C++ generally offers superior performance over Python due to its compiled nature and closer proximity to hardware, making it more efficient for resource-intensive applications.
#include <iostream>
#include <vector>
#include <chrono>
int main() {
std::vector<int> numbers(1e6);
for (int i = 0; i < 1e6; ++i) numbers[i] = i;
auto start = std::chrono::high_resolution_clock::now();
long long sum = 0;
for (int i = 0; i < 1e6; ++i) sum += numbers[i];
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Sum: " << sum << "\n";
std::cout << "Elapsed time: "
<< std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count()
<< " ms\n";
return 0;
}
Understanding the Basics of C++ and Python
Overview of C++
C++ is a general-purpose programming language that is widely recognized for its performance and efficient memory management capabilities. It was developed as an extension of the C programming language, enabling both high-level and low-level programming.
Key Advantages of C++ include:
- High performance: C++ compiles to machine code, allowing it to execute complex algorithms faster than many interpreted languages.
- Low-level memory manipulation: C++ provides pointers and direct memory access, giving programmers finer control over system resources.
- Object-oriented features: This allows for better code organization and reuse through concepts such as inheritance, polymorphism, and encapsulation.
Common Use Cases for C++ encompass game development, systems programming, real-time simulation, and performance-intensive applications.
Overview of Python
Python, on the other hand, is a high-level programming language known for its simplicity and readability. It prioritizes developer productivity and code clarity, making it an excellent choice for beginners and experienced developers alike.
Key Advantages of Python include:
- Readability and simplicity: Its syntax is clean and straightforward, allowing developers to express concepts in fewer lines of code.
- Extensive standard libraries: These libraries simplify tasks in web development, data analysis, machine learning, and more.
- Rapid development time: Python's dynamic nature allows developers to create prototypes quickly.
Common Use Cases for Python involve web applications, data science, automation, and machine learning.

Comparing Performance: C++ vs Python
Performance Metrics to Consider
When comparing C++ vs Python performance, various performance metrics come into play:
- Execution Speed: How quickly a program runs can determine its suitability for particular applications, especially in environments where time is critical.
- Memory Usage: Efficient memory allocation and management can greatly affect performance, especially in resource-constrained environments.
- Compilation vs Interpretation: The difference between compiled languages like C++ and interpreted languages like Python plays a vital role in performance.
Python Performance vs C++
One of the most significant factors in the Python performance vs C++ debate is the distinction between interpreted and compiled languages.
- Interpreted Languages vs Compiled Languages:
- Interpreted languages (like Python) are executed line-by-line, which can significantly slow down execution time, especially for large computations.
- Compiled languages (like C++) are transformed into machine code before execution, allowing efficient execution of programs.
Real-World Speed Comparisons
To illustrate the C++ vs Python speed difference, consider the following code examples, which both loop through one million iterations.
C++ Example Code Snippet:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 1000000; i++) {}
return 0;
}
Python Example Code Snippet:
for i in range(1000000):
pass
When executed, the C++ code significantly outperforms its Python counterpart. While the C++ loops run in a fraction of a second, the Python loop takes more time due to its interpreted nature. This demonstrates why C++ is often preferred for performance-critical tasks.
C++ vs Python Speed: Why C++ is Often Faster
Several inherent properties of C++ contribute to its superior execution speed:
- Compilation Time vs Execution Time: C++ transforms the whole program into machine code before execute, which can lead to longer compilation times but results in faster execution.
- Use of Static Typing: C++ uses static types, allowing for more efficient use of resources and enabling the compiler to perform optimizations that are not possible in dynamic languages like Python.

Pros and Cons of C++ and Python Performance
Advantages of C++
C++ offers several advantages that enhance its performance capabilities:
- Better memory management: Programmers can allocate and free memory manually, resulting in highly efficient memory usage that fits the specific needs of a program.
- Higher speed in execution: C++ is optimized for speed and performance, which is imperative in applications requiring real-time responses, such as gaming or simulation software.
- Utilization in system programming and game development: C++ is commonly used in scenarios where hardware interaction and speed are crucial.
Advantages of Python
Despite the performance differences, Python has its own set of advantages:
- Ease of use and learning curve: Its straightforward syntax allows beginners to grasp programming concepts quickly.
- Faster development cycle: Python's rapid prototyping capabilities make it suitable for projects with tight deadlines.
- Great for data analysis and scripting: Python's libraries like NumPy and pandas greatly enhance productivity in data-centric applications.
Disadvantages of C++
While C++ has many strengths, it isn't without drawbacks:
- Complex syntax: The intricacies of C++ can make it challenging to write and maintain code, especially for newcomers.
- Steeper learning curve: Mastering the nuances of C++ takes more time compared to more straightforward languages like Python.
- More prone to bugs due to manual memory management: Programmers can inadvertently introduce memory-related errors, such as leaks or corruptions, which can be hard to debug.
Disadvantages of Python
Python also has limitations:
- Slower execution speed: Its interpreted nature inevitably leads to slower performance compared to compiled languages.
- Greater memory consumption: Python tends to use more memory, which can be a constraint in memory-sensitive applications.
- Less control over hardware level operations: Python abstracts many low-level operations, which can limit performance tuning.
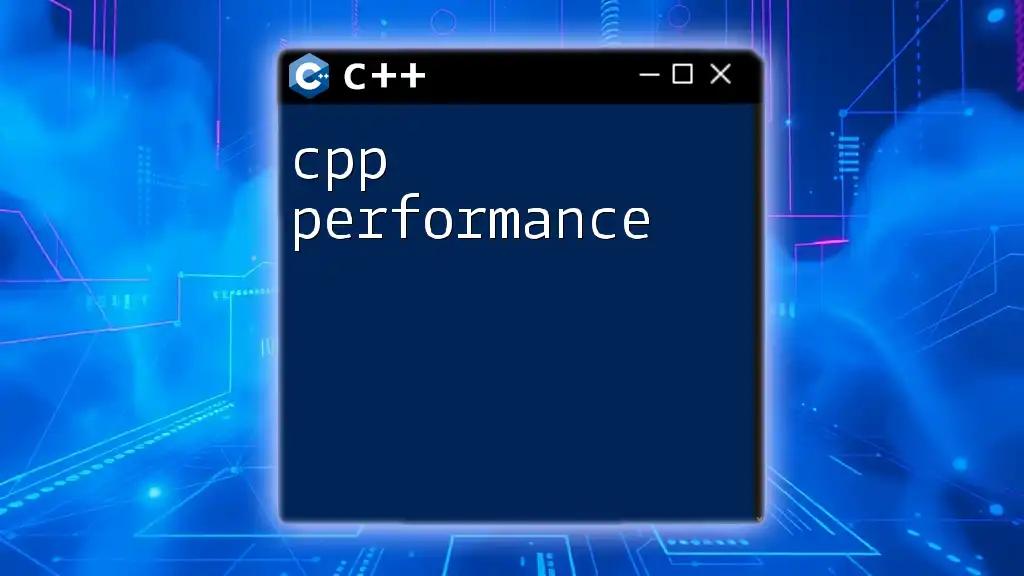
Optimizing Performance in Both Languages
C++ Performance Optimization Techniques
To maximize performance in C++, consider employing the following optimization techniques:
- Using efficient algorithms and data structures: Choosing the right algorithm is crucial. Data structures like vectors or maps can significantly influence performance.
- In-line functions: Utilizing inline functions can reduce the overhead of function calls, particularly in performance-critical loops.
Python Performance Optimization Techniques
Python programmers can also implement performance optimizations:
- Using built-in functions and libraries: Python’s built-in features are usually optimized; using them can result in better performance compared to writing custom code.
- Leveraging Just-In-Time (JIT) compilation with tools like PyPy: JIT compilation can dramatically speed up Python execution, making it comparable to C++ in some cases.
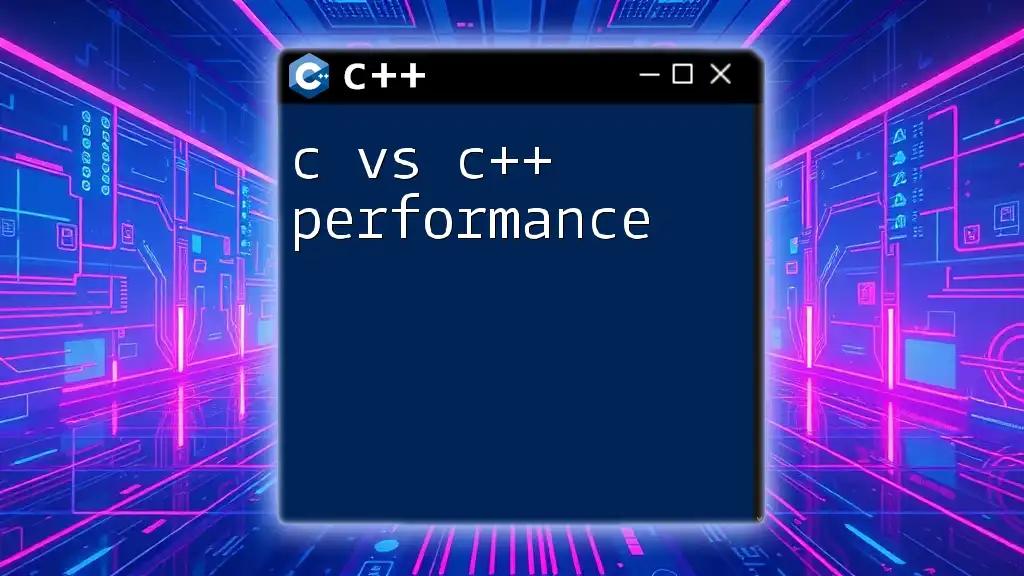
Conclusion: When to Choose C++ or Python?
Summary of Performance Differences
When evaluating C++ vs Python performance, the clear disparity in execution speed and memory management capabilities highlights why C++ tends to dominate in performance-intensive environments. However, Python's ease of use, rapid development capabilities, and vast library support make it an excellent choice for many types of applications.
Choosing the Right Language for Your Project
Ultimately, the decision between C++ and Python should consider several factors:
- Project requirements: If the project relies heavily on performance and resource management, C++ may be the best choice.
- Team expertise: The existing skills and familiarity of your team with either language can significantly affect the success of the project.
In conclusion, both C++ and Python have their unique advantages and disadvantages. The choice ultimately depends on the specific context and requirements of your work, as well as the flexibility and strengths of your development team.