Function Templates
#include <iostream>
using namespace std;
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << add<int>(5, 10) << endl; // Outputs 15
cout << add<float>(5.5, 4.5) << endl; // Outputs 10
return 0;
}
Error Handling
C++ provides exception handling mechanisms to manage errors gracefully, ensuring that your program runs smoothly instead of crashing.
Example Code Snippet: Try-Catch Block
#include <iostream>
using namespace std;
int main() {
try {
throw runtime_error("An error occurred");
} catch (const runtime_error &e) {
cout << e.what() << endl; // Catching the exception
}
return 0;
}
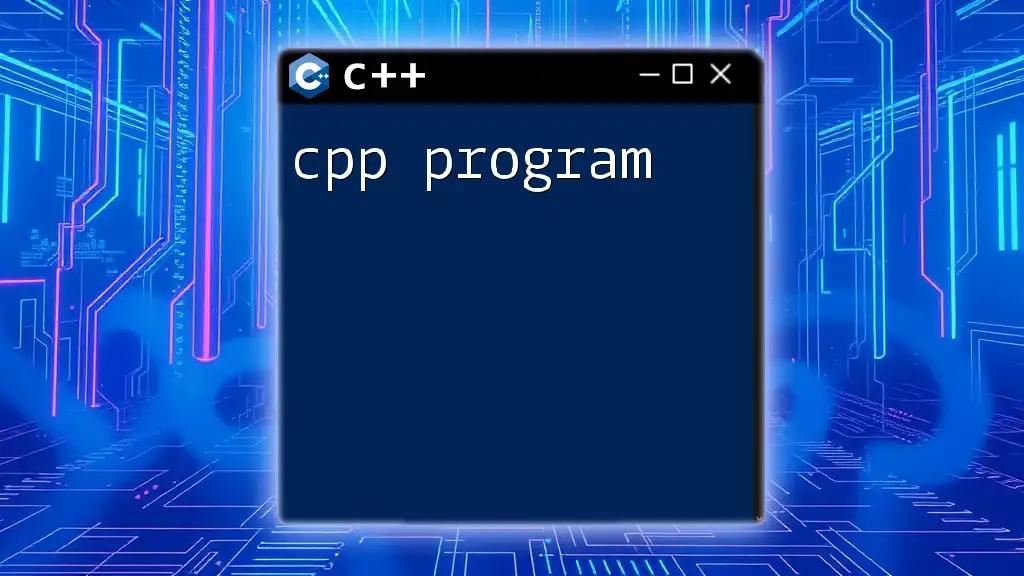
Exploring Programiz Resources for C++ Learning
Self-Learning Materials Available on Programiz
Programiz offers various tutorials and guides that cover a wide range of topics in C++. From the basics of syntax to advanced concepts such as STL (Standard Template Library), these resources are invaluable for students and professionals alike.
Community Support and Forums
Engaging with the Programiz community can amplify your learning. Sharing experiences, troubleshooting problems, and collaborating on projects not only enhances your understanding of C++ but also fosters a supportive environment.
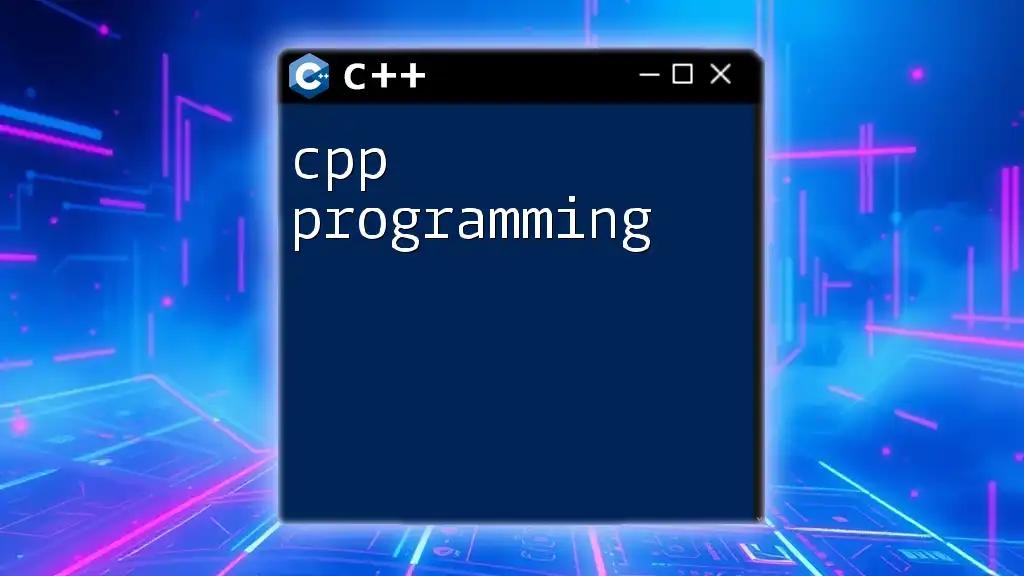
Conclusion
Learning Programiz C++ offers a streamlined path to master one of the most essential programming languages today. With its accessible compiler, fundamental teachings, and active community, Programiz serves as an excellent platform for both novices and seasoned programmers.
Call to Action
Start your programming journey with Programiz today—experiment, code, and explore the vast potential of C++. The power of learning C++ awaits you!
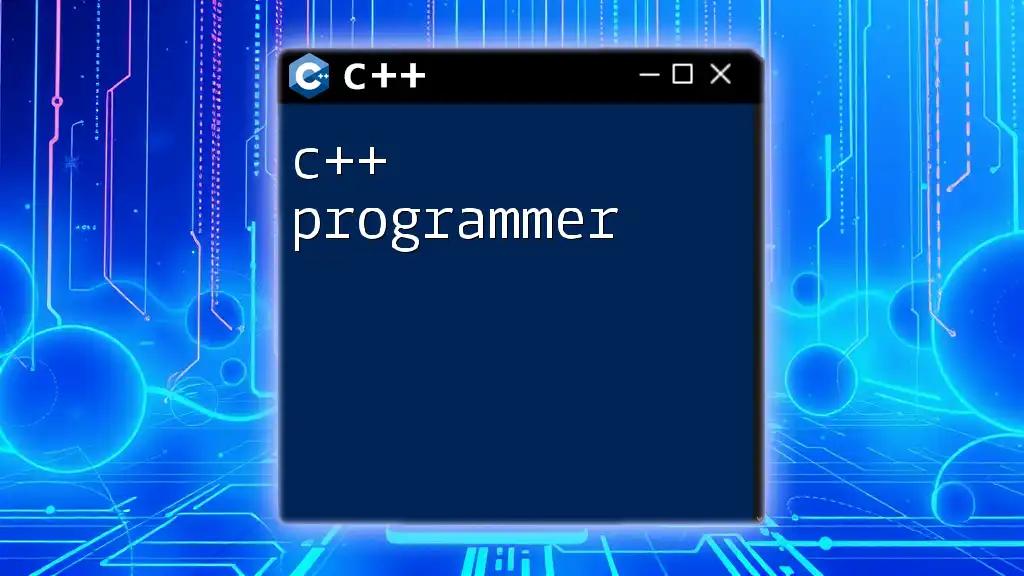
Additional Resources
- Recommended Books and Courses: Consider complementing your learning with highly-rated C++ textbooks and online courses to deepen your understanding.
- Links to Other C++ Learning Platforms: Explore other platforms to broaden your exposure to different teaching methods.
- Glossary of Key C++ Terms and Acronyms: Familiarize yourself with commonly used terminology to navigate discussions and documentation seamlessly.