"Eclipse C++ (Eclipse CDT) is an integrated development environment that simplifies C++ programming by providing features like syntax highlighting, code completion, and debugging tools for faster development."
Here's a simple code snippet that demonstrates a basic C++ "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Eclipse CPP?
Eclipse CPP refers to the integration of the Eclipse IDE with C/C++ Development Tooling (CDT) to support C++ programming. Eclipse is a widely used IDE known for its versatility and strong plugin ecosystem, making it a popular choice among developers for various programming languages, including C++.
C++ is a powerful programming language extensively utilized for system/software development, game creation, and performance-critical applications. The combination of Eclipse and C++ enables developers to leverage a robust environment for coding, debugging, and managing projects efficiently.
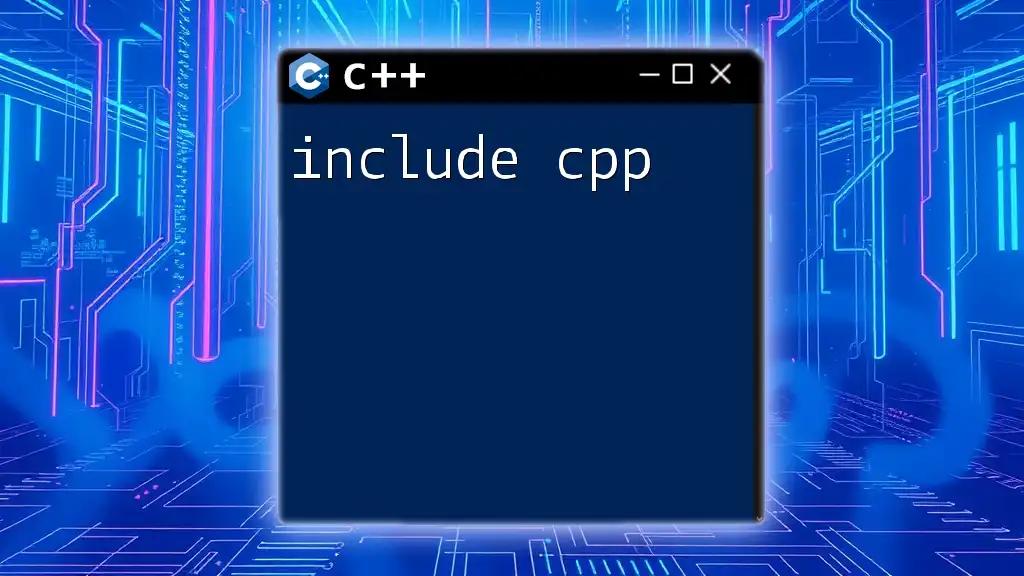
Why Choose Eclipse for C++?
When considering an IDE for C++ development, Eclipse stands out for several reasons:
-
Open Source: Eclipse is free and open-source, providing a cost-effective solution for developers.
-
Cross-Platform: Available for Windows, Linux, and macOS, Eclipse allows you to code in C++ regardless of your operating system.
-
Extensibility: The ability to customize and extend the IDE through plugins enhances its functionality to suit individual developer needs.
-
Integrated Debugging: Eclipse comes with powerful debugging tools that simplify the process of finding and fixing errors in C++ code.
-
Community Support: With a large user base, many resources, forums, and tutorials are available, making it easier to seek help and learn from others.
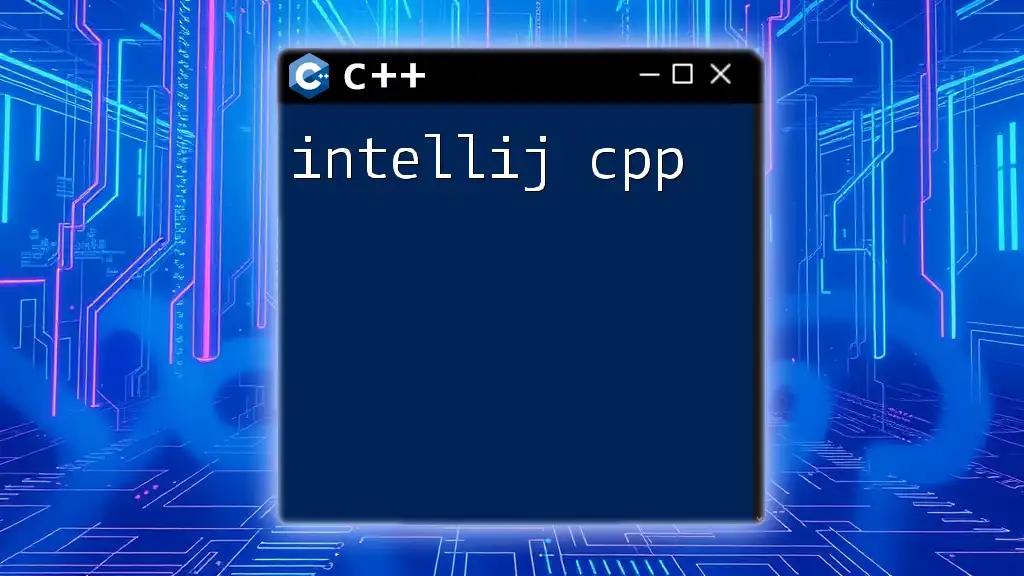
Setting Up Eclipse for C++ Development
Installation of Eclipse IDE
To begin your journey with Eclipse CPP, you first need to install the Eclipse IDE. Here is how to do it:
- Go to the [Eclipse Downloads page](https://www.eclipse.org/downloads/).
- Choose the appropriate package for C/C++ developers, often referred to as "Eclipse IDE for C/C++ Developers."
- Download the installer for your operating system.
- Run the installer and follow the prompts to complete the installation.
Before you proceed, ensure that your system meets the required specifications to run Eclipse effectively.
Installing CDT (C/C++ Development Tooling)
The next step after installing Eclipse is to set up the C/C++ Development Tooling (CDT):
- Open Eclipse and navigate to the Help menu.
- Select Eclipse Marketplace.
- In the search field, type `CDT` and look for the "C/C++ Development Tools."
- Click Go, then click Install to add the CDT plugin.
- Follow the installation prompts and restart Eclipse once completed.
After installation, ensure that the CDT features are available under the File > New > C/C++ Project menu.
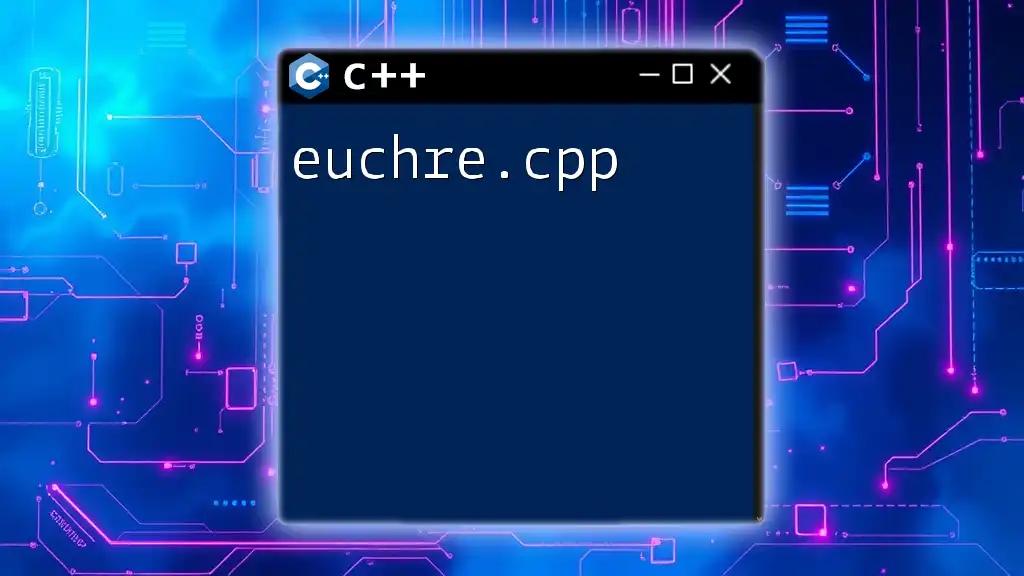
Navigating the Eclipse Environment
Overview of the IDE Interface
Upon launching Eclipse CPP, you'll be greeted by a dynamic interface designed to streamline development tasks. Familiarize yourself with the following components:
-
Project Explorer: This panel allows you to navigate through your projects and manage files easily.
-
Editor: The main area where you will write your code. It supports syntax highlighting and provides code completion features.
-
Console: This window displays output and error messages during code execution, giving immediate feedback.
Customizing your workspace effectively can improve your workflow. Explore settings under Window > Perspective to adjust layouts based on your preferences.
Creating Your First C++ Project
Starting a new project in Eclipse CPP is simple:
- From the File menu, select New and then C/C++ Project.
- Choose a Project type (e.g., "C++ Managed Build" or "C++ Makefile Project").
- Configure your Project settings, such as the project name and location.
- Click Finish to create the project.
You will find a basic structure with source files ready for coding, which is the perfect foundation to begin working with C++.
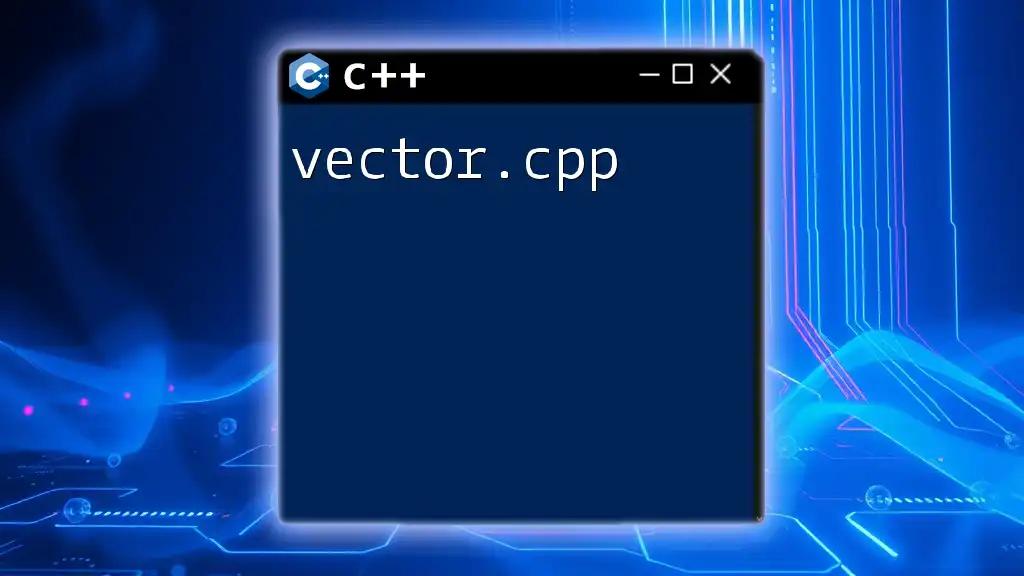
Writing and Managing C++ Code in Eclipse
Code Editor Features
The code editor in Eclipse CPP comprises several features designed to enhance productivity:
-
Syntax Highlighting: This visually distinguishes code elements (keywords, variables, etc.), making them easier to read.
-
Code Auto-Completion: As you code, Eclipse provides suggestions and can automatically complete function names, reducing errors. For example:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
This code is a simple C++ program that prints "Hello, World!" to the console.
- Code Templates: You can create templates for repetitive code blocks, saving time and reducing errors.
C++ Compiler Integration
Eclipse CPP supports a variety of C++ compilers; however, you may have to configure them first. Here's how to set up GCC, a popular compiler:
- Open your project properties by right-clicking on the project in the Project Explorer and selecting Properties.
- Navigate to C/C++ Build > Settings.
- Under the Toolchains tab, select your preferred toolchain and ensure that the compiler paths are correctly configured.
This integration allows you to build your projects through the Project > Build Project option.
Debugging Tools in Eclipse
Debugging is crucial to improving code quality and performance. Eclipse provides powerful debugging tools that ease this process. To set up debugging:
- Set Breakpoints: Click on the left margin of the code editor where you want the execution to pause.
- Run in Debug Mode: Select Debug As > C/C++ Application from the project's context menu.
- Examine Variables: As you step through the code, you can monitor variable values in real-time, which aids in identifying issues.
For example, to debug the following code:
#include <iostream>
void myFunction() {
int a = 5;
int b = 0; // Set a breakpoint here
int c = a / b; // Will cause a division by zero error
}
int main() {
myFunction();
return 0;
}
This example will allow you to monitor variable `a` and see when the program attempts to divide by zero, providing critical insights into your code’s functionality.
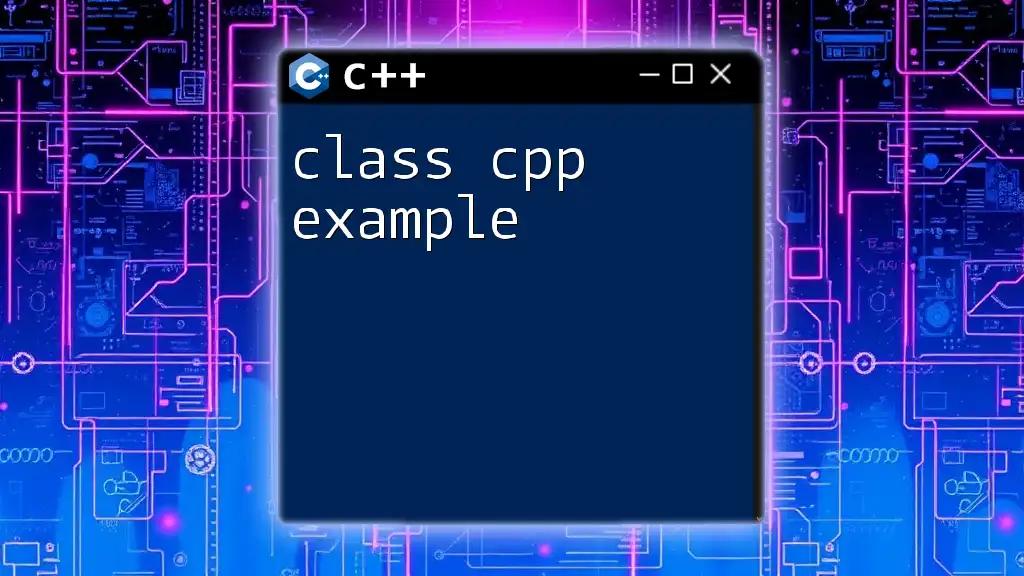
Advanced Features of Eclipse for C++ Development
Using Version Control Systems
Version control is vital for collaboration and code management. Eclipse CPP seamlessly integrates with systems like Git:
- Install the Git plugin through Eclipse Marketplace if it’s not already included.
- After installation, you can create a Git repository for your project.
- Use the Team menu to commit changes, push to remote repositories, and manage branches directly from Eclipse.
This integration streamlines the process of tracking changes and collaborating with other developers.
Refactoring and Code Analysis
Refactoring enhances code maintainability. Eclipse offers several tools:
-
Rename Variables or Functions: Right-click the item, select Refactor > Rename, and update its name across your project automatically.
-
Code Analysis Tools: Use these tools to detect potential bugs or performance issues. Eclipse can provide suggestions, warnings, and optimizations.
For instance, you can improve function readability by refactoring:
// Before Refactoring
void calc(int a, int b) {
// Do some calculations
}
// After Refactoring
void calculateSum(int firstNumber, int secondNumber) {
// Sum calculation
}
Building and Managing Projects
Eclipse allows you to manage your projects effectively:
-
Build configurations can be customized under the project's properties. You can create separate configurations for debugging and release builds.
-
Managing libraries and dependencies is key for larger projects. Use the C/C++ General > Paths and Symbols option in project properties to add and configure libraries.
For instance, if you have an external library `libMath`, you can configure it by adding it to the include paths and linking against it in the build settings.
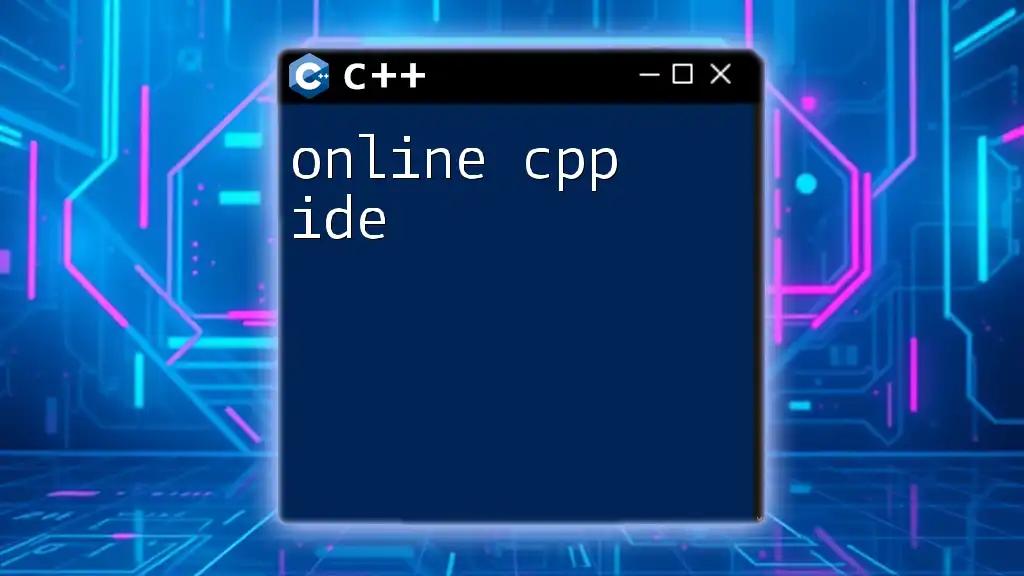
Best Practices for C++ Development in Eclipse
Tips for Writing Clean C++ Code
Quality code is easier to read, maintain, and debug. Adhere to these best practices:
-
Organize Code Effectively: Use folders in the Project Explorer to separate header files from implementation files.
-
Comment Your Code: Ensure that all functions and classes are well-documented, explaining what they do.
-
Use Meaningful Naming: Choose variable and function names that clearly describe their purpose.
Common Pitfalls to Avoid
Here are a few common mistakes to watch out for:
-
Neglecting to Test: Always test your code thoroughly. Utilize unit testing frameworks like Google Test within Eclipse.
-
Ignoring Compiler Warnings: Pay attention to the console output for any warnings. They can often highlight issues before they become problems.
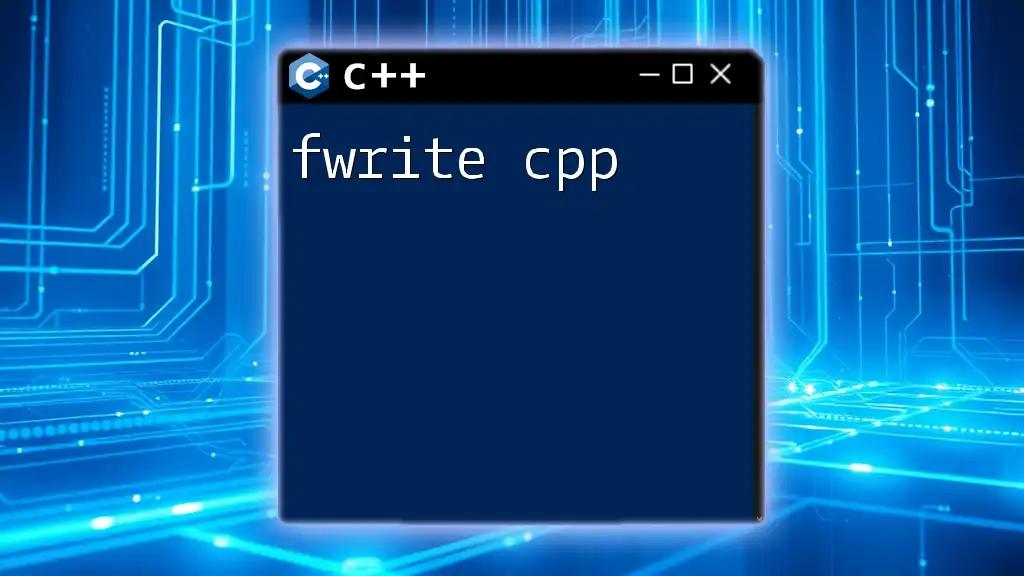
Conclusion
Eclipse CPP offers a robust and flexible environment for C++ development, catering to both beginners and experienced developers. From installation to advanced features, using Eclipse can significantly enhance your coding experience.
Next Steps for C++ Developers
To continue your journey in C++ development with Eclipse, explore further learning resources, such as online courses, local coding bootcamps, or community events. Engaging with the C++ developer community will also enhance your knowledge and skills as you work on projects in Eclipse.
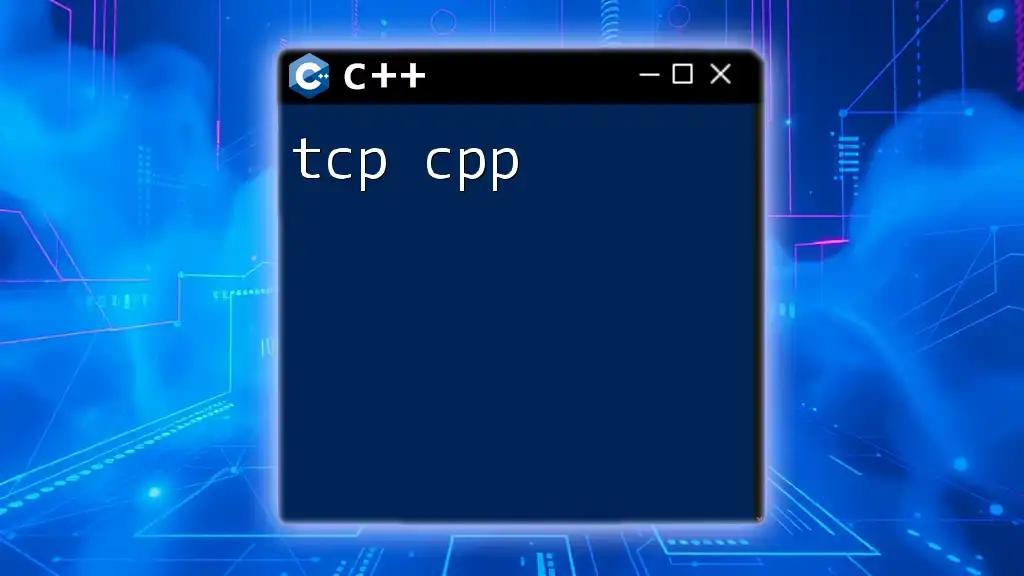
Additional Resources
Useful Plugins for Eclipse C++
Many plugins can augment your Eclipse experience. For instance, consider installing:
- Emmet for snippets and shortcuts in coding.
- Code Recommenders to enhance code completion capabilities.
Community and Support
Don’t hesitate to reach out for help. The Eclipse community is vast, with many forums and discussion boards available. Leverage platforms like Stack Overflow or the Eclipse community forums to connect with other developers and learn from their experiences.
By harnessing the power of Eclipse CPP, you position yourself for success as you develop cutting-edge software solutions in C++. Happy coding!