You can call C++ functions from C by using `extern "C"` to prevent name mangling, allowing the C program to link to the C++ function seamlessly.
Here's a simple code snippet to illustrate this:
// cpp_function.cpp
extern "C" {
void cpp_function() {
// Example C++ code
std::cout << "Hello from C++!" << std::endl;
}
}
// main.c
#include <stdio.h>
extern void cpp_function(); // Declare the C++ function
int main() {
cpp_function(); // Call the C++ function
return 0;
}
Make sure to compile the C++ code with a C++ compiler and link it with the C code during the compilation process.
Understanding C++ and C Interoperability
Differences Between C and C++
C is a procedural programming language that emphasizes function and structured programming, while C++ extends C with object-oriented features. It's essential to understand that while these languages share syntax and concepts, there are fundamental differences in data types, error handling, and memory management.
In C, structs are used to create complex data types, while C++ introduces classes, which provide encapsulation and inheritance. Function overloading is a key feature in C++ that allows multiple functions with the same name but different parameters, making it more versatile than C.
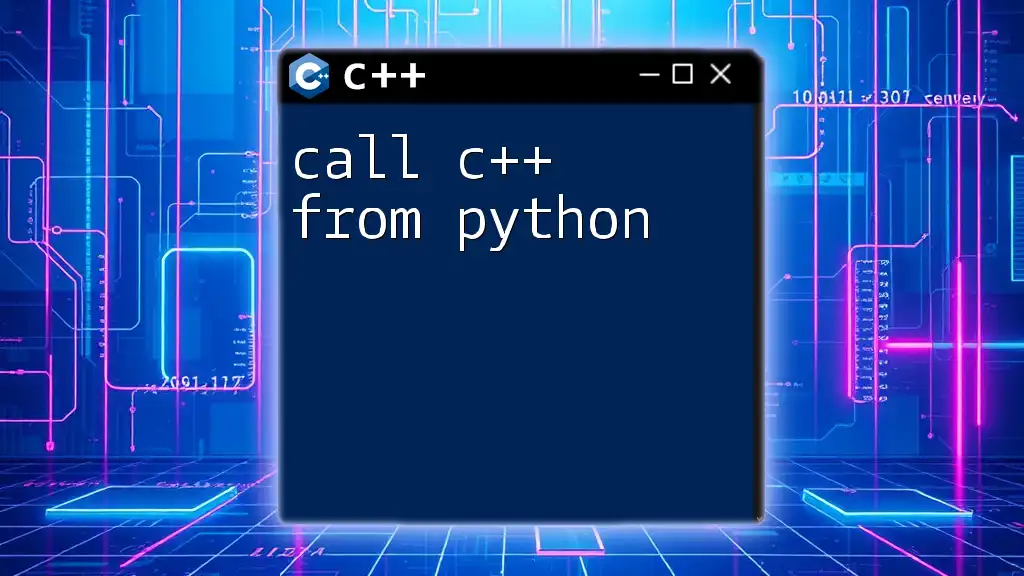
Setting Up Your Environment
Choosing the Right Tools
Before you begin, selecting the proper development environment is crucial. Recommended IDEs include Visual Studio, Code::Blocks, and CLion, which all provide excellent support for both C and C++. When it comes to compilers, GCC and Clang are widely used for their robust features and compatibility.
Basic Setup Steps
To set up your environment:
- Install the Compiler: Download and install GCC or Clang from their official websites.
- Set the Directory Structure: Create a directory for your project that organizes your C and C++ files. A common structure might look like this:
my_project/ ├── src/ │ ├── main.c │ └── my_functions.cpp └── build/
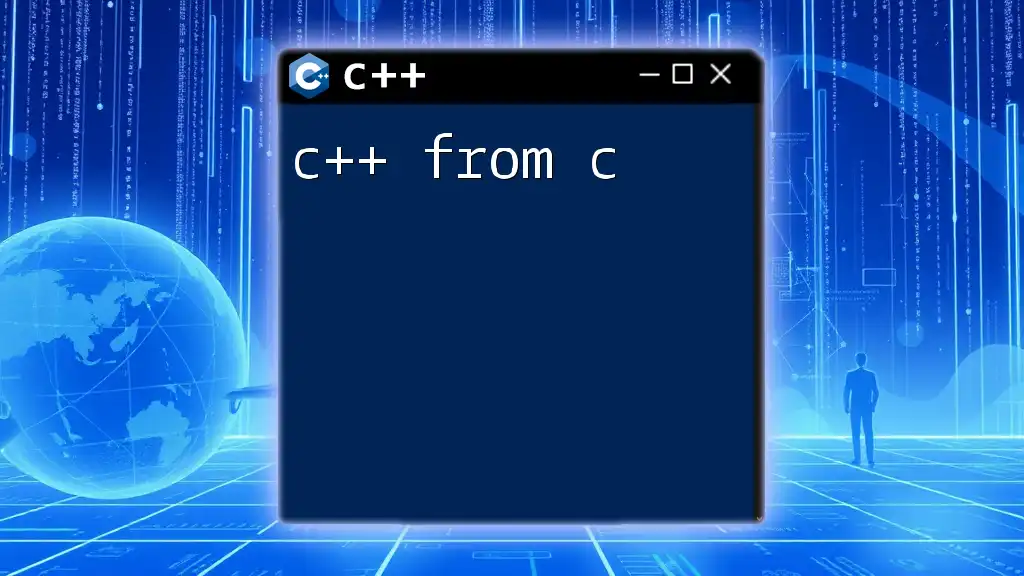
Writing a Simple C++ Function to be Called from C
Creating a C++ Function
To call a C++ function from C, you must tell the C++ compiler to avoid name mangling, which would otherwise obscure the function name when linking. You can achieve this by using `extern "C"`:
extern "C" {
int add(int a, int b) {
return a + b;
}
}
In this snippet, `extern "C"` indicates that the function should use C linkage, which allows it to be called from C code effectively.
Compiling Your C++ Code
Once you've written your C++ code, the next step is to compile it. You can compile the C++ code into an object file using the following command:
g++ -c src/my_functions.cpp -o build/my_functions.o
This command compiles `my_functions.cpp` without linking, creating an object file that can be linked later with your C code.
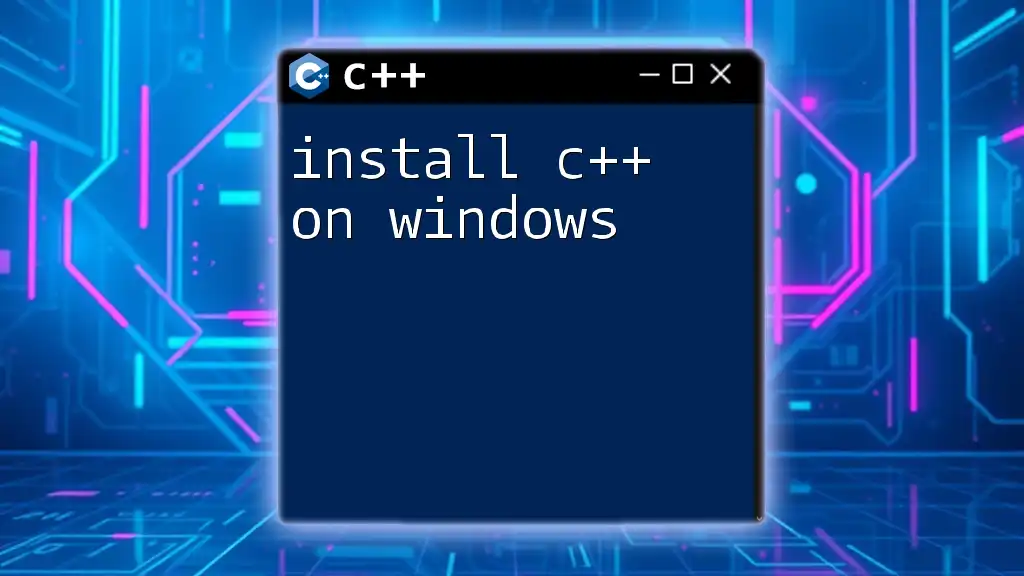
Calling the C++ Function from C
Writing the C Code
Now that you have your C++ function ready, you can write the C code to call it. Here’s a simple example:
#include <stdio.h>
extern int add(int a, int b); // Function declaration
int main() {
int result = add(5, 10); // Calling C++ function
printf("Sum is: %d\n", result);
return 0;
}
In this example, we declare the C++ function with an `extern` directive, which informs the C compiler that this function is defined elsewhere.
Compiling and Linking
To run your program, you need to compile the C code and link it with the previously compiled C++ object file:
gcc src/main.c build/my_functions.o -o build/my_program
This command compiles `main.c` and links it with `my_functions.o`, creating an executable `my_program`.
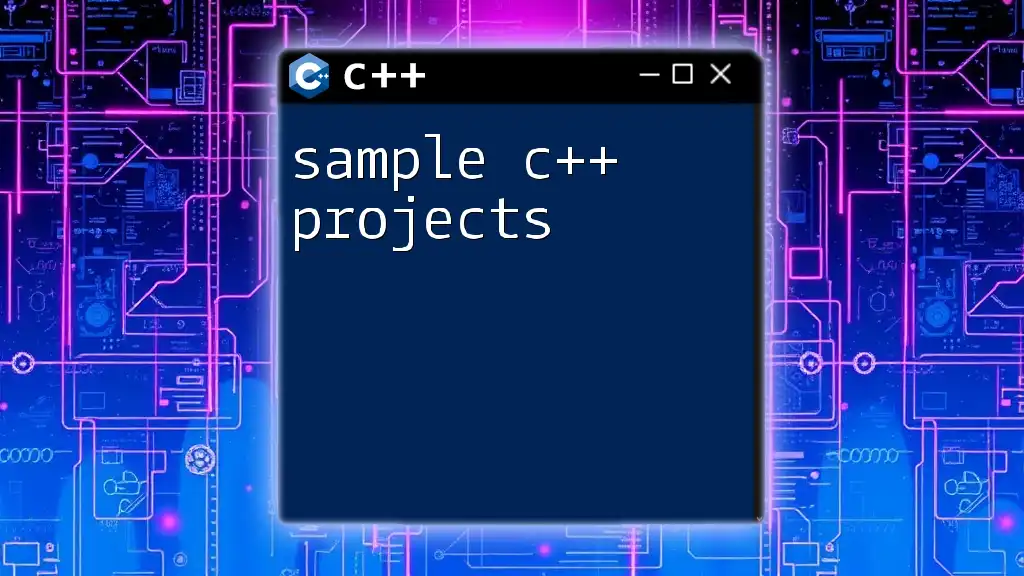
Handling Complex Data Types
Passing Structs Between C and C++
When working with complex data types, you can pass structs from C to C++. Here’s an example of a C struct definition:
typedef struct {
int x;
int y;
} Point;
Now, let's implement a C++ function that takes this struct as an argument:
extern "C" {
void print_point(Point p) {
std::cout << "X: " << p.x << ", Y: " << p.y << std::endl;
}
}
This function accepts a `Point` struct and prints its coordinates.
Memory Management Considerations
When working with mixed C and C++ code, managing memory correctly is crucial. C++ employs constructors and destructors to handle dynamic memory, while C requires manual allocation and deallocation using `malloc` and `free`. It’s essential to be consistent in memory management to avoid leaks and corruption.
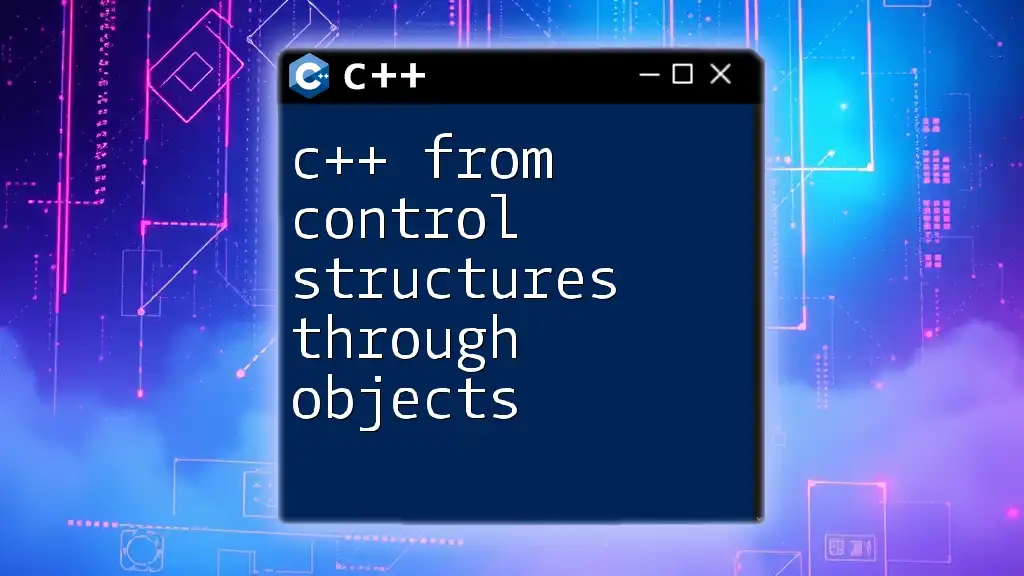
Common Pitfalls and How to Avoid Them
Name Mangling Issues
One of the most significant issues when calling C++ from C arises from name mangling. This process encodes additional information into function names to support features like function overloading. Always use `extern "C"` when declaring the functions to prevent this.
Data Type Compatibility
C and C++ have differing approaches to data types. For instance, C does not recognize the `bool` type directly, whereas C++ does. Ensure that data types exchanged between C and C++ are compatible; otherwise, you may encounter runtime errors or unexpected behavior.
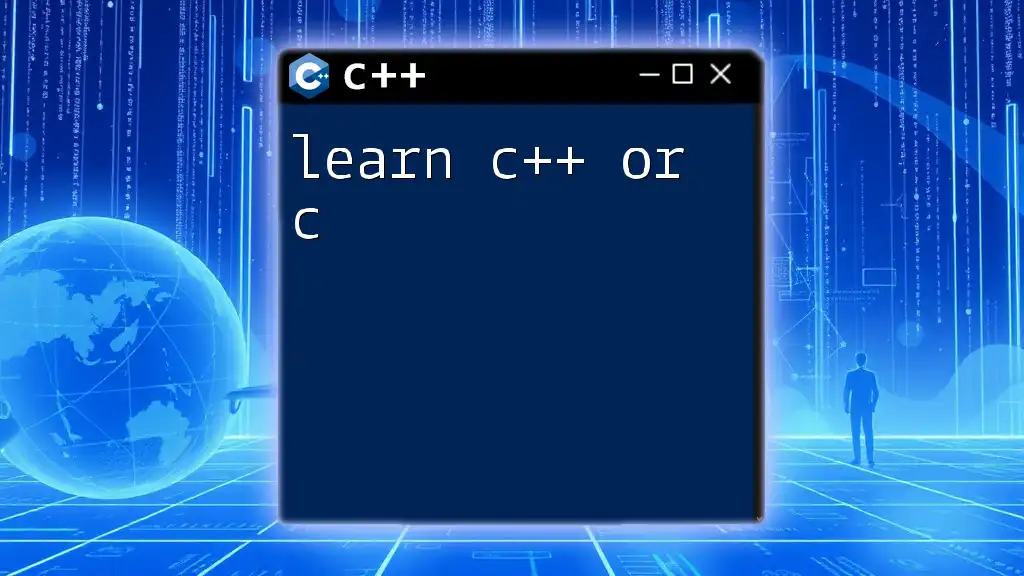
Debugging Tips
Using Debuggers Effectively
Utilizing debuggers like GDB for mixed C and C++ projects can streamline identifying issues. Set breakpoints in both C and C++ files, and step through your code to examine program flow and variable states.
Common Compiler Warnings and Errors
When trying to call C++ from C, you might run into various errors or warnings, such as:
- Undefined references
- Type conversion issues
Pay close attention to compiler output; understanding these messages can greatly assist in troubleshooting problems in your code.
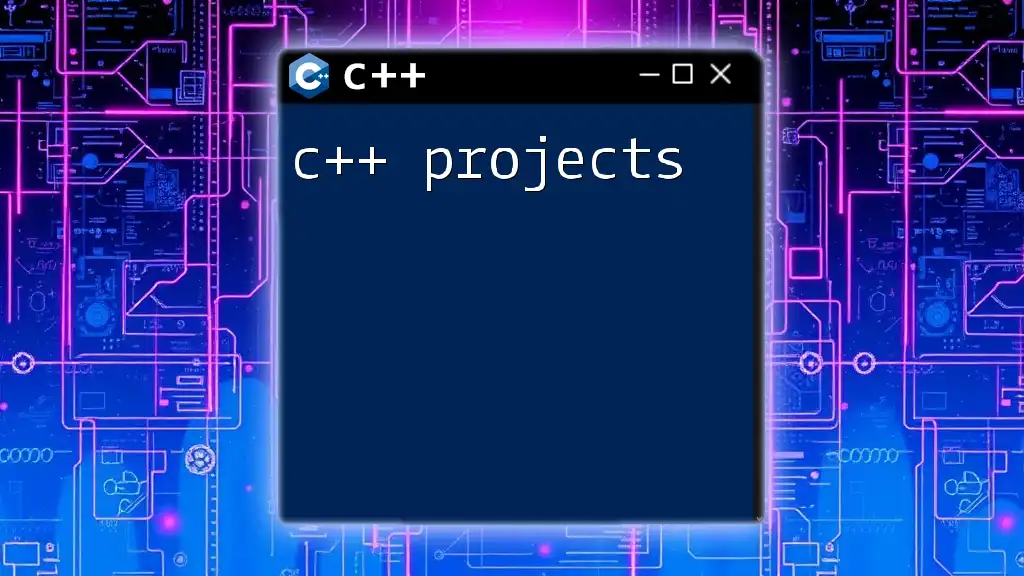
Conclusion
In this guide, we've explored how to effectively call C++ from C, from setting up your environment to handling complex data types. By adhering to the guidelines outlined, you can harness the strengths of both languages to create powerful applications.
Now, it’s time to put your knowledge to the test. Start experimenting by implementing your own mixed-language projects.
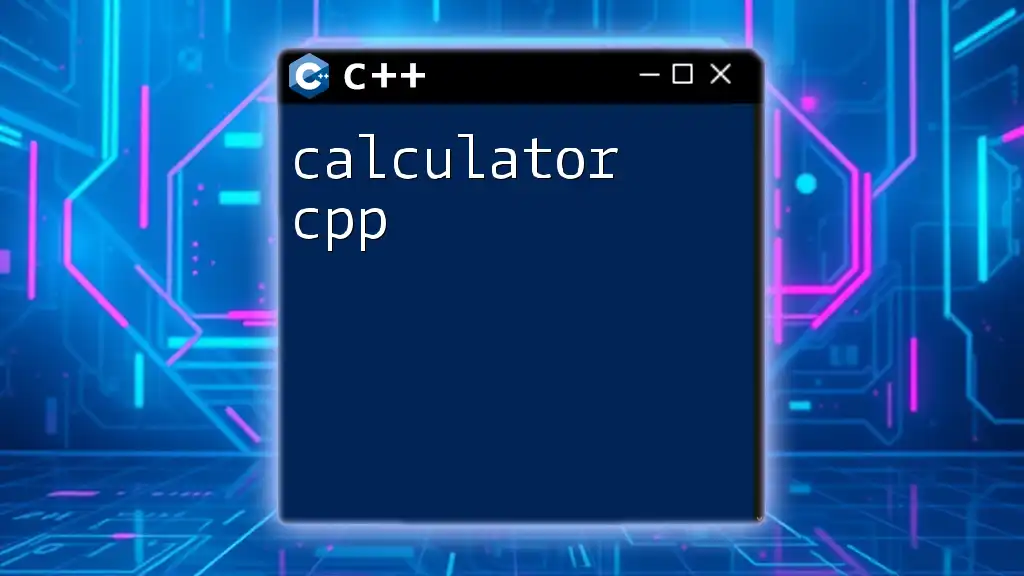
Call to Action
We encourage you to share your experiences and challenges when integrating C and C++. Your insights can help others in the community navigate similar journeys. If you’re interested in furthering your skills, consider our educational offerings that specialize in teaching C and C++ commands in a quick, concise manner!