Eclipse is a powerful IDE for C++ development on macOS, providing tools for efficient coding, debugging, and project management.
Here’s a simple "Hello, World!" C++ program to get you started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Installing Eclipse on Mac
System Requirements
Before diving into the installation process for Eclipse C++ on Mac, it’s important to ensure that your system meets the minimum requirements. Eclipse IDE is generally lightweight, but certain versions and configurations may require specific hardware and software capabilities. Make sure your Mac runs a compatible version of macOS and has enough RAM and disk space for development tasks.
Downloading Eclipse
To begin, you'll need to download the Eclipse IDE tailored for C++. Access the official Eclipse downloads page, where you will find various packages. For C++ development, choose the Eclipse IDE for C/C++ Developers. This package includes tools specifically designed for C++ programming, such as the CDT (C/C++ Development Tooling).
Installation Steps
Once you've downloaded the appropriate version, locate the installation file in your Downloads folder. Drag the Eclipse icon to your Applications folder to complete the installation. After moving it, navigate to your Applications folder and double-click on the Eclipse icon to launch the IDE. You’ll be prompted to select a workspace—the directory where your projects will be stored. Choose a location that’s easy for you to access.
Setting Up C++ Development Environment
Installing the C++ Compiler
For effective C++ development, you need a compiler. The two primary compilers used on macOS are GCC (GNU Compiler Collection) and Clang. Xcode Command Line Tools, which include the Clang compiler, provides a convenient way to access these tools.
To install Xcode Command Line Tools, open your terminal and execute the following command:
xcode-select --install
Follow the prompts to complete the installation of the command line tools.
Configuring Eclipse for C++
Upon launching Eclipse for the first time, you may need to configure it to ensure it recognizes your C++ compiler. Navigate to Eclipse’s preferences (Eclipse > Preferences) and look for the C/C++ section. Ensure the toolchain is correctly set up for your compiler, like Clang, which should be automatically detected. This setup will allow you to compile and build C++ projects seamlessly.
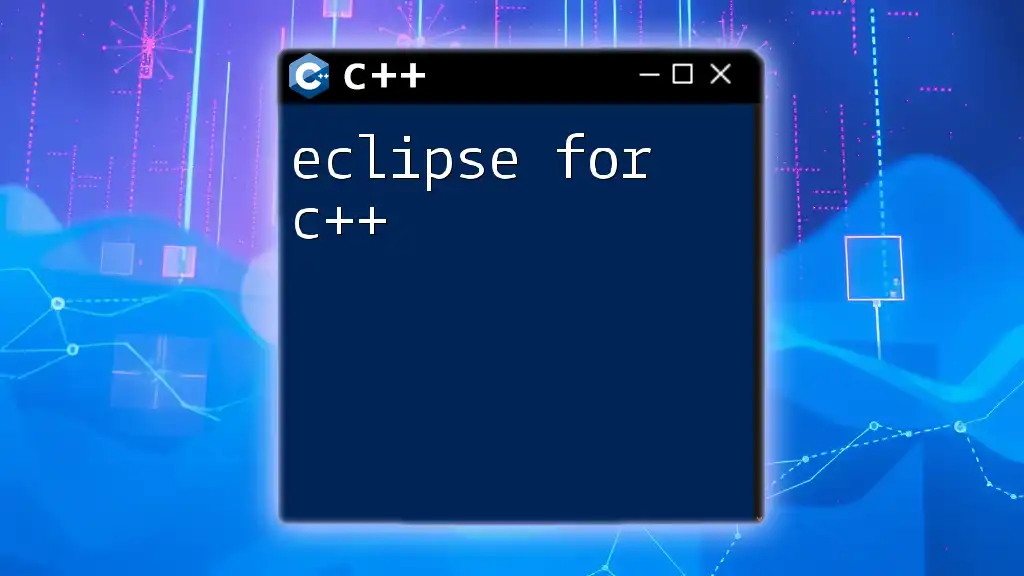
Creating Your First C++ Project in Eclipse
Step-by-Step Guide
Creating a project in Eclipse is straightforward. Within the IDE, click on File > New > C++ Project. You'll be presented with different templates; select the C++ Project option and give your project a name. Eclipse will assist you in setting up the project structure, which typically includes directories for source files, header files, and binaries.
Project Structure Overview
Understanding the project hierarchy is crucial for successful development. In your newly created project, you’ll typically find:
- src: Contains your source files (.cpp).
- include: Used for header files (.h).
- bin: Where the compiled binaries will be output.
This structure helps keep your files organized and improves project manageability as it scales.
Writing Your First C++ Program
Let’s get started with some actual code. In the src folder, create a new source file named `main.cpp`. Open this file and enter the following C++ code to display a simple greeting:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Once you've typed out the code, save the file.
Compiling and Running the Program
Now it’s time to compile and run your program. Click on the Build button (usually represented by a hammer icon) in the toolbar. If there are no compilation errors, you should now be able to run your program by clicking on the Run option (play button icon). The output will display in the console at the bottom of the Eclipse interface, confirming your success in writing and executing your first C++ program.
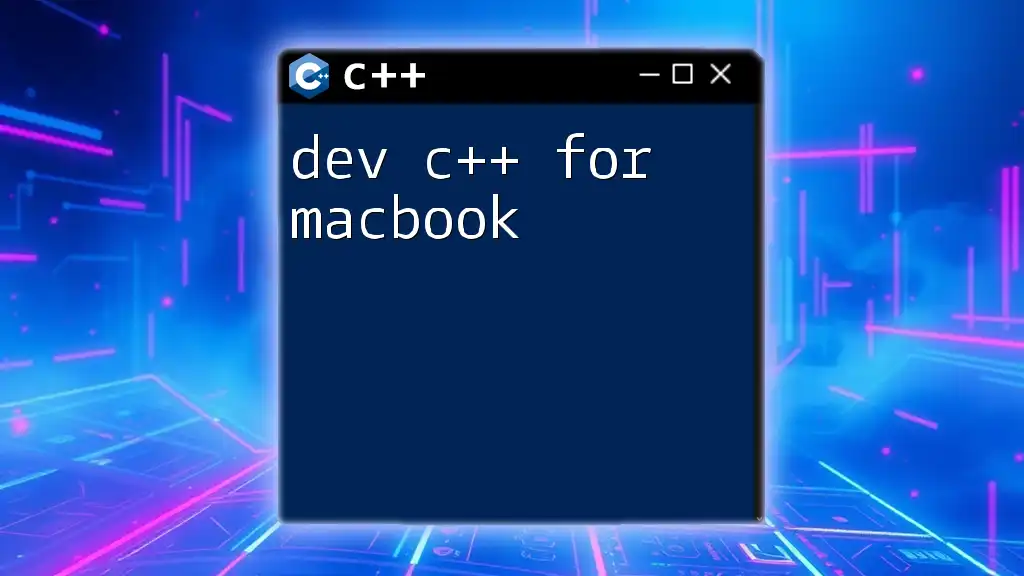
Debugging in Eclipse
Introduction to the Debugger
Debugging is an essential part of software development. Eclipse comes equipped with robust debugging tools that help identify and fix issues in your code. You can step through your code line by line, inspect variables, and view the call stack at any time during execution.
Setting Breakpoints and Inspecting Variables
To set a breakpoint in your code, simply double-click on the left margin next to the line number where you want execution to pause. After setting a breakpoint, run your program in debug mode by navigating to Run > Debug As > C/C++ Application. When the execution pauses at the breakpoint, hover over variables to see their current values, giving you insight into what's happening in your application.
Using the Debug Perspective
Switching to the Debug perspective in Eclipse provides a specialized layout for debugging tasks. You will see views for currently running threads, call stacks, and expressions, making it easier to manage your processes. Familiarizing yourself with this perspective can significantly enhance your debugging efficiency.
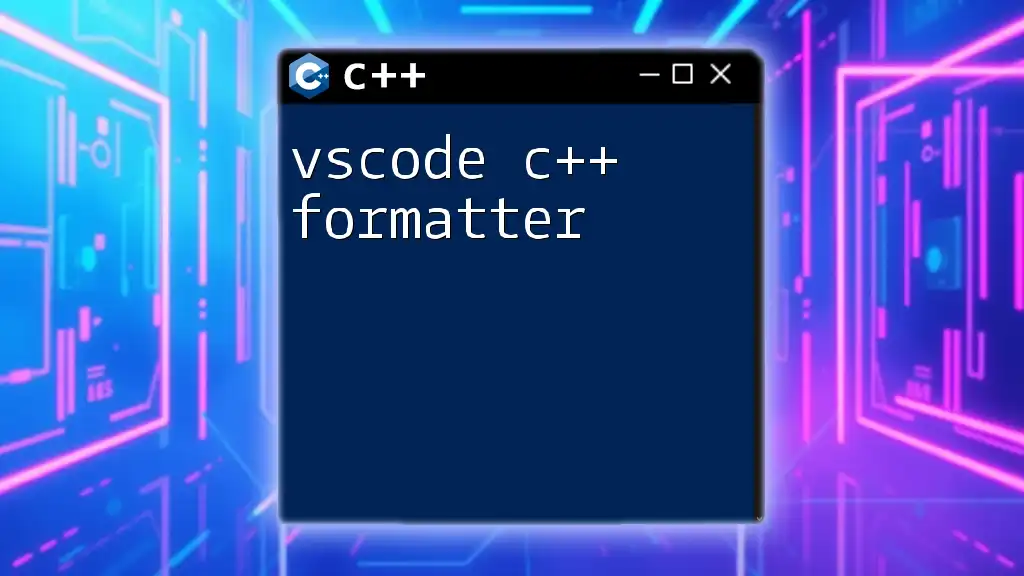
Managing Libraries in Eclipse
Using External Libraries
While writing basic C++ applications is straightforward, integrating external libraries for added functionality is common in software development. Eclipse allows you to include libraries easily. To link an external library, navigate to Project > Properties > C/C++ Build > Settings. Here, you can specify library paths and include directories necessary for your project.
Common C++ Libraries for Mac Developers
Several libraries can enhance your projects:
- Boost: A collection of portable, peer-reviewed C++ libraries.
- OpenCV: A library focused on real-time computer vision. Integrating these libraries often involves installing them via package managers like Homebrew or manually downloading and linking them as described earlier.
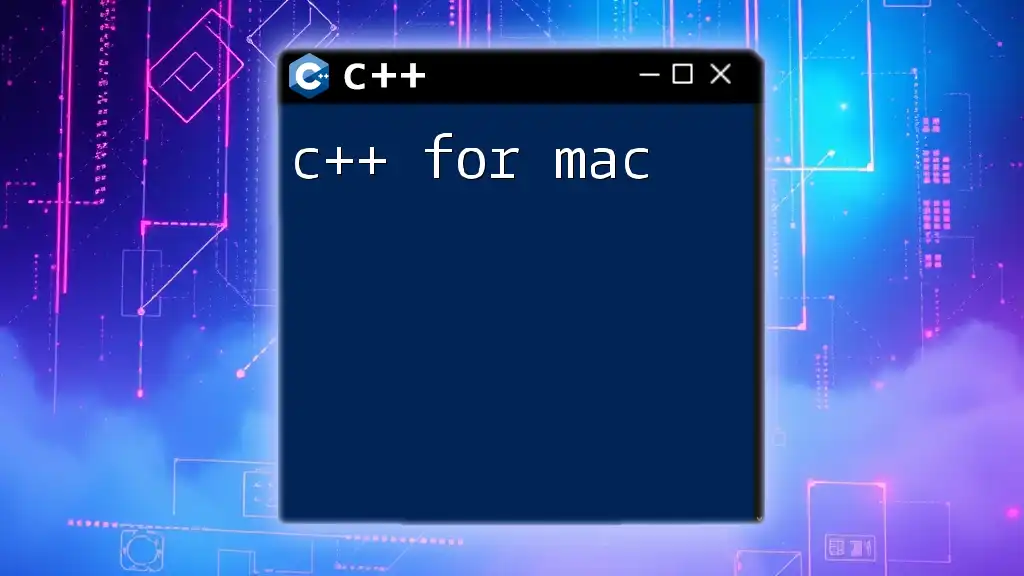
Best Practices for C++ Development in Eclipse
Writing Clean Code
Writing clean code is fundamental to becoming a successful developer. This entails using meaningful variable names, maintaining proper indentation, and adhering to a consistent style guide. Keeping your code organized will make it easier for others (and yourself) to understand and maintain over time.
Using Version Control
Version control systems, such as Git, are indispensable in collaborative environments. They allow developers to track changes, revert to previous states, and collaborate seamlessly. Eclipse has built-in support for Git; make sure to connect your project with a repository to leverage these benefits effectively.
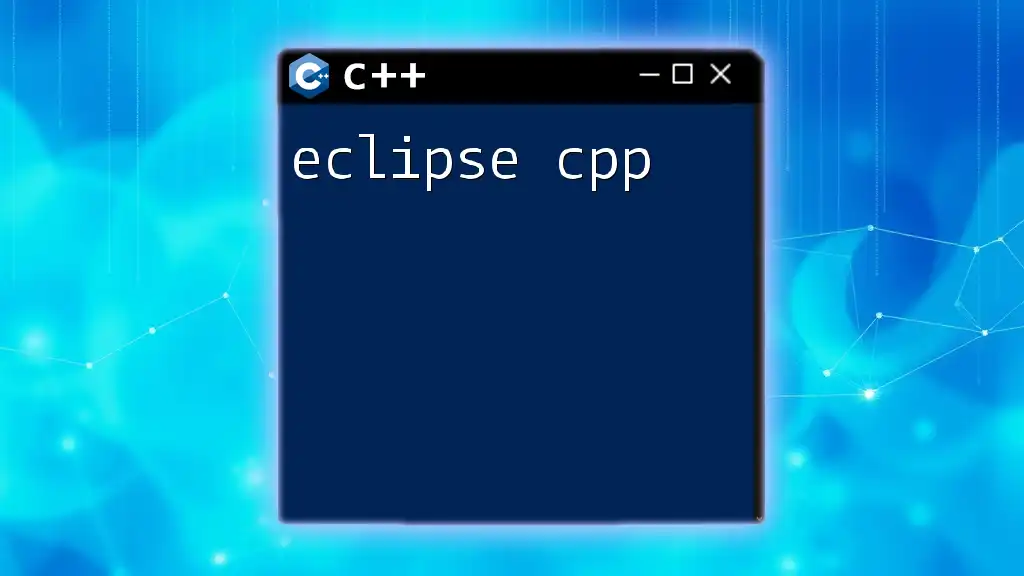
Troubleshooting Common Issues
Eclipse Not Starting or Crashing
If you find that Eclipse is crashing or not initiating, it can often be attributed to compatibility issues or misconfigurations. Check that your version of Eclipse matches your MacOS version. Additionally, reviewing the error logs, accessible through the Eclipse workspace, can provide hints for troubleshooting.
Compiler Errors and Warnings
As you write code, you may encounter various errors and warnings. Common issues include syntax errors, undeclared identifiers, and type mismatches. Versions of Eclipse generally highlight these errors in real-time, allowing for immediate corrections. Pay special attention to the Problems tab in your workspace, which lists all compilation issues in detail.
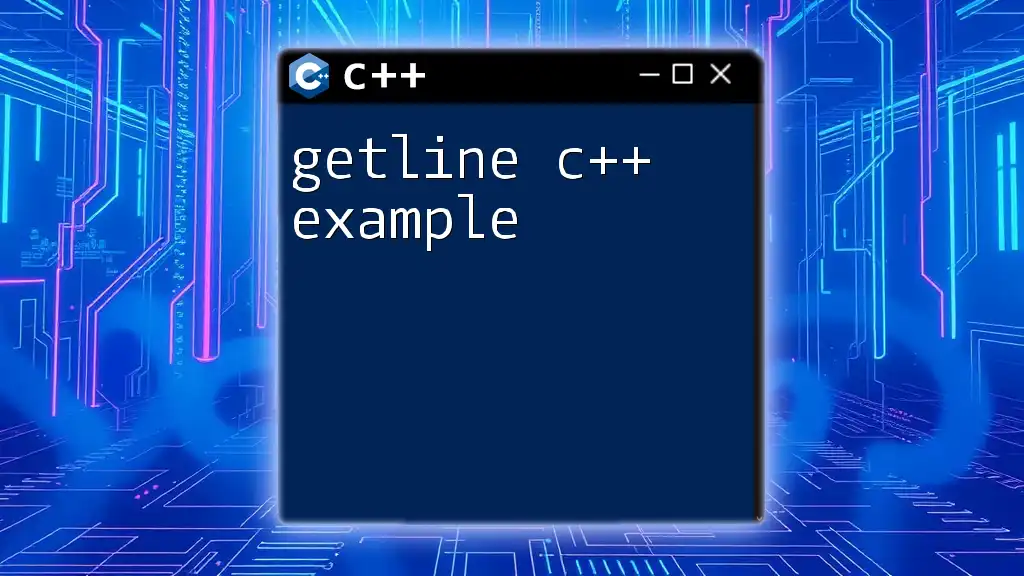
Conclusion
In this guide, we have covered everything you need to get started with Eclipse C++ for Mac. From installation to creating your first project, debugging, and implementing best practices, you now have the foundational knowledge to start your journey in C++ development using Eclipse. With practice and exploration, you will become more adept at utilizing this powerful IDE.
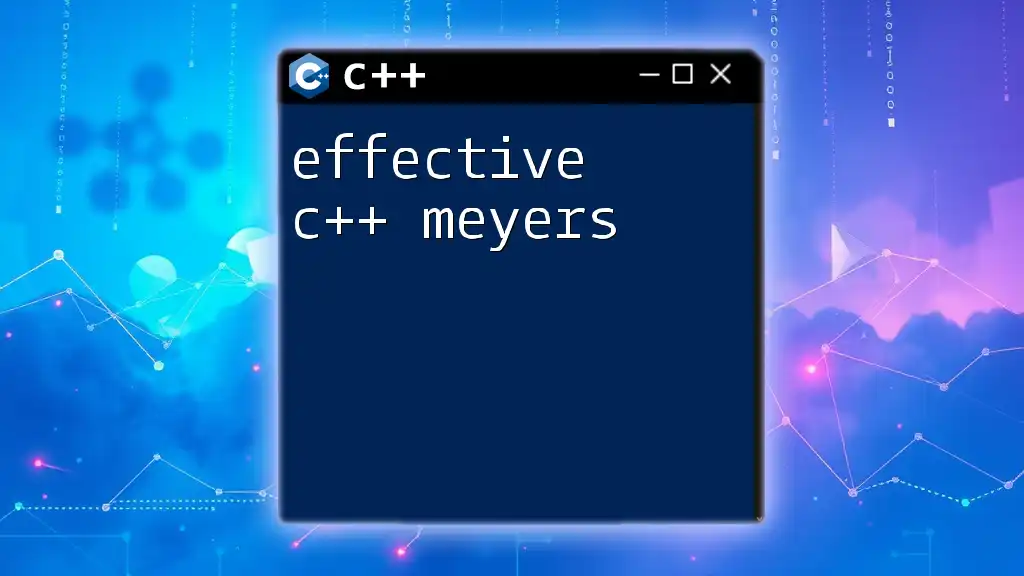
Additional Resources
To further enhance your learning, refer to the official Eclipse documentation and explore community forums. Books and online tutorials specific to C++ development can also be beneficial as you advance your skills.
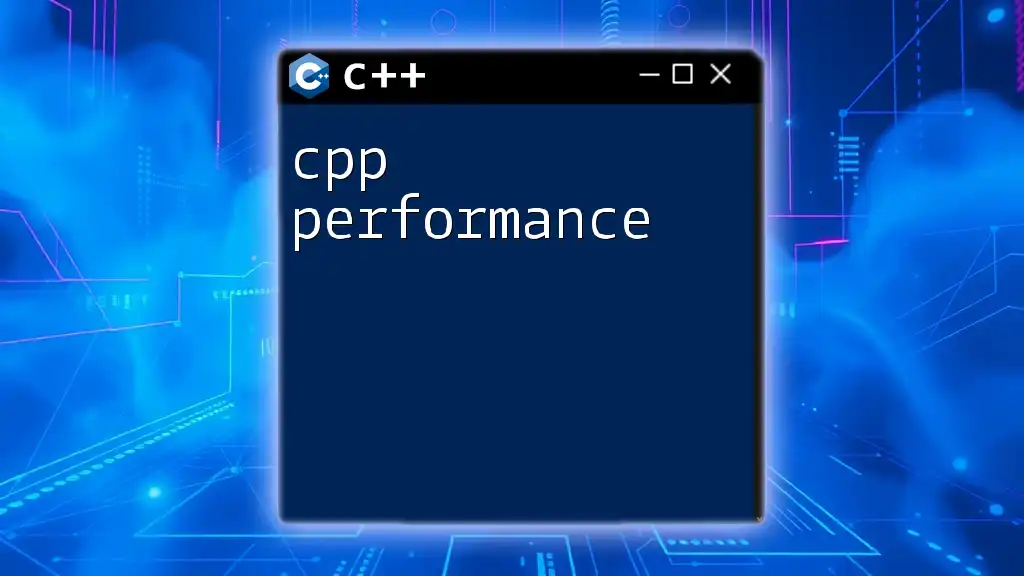
Call to Action
Download Eclipse today and begin your adventure in C++ programming! With the tools and knowledge you've gained, you're well on your way to becoming a proficient C++ developer.