C++ and Python are both powerful programming languages, but they differ in syntax and complexity, with Python generally offering a more concise and easier-to-read format for similar tasks.
Here's a simple example that demonstrates how to define a function in both languages:
// C++ function to add two numbers
int add(int a, int b) {
return a + b;
}
# Python function to add two numbers
def add(a, b):
return a + b
Understanding the Basics of C++ and Python
The Syntax Difference
When transitioning from C++ to Python, the first noticeable change is the syntax. Python is renowned for its simplicity and readability, making it an excellent choice for beginners and experienced developers alike. For example, consider the command to print "Hello, World!" in both languages:
// C++
std::cout << "Hello, World!" << std::endl;
# Python
print("Hello, World!")
In C++, you need to include libraries and specify constructs like `std::cout`, while in Python, a simple `print` function suffices, showcasing Python's streamlined syntax.
Data Types Comparison
Data types are fundamental concepts in programming, and both C++ and Python have distinct ways of handling them. Here’s a quick comparison of common data types:
C++ Data Type | Python Equivalent |
---|---|
int | int |
float | float |
char | str (single char) |
bool | bool |
string | str |
Notably, Python is dynamically typed, meaning you don't have to declare a variable's type beforehand, unlike C++, which is statically typed.
Control Structures
Control structures allow you to dictate the flow of your program. Let's take a closer look at conditional statements in both languages.
Conditional Statements
In C++, if-else statements look like this:
// C++
int x = 10;
if (x > 0) {
std::cout << "Positive";
} else {
std::cout << "Non-Positive";
}
In Python, this translates to:
# Python
x = 10
if x > 0:
print("Positive")
else:
print("Non-Positive")
As you can see, Python's syntax is more concise and less cluttered, making it easier to read and write.
Loops
Looping structures are similarly straightforward across both languages. A simple for loop in C++:
// C++
for (int i = 0; i < 5; i++) {
std::cout << i;
}
In contrast, the equivalent Python code is:
# Python
for i in range(5):
print(i)
The `range` function in Python automatically simplifies the initialization and condition checks that are necessary in C++.

Object-Oriented Programming in C++ vs. Python
Class and Object Definitions
Both languages support Object-Oriented Programming (OOP), but their syntaxes differ significantly. Here’s how you define a simple class in C++:
// C++
class Dog {
public:
void bark() { std::cout << "Woof!"; }
};
In Python, the equivalent definition looks like this:
# Python
class Dog:
def bark(self):
print("Woof!")
Note that Python uses `self` to reference the instance of the class when defining methods, while C++ inherently knows the context.
Inheritance and Polymorphism
Inheritance allows you to create a new class from an existing class. Here’s how it’s done in C++:
// C++
class Animal {};
class Dog : public Animal {};
And Python achieves the same functionality with:
# Python
class Animal:
pass
class Dog(Animal):
pass
The simplicity of Python's inheritance model can make it more intuitive, especially for those new to OOP.

Libraries and Modules
Standard Libraries
C++ and Python provide extensive libraries to facilitate various tasks. C++ has the Standard Template Library (STL), while Python boasts a massive range of built-in libraries like `os`, `sys`, and many specialized modules with straightforward installation through pip.
Third-Party Libraries
In Python, you have access to numerous third-party libraries that enhance functionality, such as NumPy for numerical computations, Pandas for data manipulation, and Flask for web development. Installing a library in Python is as simple as running:
pip install library_name
In C++, integrating third-party libraries often involves more steps, such as linking and configuring build paths.

Error Handling and Exceptions
Exception Handling in C++ and Python
Both languages handle errors through exception handling but with different syntax. Here’s how a typical exception handling looks in C++:
// C++
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << e.what();
}
In Python, the equivalent would be:
# Python
try:
raise Exception("Error occurred")
except Exception as e:
print(e)
Python’s exception handling constructs are often simpler and can handle multiple exceptions with one `except` clause.

Performance Considerations
When it comes to performance, C++ tends to excel in situations requiring high efficiency, such as game development and systems programming. Its compiled nature allows for faster execution compared to Python, which is interpreted. However, Python shines in rapid application development and is often preferred for scripting and data analysis due to its simplicity and the vast array of libraries available.

Conclusion
Transitioning from C++ to Python is a journey filled with discoveries, as you learn new paradigms and simplify your workflow. Both languages have their strengths and weaknesses, and understanding their differences can significantly enhance your programming skills. Armed with the concepts outlined above, you're better equipped to leverage both languages in your programming endeavors. Dive into the vast resources available and embrace the challenges ahead!
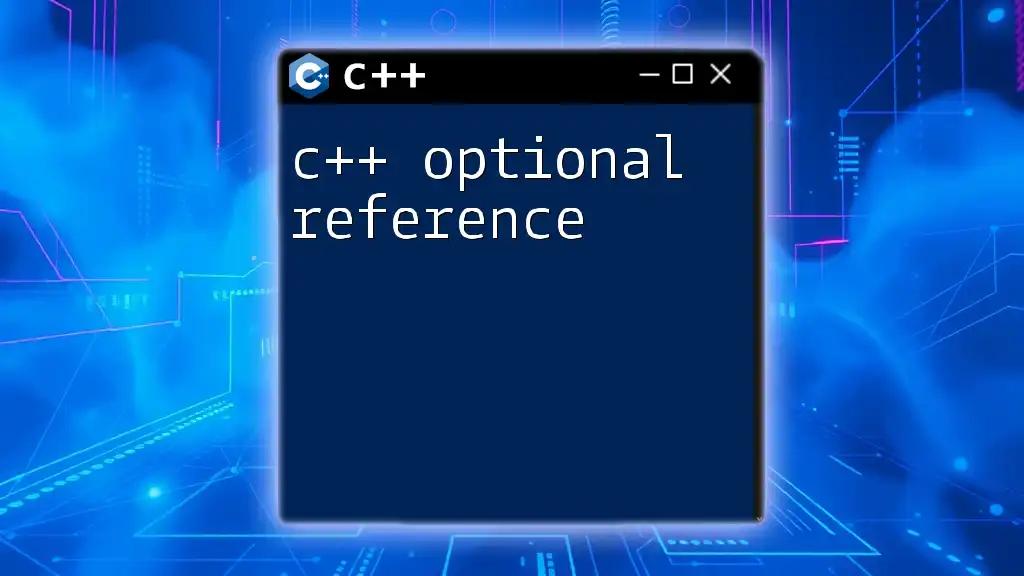
Further References
For more in-depth knowledge, consider exploring official documentation and tutorials for C++ and Python, such as:
- [C++ Documentation](https://en.cppreference.com/w/)
- [Python Documentation](https://docs.python.org/3/)
- [Online Coding Platforms for Practice](https://www.codecademy.com/)
- [Comprehensive Programming Communities](https://stackoverflow.com/)
Happy coding!