To get started with C++ development, you can download the Eclipse IDE, which supports C++ programming through the CDT (C/C++ Development Tooling) plugin.
Here’s a simple code snippet to demonstrate a basic C++ program structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Eclipse?
Overview of Eclipse IDE
Eclipse is an open-source Integrated Development Environment (IDE) that has been widely adopted by developers since its inception. Initially developed for Java programming, Eclipse has evolved into a versatile platform, supporting numerous programming languages through plugins, including C and C++. This flexibility and extensibility make Eclipse a go-to choice for many software developers.
Why Choose Eclipse for C++ Development?
Using Eclipse for C++ programming offers distinct advantages:
- User-friendly Interface: Eclipse provides an intuitive and well-organized user interface that makes coding straightforward even for beginners. The layout offers easy access to features and functions.
- Powerful Debugging Tools: Equipped with robust debugging capabilities, Eclipse allows developers to troubleshoot their code efficiently, viewing variable values and examining the execution flow.
- Multi-language Support: While specializing in C++, Eclipse can also be configured to handle other programming languages, making it a versatile tool for developers who work with multiple languages.
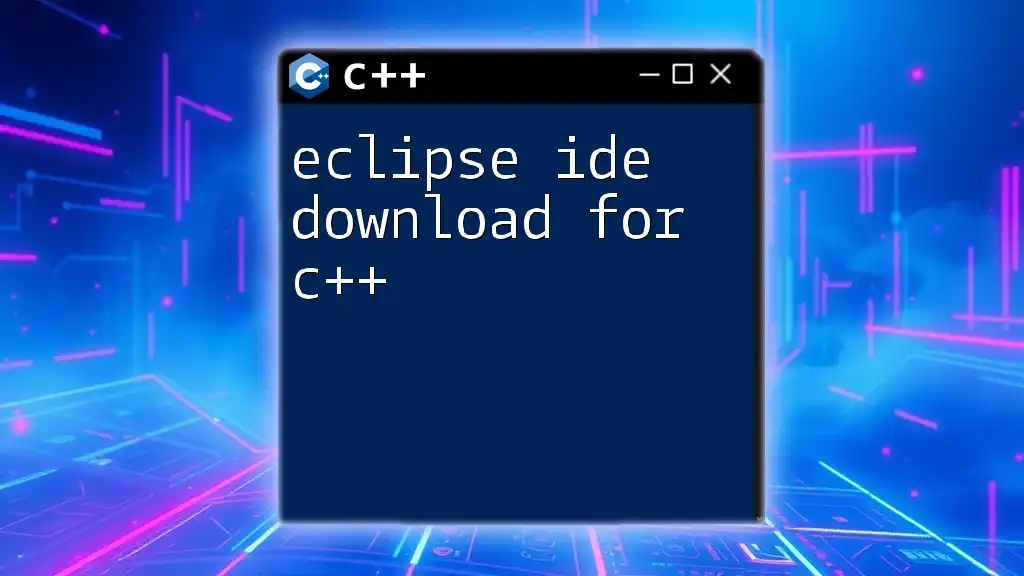
Prerequisites for Installing Eclipse
System Requirements
Before you begin the Eclipse C++ download, it’s essential to ensure that your system meets the necessary specifications. For optimal performance, consider the following:
- Windows: 64-bit Windows 7 or later
- macOS: macOS 10.12 (Sierra) or later
- Linux: 64-bit Linux with GTK 3.x
Java Development Kit (JDK) Installation
Eclipse requires Java to function. You must have the Java Development Kit (JDK) installed on your machine. The JDK enables Eclipse to run Java-based applications, including the IDE itself.
To check if JDK is installed, you can run the following command in your terminal or command prompt:
java -version
If JDK is not installed, you can download it from the official Oracle website or opt for OpenJDK. The installation process is straightforward, and following the prompts during setup will ensure it is correctly installed.
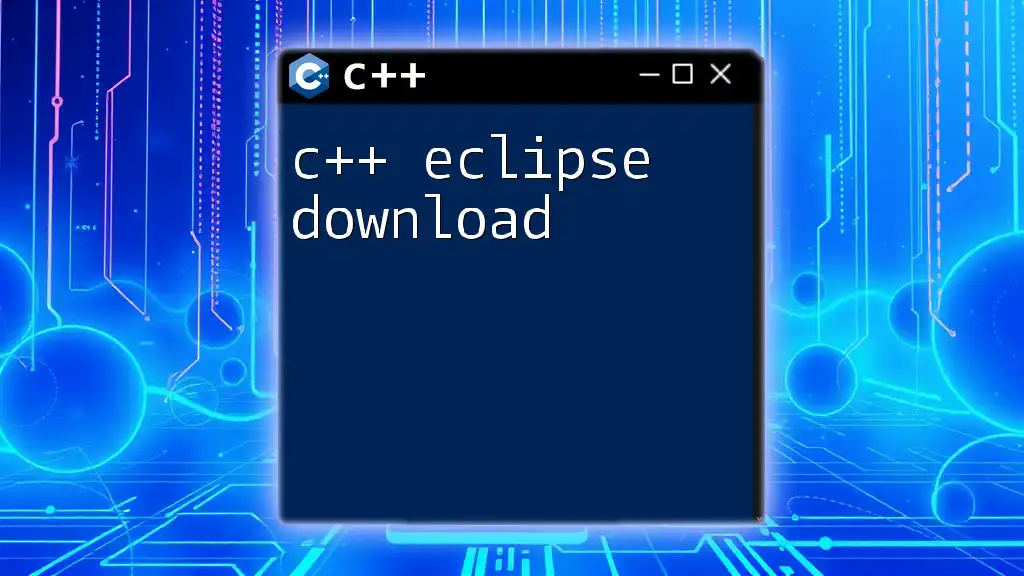
Downloading Eclipse for C++
Accessing the Eclipse Download Page
To download Eclipse, navigate to the [official Eclipse website](https://www.eclipse.org/downloads). This page offers access to the latest versions and provides necessary information on Eclipse and its components.
Selecting Eclipse IDE for C/C++ Developers
When you arrive on the download page, it’s crucial to select the right version tailored specifically for C and C++ development. Look for the option labeled "Eclipse IDE for C/C++ Developers." This version includes specialized tools, features, and plugins specifically designed for C++ programming, making it essential for your development needs.
Downloading the Installer
Once you have identified the correct IDE version, follow these steps to initiate the download:
- Click the download button associated with "Eclipse IDE for C/C++ Developers."
- Accept the terms and conditions when prompted.
- Select the version that corresponds to your operating system—Windows, macOS, or Linux.
The download will begin shortly, and you will have an installer file on your system.
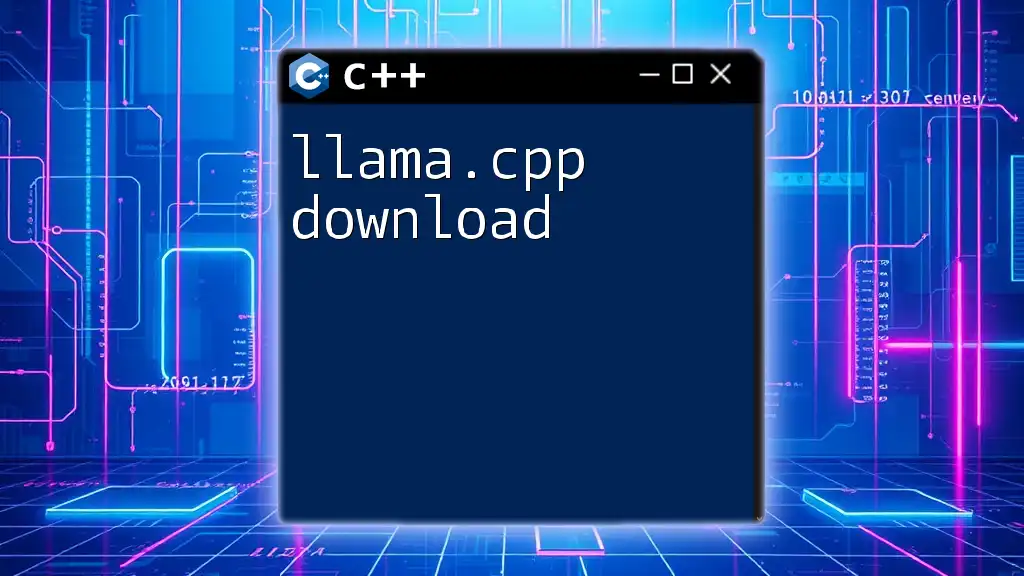
Installing Eclipse C++
Running the Installer
After downloading the installer, locate the downloaded file and execute it. The installation process varies slightly based on your operating system:
- Windows: Double-click the .exe file, and follow the installation wizard prompts.
- macOS: Open the .dmg file, drag the Eclipse application into your Applications folder.
- Linux: Extract the downloaded tarball and run the installation script. You might need to change permissions.
Common installation options are available. The default selections are typically sufficient for most users unless you prefer custom configurations.
Setting Up the Workspace
A workspace is where Eclipse gathers all your projects. During installation, you'll be prompted to define your workspace location. This directory holds your projects, configurations, and workspace settings. Take time to understand how the workspace functions, as it plays a key role in managing your development files and projects.
Initial Launch of Eclipse
Upon successful installation, launch Eclipse for the first time. You will be greeted with the welcome screen, where you can access various resources and tutorials. Familiarize yourself with the layout and features, such as the project explorer, editor pane, console, and debugging tools.
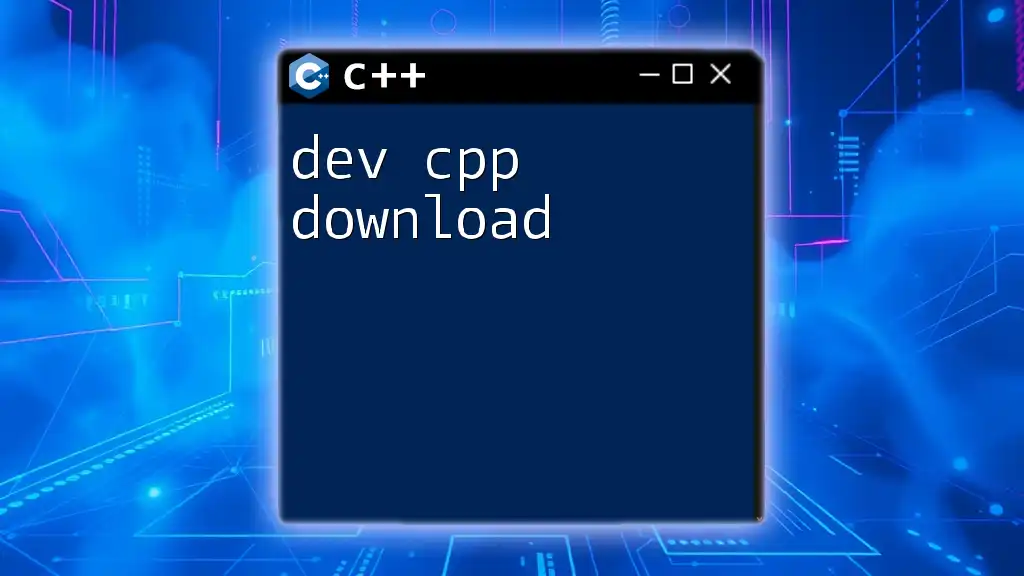
Configuring Eclipse for C++ Development
Installing C/C++ Development Tools (CDT)
To extend Eclipse's functionality for C++, it's critical to install the C/C++ Development Tools (CDT). CDT adds essential features that enhance your C++ programming experience, such as syntax highlighting, code completion, and real-time error checking.
How to install CDT:
- Open Eclipse and go to the Help menu.
- Select Eclipse Marketplace.
- In the search bar, type “C/C++ Development Tools” and press Enter.
- Locate the CDT plugin in the search results and click the Go button to install it.
- Follow the installation prompts, and restart Eclipse when prompted.
Verifying the Installation
Once CDT has been installed, it’s time to create a simple C++ project to ensure everything is functioning correctly. Follow these steps:
- Go to File > New > C++ Project.
- Choose "Executable" and then "Empty Project," select your toolchain (e.g., MinGW or GCC), and click Finish.
- In the newly created project, create a new C++ source file through File > New > Source File.
- Write a simple program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, Eclipse!" << endl;
return 0;
}
- Build and run your project using the menu options or toolbar icons.
If everything is set up correctly, you should see "Hello, Eclipse!" printed in the console window. In case you encounter any errors, take the time to troubleshoot common issues, such as ensuring the appropriate compiler is configured.
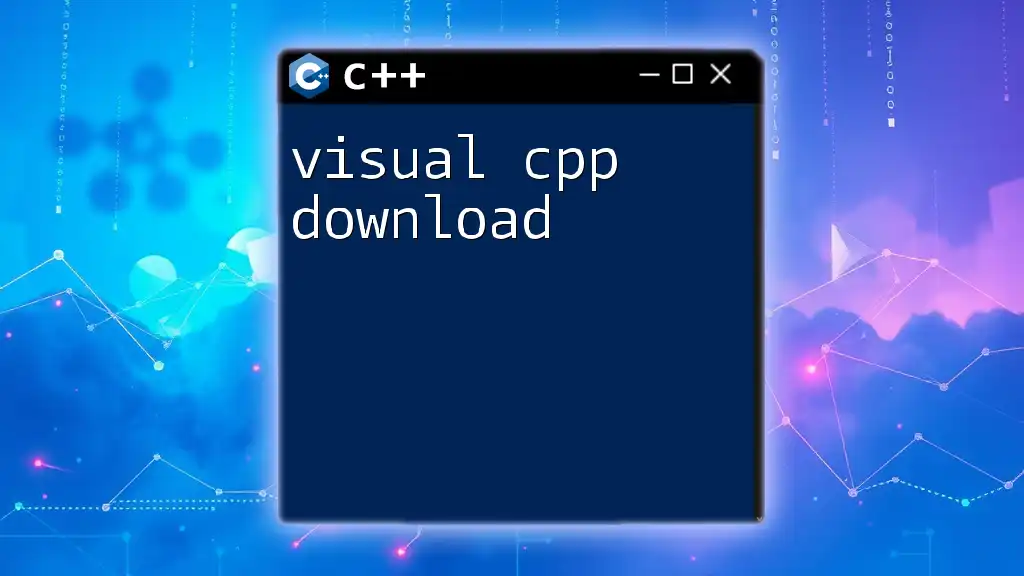
Conclusion
Downloading and installing Eclipse for C++ development is a straightforward process that equips you with a robust tool for programming. With its integrated features, debugging capabilities, and supportive community, Eclipse stands out as an excellent choice for both beginners and experienced developers. Now that you’re set up, explore the various features of Eclipse, and enhance your journey in C++ programming.
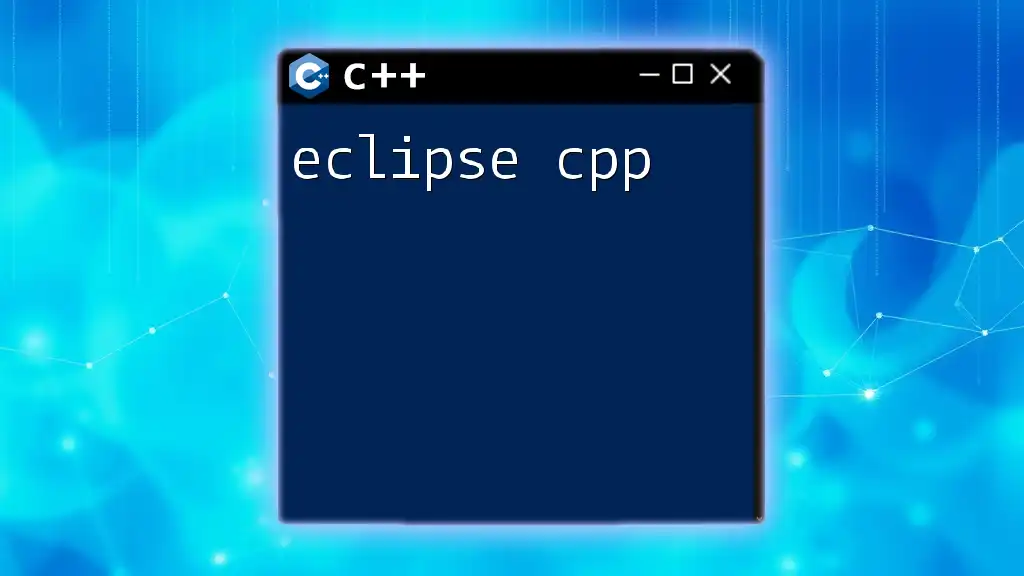
Additional Resources
For further exploration, consider checking the official Eclipse documentation for comprehensive guides and tutorials. Engaging with C++ learning platforms and communities will enhance your programming skills and provide support as you dive deeper into your projects. Don’t hesitate to seek out tutorials on advanced Eclipse features such as debugging and efficiency tips to make the most out of your development experience.