A C++ to C converter is a tool or program that translates C++ code into its equivalent C code, allowing developers to leverage the strengths of both programming languages.
// Example: C++ code
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
// Equivalent C code
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Understanding the Differences Between C++ and C
Key Features of C++
C++ is a powerful programming language that incorporates a range of features catering to complex software development needs. Some of the key features include:
- Object-oriented programming concepts: C++ supports the core principles of object-oriented programming (OOP) such as encapsulation, inheritance, and polymorphism.
- Exception handling: It allows developers to handle runtime errors gracefully, improving program robustness.
- Function overloading: C++ enables multiple functions to share the same name but differ in parameters, promoting code clarity and reusability.
- Namespaces: This feature helps in preventing naming conflicts in large projects by grouping identifiers.
Key Features of C
C, on the other hand, is often regarded as a lower-level programming language focused on simplicity and efficiency. Its key features include:
- Procedural programming: C emphasizes a procedural approach, making it straightforward for routine tasks and system-level programming.
- Simplicity and speed: C is known for its minimalistic syntax and fast execution, which makes it ideal for performance-critical applications.
- Lack of built-in support for classes and objects: Unlike C++, C does not natively support object-oriented concepts.
Comparing Syntax and Structure
When comparing syntax and structure, C++ allows for a more complex and organized approach to programming compared to C’s simplicity.
Example Snippet - C++ Code:
#include <iostream>
class Example {
public:
int value;
void show() {
std::cout << "Value: " << value << std::endl;
}
};
Equivalent C Code:
#include <stdio.h>
struct Example {
int value;
};
void show(struct Example ex) {
printf("Value: %d\n", ex.value);
}
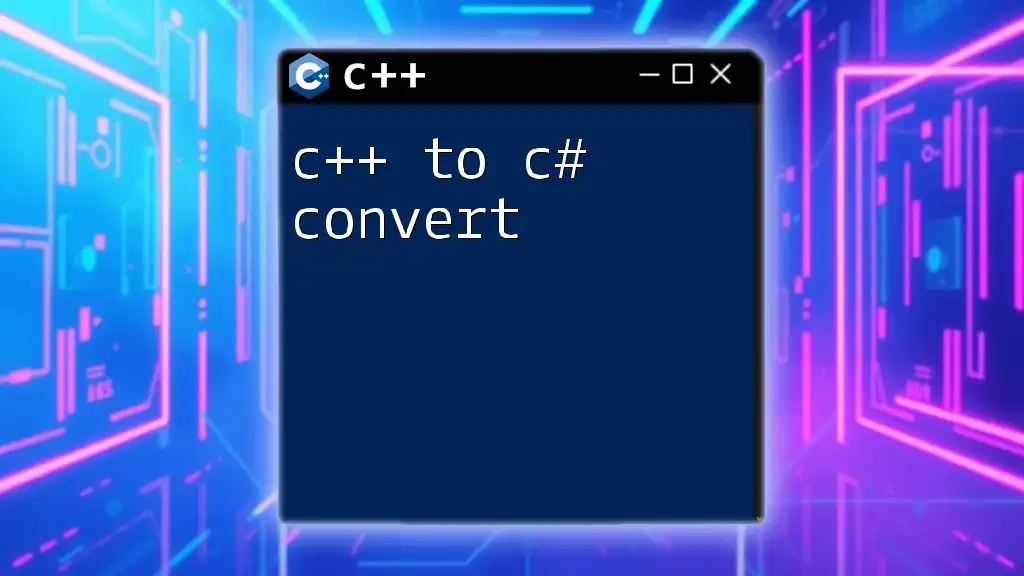
Why Convert from C++ to C?
Performance Considerations
C offers better performance in certain scenarios, particularly in systems programming where low-level memory management and quick execution are crucial. The tighter control over resources often results in optimized applications, which is particularly important for performance-sensitive areas like embedded systems.
Compatibility Issues
Legacy systems may not support C++, necessitating a conversion to C to ensure compatibility. Additionally, cross-platform support can be simpler with C, given its long-standing presence in various operating systems.
Simplicity and Maintainability
For applications that do not require the advanced features of C++, converting to C can significantly simplify the codebase. By utilizing procedural programming paradigms, developers may find it easier to understand and maintain the code.
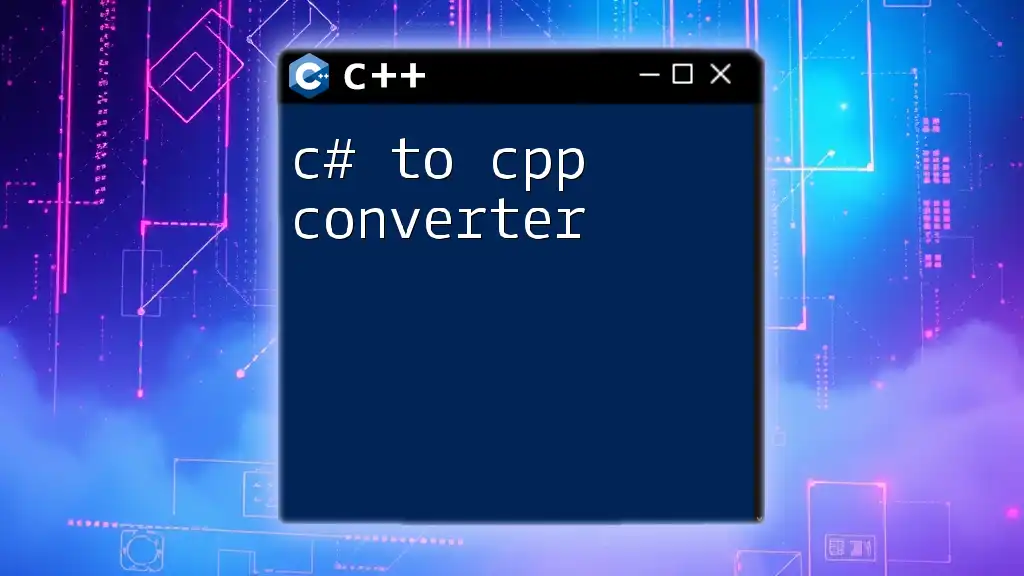
Tools for C++ to C Conversion
Automated Conversion Tools
Several automated tools can assist in converting C++ code to C, simplifying the process dramatically. These tools often provide quick solutions but come with limitations regarding complex C++ features.
Example Tools and Their Features
- Tool 1: Offers basic conversion features and handles standard syntax efficiently but may struggle with more advanced C++ concepts like templates.
- Tool 2: Focuses on maintaining code structure but may require manual intervention for complex constructs.
- Tool 3: A more robust solution that supports a wider array of C++ features, though it may result in less readable C code.
Manual Conversion
While tools can help, manual conversion allows for greater control and understanding of the translated code.
Detailed Steps for Manual Conversion
- Identifying Object-oriented Features: Recognize classes, inheritance, and other OOP constructs in your C++ code.
- Replacing Classes with Structures: Convert each class into a struct, ensuring that member variables are defined.
- Handling Function Pointers: Functions must be converted into standard function pointers where applicable.
- Code Snippets Illustrating Manual Conversion Techniques:
- C++ Classes with Inheritance:
class Base { public: void display() {} }; class Derived : public Base {};
- Converted C Code:
struct Base { void (*display)(); }; struct Derived { struct Base base; };
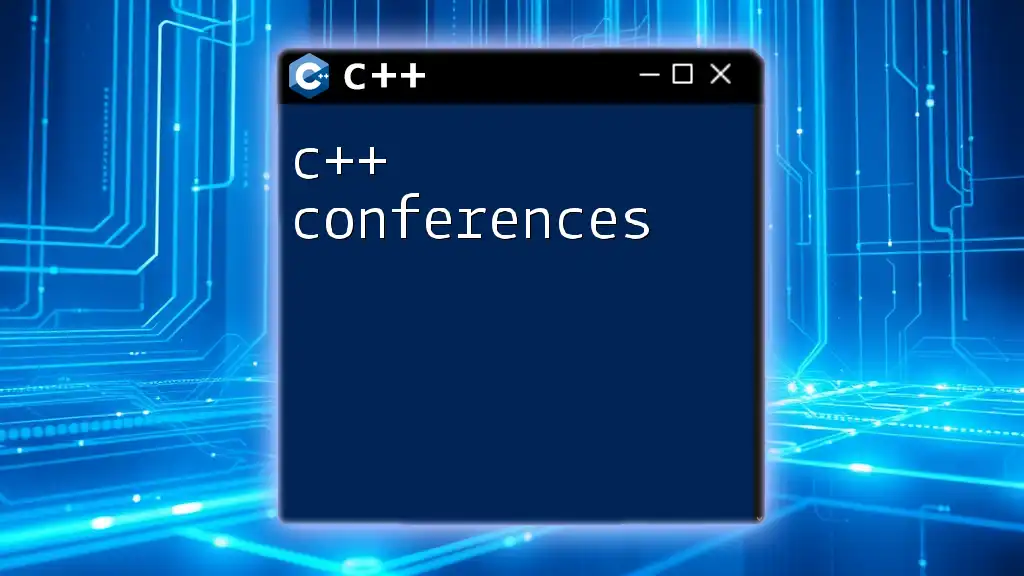
Step-by-Step Guide to Convert C++ Code to C
Before initiating the conversion, evaluate the existing code for complexities and dependencies that could influence the process.
Handling Classes and Objects
Transitioning from classes to `structs` is one of the primary tasks. This involves ensuring that all member functions of the class are converted into standard C functions that interact with the struct.
Example:
class Sample {
public:
int data;
void display() {
printf("%d", data);
}
};
Converted to C:
struct Sample {
int data;
};
void display(struct Sample s) {
printf("%d", s.data);
}
Managing Inheritance and Polymorphism
C does not support inheritance directly. Instead, you can simulate inheritance by embedding structures. This allows you to achieve a similar hierarchy of functionality.
Conversion of Templates and Standard Libraries
Templates in C++ do not have a direct counterpart in C; thus, alternative solutions, such as using macros or pre-defined structures, must be utilized. This requires a more in-depth rework of the logic that uses templates.
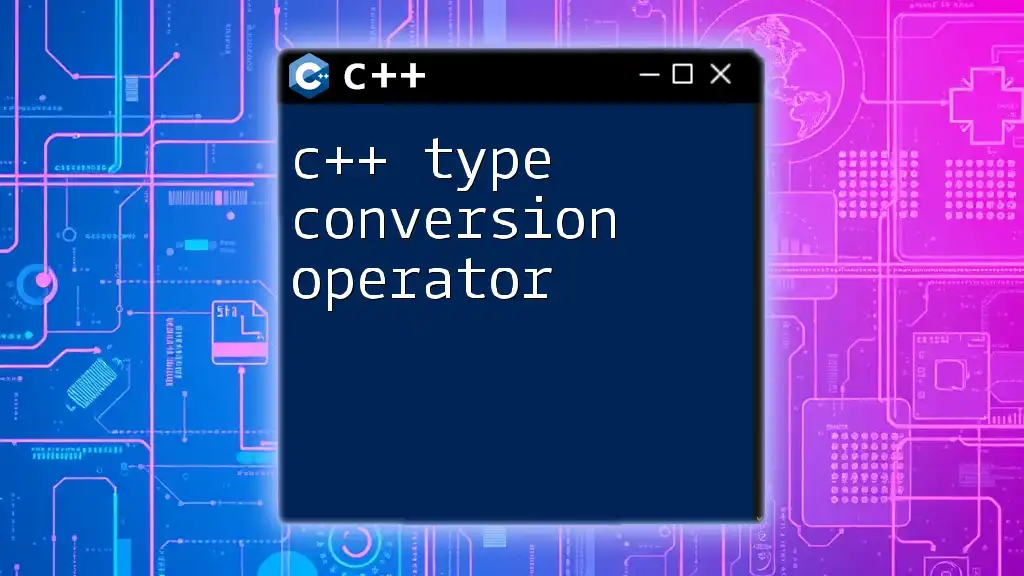
Common Pitfalls in C++ to C Conversion
Data Type Differences
Different data types between C and C++ can lead to complications. C’s simpler type system may require adjustments to maintain compatibility with C++ data structures.
Memory Management
C lacks the automatic memory management features present in C++, demanding stricter adherence to manual memory management. This means developers must be vigilant to prevent memory leaks and corruption.
Error Handling
Transitioning from exceptions to values or error codes in C may necessitate a complete rewrite of how errors are managed and reported in your original C++ code.
Maintaining Performance
Care must be taken to ensure that the C code retains performance levels comparable to the C++ version. This often involves profiling both versions and optimizing the C code as needed.
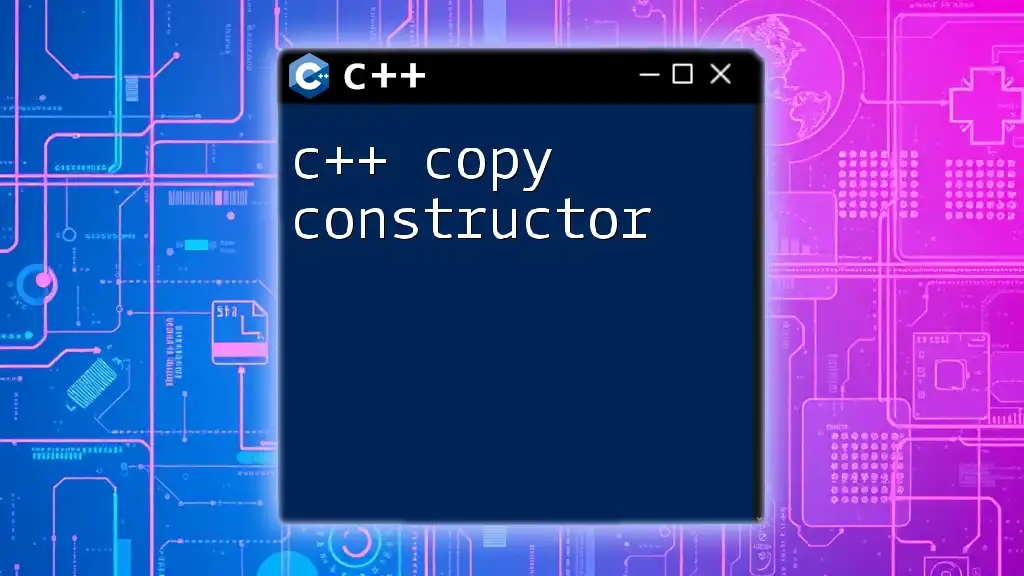
Testing the Converted Code
Importance of Testing
Testing is crucial when converting code to guarantee its operational integrity and performance. It's essential to validate functionality after any conversion to mitigate bugs and errors.
Strategies for Testing
Employ strategies such as unit tests and integration tests to evaluate both the functionality and integration points of the code. Utilizing established testing frameworks can streamline the process.
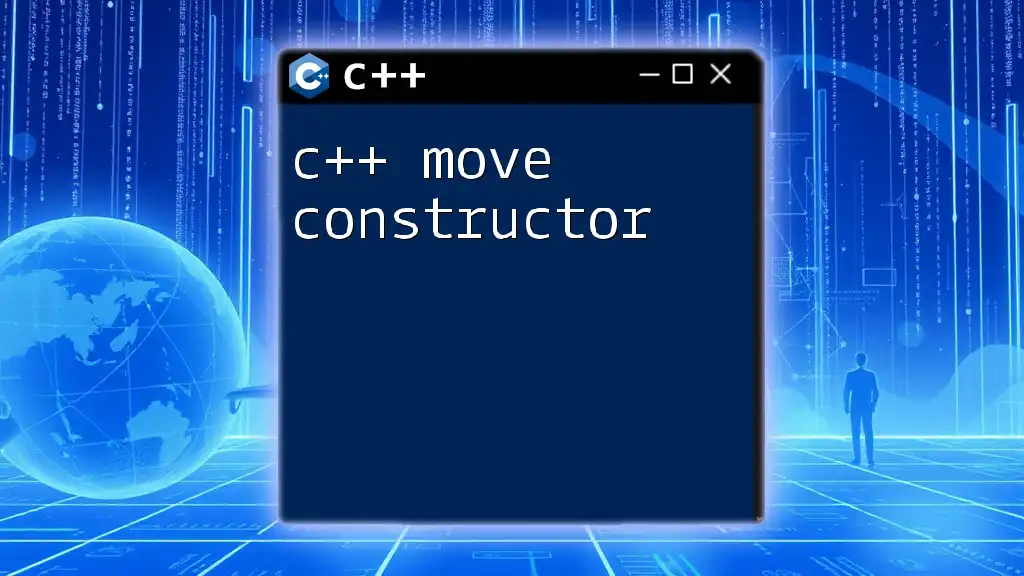
Conclusion
Converting C++ code to C can be a challenging yet rewarding endeavor. By understanding both languages and utilizing the right tools and techniques, developers can successfully transition their projects. This process can lead to increased performance, compatibility with legacy systems, and improved maintainability for certain applications.
In conclusion, embracing both C and C++ offers expansive possibilities for software development. Engage with our training programs to enhance your skills and deepen your understanding of these essential languages.