You can call C++ code from Python using the `ctypes` library, which allows you to interface with C-style functions compiled into shared libraries.
Here’s a simple example demonstrating how to define a C++ function, compile it into a shared library, and call it from Python:
C++ Code (example.cpp)
#include <iostream>
extern "C" {
int add(int a, int b) {
return a + b;
}
}
Compile the C++ Code
Assuming you are using g++, compile the code into a shared library:
g++ -shared -o example.so -fPIC example.cpp
Python Code
import ctypes
# Load the shared library
example = ctypes.CDLL('./example.so')
# Call the add function
result = example.add(5, 3)
print(f'The result of adding is: {result}')
This snippet allows you to integrate C++ functionality into your Python application efficiently.
Understanding C++ and Python Interoperability
Benefits of Calling C++ from Python
Combining C++ with Python provides a range of significant advantages.
High Performance: C++ is known for its performance, especially in execution speed and resource management. By calling C++ functions from Python, you can leverage C++’s efficiency for performance-critical tasks while maintaining Python's ease of use for high-level scripting.
Enhanced Functionality: C++ has a rich set of libraries and frameworks that cover various domains, including graphics, computational mathematics, and data processing. By integrating C++, Python can access these robust libraries, thus enhancing the functionality of your Python applications.
Leveraging Existing C++ Libraries: If you have legacy C++ code or libraries, you can integrate them into your Python programs, saving time and effort compared to rewriting them from scratch in Python.
Use Cases
The combination of C++ and Python shines in various fields:
-
Scientific Computing: Libraries like NumPy and SciPy can be augmented with high-performance C++ functions, making intensive computations faster and more efficient.
-
Game Development: The performance needs of real-time game engines often require C++. Python, with its easy scripting capabilities, can handle game logic while C++ manages the heavy lifting.
-
Machine Learning and Data Processing: C++ can accelerate data processing tasks, making it a perfect partner for Python, which is widely used in data science.
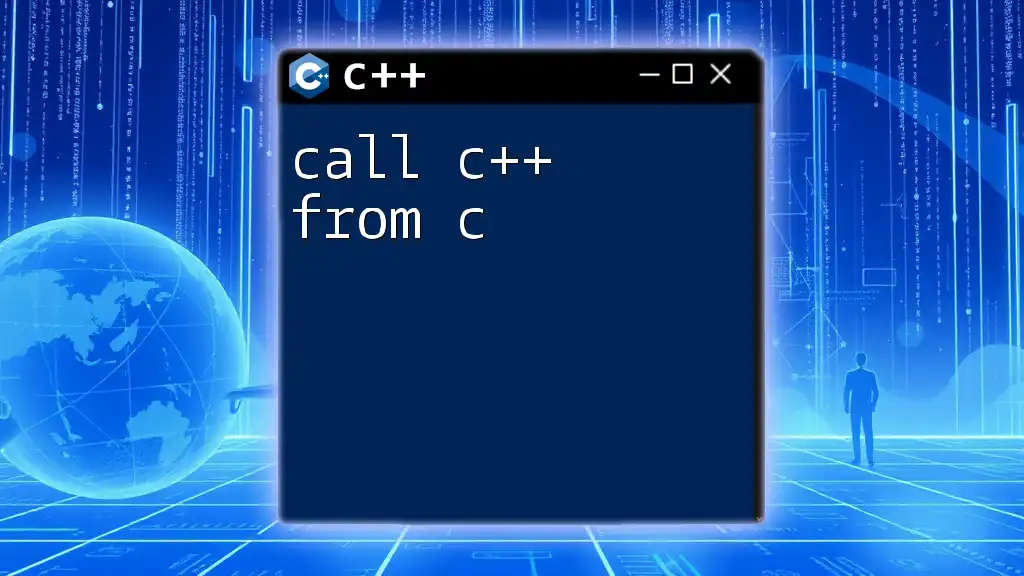
Methods for Calling C++ from Python
Using ctypes
Overview of ctypes
ctypes is a standard Python library that allows you to call functions from DLLs/shared libraries, enabling seamless integration of C/C++ code with Python. It is a lightweight option, requiring no additional dependencies.
Step-by-Step Example
-
Creating a C++ Shared Library
First, we need to write a simple C++ function and compile it as a shared library.
// example.cpp extern "C" { double add(double a, double b) { return a + b; } }
Compilation: Compile this code into a shared library (e.g., `example.so` on Linux or `example.dll` on Windows):
g++ -shared -o example.so -fPIC example.cpp
-
Using ctypes in Python
Now, in Python, we can use `ctypes` to load the shared library and call the `add` function.
import ctypes # Load the shared library example = ctypes.CDLL('./example.so') # Set argument types and return type example.add.argtypes = (ctypes.c_double, ctypes.c_double) example.add.restype = ctypes.c_double # Call the function result = example.add(3.0, 4.0) print("Result:", result) # Output: Result: 7.0
Using Cython
What is Cython?
Cython is a programming language that is a superset of Python, designed to give C-like performance with code that is mostly written in Python. Cython is particularly beneficial as it simplifies the process of creating Python modules from C/C++ code.
Step-by-Step Example
-
Creating a Cython Wrapper
Here's a simple example to wrap a C++ function using Cython:
# example.pyx cdef extern from "example.cpp": double add(double a, double b) cpdef double py_add(double a, double b): return add(a, b)
-
Compiling Cython Code
To compile your Cython code, create a `setup.py` file:
from setuptools import setup from Cython.Build import cythonize setup( ext_modules=cythonize("example.pyx"), extra_compile_args=["-std=c++11"], language="c++" )
Then, run this command to build the module:
python setup.py build_ext --inplace
-
Using the compiled module in Python
Import and use your Cython function just like any other Python function:
from example import py_add result = py_add(3.0, 4.0) print("Result:", result) # Output: Result: 7.0
Using Boost.Python
Overview of Boost.Python
Boost.Python is a part of the larger Boost C++ Libraries, designed explicitly for seamless interoperability between C++ and Python. It simplifies the process of exposing C++ functions and classes to Python.
Step-by-Step Example
-
Building a C++ Code with Boost.Python
Here's an example of how to expose a simple C++ function using Boost.Python:
// example.cpp #include <boost/python.hpp> double add(double a, double b) { return a + b; } BOOST_PYTHON_MODULE(example) { using namespace boost::python; def("add", add); }
Compilation: You will need to link against Boost.Python when compiling:
g++ -shared -o example.so example.cpp -I/usr/include/python3.x -lboost_python3.x
-
Python Usage
In Python, you can now easily import and use the C++ functionality:
import example result = example.add(3.0, 4.0) print("Result:", result) # Output: Result: 7.0
Using pybind11
What is pybind11?
pybind11 is a lightweight header-only library that exposes C++ types in Python and vice versa, with a focus on simple syntax and easy integration. It supports modern C++ features and is capable of interfacing seamlessly with Python.
Step-by-Step Example
-
Creating a pybind11 Module
Here's a basic example of a function wrapped in pybind11:
// example.cpp #include <pybind11/pybind11.h> double add(double a, double b) { return a + b; } PYBIND11_MODULE(example, m) { m.def("add", &add, "A function which adds two numbers"); }
-
Compiling and Linking pybind11 Code
You typically need to prepare a `CMakeLists.txt` file for configuration. A basic CMake setup might look like this:
cmake_minimum_required(VERSION 3.4) project(example) add_subdirectory(pybind11) pybind11_add_module(example example.cpp)
-
Using pybind11 in Python
After compiling your code with CMake, you can use it in Python just as you had with the other methods:
import example result = example.add(3.0, 4.0) print("Result:", result) # Output: Result: 7.0
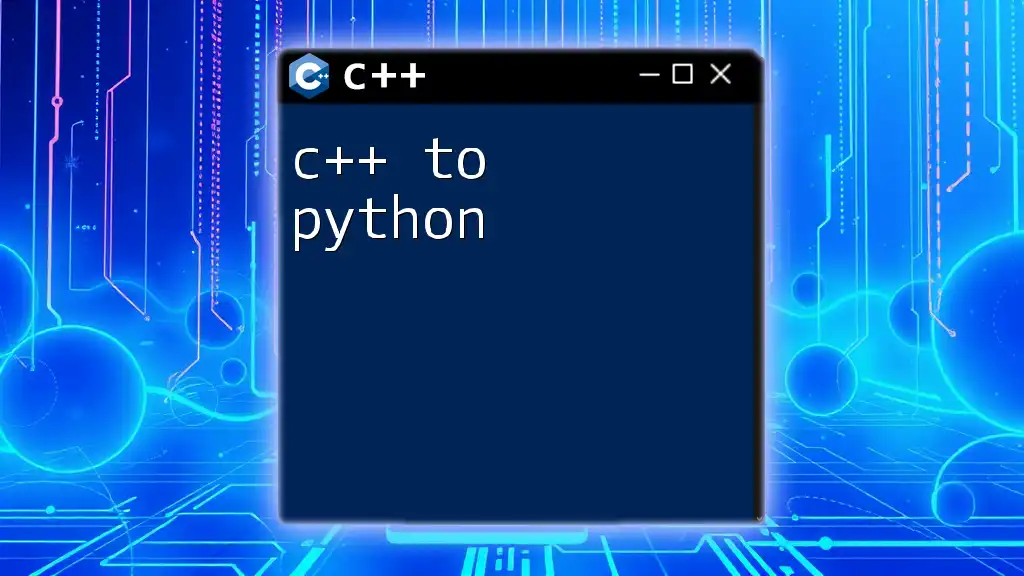
Best Practices for C++ and Python Interoperability
Managing Memory Efficiently
When integrating C++ with Python, understanding memory management is crucial. Python uses reference counting and garbage collection, while C++ uses manual memory management. Important: Always be mindful of ownership and lifetime of objects that cross language boundaries to prevent memory leaks or segmentation faults.
Error Handling
Cross-language error handling can be complex. It's recommended to translate C++ exceptions into Python exceptions when errors occur in your C++ code to maintain the stability of your Python application. Use try/catch blocks effectively in both languages to manage and debug errors.
Code Organization
To streamline the integration process, keep your C++ code modular. This modularity can simplify the interfacing process and enhance maintainability. Example: Maintain a clear directory structure separating the Python scripts, C++ source files, and build configurations.
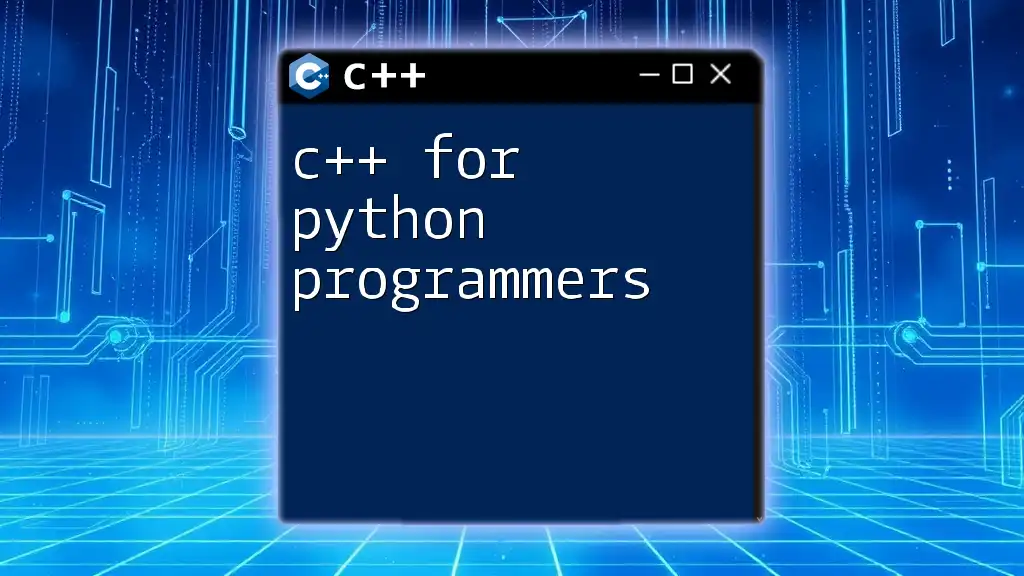
Conclusion
In this comprehensive guide, we've explored various methods and techniques to call C++ from Python. Whether you're using ctypes, Cython, Boost.Python, or pybind11, each approach offers unique advantages suited to different development needs. Experiment with these methodologies to discover how the performance and functionality of C++ can significantly enhance your Python applications.