The `pow` function in C++ is used to calculate the power of a number by raising the base to the exponent provided.
Here's an example code snippet demonstrating the use of `pow`:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Understanding the Concept of Powers and Exponents
The concept of powers and exponents is fundamental in both mathematics and programming. An exponent is a number that indicates how many times to multiply the base number by itself. For example, in the expression \(2^3\), \(2\) is the base, and \(3\) is the exponent, which means \(2 \times 2 \times 2 = 8\).
In programming, specifically in C++, exponents are often required in calculations such as algorithms involving physics, finance, or any calculations that require mathematical modeling. This underscores the significance of the power function in C++, especially the `pow` function in the `<cmath>` library.
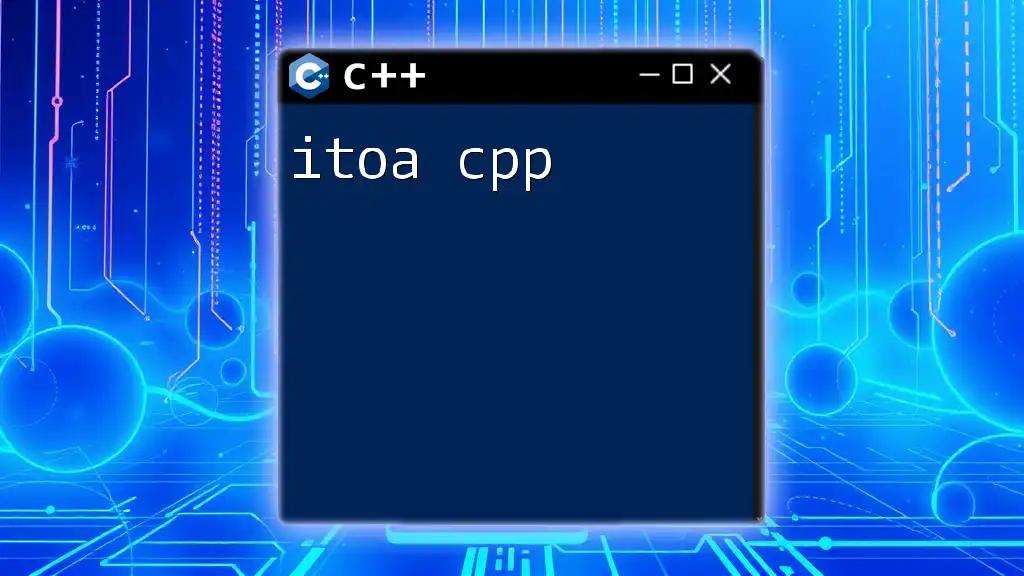
The pow Function in C++
What is the pow Function in C++?
The `pow` function is a built-in mathematical function that computes the power of a number. It raises a base to the exponent specified. Understanding the syntax and usage of the `pow` function is essential for effective programming in C++.
The syntax of the `pow` function is as follows:
double pow(double base, double exponent);
This function takes two parameters: the base to be raised and the exponent to which it is raised.
Parameters of the pow Function
Base: This represents the number that you want to raise to a power. It can be a floating-point number or an integer.
Exponent: This is the power to which the base number is raised. Similar to the base, it can also be an integer or floating-point.
Return Value of pow
The return value of the `pow` function is a double, which represents the result of raising the base to the exponent. Understanding the return value, especially when dealing with different data types, is crucial as it affects the precision of calculations.

Using the pow Function in C++
Basic Examples of pow in C++
To understand how to use the `pow` function effectively, consider the following basic examples.
Example 1 demonstrates calculating \(2\) raised to the power of \(3\):
#include <iostream>
#include <cmath>
int main() {
std::cout << "2^3 = " << pow(2, 3) << std::endl; // Output: 8
return 0;
}
In this example, the output confirms that \(2\) raised to the power \(3\) equals \(8\).
Example 2 shows how to use the `pow` function with floating-point numbers:
#include <iostream>
#include <cmath>
int main() {
std::cout << "3.5^2 = " << pow(3.5, 2) << std::endl; // Output: 12.25
return 0;
}
Here, \(3.5\) raised to the power \(2\) results in \(12.25\).
Advanced Use Cases
The `pow` function can handle a wide array of other mathematical computations. For example, calculating the power of a negative number yields different results:
#include <iostream>
#include <cmath>
int main() {
std::cout << "(-2)^3 = " << pow(-2, 3) << std::endl; // Output: -8
std::cout << "(-2)^2 = " << pow(-2, 2) << std::endl; // Output: 4
return 0;
}
In this snippet, \((-2)^3\) returns \(-8\) while \((-2)^2\) yields \(4\), showcasing how the sign of the base influences the result based on the nature of the exponent.
Using `pow` in complex mathematical expressions enhances its utility. For example, you can compute the value of an expression involving multiple operations, such as the following:
#include <iostream>
#include <cmath>
int main() {
double a = 2.0;
double b = 3.5;
double result = pow(a, b) + pow(b, a); // (2^3.5 + 3.5^2)
std::cout << "Result = " << result << std::endl; // Output can vary
return 0;
}
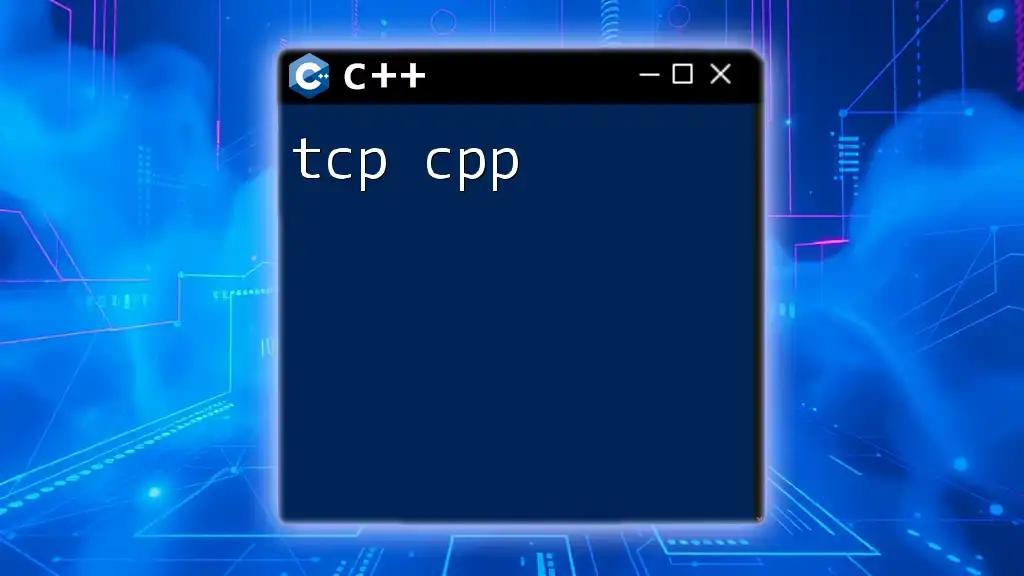
Common Errors and How to Avoid Them
Type Mismatches
When working with the `pow` function, developers may encounter type mismatch errors. It's essential to ensure that the arguments passed to `pow` are of compatible types (typically `double`). Mismatched types can lead to unexpected results or compilation issues.
Negative and Zero Exponents
Understanding how to handle negative and zero exponents is crucial. According to mathematical principles:
- Any number raised to the power of zero equals one.
- A non-zero number raised to a negative exponent is equivalent to \(1\) divided by that number raised to the absolute value of the exponent.
Here's an example demonstrating these principles:
#include <iostream>
#include <cmath>
int main() {
std::cout << "2^0 = " << pow(2, 0) << std::endl; // Output: 1
std::cout << "2^-3 = " << pow(2, -3) << std::endl; // Output: 0.125
return 0;
}

Comparison between pow Function and Other Methods
Pitfall of Using the Operator for Power Calculation
While one might consider using the `**` operator in other programming environments to perform power calculations, C++ does not support this syntax. Relying on an unsupported operator can result in errors or unintended outcomes in C++.
When to Use the pow Function Over Manual Calculations
The `pow` function is optimized for various input cases and manages floating-point precision issues better than manual multiplications. For example, it can handle larger exponents efficiently without requiring the developer to implement custom loops.
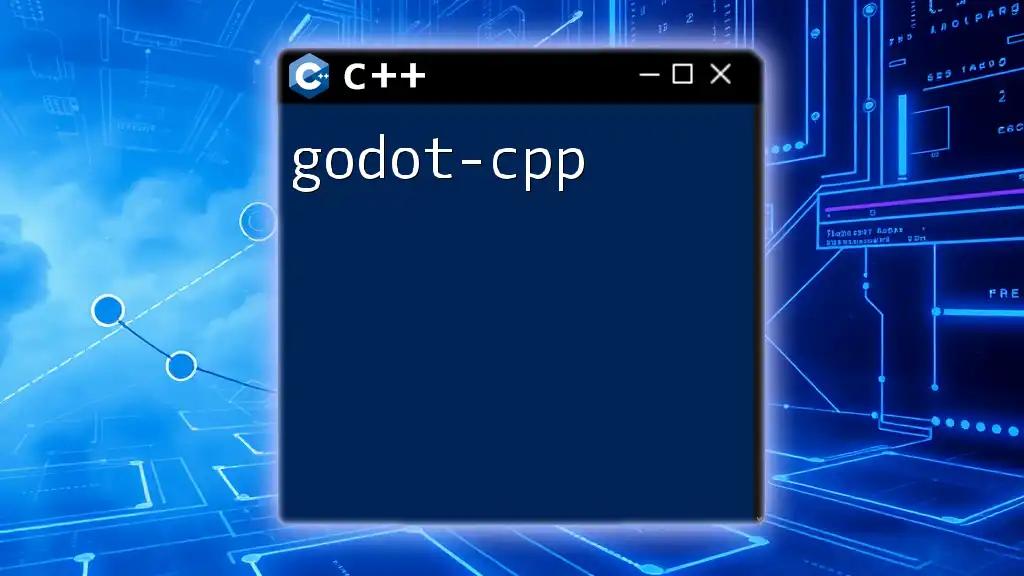
Understanding C++ Math Library
Including the Required Header Files
To utilize the `pow` function, you must include the header `<cmath>` in your program. This inclusion is critical to access the various mathematical functions provided by C++. Here's an example of including the required headers:
#include <iostream>
#include <cmath> // Necessary for pow function
// Rest of your code here
Related C++ Math Functions
The `<cmath>` library includes several essential mathematical functions beyond `pow`. Functions like `sqrt`, `exp`, and `log` can also be crucial in mathematical programming tasks.
For instance, calculating the square root can be done using `sqrt`:
#include <iostream>
#include <cmath>
int main() {
double value = 16.0;
std::cout << "Square root of 16 = " << sqrt(value) << std::endl; // Output: 4
return 0;
}

Performance Considerations with pow Function
Optimization Tips for Power Calculations
When performance metrics are critical, especially in large-scale applications, it’s vital to consider optimization strategies. For example, pre-calculating certain powers that will be used frequently can enhance performance and decrease computation time drastically.
Exploring Alternatives for Performance-Sensitive Applications
In performance-sensitive applications, where the base and exponent are known and fixed, consider using multiplication rather than `pow`. For example, if querying \(2^{10}\), it may be faster to compute directly:
int result = 1;
for(int i = 0; i < 10; i++) {
result *= 2;
}
This can reduce overhead if the calculation is performed frequently.

Conclusion
In conclusion, the `pow` function in C++ is a versatile tool for performing exponentiation across various applications in mathematics and programming. By understanding its usage, parameters, return values, and performance implications, developers can take full advantage of this essential function in their C++ code.
Further Resources
To deepen your understanding of the `pow` function and other mathematical operations, consider exploring further readings, official C++ documentation, or community forums. Engaging with these resources can provide additional insights and support you in your C++ development journey.